效果图如下:
源码:
AirslakeImage.java
package
org.test;

import
java.awt.Button;
import
java.awt.Color;
import
java.awt.Frame;
import
java.awt.Graphics;
import
java.awt.Image;
import
java.awt.Panel;
import
java.awt.event.ActionEvent;
import
java.awt.event.ActionListener;
import
java.awt.event.WindowAdapter;
import
java.awt.event.WindowEvent;
import
java.awt.image.PixelGrabber;
import
java.util.Random;

import
org.loon.framework.game.image.Bitmap;
import
org.loon.framework.game.image.LColor;

/** */
/**
* <p>Title: LoonFramework</p>
* <p>Description:java实现图片风化效果</p>
* <p>Copyright: Copyright (c) 2007</p>
* <p>Company: LoonFramework</p>
* @author chenpeng
* @email:ceponline@yahoo.com.cn
* @version 0.1
*/

public
class
AirslakeImage
extends
Panel
implements
Runnable, ActionListener
...
{


/** *//**
*
*/
private static final long serialVersionUID = 1L;
static final public int _WIDTH = 400;
static final public int _HEIGHT = 400;
private boolean _isRun=false;

private Image _img;
private Image _screen;
private Graphics _back;

private int _imgWidth;
private int _imgHeight;

private Fraction[] _fractions;

private Thread _timer;

private Button _meganteButton;
private Button _revivalButton;


private Random _rand = new Random();


public AirslakeImage() ...{
_screen=new Bitmap(_WIDTH,_HEIGHT).getImage();
_back=_screen.getGraphics();
setSize(_WIDTH, _HEIGHT);

_meganteButton = new Button("破碎图片");
_meganteButton.addActionListener(this);
add(_meganteButton);

_revivalButton = new Button("还原图片");
_revivalButton.addActionListener(this);
add(_revivalButton);
_revivalButton.setEnabled(false);

loadImage("role.png");

init(_img);
}

/** *//**
* 初始化image图像,分解其中像素
* @param _img
*/

private void init(Image _img) ...{

if (_timer != null) ...{
_timer=null;
_isRun=false;
}
_fractions = new Fraction[_imgWidth * _imgHeight];
PixelGrabber pg = new PixelGrabber(_img, 0, 0, _imgWidth, _imgHeight, true);

try ...{
pg.grabPixels();

} catch (InterruptedException e) ...{
e.printStackTrace();
}
int pixel[] = (int[]) pg.getPixels();
//重新封装像素

for (int y = 0; y < _imgHeight; y++) ...{

for (int x = 0; x < _imgWidth; x++) ...{
int n = y * _imgWidth + x;
_fractions[n] = new Fraction();
double angle = _rand.nextInt(360);
double speed = 10.0 / _rand.nextInt(30);
_fractions[n].x = x+90;
_fractions[n].y = y+20;
_fractions[n].vx = Math.cos(angle * Math.PI / 180) * speed;
_fractions[n].vy = Math.sin(angle * Math.PI / 180) * speed;
_fractions[n].color = pixel[n];
_fractions[n].countToCrush = x / 6 + _rand.nextInt(10);
}
}
}

public void update(Graphics g)...{
paint(g);
}

public void paint(Graphics g)...{
//变更背景色
_back.setColor(Color.WHITE);
//清空背景
_back.fillRect(0, 0, _WIDTH, _HEIGHT);


for (int n = 0; n < _imgWidth * _imgHeight; n++) ...{
int x = (int) _fractions[n].x;
int y = (int) _fractions[n].y;
LColor color=LColor.getLColor(_fractions[n].color);
//纯黑色区域不读取

if(!LColor.equals(color, LColor.fromArgb(0,0,0)))...{
//获得rgb三色
int red = color.R;
int green = color.G;
int blue = color.B;
_back.setColor(new Color(red, green, blue));
//绘制
_back.drawLine(x, y, x, y);
}
}
g.drawImage(_screen, 0, 0, this);
}


public void actionPerformed(ActionEvent e) ...{

if (e.getSource() == _meganteButton) ...{
execute();
_meganteButton.setEnabled(false);
_revivalButton.setEnabled(true);

} else if (e.getSource() == _revivalButton) ...{
init(_img);
repaint();
_meganteButton.setEnabled(true);
_revivalButton.setEnabled(false);
}
}




/** *//**
* 加载图像
* @param filename
*/

private void loadImage(String filename) ...{
Bitmap bitmap = new Bitmap(("./image/"+filename).intern());
//替换透明区域颜色(象素化后,转为rgb形式的透明区域色值将显示为r=0,g=0,b=0),可以直接用pixel识别透明区域,也可以替换或跳过该区域)
_img=bitmap.getImage();
_imgWidth = _img.getWidth(this);
_imgHeight = _img.getHeight(this);
}



/** *//**
* 执行操作
*
*/

private void execute() ...{
_timer = new Thread(this);
_timer.start();
_isRun=true;
}

class Fraction ...{
//图片在窗体中x
public double x;
//图片在窗体中y
public double y;
//显示图x
public double vx;
//显示图y
public double vy;
//color
public int color;
//变形颗粒数量
public int countToCrush;
}

public void run() ...{

while(_isRun)...{

for (int n = 0; n < _imgWidth * _imgHeight; n++) ...{

if (_fractions[n].countToCrush <= 0) ...{
_fractions[n].x += _fractions[n].vx;
_fractions[n].y += _fractions[n].vy;
_fractions[n].vy += 0.1;

} else ...{
_fractions[n].countToCrush--;
}
}
//间隔

try ...{
Thread.sleep(60);

} catch (InterruptedException e) ...{
e.printStackTrace();
}
repaint();
}
}

public static void main(String[]args)...{

java.awt.EventQueue.invokeLater(new Runnable() ...{

public void run() ...{
Frame frm = new Frame("java实现图片风化效果");
frm.add(new AirslakeImage());
frm.setResizable(false);
frm.setSize(_WIDTH, _HEIGHT);

frm.addWindowListener(new WindowAdapter()...{

public void windowClosing(WindowEvent e)...{
System.exit(0);
}
});
frm.setLocationRelativeTo(null);
frm.setVisible(true);
}
});
}
}
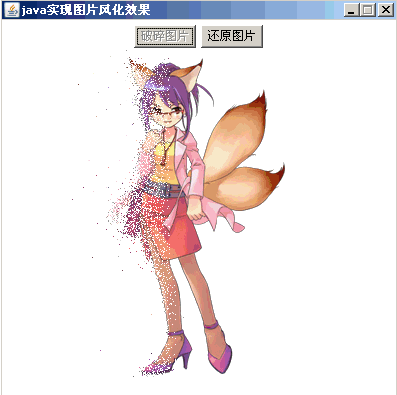
源码:
AirslakeImage.java

















































































































































































































































































再分享一下我老师大神的人工智能教程吧。零基础!通俗易懂!风趣幽默!还带黄段子!希望你也加入到我们人工智能的队伍中来!https://blog.csdn.net/jiangjunshow