一、基础绘图
00 turtle库的用法
01 正方形
import turtle
for i in range(4):
turtle.forward(100)
turtle.right(90)
turtle.done()
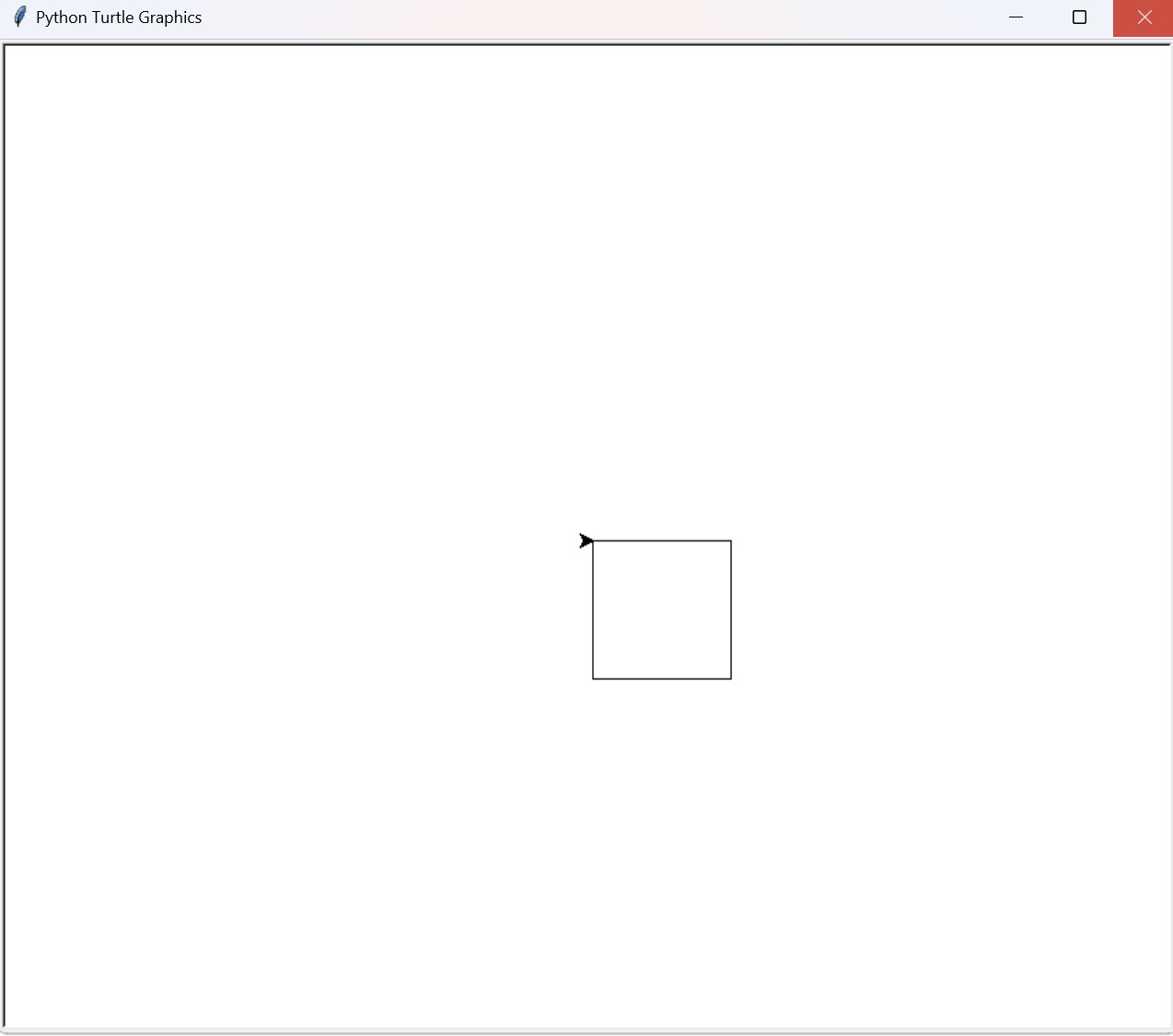
02 五角星
import turtle
turtle.left(72)
for i in range(5):
turtle.forward(100)
turtle.right(144)
turtle.forward(100)
turtle.left(72)
turtle.done()
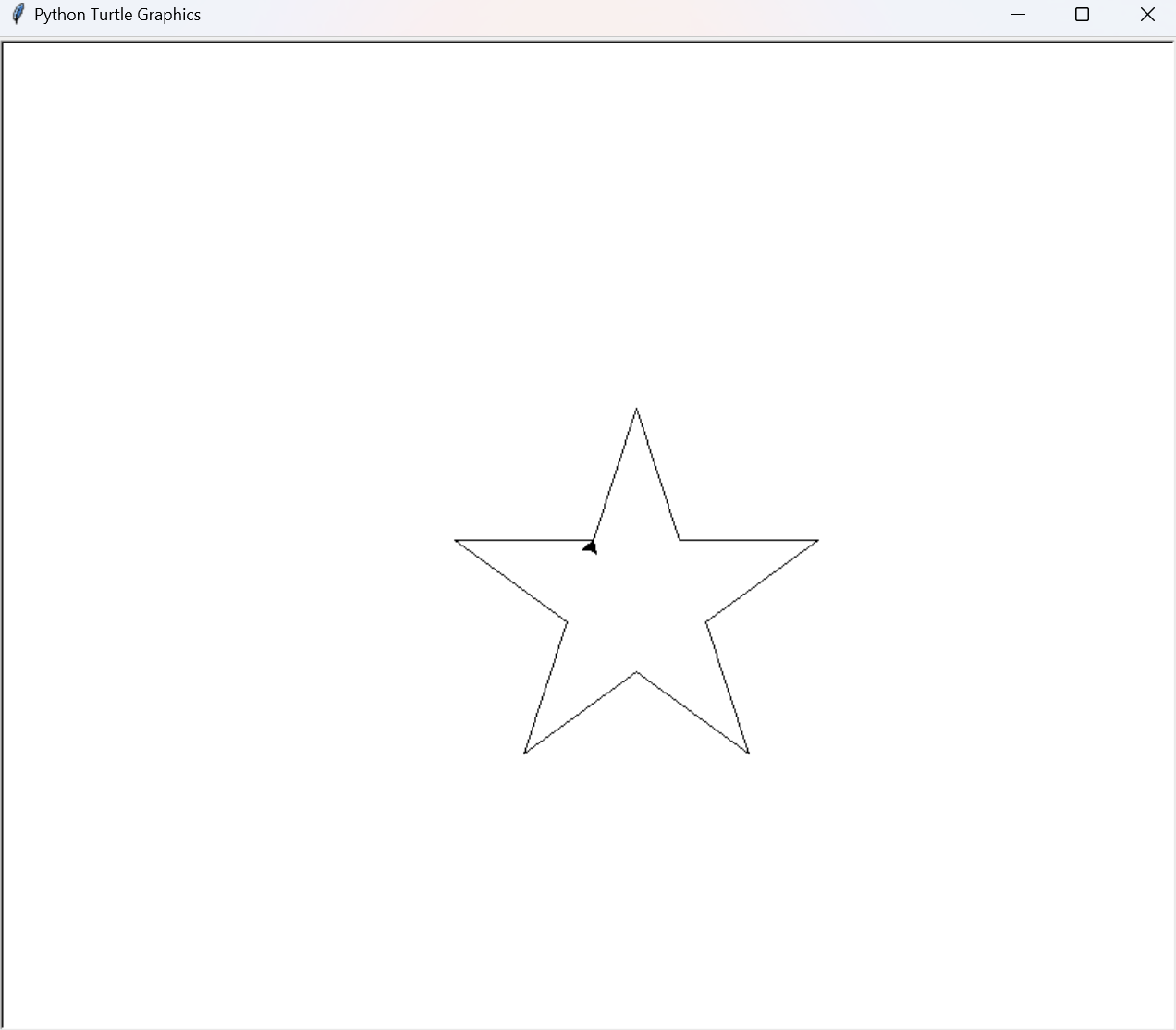
03 彩虹
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
turtle.pensize(10)
for i in range(6):
turtle.pencolor(colors[i])
turtle.circle(50)
turtle.right(60)
turtle.done()
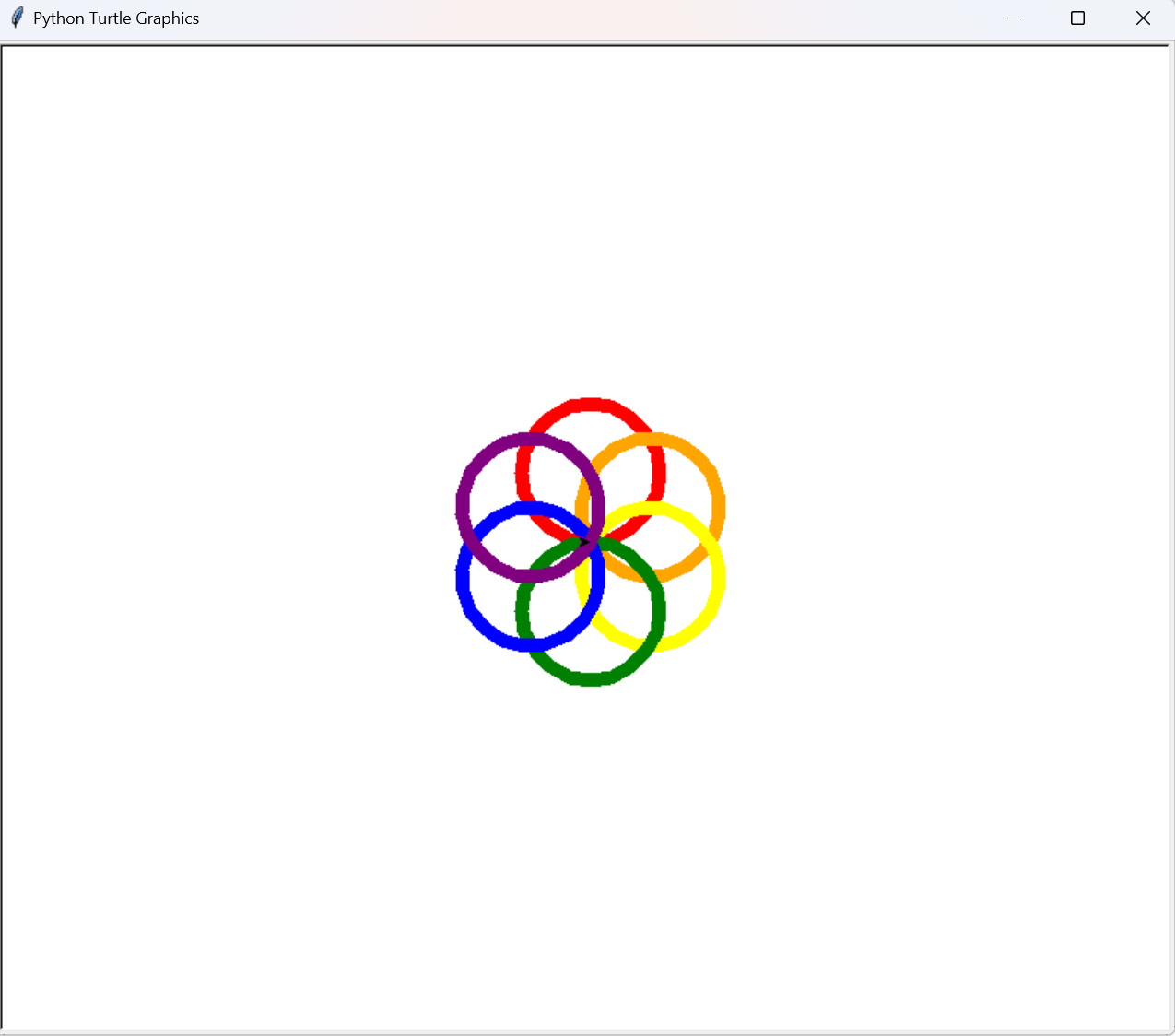
04 螺旋线
import turtle
turtle.pensize(2)
for i in range(100):
turtle.forward(i * 2)
turtle.right(90)
turtle.done()
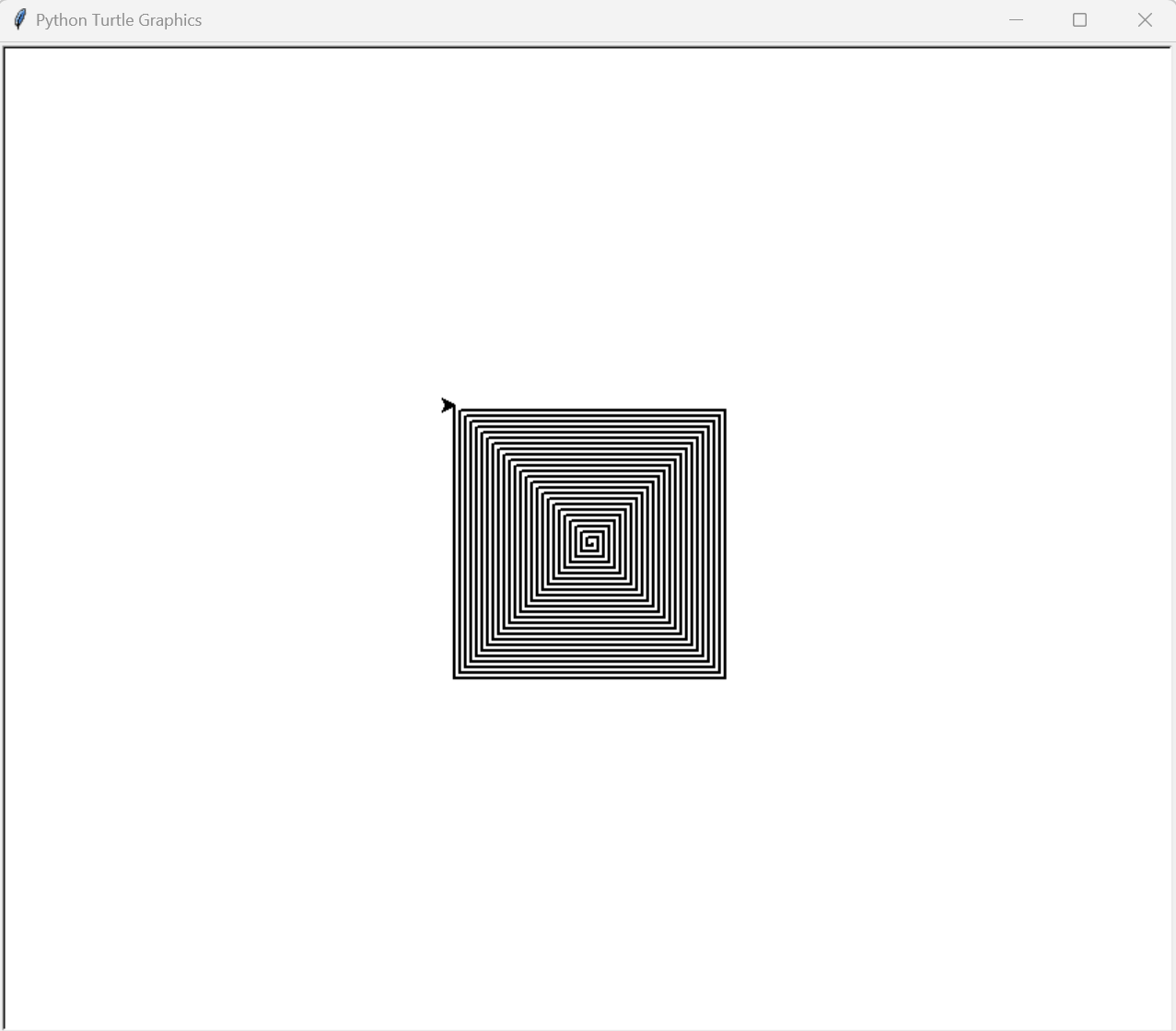
05 爱心
from turtle import *
def curvemove():
for i in range(200):
right(1)
forward(1)
color('red','pink')
begin_fill()
left(140)
forward(111.65)
curvemove()
left(120)
curvemove()
forward(111.65)
end_fill()
done()
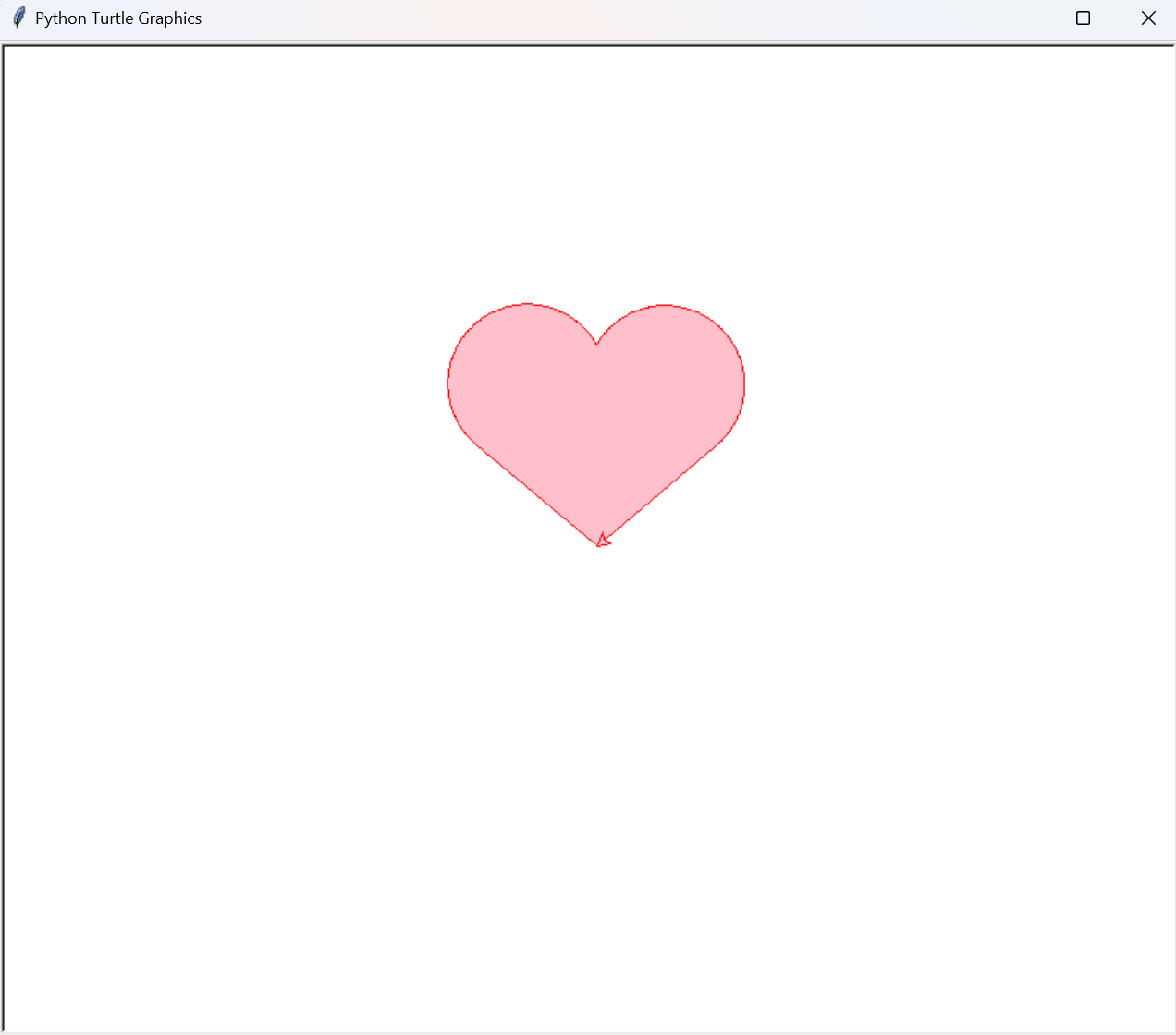
二、进阶绘图
00 一箭穿心
from turtle import *
def go_to(x, y):
up()
goto(x, y)
down()
def big_Circle(size):
speed(10)
for i in range(150):
forward(size)
right(0.3)
def small_Circle(size):
speed(10)
for i in range(210):
forward(size)
right(0.786)
def line(size):
speed(10)
forward(51 * size)
def heart(x, y, size):
go_to(x, y)
left(150)
begin_fill()
line(size)
big_Circle(size)
small_Circle(size)
left(120)
small_Circle(size)
big_Circle(size)
line(size)
end_fill()
def arrow():
pensize(10)
setheading(0)
go_to(-400, 0)
left(15)
forward(150)
go_to(339, 178)
forward(150)
def arrowHead():
pensize(1)
speed(10)
color('red', 'red')
begin_fill()
left(120)
forward(20)
right(150)
forward(35)
right(120)
forward(35)
right(150)
forward(20)
end_fill()
def main():
pensize(2)
color('red', 'pink')
heart(200, 0, 1)
setheading(0)
heart(-80, -100, 1.5)
arrow()
arrowHead()
go_to(400, -300)
done()
if __name__ == '__main__':
main()
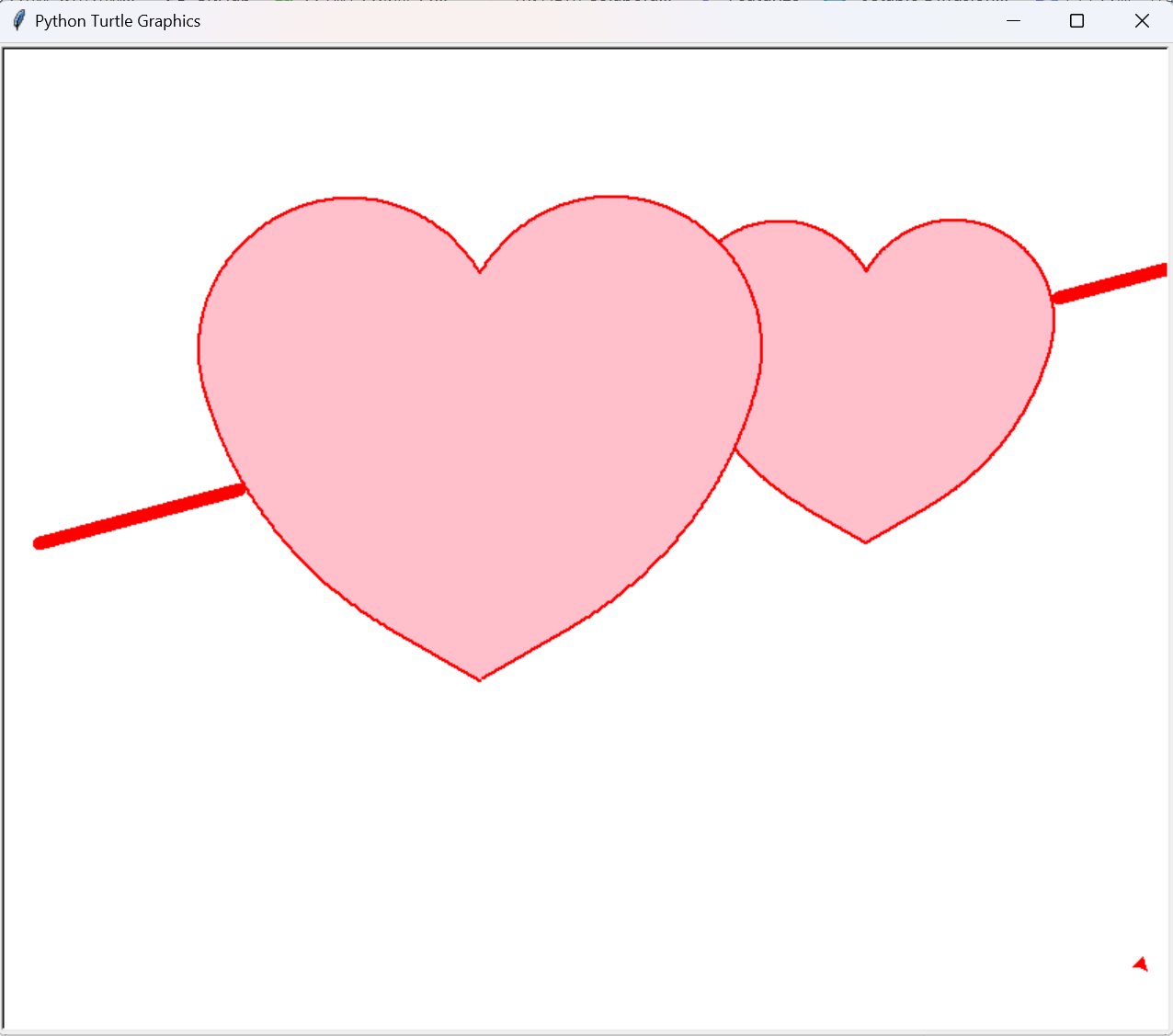
01 皮卡丘
import turtle as t
def nose():
t.penup()
t.seth(90)
t.fd(100)
t.pendown()
t.begin_fill()
t.fillcolor("black")
t.seth(45)
t.fd(25)
t.seth(135)
t.circle(25,90)
t.seth(315)
t.fd(25)
t.end_fill()
def eyes(seth,fd,c):
t.penup()
t.seth(seth)
t.fd(fd)
t.pendown()
t.begin_fill()
t.fillcolor('black')
t.circle(50)
t.end_fill()
t.penup()
t.circle(50,c)
t.pendown()
t.begin_fill()
t.fillcolor('white')
t.circle(20)
t.end_fill()
def face(seth,fd):
t.penup()
t.seth(seth)
t.fd(fd)
t.pendown()
t.begin_fill()
t.fillcolor('red')
t.circle(70)
t.end_fill()
def lip():
t.penup()
t.seth(135)
t.fd(250)
t.pendown()
t.seth(-300)
t.circle(30,-65)
t.begin_fill()
t.fillcolor('Firebrick')
t.seth(165)
t.fd(140)
t.seth(195)
t.fd(140)
t.seth(-360)
t.circle(30,-65)
t.penup()
t.seth(-60)
t.circle(30,65)
t.pendown()
t.seth(-70)
t.fd(240)
t.circle(55,140)
t.seth(70)
t.fd(240)
t.end_fill()
t.seth(-110)
t.fd(80)
t.begin_fill()
t.fillcolor('Firebrick')
t.seth(120)
t.circle(120,123)
t.seth(-70)
t.fd(165)
t.circle(55,140)
t.seth(72)
t.fd(165)
t.end_fill()
def setting():
t.pensize(4)
t.hideturtle()
t.setup(1000,600)
t.speed(10)
t.screensize(bg='yellow')
def main():
setting()
nose()
eyes(160,250,60)
eyes(-9.5,530,230)
face(195,600)
face(-11,720)
lip()
t.done()
if __name__ == '__main__':
main()
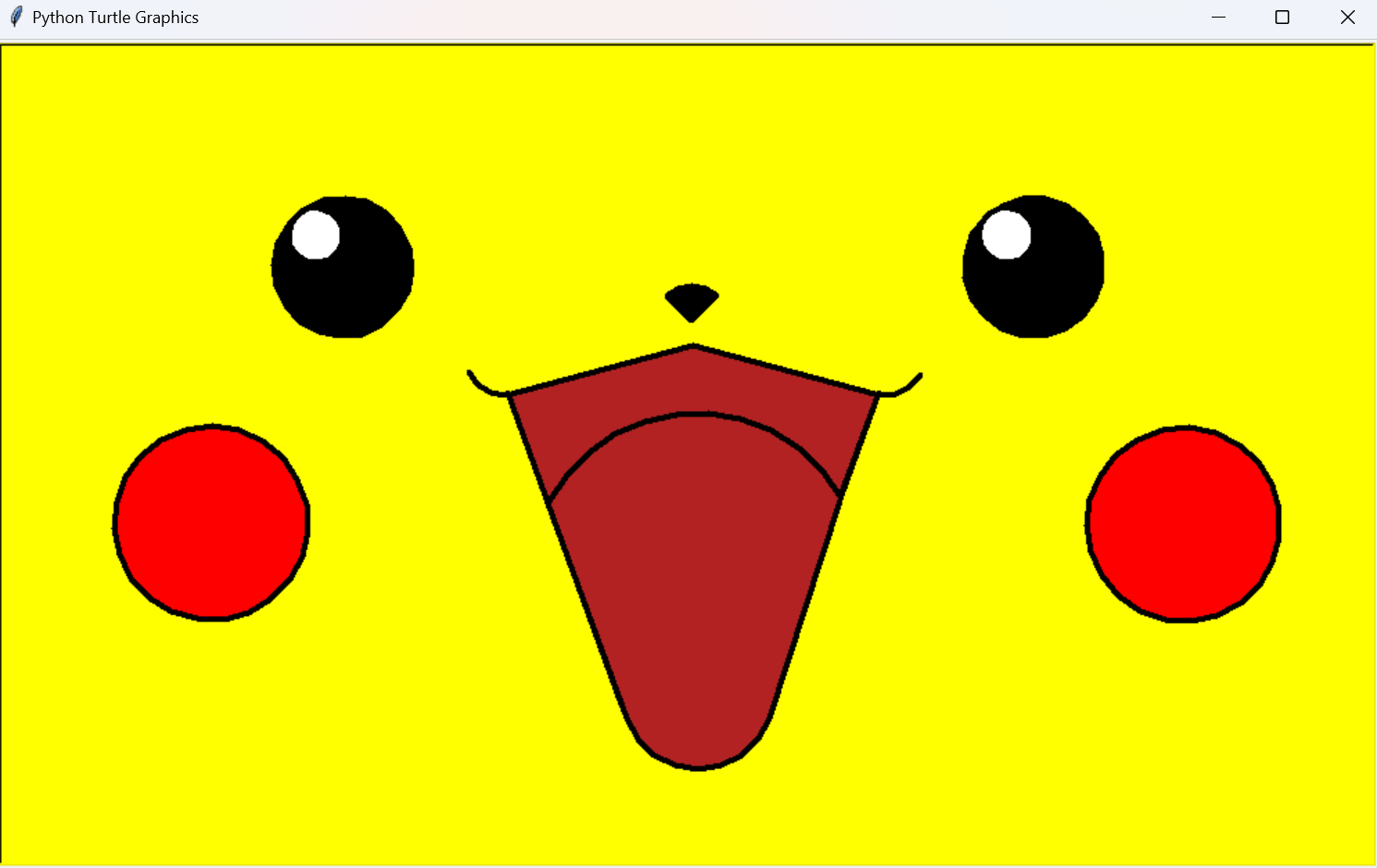
02 圣诞树
from turtle import *
import random
import time
n = 100.0
speed("fastest")
screensize(bg='seashell')
left(90)
forward(3*n)
color("orange", "yellow")
begin_fill()
left(126)
for i in range(5):
forward(n/5)
right(144)
forward(n/5)
left(72)
end_fill()
right(126)
color("dark green")
backward(n*4.8)
def tree(d, s):
if d <= 0: return
forward(s)
tree(d-1, s*.8)
right(120)
tree(d-3, s*.5)
right(120)
tree(d-3, s*.5)
right(120)
backward(s)
tree(15, n)
backward(n/2)
for i in range(200):
a = 200 - 400 * random.random()
b = 10 - 20 * random.random()
up()
forward(b)
left(90)
forward(a)
down()
if random.randint(0, 1) == 0:
color('tomato')
else:
color('wheat')
circle(2)
up()
backward(a)
right(90)
backward(b)
time.sleep(60)
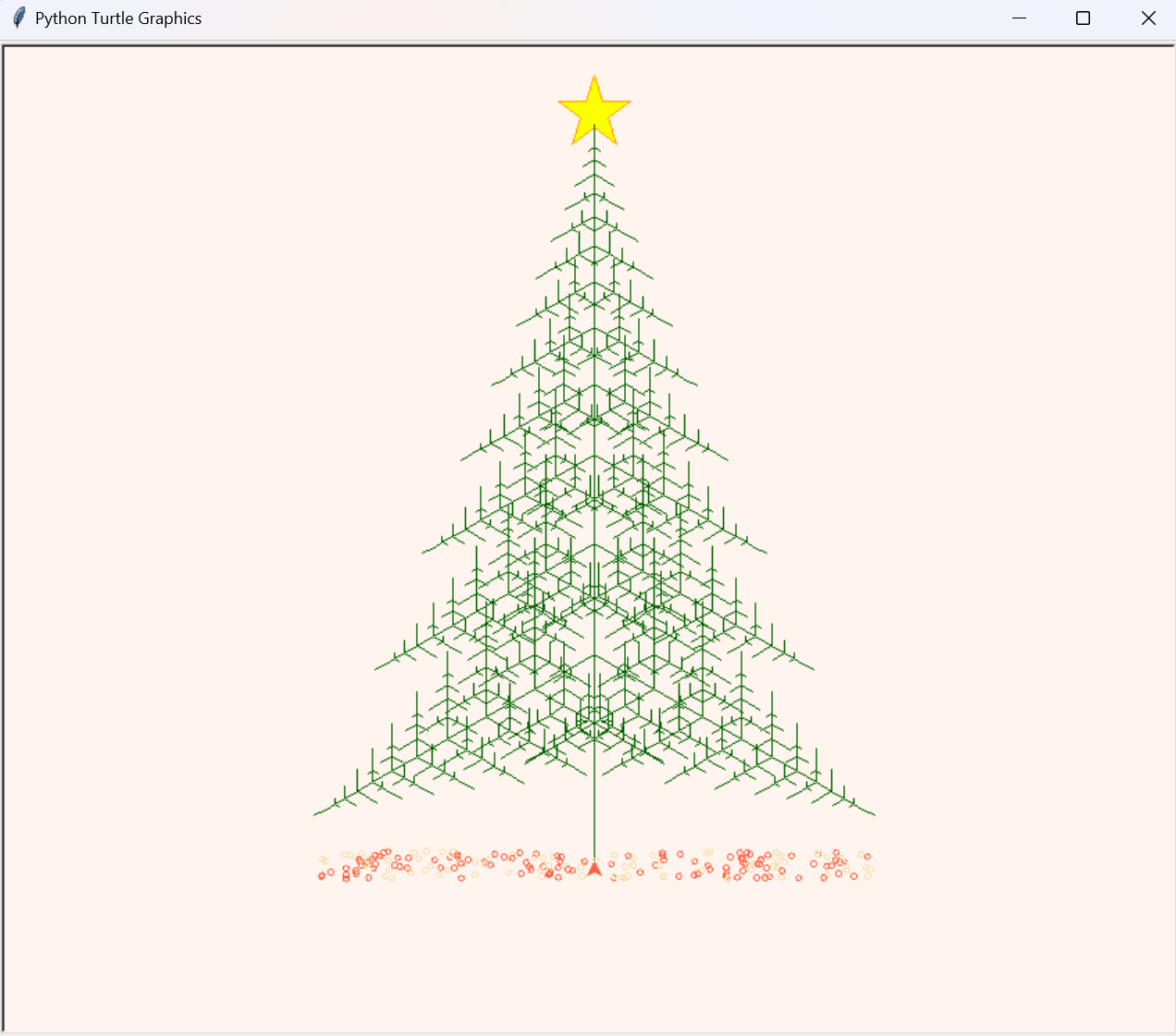
03 樱花树
import turtle
import random
from turtle import *
from time import sleep
def tree(branchLen, t):
sleep(0.0005)
if branchLen > 3:
if 8 <= branchLen <= 12:
if random.randint(0, 2) == 0:
t.color('snow')
else:
t.color('lightcoral')
t.pensize(branchLen / 3)
elif branchLen < 8:
if random.randint(0, 1) == 0:
t.color('snow')
else:
t.color('lightcoral')
t.pensize(branchLen / 2)
else:
t.color('sienna')
t.pensize(branchLen / 10)
t.forward(branchLen)
a = 1.5 * random.random()
t.right(20 * a)
b = 1.5 * random.random()
tree(branchLen - 10 * b, t)
t.left(40 * a)
tree(branchLen - 10 * b, t)
t.right(20 * a)
t.up()
t.backward(branchLen)
t.down()
def petal(m, t):
for i in range(m):
a = 200 - 400 * random.random()
b = 10 - 20 * random.random()
t.up()
t.forward(b)
t.left(90)
t.forward(a)
t.down()
t.color('lightcoral')
t.circle(1)
t.up()
t.backward(a)
t.right(90)
t.backward(b)
def main():
t = turtle.Turtle()
w = turtle.Screen()
t.hideturtle()
getscreen().tracer(5, 0)
w.screensize(bg='wheat')
t.left(90)
t.up()
t.backward(150)
t.down()
t.color('sienna')
tree(60, t)
petal(200, t)
w.exitonclick()
main()
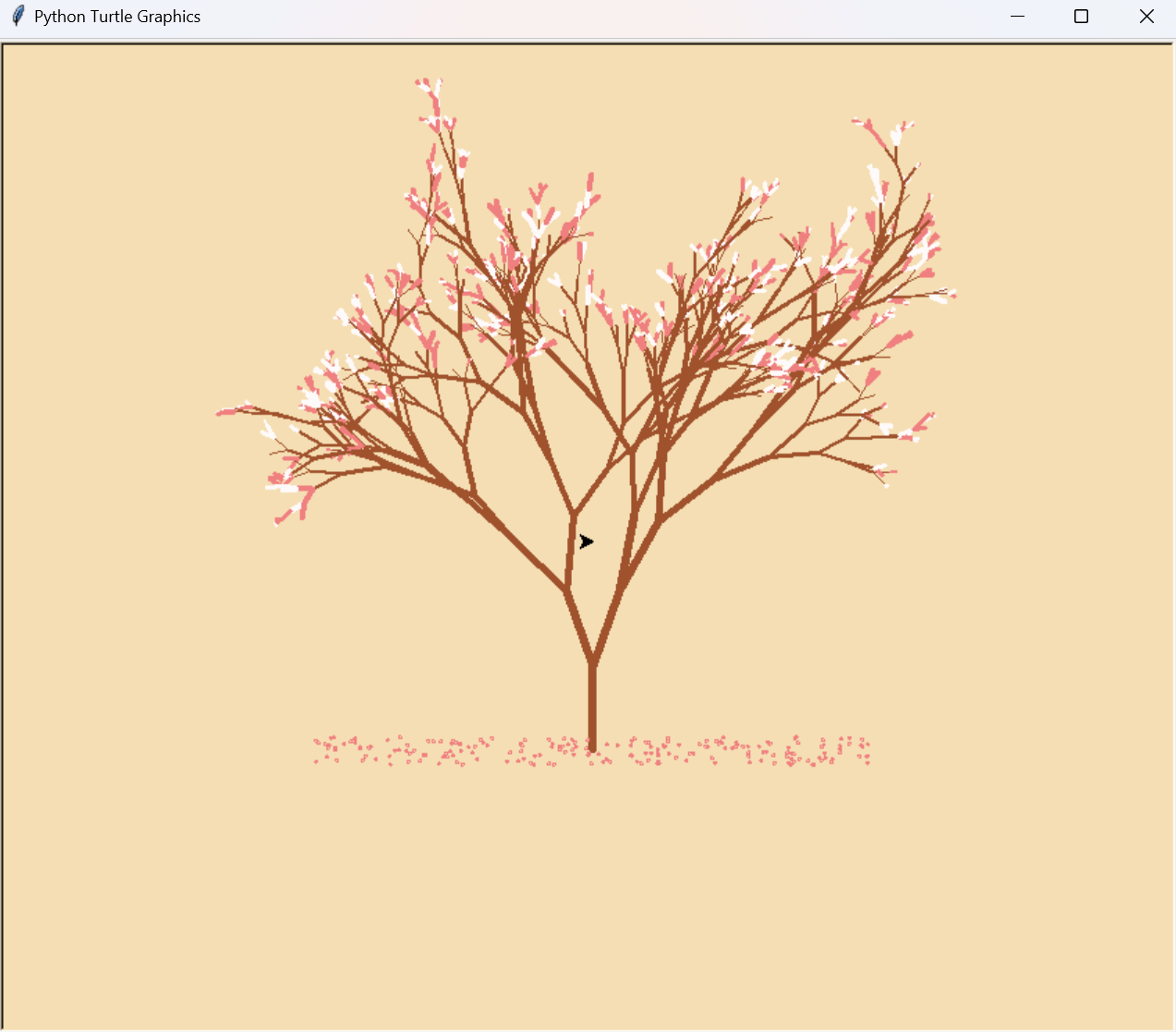
04 叮当猫
from turtle import *
def my_goto(x, y):
penup()
goto(x, y)
pendown()
def eyes():
fillcolor("#ffffff")
begin_fill()
tracer(False)
a = 2.5
for i in range(120):
if 0 <= i < 30 or 60 <= i < 90:
a -= 0.05
lt(3)
fd(a)
else:
a += 0.05
lt(3)
fd(a)
tracer(True)
end_fill()
def beard():
my_goto(-32, 135)
seth(165)
fd(60)
my_goto(-32, 125)
seth(180)
fd(60)
my_goto(-32, 115)
seth(193)
fd(60)
my_goto(37, 135)
seth(15)
fd(60)
my_goto(37, 125)
seth(0)
fd(60)
my_goto(37, 115)
seth(-13)
fd(60)
def mouth():
my_goto(5, 148)
seth(270)
fd(100)
seth(0)
circle(120, 50)
seth(230)
circle(-120, 100)
def scarf():
fillcolor('#e70010')
begin_fill()
seth(0)
fd(200)
circle(-5, 90)
fd(10)
circle(-5, 90)
fd(207)
circle(-5, 90)
fd(10)
circle(-5, 90)
end_fill()
def nose():
my_goto(-10, 158)
seth(315)
fillcolor('#e70010')
begin_fill()
circle(20)
end_fill()
def black_eyes():
seth(0)
my_goto(-20, 195)
fillcolor('#000000')
begin_fill()
circle(13)
end_fill()
pensize(6)
my_goto(20, 205)
seth(75)
circle(-10, 150)
pensize(3)
my_goto(-17, 200)
seth(0)
fillcolor('#ffffff')
begin_fill()
circle(5)
end_fill()
my_goto(0, 0)
def face():
fd(183)
lt(45)
fillcolor('#ffffff')
begin_fill()
circle(120,100)
seth(180)
fd(121)
pendown()
seth(215)
circle(120,100)
end_fill()
my_goto(63.56,218.24)
seth(90)
eyes()
seth(180)
penup()
fd(60)
pendown()
seth(90)
eyes()
penup()
seth(180)
fd(64)
def head():
penup()
circle(150,40)
pendown()
fillcolor('#00a0de')
begin_fill()
circle(150,280)
end_fill()
def Doraemon():
head()
scarf()
face()
nose()
mouth()
beard()
my_goto(0,0)
seth(0)
penup()
circle(150,50)
pendown()
seth(30)
fd(40)
seth(70)
circle(-30,270)
fillcolor('#00a0de')
begin_fill()
seth(230)
fd(80)
seth(90)
circle(1000,1)
seth(-89)
circle(-1000,10)
seth(180)
fd(70)
seth(90)
circle(30,180)
seth(180)
fd(70)
seth(100)
circle(-1000,9)
seth(-86)
circle(1000,2)
seth(230)
fd(40)
circle(-30,230)
seth(45)
fd(81)
seth(0)
fd(203)
circle(5,90)
fd(10)
circle(5,90)
fd(7)
seth(40)
circle(150,10)
seth(30)
fd(40)
end_fill()
seth(70)
fillcolor('#ffffff')
begin_fill()
circle(-30)
end_fill()
my_goto(103.74,-182.59)
seth(0)
fillcolor('#ffffff')
begin_fill()
fd(15)
circle(-15,180)
fd(90)
circle(-15,180)
fd(10)
end_fill()
my_goto(-92.26,-182.59)
seth(180)
fillcolor('#ffffff')
begin_fill()
fd(15)
circle(15, 180)
fd(90)
circle(15, 180)
fd(10)
end_fill()
my_goto(-133.97,-91.81)
seth(50)
fillcolor('#ffffff')
begin_fill()
circle(30)
end_fill()
my_goto(-103.42,15.09)
seth(0)
fd(38)
seth(230)
begin_fill()
circle(90,260)
end_fill()
my_goto(5,-40)
seth(0)
fd(70)
seth(-90)
circle(-70,180)
seth(0)
fd(70)
my_goto(-103.42,15.09)
fd(90)
seth(70)
fillcolor('#ffd200')
begin_fill()
circle(-20)
end_fill()
seth(170)
fillcolor('#ffd200')
begin_fill()
circle(-2,180)
seth(10)
circle(-100,22)
circle(-2,180)
seth(180-10)
circle(100,22)
end_fill()
goto(-13.42,15.09)
seth(250)
circle(20,110)
seth(90)
fd(15)
dot(10)
my_goto(0,-150)
black_eyes()
if __name__=='__main__':
screensize(800,600,"#f0f0f0")
pensize(3)
speed(9)
Doraemon()
my_goto(100,-300)
write('by 邻家小胡',font=("Bradley Hand ITC",30,"bold"))
mainloop()
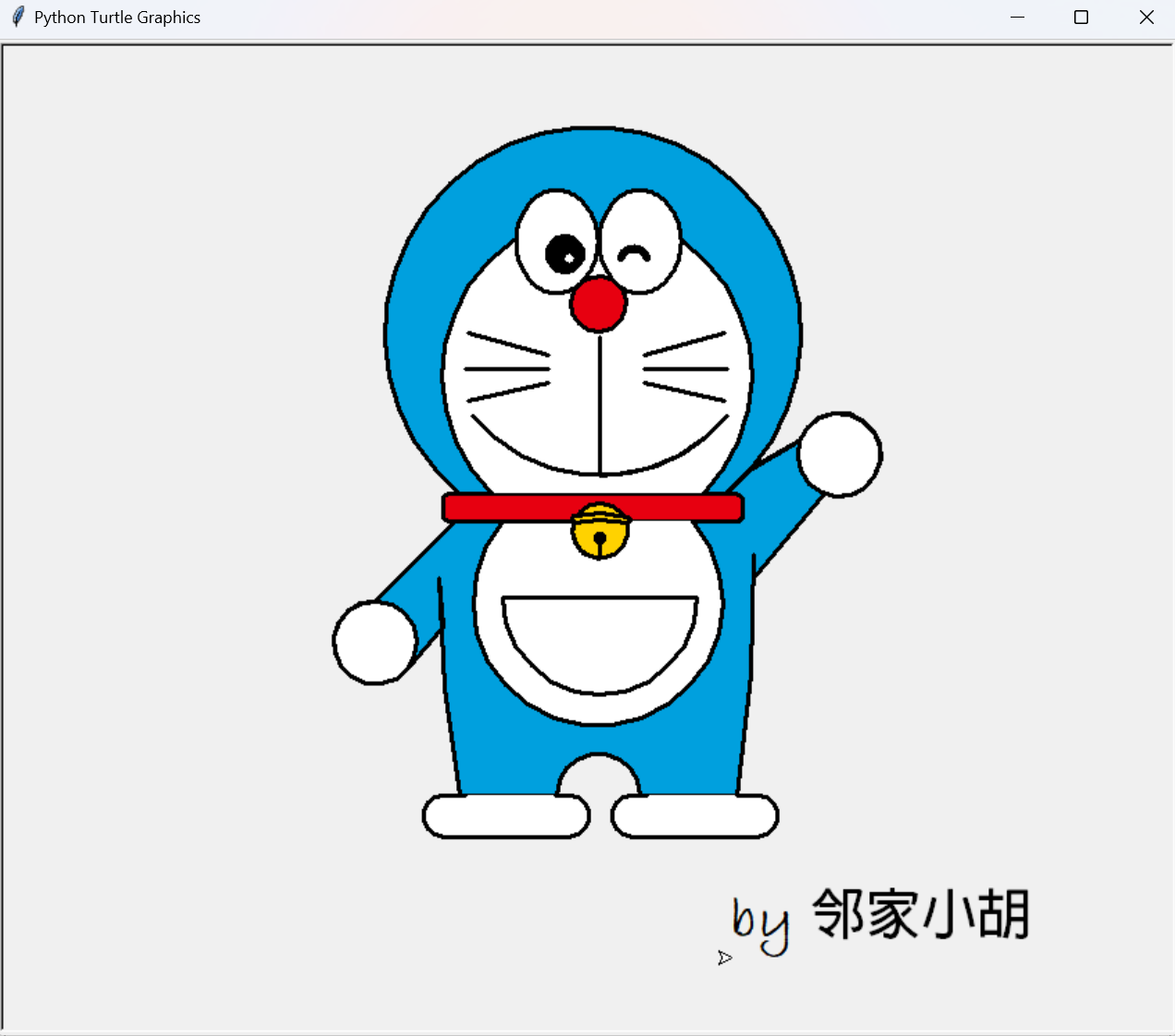
05 小猪佩奇
from turtle import*
speed(10)
def nose(x,y):
penup()
goto(x,y)
pendown()
setheading(-30)
begin_fill()
a=0.4
for i in range(120):
if 0<=i<30 or 60<=i<90:
a=a+0.08
left(3)
forward(a)
else:
a=a-0.08
left(3)
forward(a)
end_fill()
penup()
setheading(90)
forward(25)
setheading(0)
forward(10)
pendown()
pencolor(255,155,192)
setheading(10)
begin_fill()
circle(5)
color(160,82,45)
end_fill()
penup()
setheading(0)
forward(20)
pendown()
pencolor(255,155,192)
setheading(10)
begin_fill()
circle(5)
color(160,82,45)
end_fill()
def head(x,y):
color((255,155,192),"pink")
penup()
goto(x,y)
setheading(0)
pendown()
begin_fill()
setheading(180)
circle(300,-30)
circle(100,-60)
circle(80,-100)
circle(150,-20)
circle(60,-95)
setheading(161)
circle(-300,15)
penup()
goto(-100,100)
pendown()
setheading(-30)
a=0.4
for i in range(60):
if 0<=i<30 or 60<=i<90:
a=a+0.08
lt(3)
fd(a)
else:
a=a-0.08
lt(3)
fd(a)
end_fill()
def ears(x,y):
color((255,155,192),"pink")
penup()
goto(x,y)
pendown()
begin_fill()
setheading(100)
circle(-50,50)
circle(-10,120)
circle(-50,54)
end_fill()
penup()
setheading(90)
forward(-12)
setheading(0)
forward(30)
pendown()
begin_fill()
setheading(100)
circle(-50,50)
circle(-10,120)
circle(-50,56)
end_fill()
def eyes(x,y):
color((255,155,192),"white")
penup()
setheading(90)
forward(-20)
setheading(0)
forward(-95)
pendown()
begin_fill()
circle(15)
end_fill()
color("black")
penup()
setheading(90)
forward(12)
setheading(0)
forward(-3)
pendown()
begin_fill()
circle(3)
end_fill()
color((255,155,192),"white")
penup()
seth(90)
forward(-25)
seth(0)
forward(40)
pendown()
begin_fill()
circle(15)
end_fill()
color("black")
penup()
setheading(90)
forward(12)
setheading(0)
forward(-3)
pendown()
begin_fill()
circle(3)
end_fill()
def cheek(x,y):
color((255,155,192))
penup()
goto(x,y)
pendown()
setheading(0)
begin_fill()
circle(30)
end_fill()
def mouth(x,y):
color(239,69,19)
penup()
goto(x,y)
pendown()
setheading(-80)
circle(30,40)
circle(40,80)
def body(x,y):
color("red",(255,99,71))
penup()
goto(x,y)
pendown()
begin_fill()
setheading(-130)
circle(100,10)
circle(300,30)
setheading(0)
forward(230)
setheading(90)
circle(300,30)
circle(100,3)
color((255,155,192),(255,100,100))
setheading(-135)
circle(-80,63)
circle(-150,24)
end_fill()
def hands(x,y):
color((255,155,192))
penup()
goto(x,y)
pendown()
setheading(-160)
circle(300,15)
penup()
setheading(90)
forward(15)
setheading(0)
forward(0)
pendown()
setheading(-10)
circle(-20,90)
penup()
setheading(90)
forward(30)
setheading(0)
forward(237)
pendown()
setheading(-20)
circle(-300,15)
penup()
setheading(90)
forward(20)
setheading(0)
forward(0)
pendown()
setheading(-170)
circle(20,90)
def foot(x,y):
pensize(10)
color((240,128,128))
penup()
goto(x,y)
pendown()
setheading(-90)
forward(40)
setheading(-180)
color("black")
pensize(15)
fd(20)
pensize(10)
color((240,128,128))
penup()
setheading(90)
forward(40)
setheading(0)
forward(90)
pendown()
setheading(-90)
forward(40)
setheading(-180)
color("black")
pensize(15)
fd(20)
def tail(x,y):
pensize(4)
color((255,155,192))
penup()
goto(x,y)
pendown()
seth(0)
circle(70,20)
circle(10,330)
circle(70,30)
def setting():
speed(99)
pensize(4)
hideturtle()
colormode(255)
color((255,155,192),"pink")
setup(840,500)
def main():
setting()
nose(-100,100)
head(-69,167)
ears(0,160)
eyes(0,140)
cheek(80,10)
mouth(-20,30)
body(-32,-8)
hands(-56,-45)
foot(2,-177)
tail(148,-155)
done()
if __name__ == '__main__':
main()
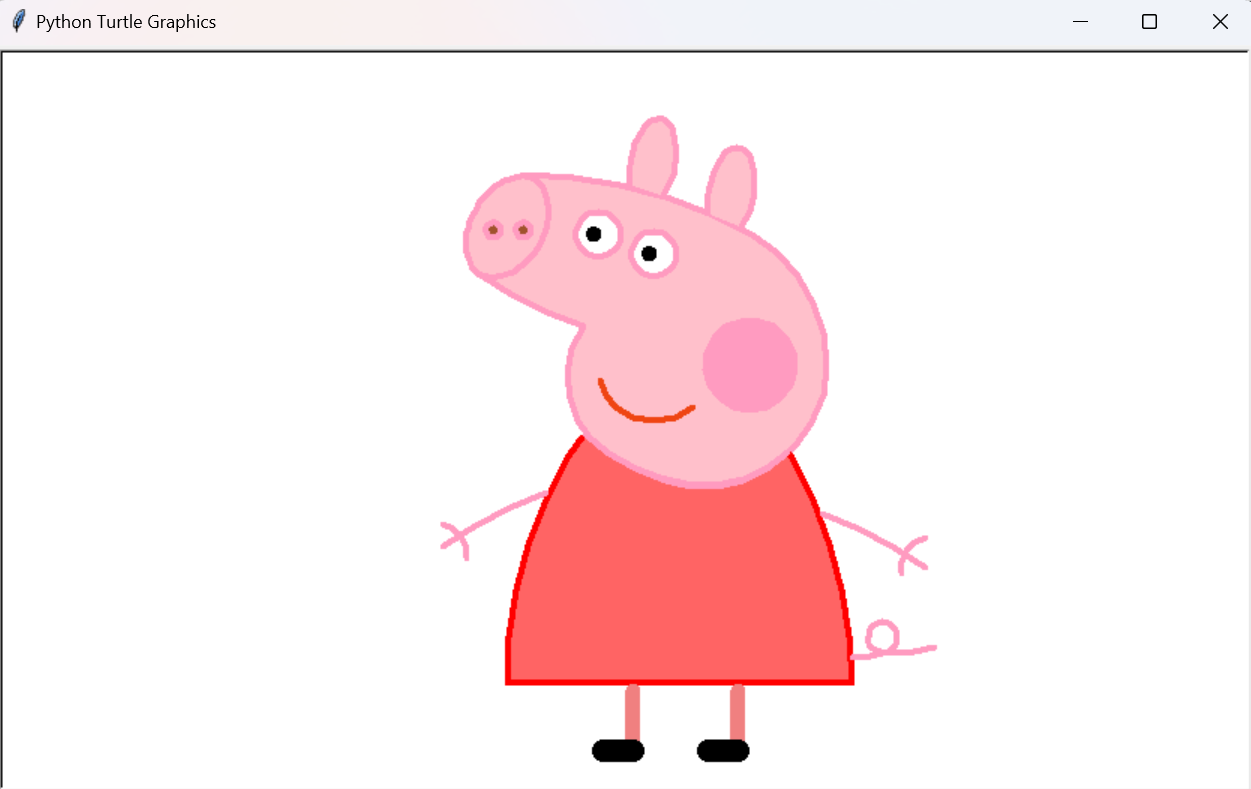
06 冰墩墩
import turtle
turtle.setup(800, 600)
turtle.speed(0)
turtle.penup()
turtle.goto(177, 112)
turtle.pencolor("lightgray")
turtle.pensize(3)
turtle.fillcolor("white")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(80)
turtle.circle(-45, 200)
turtle.circle(-300, 23)
turtle.end_fill()
turtle.penup()
turtle.goto(182, 95)
turtle.pencolor("black")
turtle.pensize(1)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.setheading(95)
turtle.pendown()
turtle.circle(-37, 160)
turtle.circle(-20, 50)
turtle.circle(-200, 30)
turtle.end_fill()
turtle.penup()
turtle.goto(-73, 230)
turtle.pencolor("lightgray")
turtle.pensize(3)
turtle.fillcolor("white")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(20)
turtle.circle(-250, 35)
turtle.setheading(50)
turtle.circle(-42, 180)
turtle.setheading(-50)
turtle.circle(-190, 30)
turtle.circle(-320, 45)
turtle.circle(120, 30)
turtle.circle(200, 12)
turtle.circle(-18, 85)
turtle.circle(-180, 23)
turtle.circle(-20, 110)
turtle.circle(15, 115)
turtle.circle(100, 12)
turtle.circle(15, 120)
turtle.circle(-15, 110)
turtle.circle(-150, 30)
turtle.circle(-15, 70)
turtle.circle(-150, 10)
turtle.circle(200, 35)
turtle.circle(-150, 20)
turtle.setheading(-120)
turtle.circle(50, 30)
turtle.circle(-35, 200)
turtle.circle(-300, 23)
turtle.setheading(86)
turtle.circle(-300, 26)
turtle.setheading(122)
turtle.circle(-53, 160)
turtle.end_fill()
turtle.penup()
turtle.goto(-130, 180)
turtle.pencolor("black")
turtle.pensize(1)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(120)
turtle.circle(-28, 160)
turtle.setheading(210)
turtle.circle(150, 20)
turtle.end_fill()
turtle.penup()
turtle.goto(-130, 180)
turtle.pencolor("black")
turtle.pensize(1)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(120)
turtle.circle(-28, 160)
turtle.setheading(210)
turtle.circle(150, 20)
turtle.end_fill()
turtle.penup()
turtle.goto(90, 230)
turtle.setheading(40)
turtle.begin_fill()
turtle.pendown()
turtle.circle(-30, 170)
turtle.setheading(125)
turtle.circle(150, 23)
turtle.end_fill()
turtle.penup()
turtle.goto(-180, -55)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.setheading(-120)
turtle.setheading(-120)
turtle.pendown()
turtle.circle(50, 30)
turtle.circle(-27, 200)
turtle.circle(-300, 20)
turtle.setheading(-90)
turtle.circle(300, 14)
turtle.end_fill()
turtle.penup()
turtle.goto(108, -168)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(-115)
turtle.circle(110, 15)
turtle.circle(200, 10)
turtle.circle(-18, 80)
turtle.circle(-180, 13)
turtle.circle(-20, 90)
turtle.circle(15, 60)
turtle.setheading(42)
turtle.circle(-200, 29)
turtle.end_fill()
turtle.penup()
turtle.goto(-38, -210)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(-155)
turtle.circle(15, 100)
turtle.circle(-10, 110)
turtle.circle(-100, 30)
turtle.circle(-15, 65)
turtle.circle(-100, 10)
turtle.circle(200, 15)
turtle.setheading(-14)
turtle.circle(-200, 27)
turtle.end_fill()
turtle.penup()
turtle.goto(-64, 120)
turtle.begin_fill()
turtle.pendown()
turtle.setheading(40)
turtle.circle(-35, 152)
turtle.circle(-100, 50)
turtle.circle(-35, 130)
turtle.circle(-100, 50)
turtle.end_fill()
turtle.penup()
turtle.goto(-47, 55)
turtle.fillcolor("white")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(25, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(-45, 62)
turtle.pencolor("darkslategray")
turtle.fillcolor("darkslategray")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(19, 360)
turtle.circle(19, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(-45, 68)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(10, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(-47, 86)
turtle.pencolor("white")
turtle.fillcolor("white")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(5, 360)
turtle.circle(5, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(51, 82)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(120)
turtle.circle(-32, 152)
turtle.circle(-100, 55)
turtle.circle(-25, 120)
turtle.circle(-120, 45)
turtle.end_fill()
turtle.penup()
turtle.goto(79, 60)
turtle.goto(79, 60)
turtle.fillcolor("white")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(24, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(79, 64)
turtle.pencolor("darkslategray")
turtle.fillcolor("darkslategray")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(19, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(79, 70)
turtle.goto(79, 70)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(10, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(79, 88)
turtle.pencolor("white")
turtle.fillcolor("white")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(0)
turtle.circle(5, 360)
turtle.end_fill()
turtle.penup()
turtle.goto(37, 80)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.pendown()
turtle.circle(-8, 130)
turtle.circle(-22, 100)
turtle.circle(-8, 130)
turtle.end_fill()
turtle.penup()
turtle.goto(-15, 48)
turtle.setheading(-36)
turtle.begin_fill()
turtle.pendown()
turtle.circle(60, 70)
turtle.setheading(-132)
turtle.circle(-45, 100)
turtle.end_fill()
turtle.penup()
turtle.goto(-135, 120)
turtle.pensize(5)
turtle.pencolor("cyan")
turtle.pendown()
turtle.setheading(60)
turtle.circle(-165, 150)
turtle.circle(-130, 78)
turtle.circle(-250, 30)
turtle.circle(-138, 105)
turtle.penup()
turtle.goto(-131, 116)
turtle.pencolor("slateblue")
turtle.pendown()
turtle.setheading(60)
turtle.setheading(60)
turtle.circle(-160, 144)
turtle.circle(-120, 78)
turtle.circle(-242, 30)
turtle.circle(-135, 105)
turtle.penup()
turtle.goto(-127, 112)
turtle.pencolor("orangered")
turtle.pendown()
turtle.setheading(60)
turtle.circle(-155, 136)
turtle.circle(-116, 86)
turtle.circle(-220, 30)
turtle.circle(-134, 103)
turtle.penup()
turtle.goto(-123, 108)
turtle.pencolor("gold")
turtle.pendown()
turtle.setheading(60)
turtle.circle(-150, 136)
turtle.circle(-104, 86)
turtle.circle(-220, 30)
turtle.circle(-126, 102)
turtle.penup()
turtle.goto(-120, 104)
turtle.pencolor("greenyellow")
turtle.pendown()
turtle.setheading(60)
turtle.circle(-145, 136)
turtle.circle(-90, 83)
turtle.circle(-220, 30)
turtle.circle(-120, 100)
turtle.penup()
turtle.penup()
turtle.goto(220, 115)
turtle.pencolor("brown")
turtle.pensize(1)
turtle.fillcolor("brown")
turtle.begin_fill()
turtle.pendown()
turtle.setheading(36)
turtle.circle(-8, 180)
turtle.circle(-60, 24)
turtle.setheading(110)
turtle.circle(-60, 24)
turtle.circle(-8, 180)
turtle.end_fill()
turtle.penup()
turtle.goto(-20, -170)
turtle.pendown()
turtle.pencolor("blue")
turtle.pensize(2)
turtle.circle(7)
turtle.penup()
turtle.goto(-5, -170)
turtle.pendown()
turtle.pencolor("black")
turtle.pensize(2)
turtle.circle(7)
turtle.penup()
turtle.goto(10, -170)
turtle.pendown()
turtle.pencolor("brown")
turtle.pensize(2)
turtle.circle(7)
turtle.penup()
turtle.goto(-17, -175)
turtle.pendown()
turtle.pencolor("lightgoldenrod")
turtle.pensize(2)
turtle.circle(7)
turtle.penup()
turtle.goto(1, -175)
turtle.pendown()
turtle.pencolor("green")
turtle.pensize(2)
turtle.circle(7)
turtle.penup()
turtle.pencolor("black")
turtle.goto(-35, -160)
turtle.write("BEIJING2022", font=('Arial', 10, 'bold italic'))
turtle.hideturtle()
turtle.done()
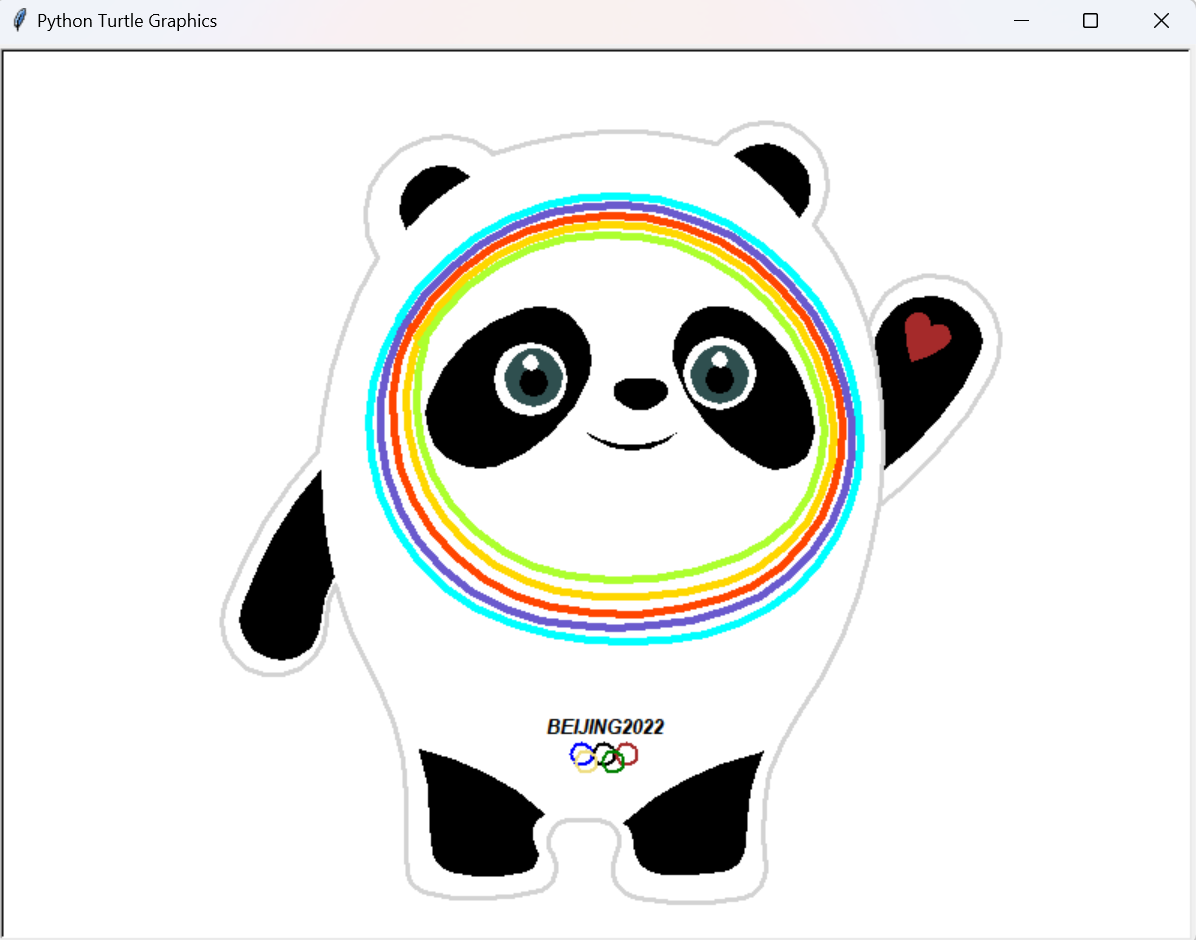
07 万圣节礼物–恶魔南瓜头
import turtle as tu
import random as ra
import math
tu.screensize(1.0, 1.0)
tu.title("万圣节")
tu.bgcolor('darkorange')
t = tu.Pen()
t.ht()
colors = ['black']
class Bat():
def __init__(self):
self.k = ra.uniform(0.1, 0.3)
self.r = 1
self.x = ra.randint(-1000, 1000)
self.y = ra.randint(-500, 500)
self.f = ra.uniform(-3.14, 3.14)
self.speed = ra.randint(5, 10)
self.color = ra.choice(colors)
self.outline = 1
def move(self):
if self.y <= 500:
self.y += self.speed
self.x += self.speed * math.sin(self.f)
self.f += 0.1
else:
self.k = ra.uniform(0.1, 0.3)
self.r = 1
self.x = ra.randint(-1000, 1000)
self.y = -500
self.f = ra.uniform(-3.14, 3.14)
self.speed = ra.randint(5, 10)
self.color = ra.choice(colors)
self.outline = 1
def bat(self):
t.penup()
t.goto(self.x, self.y)
t.pendown()
t.pencolor(self.color)
t.pensize(1)
t.begin_fill()
t.fillcolor(self.color)
t.forward(self.k * 10)
t.setheading(75)
t.forward(self.k * 35)
t.setheading(-75)
t.forward(self.k * 55)
t.setheading(0)
t.circle(self.k * 40, 90)
t.right(90)
t.forward(self.k * 100)
t.left(180)
t.circle(self.k * 100, 90)
t.setheading(180)
t.circle(self.k * 70, 90)
t.left(180)
t.circle(self.k * 70, 90)
t.right(90)
t.circle(self.k * 100, 90)
t.right(180)
t.forward(self.k * 100)
t.right(90)
t.circle(self.k * 40, 90)
t.setheading(75)
t.forward(self.k * 55)
t.setheading(-75)
t.forward(self.k * 35)
t.setheading(0)
t.forward(self.k * 10)
t.end_fill()
def pumpkin(self):
t.color('#CF5E1A', '#CF5E1A')
t.penup()
t.goto(250 * self.r, 30 * self.r)
t.pendown()
t.seth(90)
t.begin_fill()
for j in range(25):
t.fd(j * self.r)
t.left(3.6)
for j in range(25, 0, -1):
t.fd(j * self.r)
t.left(3.6)
t.seth(-90)
t.circle(254 * self.r, 180)
t.end_fill()
eyes_items = [(-60, 230, 0), (60, -50, 1)]
for items in eyes_items:
position, angle, direction = items
t.pensize(6)
t.penup()
t.goto(position * self.r, 0)
t.pendown()
t.color('#4C180D', '#4C180D')
t.begin_fill()
t.seth(angle)
for j in range(55):
t.fd(3 * self.r)
if direction:
t.left(3)
else:
t.right(3)
t.goto(position * self.r, 0)
t.end_fill()
t.penup()
t.goto(0, 0)
t.seth(180)
t.pendown()
t.begin_fill()
t.circle(50 * self.r, steps=3)
t.end_fill()
t.color('#F9D503', '#F9D503')
t.pensize(6)
t.penup()
t.goto(-150 * self.r, -100 * self.r)
t.pendown()
t.begin_fill()
t.seth(-30)
t.fd(100 * self.r)
t.left(90)
t.fd(30 * self.r)
t.right(90)
t.fd(60 * self.r)
t.left(60)
t.fd(60 * self.r)
t.right(90)
t.fd(30 * self.r)
t.left(90)
t.fd(100 * self.r)
t.end_fill()
t.penup()
t.goto(0, 180 * self.r)
t.pendown()
t.color('#2E3C01')
t.seth(100)
t.pensize(25)
t.circle(60 * self.r, 100)
Bats = []
for i in range(100):
Bats.append(Bat())
while True:
tu.tracer(0)
t.clear()
Bats[0].pumpkin()
for i in range(50):
Bats[i].move()
Bats[i].bat()
tu.update()
tu.mainloop()
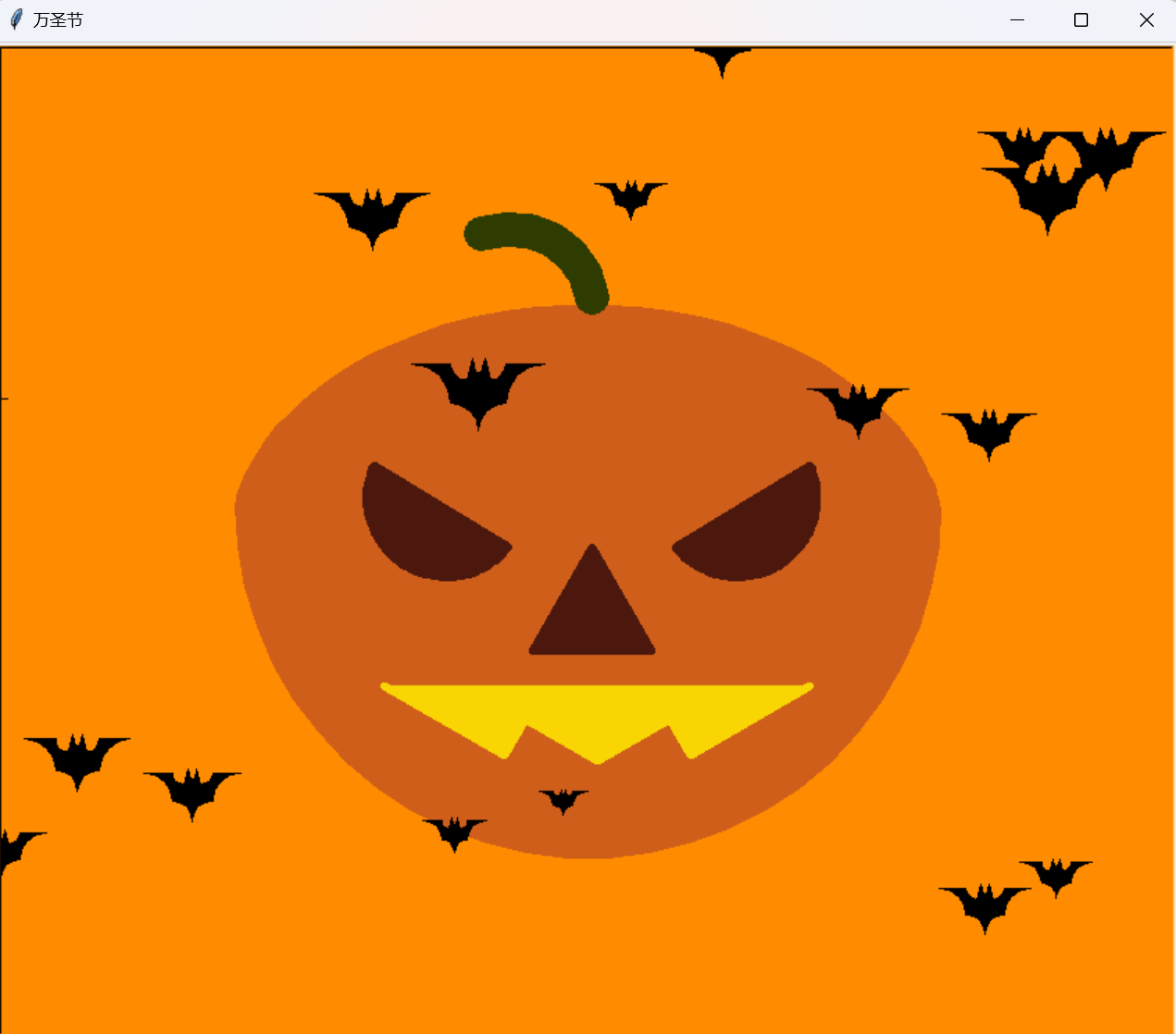
08 浪漫星空
import math
import turtle as tu
import random as ra
tu.setup(1.0, 1.0)
tu.screensize(1.0, 1.0)
tu.bgcolor('black')
t = tu.Pen()
t.ht()
colors2 = ['yellow', 'gold', 'orange']
colors3 = ['skyblue', 'white', 'cyan', 'aqua']
class Star():
def __init__(self):
self.x1 = -850
self.y1 = 300
self.x2 = ra.randint(-1500, 1000)
self.y2 = ra.randint(-500, 500)
self.r2 = ra.randint(1, 5)
self.x3 = ra.randint(-1500, 1000)
self.y3 = ra.randint(-500, 500)
self.r3 = ra.randint(50, 100)
self.t = ra.randint(1, 3)
self.speed2 = ra.randint(1, 3)
self.speed3 = ra.randint(10, 15)
self.color2 = ra.choice(colors2)
self.color3 = ra.choice(colors3)
def moon(self):
t.penup()
t.goto(self.x1, self.y1)
t.pendown()
t.pencolor("yellow")
t.begin_fill()
t.fillcolor("gold")
t.circle(66)
t.end_fill()
def star1(self):
t.pensize(1)
t.penup()
t.goto(self.x2, self.y2)
t.pendown()
t.speed(0)
t.color(self.color2)
t.begin_fill()
t.fillcolor(self.color2)
for i in range(5):
t.forward(self.r2)
t.right(144)
t.forward(self.r2)
t.left(72)
t.end_fill()
def star2(self):
t.pensize(1)
t.penup()
t.goto(self.x3, self.y3)
t.pendown()
t.color(self.color3)
t.begin_fill()
t.fillcolor(self.color3)
t.setheading(-30)
t.right(self.t)
t.forward(self.r3)
t.left(self.t)
t.circle(self.r3 * math.sin(math.radians(self.t)), 180)
t.left(self.t)
t.forward(self.r3)
t.end_fill()
def move(self):
if self.x1 <= 850:
self.x1 += 1
else:
self.x1 = -850
if self.x2 <= 1000:
self.x2 += 2 * self.speed2
else:
self.r2 = ra.randint(1, 5)
self.x2 = ra.randint(-1500, -1000)
self.speed2 = ra.randint(1, 3)
self.color2 = ra.choice(colors2)
if self.y3 >= -500:
self.y3 -= self.speed3
self.x3 += 2 * self.speed3
else:
self.r3 = ra.randint(50, 100)
self.t = ra.randint(1, 3)
self.x3 = ra.randint(-1500, -750)
self.y3 = ra.randint(-500, 1000)
self.speed3 = ra.randint(10, 15)
self.color3 = ra.choice(colors3)
"""
if self.x3 <= 1000: #当流星还在画布中时
self.x3 += 2 * self.speed3 #设置左右移动速度
else:
self.r3 = ra.randint(50,100)
self.x3 = ra.randint(-1500,-1000)
self.t = ra.randint(1, 3)
self.speed3 = ra.randint(1,3)
self.color3 = ra.choice(colors)
"""
Stars = []
for i in range(100):
Stars.append(Star())
while True:
tu.tracer(0)
t.clear()
Stars[0].moon()
for i in range(99):
Stars[i].move()
Stars[i].star1()
for i in range(19):
Stars[i].star2()
tu.update()
tu.mainloop()
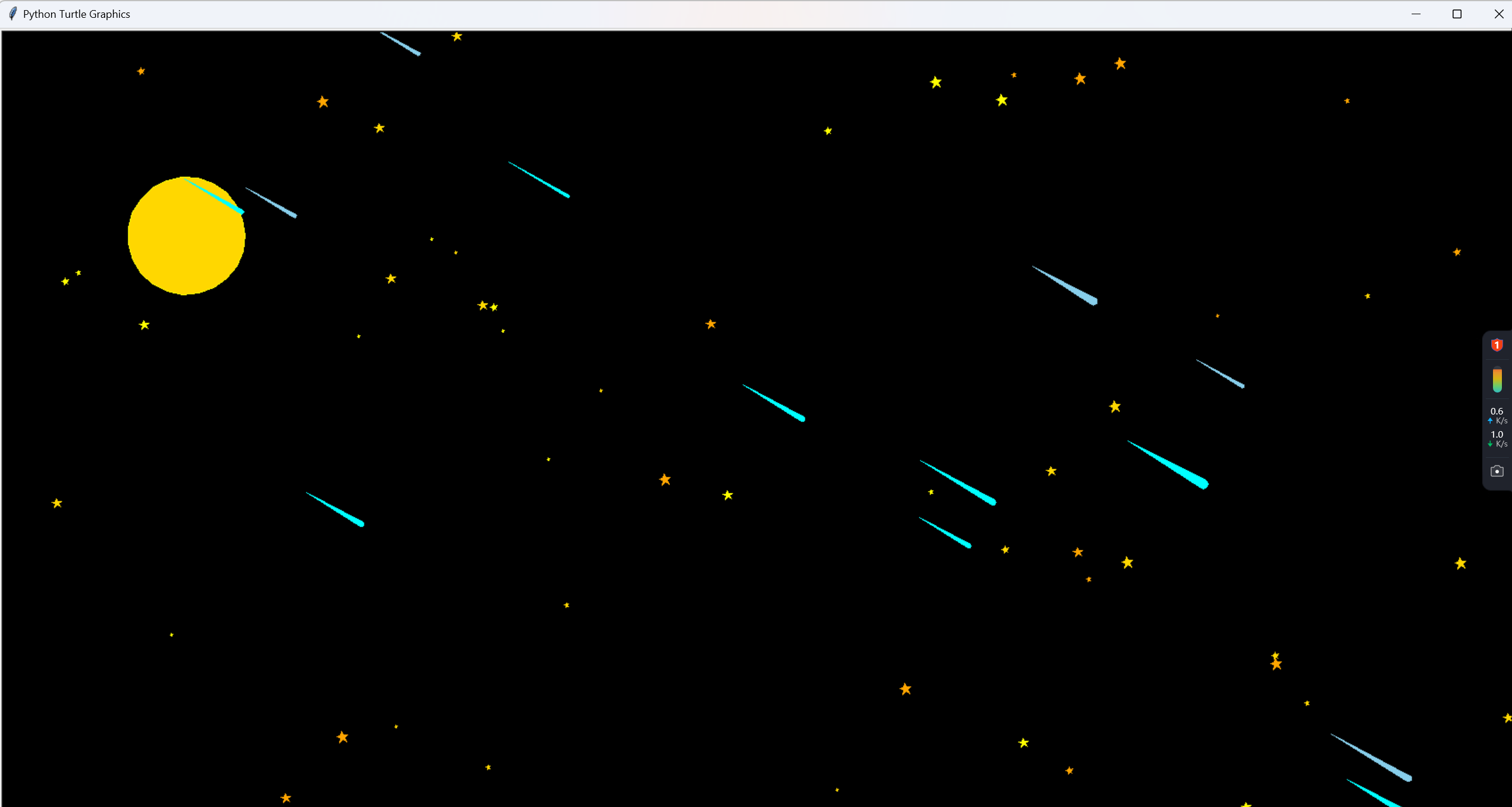