Vue 实战快速上手
登录界面(一)
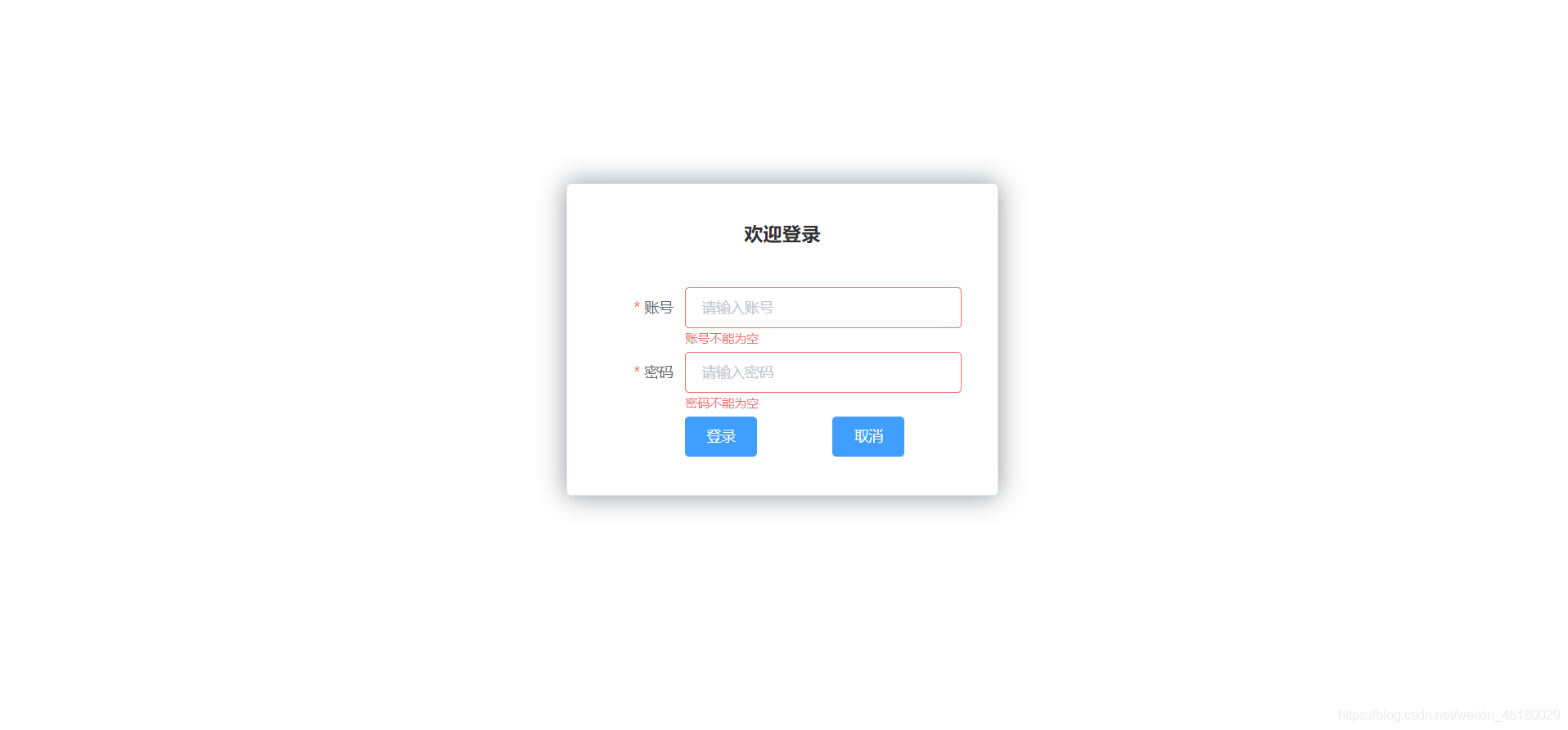
<template>
<div>
<el-form ref="loginForm" :model="form" :rules="rules" label-width="80px" class="login-box">
<h3 class="login-title">欢迎登录</h3>
<el-form-item label="账号" prop="username">
<el-input type="text" placeholder="请输入账号" v-model="form.username"></el-input>
</el-form-item>
<el-form-item label="密码"prop="password">
<el-input type="password" placeholder="请输入密码" v-model="form.password"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="onSubmit">登录</el-button>
<el-button type="primary" style="margin-left:70px ">取消</el-button>
</el-form-item>
<el-dialog title="温馨提示"
:visible.sync="dialogVisible"
width="30%"
:before-close="handleClose"
>
<span>请输入账号和密码</span>
<span slot="footer" class="dialog-footer">
<el-button type="primary" @click="dialogVisible = false">确定</el-button>
</span>
</el-dialog>
</el-form>
</div>
</template>
<script>
export default {
name: "",
data(){
return{
dialogVisible:false,
form:{
username:'',
password:''
},
rules:{
username:[
{required:true,message:'账号不能为空',trigger:'blur'}
],
password:[
{required:true,message:'密码不能为空',trigger:'blur'}
]
}
}
},
methods:{
onSubmit(){
this.$refs['loginForm'].validate((valid)=>{
if(valid){
console.log(true)
}else{
this.dialogVisible = true;
return false;
}
})
}
}
}
</script>
<style scoped>
.login-box{
border: 1px solid #DCDFE6;
width: 350px;
margin: 180px auto;
padding:35px 35px 15px 35px;
border-radius: 5px;
-webkit-border-radius: 5px;
-moz-border-radius: 5px;
box-shadow:0 0 25px #909399;
}
.login-title{
text-align:center;
margin:0 auto 40px auto;
color:#303133;
}
</style>
html,body,#app{ /*设置页面的大小*/
height: 100%;
margin: 0;
padding: 0;
}
登录界面(二)
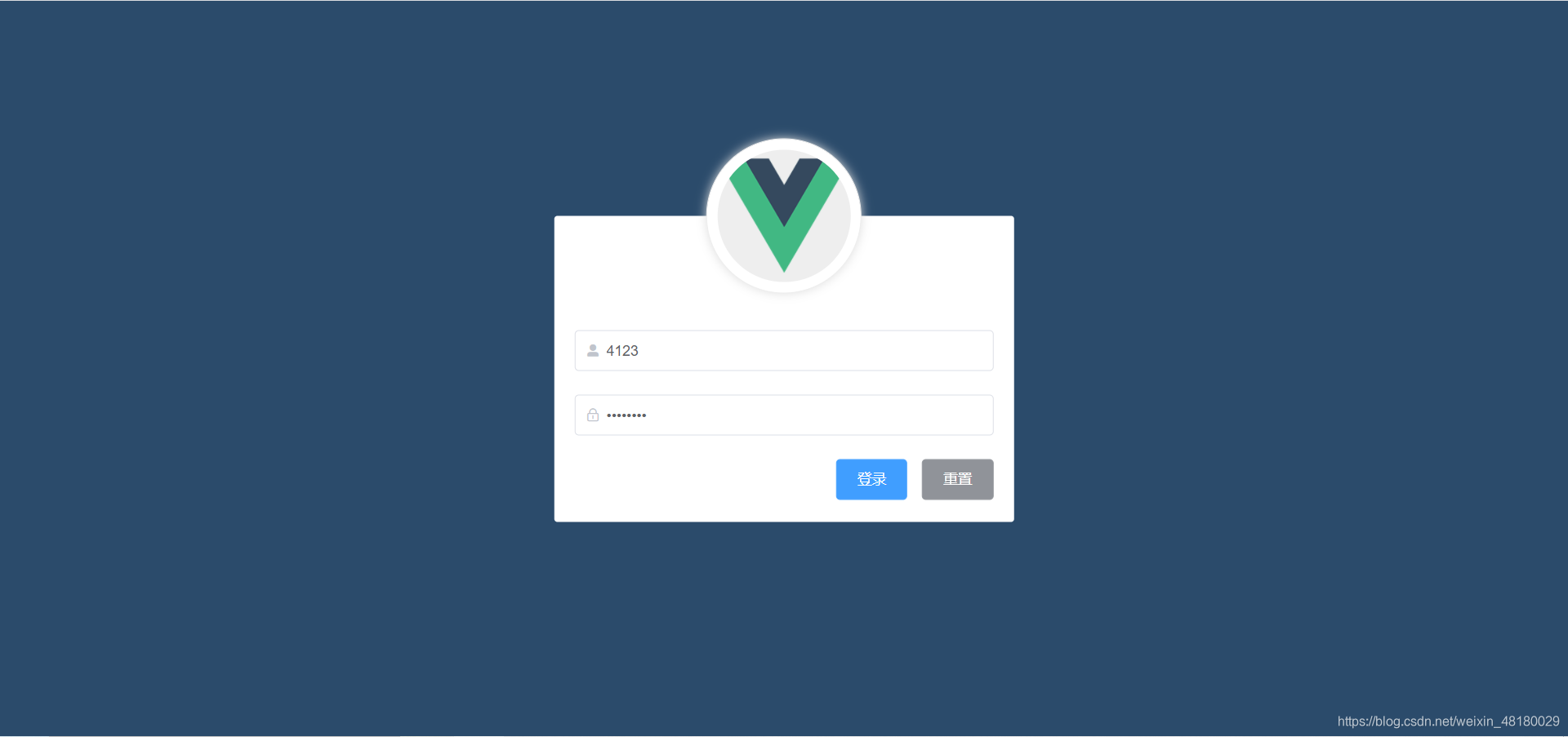
<template>
<div class="login_container">
<div class="login_box">
<div class="avatar_box">
<img src="../assets/logo.png">
</div>
<el-form ref="loginForm" :model="loginForm" :rules="rules" label-width="0px" class="login_form">
<el-form-item prop="username" >
<el-input v-model="loginForm.username" prefix-icon="el-icon-user-solid" ></el-input>
</el-form-item>
<el-form-item prop="password">
<el-input v-model="loginForm.password" type="password" prefix-icon="el-icon-lock"></el-input>
</el-form-item>
<el-form-item class="btns">
<el-button type="primary" @click="login">登录</el-button>
<el-button type="info" @click=" resetForm">重置</el-button>
</el-form-item>
</el-form>
</div>
</div>
</template>
<script>
export default {
name: "",
data(){
return{
loginForm:{
username:'',
password:''
},
rules:{
username:[
{required:true,message:"请输入登录昵称",trigger:"blur"},
{min:3,max:10,message: "长度在3到10个字符",trigger: "blur"}
],
password:[
{required:true,message:"请输入登录密码",trigger:'blur'},
{min:6,max:15,message:"长度在6到15个字符",trigger: 'blur'}
]
}
}
},
methods:{
login(){
this.$refs['loginForm'].validate((valid)=>{
return false
})
},
resetForm(){
this.$refs['loginForm'].resetFields();
}
}
}
</script>
<style lang="less" scoped>
.login_container {
background-color: #2b4b6b;
height: 100%;
}
.login_box {
width: 450px;
height: 300px;
background-color: #fff;
border-radius: 3px;
position: absolute;//居中
left: 50%;
top:50%;
transform: translate(-50%,-50%);
.avatar_box{
height: 130px;
width: 130px;
border: 1px solid #eee;
border-radius: 50%;
padding: 10px;
box-shadow: 0 0 10px #ddd;
position: absolute;
left:50%;
transform: translate(-50%,-50%);
background-color: #fff;
img{
width: 100%;
height: 100%;
border-radius: 50%;
background-color: #eee;
}
}
}
.btns {
display: flex;
justify-content: flex-end;
}
.login_form {
position: absolute;
bottom:0;
width:100%;
padding: 0 20px;
box-sizing: border-box;
}
</style>
home
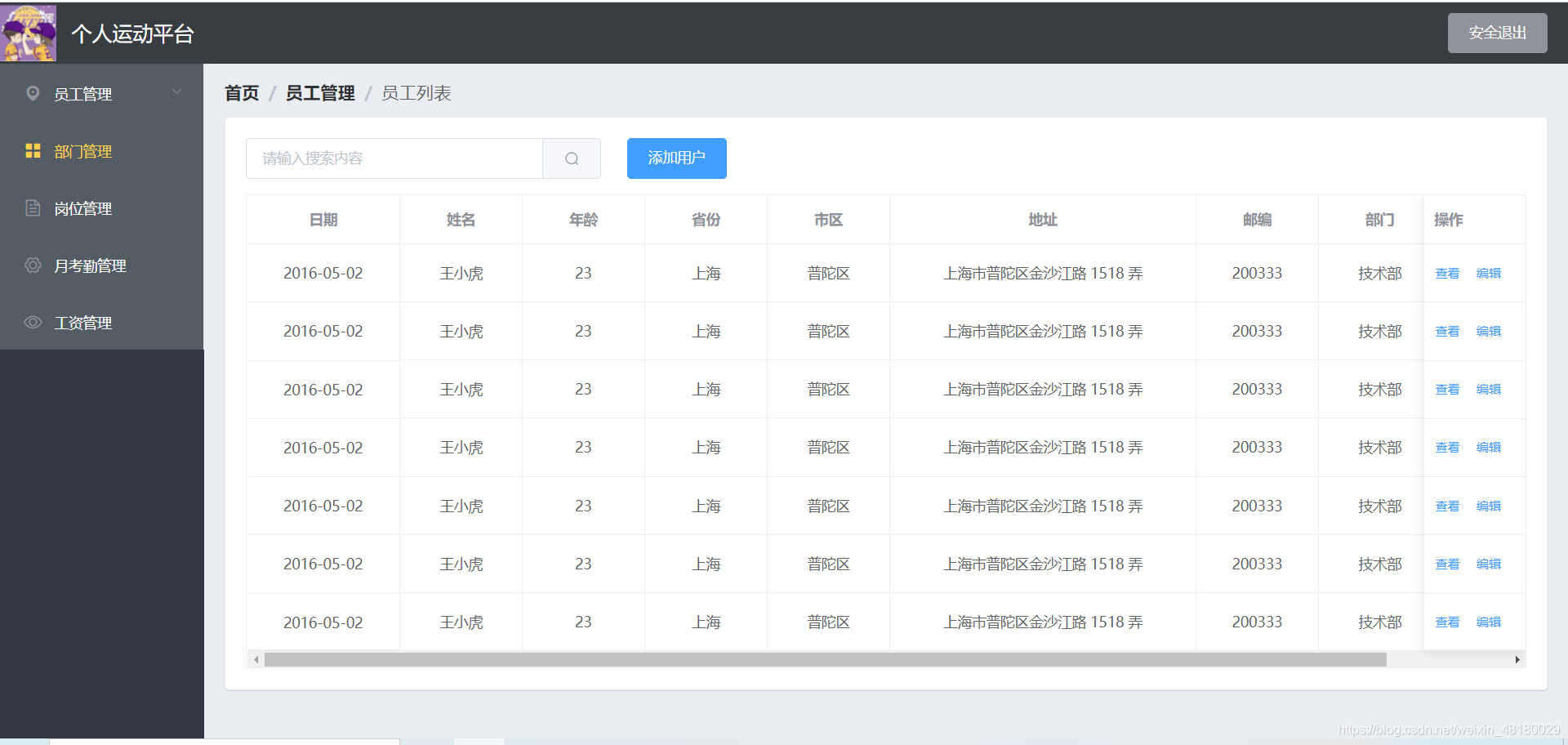
<template>
<el-container class="home-container">
<el-header>
<div>
<img src="../assets/img/profile.jpg" alt=""/>
<span>个人运动平台</span>
</div>
<el-button type="info">安全退出</el-button>
</el-header>
<el-container>
<el-aside width="200px">
<el-menu
default-active="2"
class="el-menu-vertical-demo"
@open="handleOpen"
@close="handleClose"
background-color="#545c64"
text-color="#fff"
active-text-color="#ffd04b">
<el-submenu index="1">
<template slot="title">
<i class="el-icon-location"></i>
<span>员工管理</span>
</template>
<el-menu-item index="1-1" @click="router_userList">
员工列表
</el-menu-item>
</el-submenu>
<el-menu-item index="2">
<i class="el-icon-menu"></i>
<span slot="title">部门管理</span>
</el-menu-item>
<el-menu-item index="3">
<i class="el-icon-document"></i>
<span slot="title">岗位管理</span>
</el-menu-item>
<el-menu-item index="4">
<i class="el-icon-setting"></i>
<span slot="title">月考勤管理</span>
</el-menu-item>
<el-menu-item index="5">
<i class="el-icon-view"></i>
<span slot="title">工资管理</span>
</el-menu-item>
</el-menu>
</el-aside>
<el-main>
<router-view></router-view>
</el-main>
</el-container>
</el-container>
</template>
<script>
export default {
name: "",
methods:{
router_userList(){
this.$router.push('/userList')
}
}
}
</script>
<style lang="less" scoped>
//头部样式
.el-header{
background-color: #373d41;
//background-color: #f1f1f1;
display: flex;
justify-content: space-between;//左右贴边
align-items: center;//水平居中
padding-left: 0;
color: #fff;
font-size: 20px;
>div{
display: flex;
align-items: center;//水平居中
}
span{
margin-left: 15px;
}
}
.el-aside{
background-color: #333744;
}
.el-main{
background-color: #eaedf1;
}
.home-container{
height: 100%;
}
img{
width: 55px;
height: 55px;
}
</style>
<template>
<div>
<el-breadcrumb separator="/">
<el-breadcrumb-item :to="{ path: '/home' }">首页</el-breadcrumb-item>
<el-breadcrumb-item><a href="#">员工管理</a></el-breadcrumb-item>
<el-breadcrumb-item>员工列表</el-breadcrumb-item>
</el-breadcrumb>
<el-card>
<el-row :gutter="25">
<el-col :span="7">
<el-input placeholder="请输入搜索内容">
<el-button slot="append" icon="el-icon-search"></el-button>
</el-input>
</el-col>
<el-col :span="4">
<el-button type="primary">添加用户</el-button>
</el-col>
</el-row>
<el-table
:data="tableData"
border
style="width: 100%">
<el-table-column
fixed
prop="date"
label="日期"
align="center"
width="150">
</el-table-column>
<el-table-column
prop="name"
label="姓名"
align="center"
width="120">
</el-table-column>
<el-table-column
prop="age"
label="年龄"
align="center"
width="120">
</el-table-column>
<el-table-column
prop="province"
label="省份"
align="center"
width="120">
</el-table-column>
<el-table-column
prop="city"
label="市区"
align="center"
width="120">
</el-table-column>
<el-table-column
prop="address"
label="地址"
align="center"
width="300">
</el-table-column>
<el-table-column
prop="zip"
label="邮编"
align="center"
width="120">
</el-table-column>
<el-table-column
prop="depart"
label="部门"
align="center"
width="120">
</el-table-column>
<el-table-column
prop="tel"
label="联系方式"
align="center"
width="120">
</el-table-column>
<el-table-column
fixed="right"
label="操作"
width="100">
<template slot-scope="scope">
<el-button @click="handleClick(scope.row)" type="text" size="small">查看</el-button>
<el-button type="text" size="small">编辑</el-button>
</template>
</el-table-column>
</el-table>
</el-card>
</div>
</template>
<script>
export default {
name: "",
data(){
return{
tableData: [{
date: '2016-05-02',
name: '王小虎',
age:23,
province: '上海',
city: '普陀区',
address: '上海市普陀区金沙江路 1518 弄',
zip: 200333,
depart:'技术部',
tel:'15739282053'
},
{
date: '2016-05-02',
name: '王小虎',
age:23,
province: '上海',
city: '普陀区',
address: '上海市普陀区金沙江路 1518 弄',
zip: 200333,
depart:'技术部',
tel:'15739282053'
},
{
date: '2016-05-02',
name: '王小虎',
age:23,
province: '上海',
city: '普陀区',
address: '上海市普陀区金沙江路 1518 弄',
zip: 200333,
depart:'技术部',
tel:'15739282053'
},
{
date: '2016-05-02',
name: '王小虎',
age:23,
province: '上海',
city: '普陀区',
address: '上海市普陀区金沙江路 1518 弄',
zip: 200333,
depart:'技术部',
tel:'15739282053'
},
{
date: '2016-05-02',
name: '王小虎',
age:23,
province: '上海',
city: '普陀区',
address: '上海市普陀区金沙江路 1518 弄',
zip: 200333,
depart:'技术部',
tel:'15739282053'
},
{
date: '2016-05-02',
name: '王小虎',
age:23,
province: '上海',
city: '普陀区',
address: '上海市普陀区金沙江路 1518 弄',
zip: 200333,
depart:'技术部',
tel:'15739282053'
},
{
date: '2016-05-02',
name: '王小虎',
age:23,
province: '上海',
city: '普陀区',
address: '上海市普陀区金沙江路 1518 弄',
zip: 200333,
depart:'技术部',
tel:'15739282053'
},
]
}
},
methods:{
handleClick(row) {
console.log(row);
}
}
}
</script>
<style lang="less" scoped>
.el-breadcrumb{
margin-bottom: 15px;
font-size: 17px;
}
.el-card{
box-shadow: 0 1px 1px rgba(0,8,10,0.15) !important;
}
//表格样式
.el-table{
margin-top: 15px;
font-size: 14px;
min-width: 350px;
}
</style>