C++ 与cocos2d-x-4.0完成太空飞机大战 (三)
动画演示
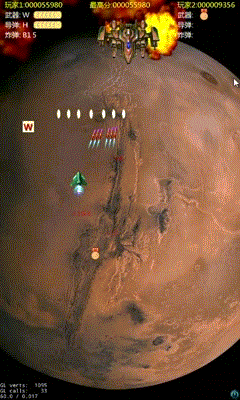
飞机炸弹精灵编码:BombSprite.cpp
#include "BombSprite.h"
#include "ActionBase.h"
#include "Util.h"
namespace SpaceWar {
BombSprite::BombSprite()
{
}
BombSprite::~BombSprite()
{
}
void BombSprite::update(float dt)
{
}
}
飞机炸弹精灵编码:BombSprite.h
#pragma once
#include "cocos2d.h"
#include "SpriteBase.h"
using namespace std;
using namespace cocos2d;
namespace SpaceWar
{
class ActionBase;
class BombSprite : public SpriteBase
{
public:
BombSprite();
virtual ~BombSprite();
void update(float dt);
ActionBase* actBase = nullptr;
int playerTag = 0;
int bombType = 0;
int bombSpeed = 4;
int bombPower = 6;
int collisionCount = 0;
int maxCollisionCount = 0;
int category = 0b111;
int collision = 0b000;
int contact = 0b110;
bool gravityEnable = false;
bool collisionEnable = false;
};
}
飞机子弹精灵编码:BulletSprite.cpp
#include "BulletSprite.h"
#include "ActionBase.h"
#include "Util.h"
namespace SpaceWar {
BulletSprite::BulletSprite()
{
}
BulletSprite::~BulletSprite()
{
}
void BulletSprite::update(float dt)
{
if (isTrack)
{
Vec2 pointStart = this->getPosition();
Node* trackNode = Util::findNode(&actBase->enemyNodes, trackKey);
if (trackNode != nullptr)
{
trackPoint = trackNode->getPosition();
moveSpeed = Util::getTrackPosition(bulletSpeed, pointStart, trackPoint);
}
Vec2 newPosition = pointStart + moveSpeed;
float deltaRotation = Util::getTrackRotation(pointStart, newPosition);
this->setPosition(newPosition);
this->setRotation(deltaRotation);
outSceneRemove();
}
}
void BulletSprite::outSceneRemove()
{
if (Util::isOutScene(this, actBase->vSize))
{
this->stopAllActions();
this->removeFromParentAndCleanup(true);
}
}
}
飞机子弹精灵编码:BulletSprite.h
#pragma once
#include <map>
#include <string>
#include "cocos2d.h"
#include "SpriteBase.h"
using namespace std;
using namespace cocos2d;
namespace SpaceWar
{
class ActionBase;
class BulletSprite : public SpriteBase
{
public:
BulletSprite();
virtual ~BulletSprite();
void update(float dt);
void outSceneRemove();
ActionBase* actBase = nullptr;
int playerTag = 0;
int bulletType = 0;
float bulletSpeed = 4.0f;
int bulletPower = 2;
bool isTrack = false;
string trackKey = "";
Vec2 trackPoint = Vec2(0, 0);
Vec2 moveSpeed = Vec2(0, 0);
int collisionCount = 0;
int maxCollisionCount = 0;
int category = 0b111;
int collision = 0b000;
int contact = 0b110;
bool gravityEnable = false;
bool collisionEnable = false;
};
}
精灵基类编码:SpriteBase.cpp
#include "SpriteBase.h"
namespace SpaceWar {
SpriteBase::SpriteBase()
{
}
SpriteBase::~SpriteBase()
{
}
SpriteBase* SpriteBase::createWithTexture(SpriteBase* sprite, Texture2D *texture)
{
if (sprite && sprite->initWithTexture(texture))
{
sprite->autorelease();
return sprite;
}
CC_SAFE_DELETE(sprite);
return nullptr;
}
SpriteBase* SpriteBase::createWithTexture(SpriteBase* sprite, Texture2D *texture, const Rect& rect, bool rotated)
{
if (sprite && sprite->initWithTexture(texture, rect, rotated))
{
sprite->autorelease();
return sprite;
}
CC_SAFE_DELETE(sprite);
return nullptr;
}
SpriteBase* SpriteBase::create(SpriteBase* sprite, const std::string& filename)
{
if (sprite && sprite->initWithFile(filename))
{
sprite->autorelease();
return sprite;
}
CC_SAFE_DELETE(sprite);
return nullptr;
}
SpriteBase* SpriteBase::create(SpriteBase* sprite, const PolygonInfo& info)
{
if (sprite && sprite->initWithPolygon(info))
{
sprite->autorelease();
return sprite;
}
CC_SAFE_DELETE(sprite);
return nullptr;
}
SpriteBase* SpriteBase::create(SpriteBase* sprite, const std::string& filename, const Rect& rect)
{
if (sprite && sprite->initWithFile(filename, rect))
{
sprite->autorelease();
return sprite;
}
CC_SAFE_DELETE(sprite);
return nullptr;
}
SpriteBase* SpriteBase::createWithSpriteFrame(SpriteBase* sprite, SpriteFrame *spriteFrame)
{
if (sprite && spriteFrame && sprite->initWithSpriteFrame(spriteFrame))
{
sprite->autorelease();
return sprite;
}
CC_SAFE_DELETE(sprite);
return nullptr;
}
SpriteBase* SpriteBase::createWithSpriteFrameName(SpriteBase* sprite, const std::string& spriteFrameName)
{
SpriteFrame *frame = SpriteFrameCache::getInstance()->getSpriteFrameByName(spriteFrameName);
#if COCOS2D_DEBUG > 0
char msg[256] = { 0 };
sprintf(msg, "Invalid spriteFrameName: %s", spriteFrameName.c_str());
CCASSERT(frame != nullptr, msg);
#endif
return createWithSpriteFrame(sprite, frame);
}
SpriteBase* SpriteBase::create(SpriteBase* sprite)
{
if (sprite && sprite->init())
{
sprite->autorelease();
return sprite;
}
CC_SAFE_DELETE(sprite);
return nullptr;
}
}
精灵基类编码:SpriteBase.h
#pragma once
#include "cocos2d.h"
using namespace std;
using namespace cocos2d;
namespace SpaceWar
{
class SpriteBase : public Sprite
{
public:
SpriteBase();
virtual ~SpriteBase();
static const int INDEX_NOT_INITIALIZED = -1;
static SpriteBase* create(SpriteBase* sprite);
static SpriteBase* create(SpriteBase* sprite, const std::string& filename);
static SpriteBase* create(SpriteBase* sprite, const PolygonInfo& info);
static SpriteBase* create(SpriteBase* sprite, const std::string& filename, const Rect& rect);
static SpriteBase* createWithTexture(SpriteBase* sprite, Texture2D *texture);
static SpriteBase* createWithTexture(SpriteBase* sprite, Texture2D *texture, const Rect& rect, bool rotated = false);
static SpriteBase* createWithSpriteFrame(SpriteBase* sprite, SpriteFrame *spriteFrame);
static SpriteBase* createWithSpriteFrameName(SpriteBase* sprite, const std::string& spriteFrameName);
int spriteType = 0;
};
}
C++ 与cocos2d-x-4.0完成太空飞机大战 (四)