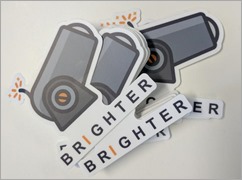
There's a ton of cool new .NET Core open source projects lately, and I've very much enjoyed exploring this rapidly growing space. Today at lunch I was checking out a project called "Brighter." It's actually been around in the .NET space for many years and is in the process of moving to .NET Core for greater portability and performance.
最近,有很多很酷的新.NET Core开源项目,我非常喜欢探索这个快速增长的领域。 今天午餐时,我正在检查一个名为“更明亮”的项目。 它实际上已经存在于.NET领域多年,并且正在迁移到.NET Core以提高可移植性和性能。
Brighter is a ".NET Command Dispatcher, with Command Processor features for QoS (like Timeout, Retry, and Circuit Breaker), and support for Task Queues"
Brighter是“ .NET命令分派器,具有用于QoS的命令处理器功能(如超时,重试和断路器),并支持任务队列”
Whoa, that's a lot of cool and fancy words. What's it mean? The Brighter project is up on GitHub incudes a bunch of libraries and examples that you can pull in to support CQRS architectural styles in .NET. CQRS stands for Command Query Responsibility Segregation. As Martin Fowler says, "At its heart is the notion that you can use a different model to update information than the model you use to read information." The Query Model reads and the Command Model updates/validates. Greg Young gives the first example of CQRS here. If you are a visual learner, there's a video from late 2015 where Ian Cooper explains a lot of this a the London .NET User Group or an interview with Ian Cooper on Channel 9.
哇,这是很多很酷很酷的话。 什么意思GitHub上的Brighter项目包括一堆库和示例,您可以引入这些库和示例来支持.NET中的CQRS建筑风格。 CQRS代表命令查询责任隔离。 正如马丁·福勒(Martin Fowler)所说:“其核心思想是,您可以使用与用于读取信息的模型不同的模型来更新信息。” 查询模型读取,命令模型更新/验证。 Greg Young在这里给出了CQRS的第一个示例。 如果您是视觉学习者,那么可以观看2015年末的视频,其中Ian Cooper会在伦敦.NET用户组中进行很多解释,或者在第9频道上对Ian Cooper的采访。
Brighter also supports "Distributed Task Queues" which you can use to improve performance when you're using a query or integrating with microservices.
Brighter还支持“分布式任务队列”,当您使用查询或与微服务集成时,可以使用它们来提高性能。
When building distributed systems, Hello World is NOT the use case. BUT, it is a valid example in that it strips aside any business logic and shows you the basic structure and concepts.
在构建分布式系统时,“ Hello World”不是用例。 但是,这是一个有效的示例,因为它撇开了任何业务逻辑,并向您展示了基本结构和概念。
Let's say there's a command you want to send. The GreetingCommand. A command can be any write or "do this" type command.
假设您要发送一个命令。 GreetingCommand。 命令可以是任何写入或“执行此”类型的命令。
internal class GreetingCommand : Command
{
public GreetingCommand(string name)
:base(new Guid())
{
Name = name;
}
public string Name { get; private set; }
}
Now let's say that something else will "handle" these commands. This is the DoIt() method. No where do we call Handle() ourselves. Similar to dependency injection, we won't be in the business of calling Handle() ourselves; the underlying framework will abstract that away.
现在,让我们说其他方法将“处理”这些命令。 这是DoIt()方法。 没有我们在哪里自己调用Handle()。 与依赖注入类似,我们不会自己调用Handle(); 底层框架会将其抽象化。
internal class GreetingCommandHandler : RequestHandler<GreetingCommand>
{
[RequestLogging(step: 1, timing: HandlerTiming.Before)]
public override GreetingCommand Handle(GreetingCommand command)
{
Console.WriteLine("Hello {0}", command.Name);
return base.Handle(command);
}
}
We then register a factory that takes types and returns handlers. In a real system you'd use IoC (Inversion of Control) dependency injection for this mapping as well.
然后,我们注册一个接受类型并返回处理程序的工厂。 在真实的系统中,您还将对映射使用IoC(控制反转)依赖项注入。
Our Main() has a registry that we pass into a larger pipeline where we can set policy for processing commands. This pattern may feel familiar with "Builders" and "Handlers."
我们的Main()有一个注册表,我们可以将其传递到更大的管道中,在这里可以设置用于处理命令的策略。 这种模式可能会让您熟悉“ Builders”和“ Handlers”。
private static void Main(string[] args)
{
var registry = new SubscriberRegistry();
registry.Register<GreetingCommand, GreetingCommandHandler>();
var builder = CommandProcessorBuilder.With()
.Handlers(new HandlerConfiguration(
subscriberRegistry: registry,
handlerFactory: new SimpleHandlerFactory()
))
.DefaultPolicy()
.NoTaskQueues()
.RequestContextFactory(new InMemoryRequestContextFactory());
var commandProcessor = builder.Build();
...
}
Once we have a commandProcessor, we can Send commands to it easier and the work will get done. Again, how you ultimately make the commands is up to you.
一旦有了commandProcessor,我们可以更轻松地向其发送命令,并且工作将完成。 同样,您最终如何制作命令取决于您自己。
commandProcessor.Send(new GreetingCommand("HanselCQRS"));
Methods within RequestHandlers can also have other behaviors associated with them, as in the case of "[RequestLogging] on the Handle() method above. You can add other stuff like Validation, Retries, or Circuit Breakers. The idea is that Brighter offers a pipeline of handlers that can all operate on a Command. The Celery Project is a similar project except written in Python. The Brighter project has stated they have lofty goals, intending to one day handle fault tolerance like Netflix's Hystrix project.
RequestHandlers中的方法也可以与其他行为相关联,例如上述Handle()方法中的“ [RequestLogging]”情况。您可以添加其他内容,例如Validation,Retries或Circuit Breakers。想法是Brighter提供了一个可以全部在Command上运行的处理程序流水线; Celery项目是一个类似的项目,但用Python编写; Brighter项目表示他们有崇高的目标,打算像Netflix的Hystrix项目那样,有一天要处理故障。
One of the nicest aspects to Brighter is that it's prescriptive but not heavy-handed. They say:
Brighter最好的方面之一是它具有说明性,但不笨拙。 他们说:
Brighter is intended to be a library not a framework, so it is consciously lightweight and divided into packages that allow you to consume only those facilities that you need in your project.
Brighter旨在成为一个库而不是一个框架,因此它有意识地轻巧,并且分成多个包,这些包使您可以仅使用项目中需要的那些功能。
Moving beyond Hello World, there are more fleshed out examples like a TaskList with a UI, back end Http API, a Mailer service, and core library.
除了Hello World之外,还有更多充实的示例,例如带有UI的TaskList ,后端Http API,Mailer服务和核心库。
Be sure to explore Brighter's excellent documentation and examples, but be aware, this is a project under active development. Perhaps if you're new to OSS, if you find a broken link or two or a misspelling, you can do Your First Pull Request with a small fix?
一定要探索Brighter的出色文档和示例,但是请注意,这是一个正在积极开发的项目。 也许,如果您是OSS的新手,如果发现一个或两个断开的链接或拼写错误,则可以通过一个小的修复程序来执行“首次请求” ?
Do be aware, again, that CQRS is not for every project. It's non-trivial and it's a "mental leap" as Martin Fowler puts it. If you buy in, you're adding complexity...for a reason. Keep your eyes open and do your research. It's a great pattern if you have a high performance/volume application that struggles with write concurrency or a flaky backend.
再次请注意,CQRS并非适用于每个项目。 正如马丁·福勒(Martin Fowler)所说,这是不平凡的,是“精神飞跃”。 如果您买入,那是在增加复杂性……是有原因的。 睁大眼睛,做研究。 如果您有一个高性能/大容量的应用程序在写并发或后端不稳定的情况下工作,这是一个很好的模式。
In fact there are quite a few mature CQRS libraries in the .NET open source space. I'll explore a few - which are your favorites?
实际上,.NET开源空间中有许多成熟的CQRS库。 我将探索一些-您最喜欢的是哪几个?
翻译自: https://www.hanselman.com/blog/exploring-cqrs-within-the-brighter-net-open-source-project