文章目录
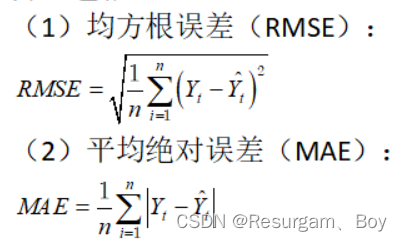
解读math_utils.py
import numpy as np
z_score()函数
def z_score(x, mean, std):
'''
Z-score normalization function: $z = (X - \mu) / \sigma $,
where z is the z-score, X is the value of the element,
$\mu$ is the population mean, and $\sigma$ is the standard deviation.
:param x: np.ndarray, input array to be normalized.
:param mean: float, the value of mean.
:param std: float, the value of standard deviation.
:return: np.ndarray, z-score normalized array.
'''
return (x - mean) / std
z_score 函数
该函数用于执行Z-score标准化,将数据转化为均值为0和标准差为1的形式。
- 参数:
x
: 输入的numpy数组。mean
: 均值。std
: 标准差。
- 返回值: 经过Z-score标准化后的数组。
z_inverse()函数
def z_inverse(x, mean, std):
'''
The inverse of function z_score().
:param x: np.ndarray, input to be recovered.
:param mean: float, the value of mean.
:param std: float, the value of standard deviation.
:return: np.ndarray, z-score inverse array.
'''
return x * std + mean
z_inverse 函数
这个函数是z_score函数的逆操作,用于恢复被标准化的数据。
- 参数和返回值与z_score类似。
MAPE()函数
def MAPE(v, v_):
'''
Mean absolute percentage error.
:param v: np.ndarray or int, ground truth.
:param v_: np.ndarray or int, prediction.
:return: int, MAPE averages on all elements of input.
'''
return np.mean(np.abs(v_ - v) / (v + 1e-5))
MAPE 函数
该函数用于计算平均绝对百分比误差(Mean Absolute Percentage Error)。
- 参数:
v
: 真实值。v_
: 预测值。
- 返回值: MAPE值。
RMSE()函数
def RMSE(v, v_):
'''
Mean squared error.
:param v: np.ndarray or int, ground truth.
:param v_: np.ndarray or int, prediction.
:return: int, RMSE averages on all elements of input.
'''
return np.sqrt(np.mean((v_ - v) ** 2))
RMSE 函数
该函数用于计算均方根误差(Root Mean Square Error)。
- 参数与MAPE类似。
- 返回值: RMSE值。
MAE()函数
def MAE(v, v_):
'''
Mean absolute error.
:param v: np.ndarray or int, ground truth.
:param v_: np.ndarray or int, prediction.
:return: int, MAE averages on all elements of input.
'''
return np.mean(np.abs(v_ - v))
MAE 函数
该函数用于计算平均绝对误差(Mean Absolute Error)。
- 参数与MAPE类似。
- 返回值: MAE值。
evaluation()函数
def evaluation(y, y_, x_stats):
'''
Evaluation function: interface to calculate MAPE, MAE and RMSE between ground truth and prediction.
Extended version: multi-step prediction can be calculated by self-calling.
:param y: np.ndarray or int, ground truth.
:param y_: np.ndarray or int, prediction.
:param x_stats: dict, paras of z-scores (mean & std).
:return: np.ndarray, averaged metric values.
'''
dim = len(y_.shape)
if dim == 3:
# single_step case
v = z_inverse(y, x_stats['mean'], x_stats['std'])
v_ = z_inverse(y_, x_stats['mean'], x_stats['std'])
return np.array([MAPE(v, v_), MAE(v, v_), RMSE(v, v_)])
else:
# multi_step case
tmp_list = []
# y -> [time_step, batch_size, n_route, 1]
y = np.swapaxes(y, 0, 1)
# recursively call
for i in range(y_.shape[0]):
tmp_res = evaluation(y[i], y_[i], x_stats)
tmp_list.append(tmp_res)
return np.concatenate(tmp_list, axis=-1)
evaluation 函数
这是一个评估函数,用于计算预测模型的性能。具体来说,它计算三种常用的误差指标:平均绝对百分比误差(MAPE)、平均绝对误差(MAE)和均方根误差(RMSE)。
函数参数:
y
: 这是模型预测的真实值(ground truth),可以是一个多维的Numpy数组或整数。y_
: 这是模型的预测值,其维度和类型应与y
相同。x_stats
: 这是一个字典,其中包含用于Z-score标准化的均值和标准差。
返回值:
- 函数返回一个Numpy数组,包含了MAPE、MAE和RMSE的值。
代码流程:
-
检查维度:
dim = len(y_.shape)
用于获取预测值y_
的维度。 -
单步预测 vs. 多步预测:根据
y_
的维度,函数会区分处理单步预测和多步预测。-
单步预测(dim==3):
- 使用
z_inverse
函数将标准化的y
和y_
逆转为原始尺度。 - 计算MAPE、MAE和RMSE。
- 返回一个包含这三个指标的Numpy数组。
- 使用
-
多步预测(dim!=3):
- 创建一个空列表
tmp_list
用于存储每一步的评估结果。 - 使用
np.swapaxes(y, 0, 1)
交换y
数组的轴以适应递归调用。 - 使用递归调用
evaluation
函数来评估每一个时间步,并将结果存储在tmp_list
中。 - 使用
np.concatenate
将所有时间步的结果合并,并返回。
- 创建一个空列表
-
总体而言,这个函数非常灵活,能够处理单步预测和多步预测,并为每种情况提供多种评估指标。这使得它成为评估时间序列预测模型性能的有力工具。