画饼图
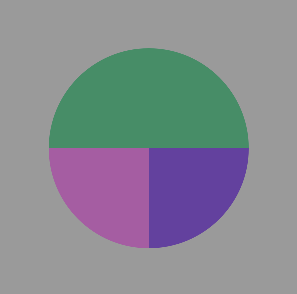
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event
{
[self setNeedsDisplay];
}
- (void)drawRect:(CGRect)rect {
NSArray *data = @[@25,@25,@50];
CGPoint center = CGPointMake(rect.size.width * 0.5, rect.size.height * 0.5);
CGFloat startA = 0;
CGFloat endA = 0;
CGFloat angle = 0;
for (NSNumber *num in data) {
startA = endA;
angle = [num integerValue] / 100.0 * M_PI * 2;
endA = startA + angle;
UIBezierPath *path = [UIBezierPath bezierPathWithArcCenter:center radius:100 startAngle:startA endAngle:endA clockwise:YES];
[path addLineToPoint:center];
[[self randomColor] set];
[path fill];
}
}
- (UIColor *)randomColor
{
CGFloat r = arc4random_uniform(256) / 255.0;
CGFloat g = arc4random_uniform(256) / 255.0;
CGFloat b = arc4random_uniform(256) / 255.0;
return [UIColor colorWithRed:r green:g blue:b alpha:1];
}
模仿下载进度
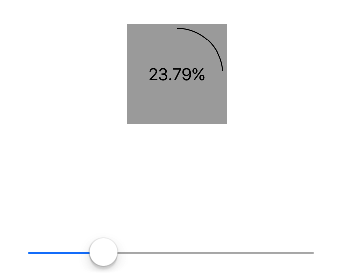
@interface ViewController ()
@property (weak, nonatomic) IBOutlet UILabel *labelView;
@property (weak, nonatomic) IBOutlet ProgressView *progressView;
@end
@implementation ViewController
- (IBAction)valueChange:(UISlider *)sender {
_labelView.text = [NSString stringWithFormat:@"%.2f%%",sender.value * 100];
_progressView.progress = sender.value;
}
@implementation ProgressView
- (void)setProgress:(CGFloat)progress
{
_progress = progress;
[self setNeedsDisplay];
}
- (void)drawRect:(CGRect)rect {
NSLog(@"%s",__func__);
CGPoint center = CGPointMake(rect.size.width * 0.5, rect.size.height * 0.5);
CGFloat endA = - M_PI_2 + M_PI * 2 * _progress;
UIBezierPath *path = [UIBezierPath bezierPathWithArcCenter:center radius:rect.size.width * 0.5 - 4 startAngle:-M_PI_2 endAngle:endA clockwise:YES];
[path stroke];
}
绘制文字
- (void)drawText
{
NSString *str = @"hello world hello world hello world";
NSMutableDictionary *strAttr = [NSMutableDictionary dictionary];
strAttr[NSFontAttributeName] = [UIFont systemFontOfSize:50];
strAttr[NSForegroundColorAttributeName] = [UIColor redColor];
strAttr[NSStrokeWidthAttributeName] = @1;
NSShadow *shadow = [[NSShadow alloc] init];
shadow.shadowOffset = CGSizeMake(10, 10);
shadow.shadowColor = [UIColor yellowColor];
shadow.shadowBlurRadius = 5;
strAttr[NSShadowAttributeName] = shadow;
[str drawInRect:self.bounds withAttributes:strAttr];
}
绘制图片
- (void)drawRect:(CGRect)rect {
UIImage *image = [UIImage imageNamed:@"阿狸头像"];
[image drawInRect:rect];
}
旋转,平移,缩放
- (void)drawRect:(CGRect)rect {
CGContextRef ctx = UIGraphicsGetCurrentContext();
CGContextTranslateCTM(ctx, 100, 100);
CGContextRotateCTM(ctx, M_PI_4);
CGContextScaleCTM(ctx, 0.5, 0.5);
UIBezierPath *path = [UIBezierPath bezierPathWithOvalInRect:CGRectMake(-50, -100, 100, 200)];
[[UIColor redColor] set];
[path fill];
}