using SolrNet.Impl;
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Wrox6
{
//泛型类
//先创建一个文档管理器
public class DocumentManager<T>
{
private readonly Queue<T> _documentQueue = new Queue<T>();
private readonly object _lockQueue = new object();
public void AddDocument(T doc)
{
lock (_lockQueue)
{
_documentQueue.Enqueue(doc);
}
}
public bool IsDocumentAvailable => _documentQueue.Count > 0;
//泛型类型可以实例化为值类型,但是null只能引用类型,为解决这个问题,可以使用default关键字
public T GetDocument()
{
T doc = default;//通过default关键字,将null赋予引用类型,将0赋予值类型
lock (_lockQueue)
{
doc = _documentQueue.Dequeue();
}
return doc;
}
//如果泛型类需要调用泛型类型中的方法,就必须添加约束
public interface IDocument
{
string Title { get; }
string Content { get; }
}
public class Document: IDocument
{
public Document(string title, string content)
{
title = title;
content = content;
}
public string Title { get; }
public string Content { get; }
}
//文档的所有标题应在DisplayAllDocuments()方法中显示,Document类实现带有Title和Content只读属性的IDocument接口
public void DisplayAllDocuments()
{
foreach(T doc in _documentQueue)
{
Console.WriteLine(((IDocument)doc).Title);
}
}
//如果类型T没有实现IDocument接口,这时就要给DocumentManager<TDocument>类定义一个约束:TDocument类型必须实现IDocument接口
public class DocumentManager1<TDocument>
//给泛型类型添加约束时,最好包含泛型参数名称的一些信息
where TDocument : IDocument
{
private IEnumerable<TDocument> documentQueue;
//编写foreach语句,从而使类型TDocument包含属性Title
public void DisplayAllDocument()
{
foreach (TDocument doc in documentQueue)
{
Console.WriteLine(doc.Title);
}
}
}
//在main方法中,用Document类型实例化DocumentManager<TDocument>类,而Document类型实现了需要的IDocument接口,接着添加和显示文档,检索其中一个文档
public static void Main()
{
var dm = new DocumentManager<Document>();
dm.AddDocument(new Document("Title A", "Sample A"));
dm.AddDocument(new Document("Title B", "Sample B"));
if (dm.IsDocumentAvailable)
{
Document document = dm.GetDocument();
Console.WriteLine(document.Title);
}
}
//泛型支持的约束类型
//where T.struct 对于结构约束,类型T必须是值类型
//where T.class 类约束指定类型T必须是引用类型
//where T.IFoo 指定类型T必须实现接口IFoo
//where T.new() 这是一个构造函数约束,指定类型T必须有一个默认构造函数
//where T1.T2 这个约束也可以指定,类型T1派生自泛型类型T2
public class MyClass<T>
where T : IFoo, new()
{
//
}
//合并多个约束,类型T必须实现IFoo接口,且必须有一个默认构造函数
//where子句限制:不能定义必须由泛型类型实现的运算符,运算符也不能在接口中定义,
//只能定义基类,接口和默认构造函数
//继承
//创建LinkList<T>类实现IEnumerable<T>接口
public class LinkList<T>:IEnumerable<T>
{
public LinkList() { }
public IEnumerator<T> GetEnumerator()
{
throw new NotImplementedException();
}
IEnumerator IEnumerable.GetEnumerator()
{
throw new NotImplementedException();
}
}
//可以实现泛型接口,也可以派生自一个类
public class Base<t>
{
}
public class Derived<T>:Base<T>
{
}
//必须是重复接口的泛型类型,或者必须指定基类的类型
public class Base1<T>
{
}
public class Derived1<T>: Base1<string>
{
}
//泛型类的静态成员只能在类的一个实例中共享
public class StaticDemo<T>
{
public static int x;
}
//StaticDemo<string>.x=4;
//StaticDemo<int>.x=4;
//下回-泛型接口
}
public interface IFoo
{
}
}
C#基础-8
最新推荐文章于 2024-08-09 11:35:57 发布
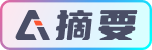