上午
python
开发工具
:
1
、安装自带的
ide
2
、
pycharm
(付费)
3
、
anaconda
1
、环境
python2
内置,需要换为
python3
[
root
@
3
~
]
# pip3 install -i https://pypi.tuna.tsinghua.edu.cn/simple/ some
package //
切换国内下载
[
root
@
3
~
]
# yum list installed | grep python
[
root
@
3
~
]
# yum list installed | grep epel
[
root
@
3
~
]
# yum list | grep python3
[
root
@
3
~
]
# yum -y install python3.x86_64
[
root
@
3
~
]
# python3 --version
Python
3.6.8
#
最新版
3.12
版可以使用源码安装
[
root
@
3
~
]
# python3 #
进入到
python
的编辑状态
Python
3.6.8
(
default
,
Nov
14 2023
,
16
:
29
:
52
)
[
GCC
4.8.5 20150623
(
Red Hat
4.8.5
-
44
)]
on linux
Type
"help"
,
"copyright"
,
"credits"
or
"license"
for
more information
.
>>>
print
(
"hello world"
)
hello world
#
如果直接输入
python
,会直接进入到
python2
中
2
、变量和数据类型
1
、三大类数据类型
字符
字符串
str
数值
整数
int
,浮点
float
逻辑
True False
(注意首字母大写)
>>>
print
(
1
==
1
)
True
>>>
print
(
1
!=
1
)
False
>>>
b
=
'zhangsan'
>>>
type
(
b
)
<
type
'str'
>
>>>
c
=
3
>>>
type
(
c
)
<
type
'int'
>
>>>
d
=
3.14
>>>
type
(
d
)
<
type
'float'
>
3
、数据集合
计算是在
python
内存中计算的,必须要有指定内存空间保存数据
这些内存空间其实就是变量(
a,b,c
)
我们使用数据集合批量管理内存空间
[]
列表,
{}
字典,
()
元组
(
1
)列表
在
python
中列表是使用最为广泛的一个数据集合工具
是
java
中数组和
list
的综合体
当有多个数据需要管理,可以定义一个列表(管理列表)
# python
为开发提供了丰富的使用手册
help
(
lista
)
#
通过上下方向,
enter
,
space
键来翻阅信息,使用
q
退出查看
more less
#
创建列表
lista
=
[]
listc
=
[
1
,
2
,
3
]
#
修改列表
#
追加元素
lista
.
append
(
item
)
#
在所有元素之后添加元素
#
插入元素
listb
.
insert
(
pos
,
item
)
#
在
pos
序号之前插入
item
#
删除元素
remove
和
pop
list
.
pop
()
#
删除
list
中的最后一个元素
list
.
remove
(
list
[
index
])
#
删除序号为
index
的元素
#
修改元素
list
[
index
]
=
newvalue
#
删除列表
del
list
练习:
>>>
listb
=
[
"tom"
,
"jerry"
]
>>>
listb
[
'tom'
,
'jerry'
]
>>>
listb
.
append
(
"tomcat"
)
>>>
listb
[
'tom'
,
'jerry'
,
'tomcat'
]
>>>
listc
=
[
"tom"
,
"jerry"
]
>>>
listc
.
pop
()
'jerry'
>>>
listc
[
'tom'
]
>>>
listd
=
listb
.
pop
()
>>>
listd
'tom'
>>>
liste
=
listb
.
pop
()
Traceback
(
most recent call last
):
File
"<stdin>"
,
line
1
,
in
<
module
>
IndexError
:
pop
from
empty
list
>>>
listb
[]
#
当在列表中删除或者修改一个元素的时候,列表会返回新的列表
>>>
listb
.
append
(
'lisi'
)
>>>
listb
.
append
(
'zhangsan'
)
>>>
listb
.
append
(
'wangwu'
)
>>>
listb
[
'lisi'
,
'zhangsan'
,
'wangwu'
]
>>>
listb
[
0
]
'lisi'
>>>
listb
[
1
]
'zhangsan'
>>>
listb
[
2
]
'wangwu'
>>>
listb
.
remove
(
listb
[
1
])
>>>
listb
[
'lisi'
,
'wangwu'
]
(
2
)字典
dict
dictionary
key-value
键值对
{"name":"
张三
","age":"19","gender":"male","height":"145","weight":"180"}
健
:
值
如下:
{
"from"
:
"me"
,
"to"
:
"you"
,
"message"
:
"
你吃饭了吗?
"
,
"time"
:
"2024-7-8 9:00:32"
,
"user"
:{
"username"
:
"abc"
,
"password"
:
"abc"
}
}
#
创建字典
dict0
=
{
健
:
值
,
健
:
值
}
#
追加元素
dict0
[
"
健
"
]
=
"
值
"
#
删除元素
dict0
.
pop
(
"
健
"
)
#
修改元素
dict0
[
"
存在的健
"
]
=
"
修改后的值
"
练习:
>>>
dict0
=
{
...
"id"
:
1
,
...
"username"
:
"abc"
,
...
"password"
:
"123"
...
}
>>>
help
(
dict0
)
>>>
dict0
{
'id'
:
1
,
'username'
:
'abc'
,
'password'
:
'123'
}
>>>
dict0
[
"realname"
]
=
"zhangsan"
>>>
dict0
{
'id'
:
1
,
'username'
:
'abc'
,
'password'
:
'123'
,
'realname'
:
'zhangsan'
}
>>>
dict0
.
pop
(
"id"
)
1
>>>
dict0
{
'username'
:
'abc'
,
'password'
:
'123'
,
'realname'
:
'zhangsan'
}
>>>
dict0
[
"password"
]
=
"123456"
>>>
dict0
{
'username'
:
'abc'
,
'password'
:
'123456'
,
'realname'
:
'zhangsan'
}
# list
列表与
dict
字典嵌套
>>>
a
=
[
1
,
2
,
3
]
>>>
b
=
{
"username"
:
"abc"
,
"password"
:
"abc"
}
>>>
a
[
1
,
2
,
3
]
>>>
b
{
'username'
:
'abc'
,
'password'
:
'abc'
}
>>>
a
.
append
(
b
)
>>>
b
[
"a"
]
=
a
>>>
a
[
1
,
2
,
3
, {
'username'
:
'abc'
,
'password'
:
'abc'
,
'a'
: [
...
]}]
>>>
b
{
'username'
:
'abc'
,
'password'
:
'abc'
,
'a'
: [
1
,
2
,
3
, {
...
}]}
(
3
)元组
不能修改,只可以查看
tuple[index]
,
list(tuple)
,
tuple(list)
list()
可以把
dict
的
key
生成一个列表
list()
可以把
tupl
变成列表
tupl
可以把
dict
和
list
变成元组
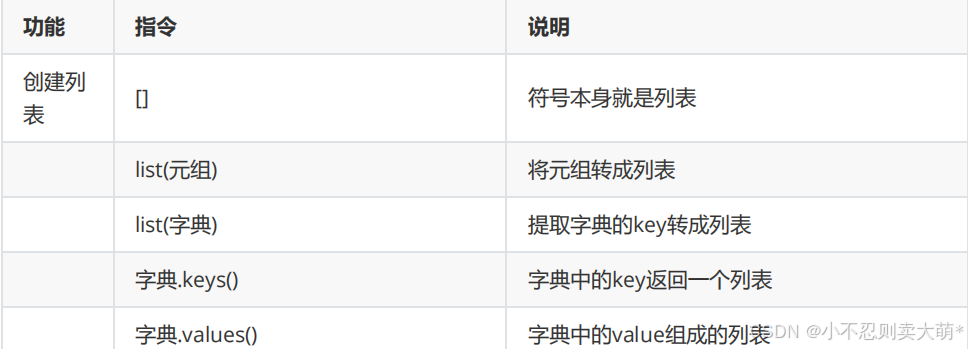
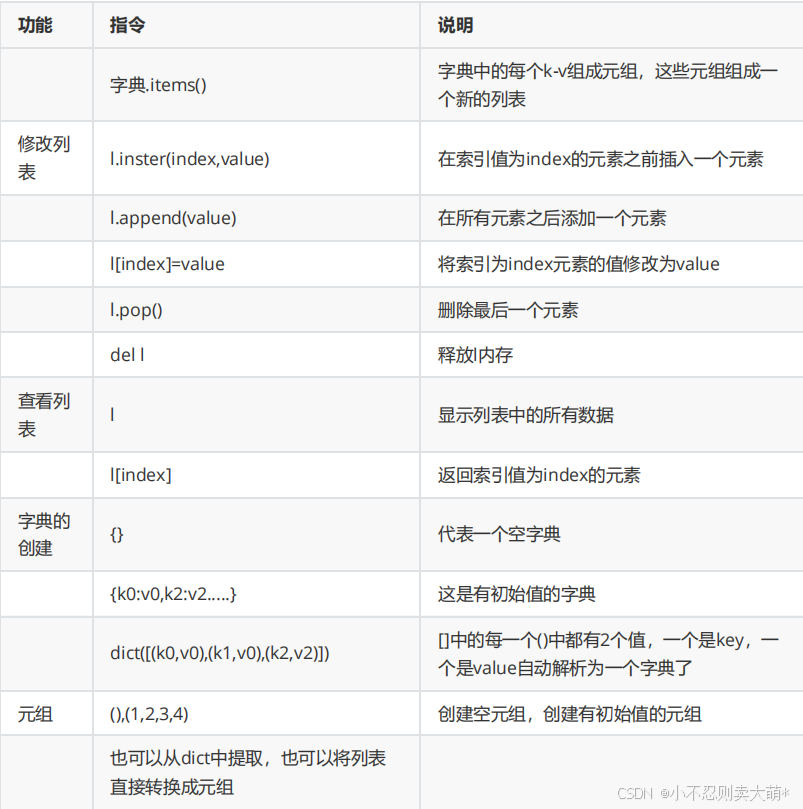
登录信息返回的键值对:
{
"method"
:
"post"
,
"username"
:
"abc"
,
"password"
:
"123"
,
"params"
:[
1
,
2
,
3
],
"cntroller"
:
"login"
}
4
、选择语句和循环语句
(
1
)
if
选择语句
缩进是必须的
(一个
tab
健或者四个空格)
if
condition0
:
statement0
if
condition1
:
block1
else
:
block2
else
:
statement1
多分支
if
语句
if
condition0
:
block0
elif
condition1
:
block1
elif
condition2
:
block2
...
else
:
blockn
练习:
[
root
@
3
~
]
# vim py001.py
if True
:
print
(
"i'm true"
)
else
:
print
(
"i'm false"
)
[
root
@
3
~
]
# python3 py001.py
i
'm true
>>>
n
=
58
>>>
if
n
>
90
:
...
print
(
"
优秀
"
)
...
elif
n
>
80
:
...
print
(
"
良好
"
)
...
elif
n
>
70
:
...
print
(
"
中等
"
)
...
elif
n
>
60
:
...
print
(
"
及格
"
)
...
else
:
...
print
(
"
不及格
"
)
...
不及格
[
root
@
3
~
]
# vim py002.py
import
random
n
=
random
.
randint
(
0
,
100
)
print
(
"
随机分数为:
"
,
n
)
if
n
>
90
:
print
(
"
优秀
"
)
elif
n
>
80
:
print
(
"
良好
"
)
elif
n
>
70
:
print
(
"
中等
"
)
elif
n
>
60
:
print
(
"
及格
"
)
else
:
print
(
"
不及格
"
)
[
root
@
3
~
]
# python3 py002.py
随机分数为:
68
及格
[
root
@
3
~
]
# vim py003.py
import
random
n
=
random
.
randint
(
50
,
100
)
print
(
"
随机数值为:
"
,
n
)
if
n
>
90
:
print
(
"youxiu"
)
else
:
if
n
>
80
:
print
(
"lianghao"
)
else
:
if
n
>
70
:
print
(
"zhongdeng"
)
else
:
if
n
>
60
:
print
(
"jige"
)
else
:
print
(
"bujige"
)
[
root
@
3
~
]
# python3 py003.py
随机数值为:
93
youxiu
(
2
)
swith
插槽
(
3
)
input
与
print
>>>
print
(
"
请输入您的选择
"
)
请输入您的选择
>>>
print
(
"1
、创建
master
,
2
、创建
slave"
)
1
、创建
master
,
2
、创建
slave
>>>
input
(
"---:"
)
---
:
1
'1'
>>>
input
(
"---:"
)
---
:
2
'2'
(
3
)
for
循环语句
练习:
>>>
list
=
[
1
,
2
,
3
,
4
,
5
]
>>>
for
var
in
list
:
#
列表遍历
...
print
(
var
)
...
1
2
3
4
5
>>>
for
var
in
[
"a"
,
"b"
,
"c"
]:
#
列表遍历
...
print
(
var
)
...
a
b
c
>>>
d
=
{
"a"
:
1
,
"b"
:
2
,
"c"
:
3
}
#
字典遍历
key
>>>
for
var
in
d
:
...
print
(
var
)
...
a
b
c
>>>
for
var
in
d
:
#
字典遍历
value
...
print
(
d
[
var
])
...
1
2
3
>>>
tupl0
=
(
"a"
,
"b"
,
"c"
)
#
遍历元组
>>>
for
var
in
tupl0
:
...
print
(
var
)
...
a
b
c
>>>
range
(
9
)
range
(
0
,
9
)
>>>
list
(
range
(
9
))
[
0
,
1
,
2
,
3
,
4
,
5
,
6
,
7
,
8
]
>>>
for
i
in
range
(
9
):
...
print
(
i
)
...
0
1
2
3
4
5
6
7
8
>>>
for
i
in
range
(
101
):
...
n
=
n
+
i
...
>>>
n
5050
[
root
@
3
~
]
# vim py004.py
n
=
0
for
i
in
range
(
101
):
n
=
n
+
i
print
(
n
)
[
root
@
3
~
]
# python3 py004.py
5050
(
4
)
while
循环语句(
continue
与
break
)
break
和
continue
也可以应⽤于
for
while
condition
:
blocak
#continue,break;
练习:
>>>
i
=
0
>>>
while
i
<
10
:
...
i
+=
1
...
if
i
%
2
!=
0
:
...
continue
...
print
(
i
)
...
2
4
6
8
10
>>>
c
=
0
>>>
while True
:
...
print
(
c
)
...
c
+=
1
...
if
c
==
5
:
...
break
...
0
1
2
3
4
5
、常用的工具
api
#
指令
vim
001.
py
#
执
⾏
py
脚本
python3
001.
py
#
调试
py
脚本
python3
-
m pdb
001.
py
#
输
⼊
n
按回
⻋
执
⾏
下
⼀⾏
代码
#
输
⼊
q
退出调试
#
生成随机数
import
random
n
=
random
.
randint
(
0
,
10
)
#
创建目录
import
os
os
.
mkdir
(
"/opt/aaa"
)
练习:
[
root
@
3
~
]
# python3 -m pdb py003.py
> /
root
/
py003
.
py
(
1
)
<
module
>
()
->
import
random
(
Pdb
)
n
> /
root
/
py003
.
py
(
2
)
<
module
>
()
->
n
=
random
.
randint
(
50
,
100
)
(
Pdb
)
n
> /
root
/
py003
.
py
(
3
)
<
module
>
()
->
print
(
"
随机数值为:
"
,
n
)
(
Pdb
)
n
随机数值为:
92
......
(
Pdb
)
q
>>>
import
random
>>>
n
=
random
.
randint
(
0
,
10
)
>>>
n
10
>>>
n
=
random
.
randint
(
0
,
10
)
>>>
n
4
>>>
import
os
>>>
os
.
mkdir
(
"/opt/aaa"
)
>>>
quit
()
[
root
@
3
~
]
# ls /opt
aaa