提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档
文章目录
在这里插入代码片
提示:以下是本篇文章正文内容,下面案例可供参考
一、STL是什么?
1、标准模版库
2.广义上分为 容器,算法迭代器
3.STL几乎所有代码采用模版类和模版函数
二、容器vector
1.vector的基础语法
容器vector
算法for_each
迭代器vector::iterator
代码实现
#include <iostream>
using namespace std;
#include<vector>
#include<algorithm>
void print(int val) {
cout << val << endl;
}
void test01()
{
vector<int> v;//定义一个容器
v.push_back(1);//给容器赋值
v.push_back(2);
v.push_back(3);
**//第一种遍历方法**
/*vector<int>:: iterator pbegin = v.begin();
vector<int>::iterator pend = v.end();*/
/*while (pbegin != pend)
{
cout << *pbegin << endl;
pbegin++;
}*/
**第二种遍历方法****最常用的遍历方法
**/*for (vector<int>::iterator it = v.begin(); it != v.end(); it++) { cout << *it << endl; }*/
**第三种遍历方法**
for_each(v.begin(),v.end(), print);
}
int main()
{
test01();
system("pause");
return 0;
}
**容器嵌套的实现**
```cpp
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void test()
{
//创建一维向量
vector<int> v1;
vector<int> v2;
vector<int> v3;
vector<int> v4;
vector<int> v5;
vector<int> v6;
//必须先给一维向量赋值
原因如下
for (int i = 0; i < 5; i++)
{
v1.push_back(i+1);
v2.push_back(i+2);
v3.push_back(i+3);
v4.push_back(i+4);
v5.push_back(i+5);
v6.push_back(i+6);
}
//创建二维向量,并且与一维向量建立关系
vector<vector<int>> v;
v.push_back(v1);
v.push_back(v2);
v.push_back(v3);
v.push_back(v4);
v.push_back(v5);
v.push_back(v6);
//遍历 其中(*it).begin()指的就是v.begin().begin()
for (vector<vector<int>>::iterator it = v.begin(); it != v.end(); it++)
{
for (vector<int>::iterator vit = (*it).begin(); vit != (*it).end(); vit++)
{
cout << *vit << " ";
}
cout<<endl;
}
}
int main()
{
test();
system("pause");
return 0;
}
2.vector的构造函数和赋值
vector<int> v1;//无参构造
for (int i = 0; i < 10; i++)
{
v1.push_back(i);
}
p(v1);
vector<int>v2(v1.begin(), v1.end());//用区间构造
p(v2);
vector<int>v3(2, 8);//n个数,这里是两个8
p(v3);
vector<int>v4(v3);//拷贝构造函数
p(v4);
vector<int>v5;
v5 = v1;//用等号赋值
p(v5);
vector<int>v6;
v6.assign(v3.begin(), v3.end());//用assign赋值
p(v6);
vector<int>v7;
v7.assign(2,9);
p(v7);
if (v7.empty())
{
cout << "empty";
}
else
{
cout << "v7个数为" << v7.size() << endl;
cout << "v7容量为" << v7.capacity() << endl;
}
v7.resize(3, 10);
p(v7);
3.vector的插入删除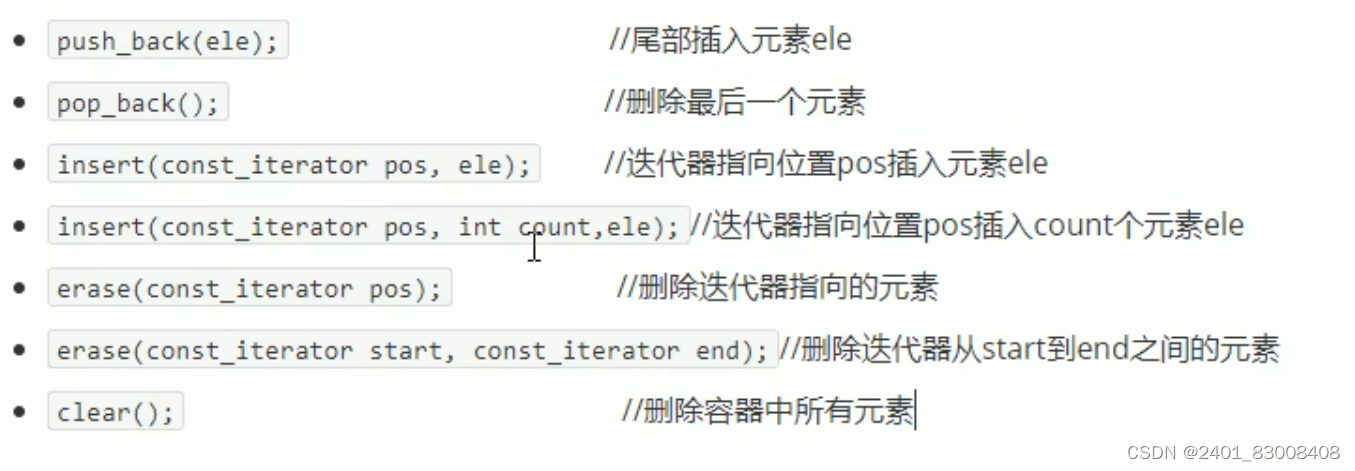
v1.pop_back();
v1.insert(v1.begin(), 4, 3);
v1.erase(v1.begin());
p(v1);
v1.clear();
p(v1);
4.vector的数据存取
vector<int>v8;
for (int i = 1; i < 10; i++)
{
v8.push_back(i);
}
for (int i = 1; i < 9; i++)
{
cout << v8[i] << " ";
}
cout << endl;
for (int i = 1; i < 9; i++)
{
cout << v8.at(i) << " ";
}
cout << endl;
cout << v8.front() << " " << v8.back() << endl;
5.vector的swap函数,巧用匿名对象和swap函数收缩空间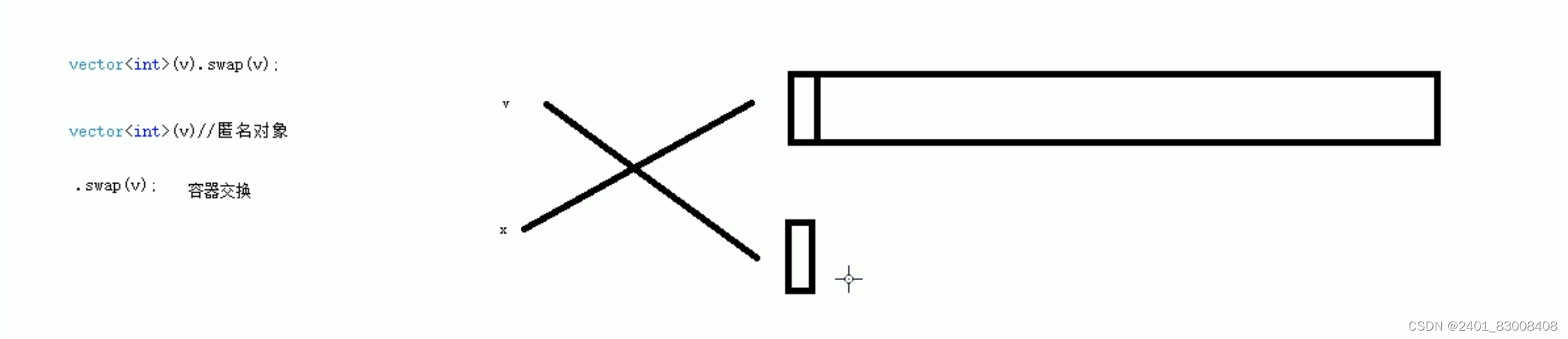
匿名对象是在创建对象时不使用对象名来引用该对象的一种方式。可以直接在创建对象的时候使用该对象进行方法调用或者操作,而不需要将其赋值给一个对象变量。匿名对象主要用于一次性的操作或者方法调用,不需要在其他地方再次使用该对象。一般来说,垃圾回收机制会在程序运行时根据一定的策略来确定何时回收对象,并释放其占用的内存空间。
vector<int>v9;
for (int i = 0; i < 1000; i++)
{
v9.push_back(i);
}
cout << "v9的容量为" << v9.capacity() << endl;
cout << "v9的大小" << v9.size() << endl;
v9.resize(4);
vector<int>(v9).swap(v9);
cout << "v9的容量为" << v9.capacity() << endl;
cout << "v9的大小" << v9.size() << endl;
6.vector预留空间
利用这段代码算一共开辟了多少次空间
vector<int>v10;
int num = 0;
int* p = NULL;
for (int i = 0; i < 1000; i++)
{
v10.push_back(i);
if (p != &v10[0])
{
p = &v10[0];
num++;
}
}
cout << "num为" << num<<endl;
在前面加上v10.reserve(1000)后只需开辟一次
vector<int>v10;
v10.reserve(1000);
int num = 0;
int* p = NULL;
for (int i = 0; i < 1000; i++)
{
v10.push_back(i);
if (p != &v10[0])
{
p = &v10[0];
num++;
}
}
cout << "num为" << num<<endl;
}