This year at Xamarin's Evolve there was a Robot Building area that introduced a new beta platform called Monkey.Robotics (GitHub). There is a disclaimer on the GitHub: "Monkey.Robotics is still a beta/work in progress and is not officially supported by Xamarin" but I can say that I was able to successfully build this robot with my 6 year old and we've had a blast driving it around the house! He was thrilled to solder (but not too much) and setup all the wires on the breadboard.
今年,在Xamarin的Evolve中,有一个机器人大厦区域,引入了一个名为Monkey.Robotics ( GitHub )的新beta平台。 GitHub上有一个免责声明:“ Monkey.Robotics仍处于测试阶段/尚在开发中,尚未得到Xamarin的正式支持”,但我可以说我能够在6岁的时候成功地构建了这个机器人,炸毁了房子周围! 他很高兴能焊接(但不要太多)并在面包板上设置所有电线。
This robot is a great showcase for the larger Monkey.Robotics stack PLUS a great way to learn how to use Xamarin tools AND the .NET Micro Framework. And the end you'll have not just a robot with code running on a Netduino, but also have deployed C# code using Xamarin onto (in my case) an iPhone.
该机器人是大型Monkey.Robotics堆栈的绝佳展示,也是学习如何使用Xamarin工具和.NET Micro Framework的绝佳方法。 最后,您不仅将拥有一个在Netduino上运行代码的机器人,而且还将使用Xamarin部署C#代码到iPhone上。
The resulting stuff you get to build and use are:
您可以构建和使用的最终结果是:
iPhone/Android app using Xamarin.Forms and controls or a gyroscope talking over your phone's Bluetooth radio to some code running on an...
Netduino running the open source .NET Micro Framework. This Netduino will receive communications via a Bluetooth radio on a chip. This BT LE board is connected to the GPIO (general purpose input output) pins on the Netduino. The board then controls...
Netduino运行开源.NET Micro Framework。 该Netduino将通过芯片上的蓝牙无线电接收通信。 该BT LE板连接到Netduino的GPIO(通用输入输出)引脚。 然后,董事会控制...
- Motors and wheels on a nice base, powered by 4 AA batteries. 电动机和车轮均由4节AA电池供电,底座良好。
The authors are some of my favorite people, including Bryan Costanich, Frank Krueger, Craig Dunn, David Karlas, and Oleg Rakhmatulin.
作者是我最喜欢的人,包括Bryan Costanich,Frank Krueger,Craig Dunn,David Karlas和Oleg Rakhmatulin。
There's a deceptively large amount of code here and it's whole job is to hide the yucky parts of a project that connects mobile devices to wearables, sensors, peripherals, and low-level communication. They include a cross-platform API for talking with BLE (Bluetooth Low-Energy) devices, as well as the beginnings of a similar API over Wifi. Even more important is a higher level messaging framework sitting on top of these lower=level APIs. The net result is that talking Bluetooth between your phone and a device isn't very hard.
这里似乎有大量的代码,它的全部工作是隐藏将移动设备连接到可穿戴设备,传感器,外围设备和低级通信的项目的棘手部分。 它们包括用于与BLE(蓝牙低功耗)设备进行通信的跨平台API,以及类似的基于Wifi的API的开始。 更为重要的是位于这些较低级别API之上的高层消息传递框架。 最终结果是,在手机和设备之间通话蓝牙并不难。
On the device side (in my case with .NET Micro Framework) they make things more compose-able with "blocks and scopes" style abstractions, allowing one to fairly easily connect output pins (LEDs, motors) to input pins (buttons, sensors).
在设备方面(以.NET Micro Framework为例),它们通过“块和作用域”样式抽象使事情变得更容易组合,从而使人们可以轻松地将输出引脚(LED,电动机)连接到输入引脚(按钮,传感器) )。
Here is one of their basic examples. This makes an LED blink when a button is pressed.
这是他们的基本例子之一。 当按下按钮时,这会使LED闪烁。
public class Program
{
static H.Cpu.Pin buttonHardware = Pins.ONBOARD_BTN;
static H.Cpu.Pin ledHardware = Pins.ONBOARD_LED;
public static void Main()
{
var button = new DigitalInputPin (buttonHardware);
var led = new DigitalOutputPin (ledHardware);
button.Output.ConnectTo (led.Input);
// keep the program alive
while (true) {
System.Threading.Thread.Sleep (1000);
}
}
}
I went through the very excellent Project Walkthrough on building a Robot. Note that all this is on GitHub so if you have any issues, you can fix them and submit a pull request. I'm sure the project would appreciate it!
我经历了关于构建机器人的非常出色的项目演练。 请注意,所有这些都在GitHub上,因此,如果您有任何问题,可以修复它们并提交请求请求。 我确信该项目将不胜感激!
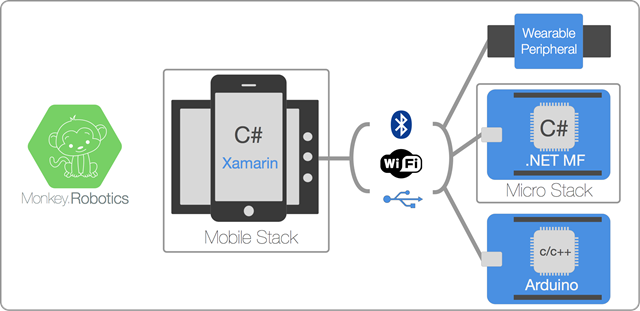
Here's what you need to buy/or have (I took from their GitHub site) if you want to build this same robot.
如果您想构建相同的机器人,这就是您需要购买/拥有的东西(我从他们的GitHub网站获得)。
bot Chassis w/Motors - Just about any 2 wheeled robot chassis with motors and gears will work, but we like these. They're only $11 and they work well. Spark Fun also makes a similar robot chassis for $15, but the battery holder is difficult to access, otherwise, it's also very nice.
带电机的bot底盘-几乎任何带有电动机和齿轮的2轮机器人底盘都可以使用,但是我们喜欢这些。 它们只有11美元,而且运作良好。 Spark Fun还以15美元的价格制造了类似的机器人底盘,但是电池盒很难拿到,否则,也非常好。
Netduino 2 - From Secret Labs, the Netduino is a programmable micro controller that runs the .NET Micro Framework.
Netduino 2-从Secret Labs,Netduino是运行.NET Micro Framework的可编程微控制器。
BLE Mini - From Redbear Labs. Used to communicate with the robot from Xamarin.iOS and Xamarin.Android.
BLE Mini-来自Redbear Labs。 用于与Xamarin.iOS和Xamarin.Android中的机器人进行通信。
400 Point Breadboard - The breadboard is where our electronics will all get connected. It has holes in it to put chips, sensors, wires, etc., into.
400点面包板-面包板是我们所有电子设备都可以连接的地方。 它上面有Kong,可将芯片,传感器,电线等放入其中。
Breadboard Wiring Kit - Any wiring kit will work, but we especially like these. You may also want to get some male-to-male flexible jumper wires.
Dual H-Bridge Chip - Used to power the motors, H-bridges are simple chips that allow you to control motors with external power, so you don't burn out your Netduino trying to drive them with that. :) You can also build an H-bridge with some simple components, but buying a chip is easier and will likely cost about the same.
双H桥芯片-用于为电动机供电的H桥是简单的芯片,允许您使用外部电源控制电动机,因此您不会烧坏Netduino来尝试用其驱动电动机。 :)您也可以使用一些简单的组件构建H桥,但是购买芯片更容易,并且成本大约相同。
iOS or Android Device w/BLE Support - If using an iOS device, you'll need an iPhone 4 or later. If using an Android Device, you'll want to make sure that it has BLE support and is running at least Android v4.3.
带BLE支持的iOS或Android设备-如果使用iOS设备,则需要iPhone 4或更高版本。 如果使用的是Android设备,则需要确保它具有BLE支持并至少运行Android v4.3。
Make sure you have you development and build environment setup, and then follow the guides below to get this thing up and running!
确保您具有开发和构建环境设置,然后按照以下指南进行操作!
Step 1 - Assemble the Robot Chassis
第1步-组装机器人机箱
Step 2 - Wire up the Robot
第2步-连接机器人
Step 3 - Deploy the Robot Code
第3步-部署机器人代码
Step 4 - Drive the Robot!
第4步-驾驶机器人!
Basically you'll need Xamarin Studio or Visual Studio with Xamarin Tools. You'll also need the .NET Micro Framework bits, which are free for Visual Studio 2013, as well as the Netduino 4.3.1 SDK. There's a thread over at the Netduino Forums if you have trouble getting your device ready. I had no issues at all.
基本上,您将需要Xamarin Studio或带有Xamarin Tools的Visual Studio。 您还需要.NET Micro Framework位(对于Visual Studio 2013是免费的)以及Netduino 4.3.1 SDK 。 如果您无法准备好设备,则Netduino论坛上有一个话题。 我一点都没有问题。
The Robot code is in a larger solution called Robotroller that's pretty nicely factored. You've got the Core functionality for the phone and the Core for the Robot.
Robot代码存在于称为Robotroller的较大解决方案中,这是很好的考虑因素。 您已经拥有手机的核心功能和机器人的核心。
The Phone code makes great use of databinding. The Car code is a little messy (it's beta and looks like Tests that turned into a working Robot! ) but it worked out of the box! It's surprisingly simple, in fact, due to the next abstraction layer provided by Monkey.Robotics.
电话代码充分利用了数据绑定。 Car代码有点混乱(它是beta版,看起来像Tests变成了可以正常工作的Robot!),但是开箱即用! 实际上,由于Monkey.Robotics提供的下一个抽象层,它非常简单。
How cool is this. That's basically it. Love it.
这太酷了。 基本上就是这样。 爱它。
public class TestRCCar
{
public static void Run ()
{
// initialize the serial port for COM1 (using D0 & D1)
// initialize the serial port for COM3 (using D7 & D8)
var serialPort = new SerialPort (SerialPorts.COM3, 57600, Parity.None, 8, StopBits.One);
serialPort.Open ();
var server = new ControlServer (serialPort);
// Just some diagnostic stuff
var uptimeVar = server.RegisterVariable ("Uptime (s)", 0);
var lv = false;
var led = new Microsoft.SPOT.Hardware.OutputPort (Pins.ONBOARD_LED, lv);
// Make the robot
var leftMotor = HBridgeMotor.CreateForNetduino (PWMChannels.PWM_PIN_D3, Pins.GPIO_PIN_D1, Pins.GPIO_PIN_D2);
var rightMotor = HBridgeMotor.CreateForNetduino (PWMChannels.PWM_PIN_D6, Pins.GPIO_PIN_D4, Pins.GPIO_PIN_D5);
var robot = new TwoWheeledRobot (leftMotor, rightMotor);
// Expose some variables to control it
robot.SpeedInput.ConnectTo (server, writeable: true, name: "Speed");
robot.DirectionInput.ConnectTo (server, writeable: true, name: "Turn");
leftMotor.SpeedInput.ConnectTo (server);
rightMotor.SpeedInput.ConnectTo (server);
// Show diagnostics
for (var i = 0; true; i++) {
uptimeVar.Value = i;
led.Write (lv);
lv = !lv;
Thread.Sleep (1000);
}
}
}
Here's a video (don't play it too loud, my kids are yelling in the background) of me controlling the robot using my iPhone. Note I'm using the gyroscope control so I twist my hand to steer.
这是一个视频(不要太大声播放,我的孩子在后台大喊大叫),我用我的iPhone控制机器人。 请注意,我使用的是陀螺仪控件,所以我要用手扭转。
Robot via iPhone over BT LE. It's @Xamarin on phone plus @SecretLabs and @Xamarin on robot!
通过BT LE通过iPhone进行机器人操作。 它是手机上的@Xamarin以及机器人上的@SecretLabs和@Xamarin!
A video posted by Scott Hanselman (@shanselman) on
斯科特·汉塞尔曼(@shanselman)在
All in all, I'm enjoying Monkey.Robotics and I hope it takes off with more projects, more ideas, more things to build. If you've got interest or expertise in this area, go star their project and get involved! There's a LOT of stuff going on over there, so explore all the docs and diagrams.
总而言之,我很享受Monkey.Robotics,并希望它能伴随着更多的项目,更多的想法,更多的东西来发展。 如果您对此领域有兴趣或专业知识,请给他们的项目加星标,并参与进来! 那边有很多东西,所以浏览所有的文档和图表。
Sponsor: Big thanks to my friends at Octopus Deploy. They are the deployment secret that everyone is talking about. Using NuGet and powerful conventions, Octopus Deploy makes it easy to automate releases of ASP.NET applications and Windows Services. Say goodbye to remote desktop and start automating today!
赞助商:非常感谢我的朋友在Octopus Deploy。 它们是每个人都在谈论的部署秘密。 使用NuGet和强大的约定,Octopus Deploy可以轻松实现ASP.NET应用程序和Windows Services版本的自动化。 告别远程桌面,立即开始自动化!