#include <iostream>
#include <memory>
#include <list>
#include <vector>
using namespace std;
记录一下unique_ptr的用法
class CBase
{
public:
CBase(){printf("Base create..\r\n");}
virtual ~CBase(){printf("Base delete\r\n");}
virtual void PrintInfo(){printf("Base class print..\r\n");}
};
class CFather : public CBase
{
public:
CFather(){printf("Father create..\r\n");}
~CFather(){printf("Father delete..\r\n");}
virtual void PrintInfo() override {printf("Father class print..\r\n");}
};
class CMother : public CBase
{
public:
CMother(){printf("Mother create..\r\n");}
~CMother(){printf("Mother delete..\r\n");}
virtual void PrintInfo() override {printf("Mother class print..\r\n");}
};
int main()
{
int flag = 5;
while(flag --)
{
/*设置数组*/
std::vector<std::unique_ptr<CBase>> vecBase;
for(int i = 0;i<4;i++)
{
std::unique_ptr<CBase> base;
/*交替压入father,mother类*/
if(i % 2 == 0)
vecBase.push_back(std::unique_ptr<CBase>(new CFather));
else
vecBase.push_back(std::unique_ptr<CBase>(new CMother));
}
std::vector<std::unique_ptr<CBase>>::iterator iter = vecBase.begin();
for(;iter != vecBase.end();iter++)
{
/*多态打印*/
(*iter)->PrintInfo();
}
}
return 0;
}
C++11 std::unique_ptr
最新推荐文章于 2024-06-01 21:31:08 发布
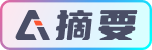