1.智能家居添加火灾检测模块
1.概述
火灾检测模块大致与灯光模块相同。
不同点:
火灾模块要读取引脚状态
pinMode要把output 改为input
不用打开和关闭
2.代码
1.contrlDevice.h
#include <wiringPi.h>
struct Devices{
char deviceName[32];
int status;
int pinNum;
int (*open)(int pinNum);
int (*close)(int pinNum);
int (*deviceInit)(int pinNum);
int (*readStatus)(int pinNum);
int (*changeStatus)(int status);
struct Devices *next;
};
struct Devices* addBathroomLightToDeviceLink(struct Devices *phead);
struct Devices* addUpstairLightToDeviceLink(struct Devices *phead);
struct Devices* addLivingroomLightToDeviceLink(struct Devices *phead);
struct Devices* addRestaurantLightToDeviceLink(struct Devices *phead);
struct Devices* addfireIfOrNotToDeviceLink(struct Devices *phead);
2…fire.c
#include <stdio.h>
#include "contrlDevice.h"
#include "inputCommand.h"
int fireIfOrNotInit(int pinNum){
pinMode(pinNum,INPUT);
digitalWrite(pinNum,HIGH);
}
int fireStatusRead(int pinNum){
return digitalRead(pinNum);
}
struct Devices fireIfOrNot={
.deviceName="fireIfOrNot",
.pinNum=6,
.deviceInit=fireIfOrNotInit,
.readStatus=fireStatusRead
};
struct Devices* addfireIfOrNotToDeviceLink(struct Devices *phead){
if(phead==NULL){
return &fireIfOrNot;
}else{
fireIfOrNot.next=phead;
phead=&fireIfOrNot;
return phead;
}
}
2.智能家居添加语音模块和socket模块
1.头文件inputCommand.h
#include <wiringSerial.h>
#include <unistd.h>
#include <stdlib.h>
#include <wiringPi.h>
struct InputCommander{
char commandName[128];
char deviceName[32];
char command[32];
int fd;
int sfd;
char port[12];
char ipAddress[32];
int (*Init)(struct InputCommander *voicer,char *ipAdress,char *port);
int (*getCommand)(struct InputCommander *voicer);
char log[1024];
struct InputCommander *next;
};
struct InputCommander *addVoiceContrlToInputCommandLink(struct InputCommander *phead);
struct InputCommander *addSocketContrlToInputCommandLink(struct InputCommander *phead);
2.语音模块
#include "inputCommand.h"
int voiceInit(struct InputCommander *voicer,char *ipAdress,char *port){
int fd=serialOpen(voicer->deviceName,115200);
if(fd == -1){
printf("open failed\n");
exit(-1);
}
voicer->fd=fd;
return fd;
}
int voiceGetCommand(struct InputCommander *voicer){
int nread=0;
nread=read(voicer->fd,voicer->command,sizeof(voicer->command));
if(nread==0){
printf("over time\n");
}
return nread;
}
struct InputCommander voiceContrl = {
.commandName="voice",
.deviceName="/dev/ttyS5",
.command={'\0'},
.Init=voiceInit,
.getCommand=voiceGetCommand,
.log={'\0'},
.next=NULL
};
struct InputCommander *addVoiceContrlToInputCommandLink(struct InputCommander *phead){
if(phead==NULL){
return &voiceContrl;
}
else{
voiceContrl.next=phead;
phead=&voiceContrl;
return phead;
}
}
3.socket模块
#include "inputCommand.h"
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <errno.h>
#include <stdlib.h>
#include <string.h>
int socketInit(struct InputCommander *socketMes,char *ipAdress,char *port){
struct sockaddr_in addr;
memset(&addr,0,sizeof(struct sockaddr_in));
int s_fd;
s_fd=socket(AF_INET,SOCK_STREAM,0);
if(s_fd==-1){
perror("socket");
exit(-1);
}
addr.sin_family=AF_INET;
addr.sin_port= htons(atoi(socketMes->port));
inet_aton(socketMes->ipAddress,&addr.sin_addr);
bind(s_fd,(struct sockaddr*)&addr,sizeof(struct sockaddr_in));
listen(s_fd,10);
socketMes->sfd=s_fd;
return s_fd;
}
int socketGetCommand(struct InputCommander *socketMes){
int c_fd;
int nread;
struct sockaddr_in addr1;
memset(&addr1,0,sizeof(struct sockaddr_in));
int size=sizeof(struct sockaddr_in);
c_fd=accept(socketMes->sfd,(struct sockaddr*)&addr1,&size);
if(c_fd==-1){
perror("accept");
}
printf("celinte IP:%s\n",inet_ntoa(addr1.sin_addr));
memset(socketMes->command,'\0',sizeof(socketMes->command));
nread=read(c_fd,socketMes->command,sizeof(socketMes->command));
if(nread<0){
perror("read");
}
else if(nread>0){
printf("read %d, %s\n",nread,socketMes->command);
}
else{
printf("client is quit\n");
}
return nread;
}
struct InputCommander socketContrl = {
.commandName="socketServer",
.command={'\0'},
.Init=socketInit,
.port="8088",
.ipAddress="172.20.10.4",
.getCommand=socketGetCommand,
.log={'\0'},
.next=NULL
};
struct InputCommander *addSocketContrlToInputCommandLink(struct InputCommander *phead){
if(phead==NULL){
return &socketContrl;
}
else{
socketContrl.next=phead;
phead=&socketContrl;
return phead;
}
}
3.编写主程序验证代码
#include <stdio.h>
#include <string.h>
#include "contrlDevice.h"
#include "inputCommand.h"
#include <wiringPi.h>
#include <pthread.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <errno.h>
struct Devices *pDeviceHead=NULL;
struct Devices *tmp=NULL;
struct InputCommander *pCommandHead=NULL;
struct InputCommander *socketHander=NULL;
int c_fd;
struct Devices *findDeviceByName(char *name,struct Devices *phead){
struct Devices *ptmp=phead;
if(ptmp==NULL){
printf("none\n");
return NULL;
}else{
while(ptmp!=NULL){
if(strcmp(ptmp->deviceName,name)==0){
return ptmp;
}
ptmp=ptmp->next;
}
return NULL;
}
}
struct InputCommander *findCommandByName(char *name,struct InputCommander*phead){
struct InputCommander*ptmp=phead;
if(ptmp==NULL){
printf("none\n");
return NULL;
}else{
while(ptmp!=NULL){
if(strcmp(ptmp->commandName,name)==0){
return ptmp;
}
ptmp=ptmp->next;
}
return NULL;
}
}
void *voice_Thread(void *datas){
int fd;
int n_read;
struct InputCommander *voiceHander=NULL;
voiceHander=findCommandByName("voice",pCommandHead);
if(voiceHander==NULL){
printf("find voiceHander error\n");
pthread_exit(NULL);
}
fd=voiceHander->Init(voiceHander,NULL,NULL);
if(fd < 0){
printf("voice init error\n");
pthread_exit(NULL);
}else{
printf("voice init success\n");
}
while(1){
n_read=voiceHander->getCommand(voiceHander);
if(n_read==0){
printf("not command\n");
}else{
printf("read %d bytes %s\n",n_read,voiceHander->command);
}
}
}
void *read_Thread(void *datas){
int n_read;
memset(socketHander->command,'\0',sizeof(socketHander->command));
n_read=read(c_fd,socketHander->command,sizeof(socketHander->command));
if(n_read<0){
perror("read");
}
else if(n_read>0){
printf("read %d, %s\n",n_read,socketHander->command);
}
else{
printf("client is quit\n");
}
}
void *socket_Thread(void *datas){
pthread_t readThread;
struct sockaddr_in addr1;
socketHander=findCommandByName("socketServer",pCommandHead);
if(socketHander==NULL){
printf("not find\n");
pthread_exit(NULL);
}
socketHander->Init(socketHander,NULL,NULL);
memset(&addr1,0,sizeof(struct sockaddr_in));
int size=sizeof(struct sockaddr_in);
while(1){
c_fd=accept(socketHander->sfd,(struct sockaddr*)&addr1,&size);
printf("celinte IP:%s\n",inet_ntoa(addr1.sin_addr));
pthread_create(&readThread,NULL,read_Thread,NULL);
}
}
int main(){
if(wiringPiSetup()==-1){
return -1;
}
char name[32]={'\0'};
pthread_t voiceThread;
pthread_t socketThread;
pDeviceHead=addBathroomLightToDeviceLink(pDeviceHead);
pDeviceHead=addUpstairLightToDeviceLink(pDeviceHead);
pDeviceHead=addLivingroomLightToDeviceLink(pDeviceHead);
pDeviceHead=addRestaurantLightToDeviceLink(pDeviceHead);
pDeviceHead=addfireIfOrNotToDeviceLink(pDeviceHead);
pCommandHead=addVoiceContrlToInputCommandLink(pCommandHead);
pCommandHead=addSocketContrlToInputCommandLink(pCommandHead);
pthread_create(&voiceThread,NULL,voice_Thread,NULL);
pthread_create(&socketThread,NULL,socket_Thread,NULL);
pthread_join(voiceThread,NULL);
pthread_join(socketThread,NULL);
return 0;
}
打开网络调试助手验证socket server是否可以接受数据
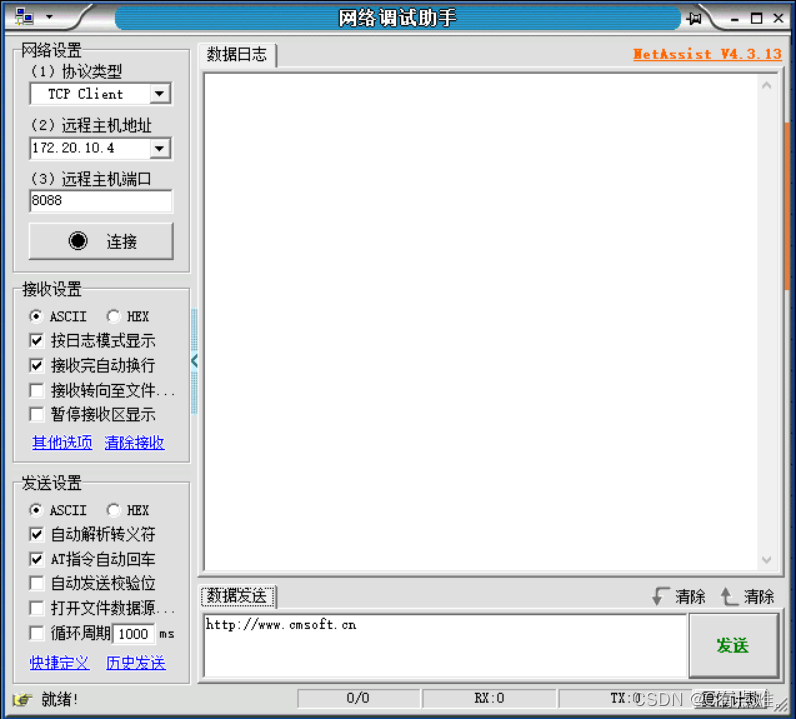