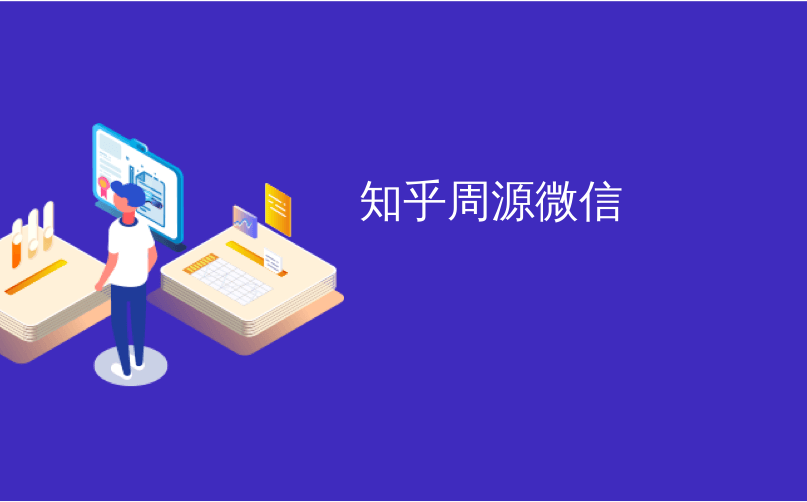
知乎周源微信
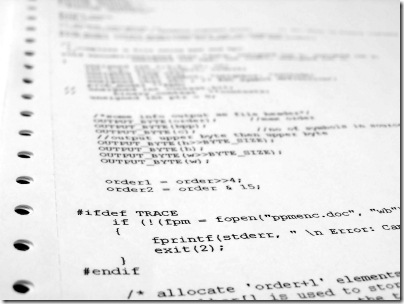
If you're new to this, each week I post some snippets of particularly interesting (read: beautiful, ugly, clever, obscene) source and the project it came from. This started from a belief that reading source is as important (or more so) as writing it. We read computer books to become better programmers, but unless you're reading books like Programming Pearls, you ought to peruse some Open Source projects for inspiration.
如果您是新手,我每周都会发布一些片段,这些片段特别有趣(阅读:美丽,丑陋,聪明,淫秽)和其来源。 这源于一种信念,即阅读源与编写源同样重要(甚至更重要) 。 我们阅读计算机书籍以成为更好的程序员,但是除非您阅读《 Programming Pearls》之类的书,否则您应该细读一些开放源代码项目以获取灵感。
And so, Dear Reader, I present to you the thirteenth in a infinite number of posts of "The Weekly Source Code." Here's some source I was reading this week. I wanted to see what a Fibonacci number generator looked like in a number of different languages.
因此,尊敬的读者,我在“每周源代码”的无数帖子中向您介绍了第十三篇。 这是我本周正在阅读的一些资料。 我想看看用多种不同语言编写的斐波那契数生成器的外观。
Remember (from Wikipedia) that the Fibonacci sequence looks like this:
请记住(来自Wikipedia )斐波那契数列如下所示:
...after two starting values, each number is the sum of the two preceding numbers. The first Fibonacci numbers also denoted as Fn, for n = 0, 1, … , are:
...在两个起始值之后,每个数字是前两个数字的总和。 对于n = 0,1,…的第一个斐波那契数也表示为F n为:
- 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765, 10946, 17711, 28657, 46368, 75025, 121393, ... 0,1,1,2,3,5,8,13,21,34,55,89,144,233,377,610,987,1597,2584,4181,6765,10946,17711,28657,46368, 75025、121393,...
Here's a few implementations I thought contrasted nicely. There's also a great writeup on Fibonacci related things on Dustin Campbell's blog.
我认为这是一些很好的实现。 在达斯汀·坎贝尔(Dustin Campbell)的博客上,也有很多关于斐波那契相关内容的文章。
F# (F#)
Here's a basic Fibonacci function in F#.
这是F#中的基本斐波那契函数。
Here's a basic Fibonacci function in F#.
这是F#中的基本斐波那契函数。
let rec fib n = if n < 2 then 1 else fib (n-2) + fib(n-1)
Or, if you want input and output as an F# console application:
或者,如果要将输入和输出作为F#控制台应用程序:
Or, if you want input and output as an F# console application:
或者,如果要将输入和输出作为F#控制台应用程序:
let fib_number = int_of_string (System.Environment.GetCommandLineArgs().GetValue(1).ToString());; let rec fib n = if n < 2 then 1 else fib (n-2) + fib(n-1);; Printf.printf "\nThe Fibonacci value of %u is: %u\n" fib_number (fib fib_number);; exit 0;;
Ruby (Ruby)
Here it is in Ruby, from RubyTips.org:
这是RubyTips.org中的Ruby:
Here it is in Ruby, from RubyTips.org:
这是RubyTips.org中的Ruby:
x1,x2 = 0,1; 0.upto(size){puts x1; x1 += x2; x1,x2 = x2,x1}
But that's really hard to read and kind of smooshed onto one line. A more typical console app would look like this:
但是,这确实很难阅读,而且有点模糊。 一个更典型的控制台应用程序将如下所示:
But that's really hard to read and kind of smooshed onto one line. A more typical console app would look like this:
但这确实很难阅读,而且有点模糊。 一个更典型的控制台应用程序将如下所示:
class FibonacciGenerator def printFibo(size) x1,x2 = 0, 1 0.upto(size){puts x1;x1+=x2; x1,x2= x2,x1} # note the swap for the next iteration end f = FibonacciGenerator.new f.printFibo(10) # print first 10 fibo numbers end
C#(C#)
There's many ways to do this in C#, so let's start with a basic C# 2.0 implementation.
在C#中有很多方法可以做到这一点,所以让我们从一个基本的C#2.0实现开始。
There's many ways to do this in C#, so let's start with a basic C# 2.0 implementation.
在C#中有很多方法可以做到这一点,所以让我们从一个基本的C#2.0实现开始。
static int Fibonacci (int x) { if (x <= 1) return 1; return Fibonacci (x-1) + Fibonacci (x-2); }
Now, here's a great way using C# 3.0 (actually, .NET 3.5 and System.Func from the new System.Core:
现在,这是使用C#3.0(实际上是来自新System.Core的.NET 3.5和System.Func)的一种好方法:
Now, here's a great way using C# 3.0 (actually, .NET 3.5 and System.Func from the new System.Core:
现在,这是使用C#3.0(实际上是来自新System.Core的.NET 3.5和System.Func)的一种好方法:
Func<INT , int> fib = null; fib = n => n > 1 ? fib(n - 1) + fib(n - 2) : n;
Scala (Scala)
Lots of folks are excited about Scala, and many are calling it "the latest programmer's shiny object." It's interesting not just for its syntax, but it's full interoperability with Java. Here's a recursive Fibonacci in Scala.
许多人都对Scala感到兴奋,许多人称它为“最新程序员的闪亮对象”。 不仅因为其语法有趣,而且与Java完全互操作。 这是Scala中的递归斐波那契。
Lots of folks are excited about Scala, and many are calling it "the latest programmer's shiny object." It's interesting not just for its syntax, but it's full interoperability with Java. Here's a recursive Fibonacci in Scala.
许多人都对Scala感到兴奋,许多人称它为“最新程序员的闪亮对象”。 不仅因为其语法有趣,而且与Java完全互操作。 这是Scala中的递归斐波那契。
def fib( n: Int): Int = n match { case 0 => 0 case 1 => 1 case _ => fib( n -1) + fib( n-2) }
Erlang (Erlang)
Here's Fibonacci in Erlang, and you can find many other implementations at LiteratePrograms.org, a great site for reading source!
这是Erlang的Fibonacci ,您可以在LiteratePrograms.org上找到许多其他实现,这是一个阅读源代码的好网站!
Here's Fibonacci in Erlang, and you can find many other implementations at LiteratePrograms.org, a great site for reading source!
这是位于Erlang的Fibonacci ,您可以在LiteratePrograms.org上找到许多其他实现,这是一个阅读源的绝佳网站!
fibo(0) -> 0 ; fibo(1) -> 1 ; fibo(N) when N > 0 -> fibo(N-1) + fibo(N-2) .
Which is your favorite? I like the C# 3.0 one and the F# ones. Also the Ruby double variable swap is pretty cool. They just feel right, although a close runner-up is the LOLCode implementation of Fibonacci from a few weeks back.
哪个是你最喜欢的? 我喜欢C#3.0和F#。 Ruby的双变量交换也很酷。 他们只是感觉不错,尽管从几周前开始,斐波那契的LOLCode实现获得了亚军。
翻译自: https://www.hanselman.com/blog/the-weekly-source-code-13-fibonacci-edition
知乎周源微信