AppActiveMatrixDelegate.INSTANCE.addListener(this);
// 启动定时器轮训检测
scheduleDetectProcedure();
}
}
获取可能存在泄漏的Activity信息
private final Application.ActivityLifecycleCallbacks mRemovedActivityMonitor = new ActivityLifeCycleCallbacksAdapter() {
@Override
public void onActivityCreated(Activity activity, Bundle savedInstanceState) {
mCurrentCreatedActivityCount.incrementAndGet();
}
@Override
public void onActivityDestroyed(Activity activity) {
//记录已被destory的Activity
pushDestroyedActivityInfo(activity);
}
};
private void pushDestroyedActivityInfo(Activity activity) {
final String activityName = activity.getClass().getName();
//该Activity确认存在泄漏,且已经上报
if (isPublished(activityName)) {
MatrixLog.d(TAG, “activity leak with name %s had published, just ignore”, activityName);
return;
}
final UUID uuid = UUID.randomUUID();
final StringBuilder keyBuilder = new StringBuilder();
//生成Activity实例的唯一标识
keyBuilder.append(ACTIVITY_REFKEY_PREFIX).append(activityName)
.append(‘_’).append(Long.toHexString(uuid.getMostSignificantBits())).append(Long.toHexString(uuid.getLeastSignificantBits()));
final String key = keyBuilder.toString();
//构造一个数据结构,表示一个已被destroy的Activity
final DestroyedActivityInfo destroyedActivityInfo
= new DestroyedActivityInfo(key, activity, activityName, mCurrentCreatedActivityCount.get());
//放入后续待检测的Activity list
mDestroyedActivityInfos.add(destroyedActivityInfo);
}
存储过后,静静等待轮训分析。
scheduleDetectProcedure 方法会启动轮训来分析:
private void scheduleDetectProcedure() {
mDetectExecutor.executeInBackground(mScanDestroyedActivitiesTask);
}
executeInBackground 会走到如下方法中:
private void postToBackgroundWithDelay(final RetryableTask task, final int failedAttempts) {
mBackgroundHandler.postDelayed(new Runnable() {
@Override
public void run() {
RetryableTask.Status status = task.execute();
if (status == RetryableTask.Status.RETRY) {
postToBackgroundWithDelay(task, failedAttempts + 1);
}
}
}, mDelayMillis);
}
最终会以间隔 mDelayMillis 毫秒进行轮训执行 RetryableTask 任务,当 RetryableTask.Status 为 RETRY 时停止,那么,我们来看看传入进来的 RetryableTask ,也就是 mScanDestroyedActivitiesTask 的 execute 方法 execute 方法的内容比较多,我们只分析 ResourceConfig.DumpMode == AUTO_DUMP
检测是否存在内存泄漏
private final RetryableTask mScanDestroyedActivitiesTask = new RetryableTask() {
@Override
public Status execute() {
// Fake leaks will be generated when debugger is attached.
//Debug调试模式,检测可能失效,直接return
if (Debug.isDebuggerConnected() && !mResourcePlugin.getConfig().getDetectDebugger()) {
MatrixLog.w(TAG, “debugger is connected, to avoid fake result, detection was delayed.”);
return Status.RETRY;
}
//没有已被destory的Activity实例
if (mDestroyedActivityInfos.isEmpty()) {
return Status.RETRY;
}
//创建一个对象的弱引用
final WeakReference sentinelRef = new WeakReference<>(new Object());
//尝试触发GC
triggerGc();
//系统未执行GC,直接return
if (sentinelRef.get() != null) {
// System ignored our gc request, we will retry later.
MatrixLog.d(TAG, “system ignore our gc request, wait for next detection.”);
return Status.RETRY;
}
final Iterator infoIt = mDestroyedActivityInfos.iterator();
while (infoIt.hasNext()) {
final DestroyedActivityInfo destroyedActivityInfo = infoIt.next();
//该实例对应的Activity已被标泄漏,跳过该实例
if (isPublished(destroyedActivityInfo.mActivityName)) {
MatrixLog.v(TAG, “activity with key [%s] was already published.”, destroyedActivityInfo.mActivityName);
infoIt.remove();
continue;
}
//若不能通过弱引用获取到Activity实例,表示已被回收,跳过该实例
if (destroyedActivityInfo.mActivityRef.get() == null) {
// The activity was recycled by a gc triggered outside.
MatrixLog.v(TAG, “activity with key [%s] was already recycled.”, destroyedActivityInfo.mKey);
infoIt.remove();
continue;
}
//该Activity实例 检测到泄漏的次数+1
++destroyedActivityInfo.mDetectedCount;
//当前显示的Activity实例与泄漏的Activity实例相差几个Activity跳转
long createdActivityCountFromDestroy = mCurrentCreatedActivityCount.get() - destroyedActivityInfo.mLastCreatedActivityCount;
//若改Activity实例 检测到泄漏的次数未达到阈值,或者泄漏的Activity与当前显示的Activity很靠近,可认为是一种容错手段(实际应用中有这种场景),跳过该实例
if (destroyedActivityInfo.mDetectedCount < mMaxRedetectTimes
|| (createdActivityCountFromDestroy < CREATED_ACTIVITY_COUNT_THRESHOLD && !mResourcePlugin.getConfig().getDetectDebugger())) {
// Although the sentinel tell us the activity should have been recycled,
// system may still ignore it, so try again until we reach max retry times.
MatrixLog.i(TAG, “activity with key [%s] should be recycled but actually still \n”
- “exists in %s times detection with %s created activities during destroy, wait for next detection to confirm.”,
destroyedActivityInfo.mKey, destroyedActivityInfo.mDetectedCount, createdActivityCountFromDestroy);
continue;
}
MatrixLog.i(TAG, “activity with key [%s] was suspected to be a leaked instance.”, destroyedActivityInfo.mKey);
//若允许dump内存信息
if (mHeapDumper != null) {
final File hprofFile = mHeapDumper.dumpHeap();
if (hprofFile != null) {
markPublished(destroyedActivityInfo.mActivityName);
final HeapDump heapDump = new HeapDump(hprofFile, destroyedActivityInfo.mKey, destroyedActivityInfo.mActivityName);
//处理dump出的内存信息(裁剪)
mHeapDumpHandler.process(heapDump);
infoIt.remove();
} else {
//内存dump失败
MatrixLog.i(TAG, “heap dump for further analyzing activity with key [%s] was failed, just ignore.”,
destroyedActivityInfo.mKey);
infoIt.remove();
}
} else {
// Lightweight mode, just report leaked activity name.
//不允许dump内存的情况下,直接上报泄漏的Activity类名
MatrixLog.i(TAG, “lightweight mode, just report leaked activity name.”);
markPublished(destroyedActivityInfo.mActivityName);
if (mResourcePlugin != null) {
final JSONObject resultJson = new JSONObject();
try {
resultJson.put(SharePluginInfo.ISSUE_ACTIVITY_NAME, destroyedActivityInfo.mActivityName);
} catch (JSONException e) {
MatrixLog.printErrStackTrace(TAG, e, “unexpected exception.”);
}
mResourcePlugin.onDetectIssue(new Issue(resultJson));
}
}
}
return Status.RETRY;
}
};
① 哨兵
这个哨兵是判断 gc 真正触发的条件,为什么要做哨兵呢,正如文档所言:
VM并没有提供强制触发GC的API,通过System.gc()或Runtime.getRuntime().gc()只能“建议”系统进行GC,如果系统忽略了我们的GC请求,可回收的对象就不会被加入ReferenceQueue
② 手动 gc
虽然 Runtime.getRuntime().gc()
无法保证,但还是要手动去触发一下 gc
③ 哨兵是否被回收
如果哨兵被回收了,则说明 gc 被真正触发了,如果未回收,则让轮训接着重试
④ 累计检测次数
这个地方是有一句英文注释的,主要是为了延长检测的时间,哨兵并不能是他的唯一条件 Although the sentinel tell us the activity should have been recycled,system may still ignore it, so try again until we reach max retry times.
⑤ dump 泄漏实例 mHeapDumper 是 AndroidHeapDumper 类,在 ComponentFactory 中有提供,然后来看看 dumpHeap 方法:
public File dumpHeap() {
// 通过存储 Manager 来生成一个 File 文件
final File hprofFile = mDumpStorageManager.newHprofFile();
…
try {
// 将 dump 信息存储到 hprofFile 中
Debug.dumpHprofData(hprofFile.getAbsolutePath());
…
// 返回 dump file
return hprofFile;
} catch (Exception e) {
MatrixLog.printErrStackTrace(TAG, e, “failed to dump heap into file: %s.”, hprofFile.getAbsolutePath());
return null;
}
}
⑥ 压缩、上报 dumpFile
mHeapDumpHandler 是 AndroidHeapDumper.HeapDumpHandler 类,它也是在 ComponentFactory 提供,然后我们来看看 process 方法:
protected AndroidHeapDumper.HeapDumpHandler createHeapDumpHandler(final Context context, ResourceConfig resourceConfig) {
return new AndroidHeapDumper.HeapDumpHandler() {
@Override
public void process(HeapDump result) {
//process流程最终调用CanaryWorkerService进行裁剪和上报
CanaryWorkerService.shrinkHprofAndReport(context, result);
}
};
}
public static void shrinkHprofAndReport(Context context, HeapDump heapDump) {
final Intent intent = new Intent(context, CanaryWorkerService.class);
intent.setAction(ACTION_SHRINK_HPROF);
intent.putExtra(EXTRA_PARAM_HEAPDUMP, heapDump);
enqueueWork(context, CanaryWorkerService.class, JOB_ID, intent);
}
CanaryWorkerService、CanaryResultService都是在独立进程运行的。其中CanaryWorkerService主要执行doShrinkHprofAndReport方法:
private void doShrinkHprofAndReport(HeapDump heapDump) {
final File hprofDir = heapDump.getHprofFile().getParentFile();
//裁剪之后的Hprof文件名
final File shrinkedHProfFile = new File(hprofDir, getShrinkHprofName(heapDump.getHprofFile()));
final File zipResFile = new File(hprofDir, getResultZipName(“dump_result_” + android.os.Process.myPid()));
final File hprofFile = heapDump.getHprofFile();
ZipOutputStream zos = null;
try {
long startTime = System.currentTimeMillis();
//执行Hprof裁剪
new HprofBufferShrinker().shrink(hprofFile, shrinkedHProfFile);
MatrixLog.i(TAG, “shrink hprof file %s, size: %dk to %s, size: %dk, use time:%d”,
hprofFile.getPath(), hprofFile.length() / 1024, shrinkedHProfFile.getPath(), shrinkedHProfFile.length() / 1024, (System.currentTimeMillis() - startTime));
//打成压缩包
zos = new ZipOutputStream(new BufferedOutputStream(new FileOutputStream(zipResFile)));
//记录一些设备信息
final ZipEntry resultInfoEntry = new ZipEntry(“result.info”);
//裁剪后的Hprof文件
final ZipEntry shrinkedHProfEntry = new ZipEntry(shrinkedHProfFile.getName());
zos.putNextEntry(resultInfoEntry);
final PrintWriter pw = new PrintWriter(new OutputStreamWriter(zos, Charset.forName(“UTF-8”)));
pw.println(“# Resource Canary Result Infomation. THIS FILE IS IMPORTANT FOR THE ANALYZER !!”);
//系统版本
pw.println(“sdkVersion=” + Build.VERSION.SDK_INT);
//厂商信息
pw.println(“manufacturer=” + Build.MANUFACTURER);
//裁剪后Hprof文件名
pw.println(“hprofEntry=” + shrinkedHProfEntry.getName());
//泄漏Activity实例的key
pw.println(“leakedActivityKey=” + heapDump.getReferenceKey());
pw.flush();
zos.closeEntry();
zos.putNextEntry(shrinkedHProfEntry);
copyFileToStream(shrinkedHProfFile, zos);
zos.closeEntry();
//原始数据删除
shrinkedHProfFile.delete();
hprofFile.delete();
MatrixLog.i(TAG, “process hprof file use total time:%d”, (System.currentTimeMillis() - startTime));
//CanaryResultService执行上报逻辑
CanaryResultService.reportHprofResult(this, zipResFile.getAbsolutePath(), heapDump.getActivityName());
} catch (IOException e) {
MatrixLog.printErrStackTrace(TAG, e, “”);
} finally {
closeQuietly(zos);
}
}
裁剪的核心代码如下:
public void shrink(File hprofIn, File hprofOut) throws IOException {
FileInputStream is = null;
OutputStream os = null;
try {
is = new FileInputStream(hprofIn);
os = new BufferedOutputStream(new FileOutputStream(hprofOut));
final HprofReader reader = new HprofReader(new BufferedInputStream(is));
//1、收集Bitmap和String信息
reader.accept(new HprofInfoCollectVisitor());
// Reset.
is.getChannel().position(0);
//2、找到Bitmap、String中持有的byte数组,并找到内容重复的Bitmap
reader.accept(new HprofKeptBufferCollectVisitor());
// Reset.
is.getChannel().position(0);
//3、裁剪掉内容重复的Bitmap,和其他byte数组
reader.accept(new HprofBufferShrinkVisitor(new HprofWriter(os)));
} finally {
if (os != null) {
try {
os.close();
} catch (Throwable thr) {
// Ignored.
}
}
if (is != null) {
try {
is.close();
} catch (Throwable thr) {
// Ignored.
}
}
}
}
HprofInfoCollectVisitor
private class HprofInfoCollectVisitor extends HprofVisitor {
HprofInfoCollectVisitor() {
super(null);
}
@Override
public void visitHeader(String text, int idSize, long timestamp) {
mIdSize = idSize;
mNullBufferId = ID.createNullID(idSize);
}
@Override
public void visitStringRecord(ID id, String text, int timestamp, long length) {
if (mBitmapClassNameStringId == null && “android.graphics.Bitmap”.equals(text)) {
//Bitmap类型String字符串的索引
mBitmapClassNameStringId = id;
} else if (mMBufferFieldNameStringId == null && “mBuffer”.equals(text)) {
//mBuffer字段String字符串的索引
mMBufferFieldNameStringId = id;
} else if (mMRecycledFieldNameStringId == null && “mRecycled”.equals(text)) {
//mRecycled字段String字符串的索引
mMRecycledFieldNameStringId = id;
} else if (mStringClassNameStringId == null && “java.lang.String”.equals(text)) {
//String类型 字符串的索引
mStringClassNameStringId = id;
} else if (mValueFieldNameStringId == null && “value”.equals(text)) {
//value字段字符串的索引
mValueFieldNameStringId = id;
}
}
@Override
public void visitLoadClassRecord(int serialNumber, ID classObjectId, int stackTraceSerial, ID classNameStringId, int timestamp, long length) {
if (mBmpClassId == null && mBitmapClassNameStringId != null && mBitmapClassNameStringId.equals(classNameStringId)) {
//找到Bitmap这个类的索引
mBmpClassId = classObjectId;
} else if (mStringClassId == null && mStringClassNameStringId != null && mStringClassNameStringId.equals(classNameStringId)) {
//找到String这个类的索引
mStringClassId = classObjectId;
}
}
@Override
public HprofHeapDumpVisitor visitHeapDumpRecord(int tag, int timestamp, long length) {
return new HprofHeapDumpVisitor(null) {
@Override
public void visitHeapDumpClass(ID id, int stackSerialNumber, ID superClassId, ID classLoaderId, int instanceSize, Field[] staticFields, Field[] instanceFields) {
if (mBmpClassInstanceFields == null && mBmpClassId != null && mBmpClassId.equals(id)) {
/找到Bitmap所有实例的字段信息
mBmpClassInstanceFields = instanceFields;
} else if (mStringClassInstanceFields == null && mStringClassId != null && mStringClassId.equals(id)) {
//找到String所有势力的字段信息
mStringClassInstanceFields = instanceFields;
}
}
};
}
}
这里对Bitmap、String两种类型做了处理(因为后续步骤中要采集掉byte数组)。
Bitmap在android sdk < 26之前,存储像素的byte数组是放在Java层的,26之后是放在native层的。
String在android sdk < 23之前,存储字符的byte数组是放在Java层的,23之后是放在native层的。
HprofKeptBufferCollectVisitor
private class HprofKeptBufferCollectVisitor extends HprofVisitor {
HprofKeptBufferCollectVisitor() {
super(null);
}
@Override
public HprofHeapDumpVisitor visitHeapDumpRecord(int tag, int timestamp, long length) {
return new HprofHeapDumpVisitor(null) {
@Override
public void visitHeapDumpInstance(ID id, int stackId, ID typeId, byte[] instanceData) {
try {
//找到Bitmap实例
if (mBmpClassId != null && mBmpClassId.equals(typeId)) {
ID bufferId = null;
Boolean isRecycled = null;
final ByteArrayInputStream bais = new ByteArrayInputStream(instanceData);
for (Field field : mBmpClassInstanceFields) {
final ID fieldNameStringId = field.nameId;
final Type fieldType = Type.getType(field.typeId);
if (fieldType == null) {
throw new IllegalStateException("visit bmp instance failed, lost type def of typeId: " + field.typeId);
}
if (mMBufferFieldNameStringId.equals(fieldNameStringId)) {
//找到这个实例mBuffer字段的索引id
bufferId = (ID) IOUtil.readValue(bais, fieldType, mIdSize);
} else if (mMRecycledFieldNameStringId.equals(fieldNameStringId)) {
//找到这个实例mRecycled的boolean值(基础数据类型,没有引用关系)
isRecycled = (Boolean) IOUtil.readValue(bais, fieldType, mIdSize);
} else if (bufferId == null || isRecycled == null) {
IOUtil.skipValue(bais, fieldType, mIdSize);
} else {
break;
}
}
bais.close();
//确认Bitmap没有被回收
final boolean reguardAsNotRecycledBmp = (isRecycled == null || !isRecycled);
if (bufferId != null && reguardAsNotRecycledBmp && !bufferId.equals(mNullBufferId)) {
//将mBuffer对应的byte数组索引id加入集合
mBmpBufferIds.add(bufferId);
}
//如果是String类型
} else if (mStringClassId != null && mStringClassId.equals(typeId)) {
ID strValueId = null;
final ByteArrayInputStream bais = new ByteArrayInputStream(instanceData);
for (Field field : mStringClassInstanceFields) {
final ID fieldNameStringId = field.nameId;
final Type fieldType = Type.getType(field.typeId);
if (fieldType == null) {
throw new IllegalStateException("visit string instance failed, lost type def of typeId: " + field.typeId);
}
if (mValueFieldNameStringId.equals(fieldNameStringId)) {
//找到这个String实例的value字段对应的byte数组的索引id
strValueId = (ID) IOUtil.readValue(bais, fieldType, mIdSize);
} else if (strValueId == null) {
IOUtil.skipValue(bais, fieldType, mIdSize);
} else {
break;
}
}
bais.close();
if (strValueId != null && !strValueId.equals(mNullBufferId)) {
//将value字段对应的byte数组索引id加入集合
mStringValueIds.add(strValueId);
}
}
} catch (Throwable thr) {
throw new RuntimeException(thr);
}
}
@Override
public void visitHeapDumpPrimitiveArray(int tag, ID id, int stackId, int numElements, int typeId, byte[] elements) {
//将所有byte数组的索引id,以及对应byte[]数据加入集合
mBufferIdToElementDataMap.put(id, elements);
}
};
}
@Override
public void visitEnd() {
final Set<Map.Entry<ID, byte[]>> idDataSet = mBufferIdToElementDataMap.entrySet();
final Map<String, ID> duplicateBufferFilterMap = new HashMap<>();
for (Map.Entry<ID, byte[]> idDataPair : idDataSet) {
final ID bufferId = idDataPair.getKey();
final byte[] elementData = idDataPair.getValue();
//如果这块byte数组不属于Bitmap,continue
if (!mBmpBufferIds.contains(bufferId)) {
// Discard non-bitmap buffer.
continue;
}
计算byte[]数据的md5
final String buffMd5 = DigestUtil.getMD5String(elementData);
final ID mergedBufferId = duplicateBufferFilterMap.get(buffMd5);
//若内存中Bitmap不存在重复的byte[]数据
if (mergedBufferId == null) {
duplicateBufferFilterMap.put(buffMd5, bufferId);
} else {
//若Bitmap存在重复的byte[]数据,所有引用都指向同一块byte数组的索引(方便后续裁剪掉重复的byte[]数据)
mBmpBufferIdToDeduplicatedIdMap.put(mergedBufferId, mergedBufferId);
mBmpBufferIdToDeduplicatedIdMap.put(bufferId, mergedBufferId);
}
}
// Save memory cost.
mBufferIdToElementDataMap.clear();
}
}
HprofBufferShrinkVisitor
private class HprofBufferShrinkVisitor extends HprofVisitor {
HprofBufferShrinkVisitor(HprofWriter hprofWriter) {
super(hprofWriter);
}
@Override
public HprofHeapDumpVisitor visitHeapDumpRecord(int tag, int timestamp, long length) {
return new HprofHeapDumpVisitor(super.visitHeapDumpRecord(tag, timestamp, length)) {
@Override
public void visitHeapDumpInstance(ID id, int stackId, ID typeId, byte[] instanceData) {
try {
//如果是Bitmap类型
if (typeId.equals(mBmpClassId)) {
ID bufferId = null;
int bufferIdPos = 0;
final ByteArrayInputStream bais = new ByteArrayInputStream(instanceData);
for (Field field : mBmpClassInstanceFields) {
final ID fieldNameStringId = field.nameId;
final Type fieldType = Type.getType(field.typeId);
if (fieldType == null) {
throw new IllegalStateException("visit instance failed, lost type def of typeId: " + field.typeId);
}
if (mMBufferFieldNameStringId.equals(fieldNameStringId)) {
bufferId = (ID) IOUtil.readValue(bais, fieldType, mIdSize);
break;
} else {
bufferIdPos += IOUtil.skipValue(bais, fieldType, mIdSize);
}
}
//如果该实例的mBuffer字段的索引不为null
if (bufferId != null) {
//获取去重后的byte数组索引(若有内容重复的byte[]数据,最后都会指向一个id索引)
final ID deduplicatedId = mBmpBufferIdToDeduplicatedIdMap.get(bufferId);
if (deduplicatedId != null && !bufferId.equals(deduplicatedId) && !bufferId.equals(mNullBufferId)) {
//更新byte数组的索引id
modifyIdInBuffer(instanceData, bufferIdPos, deduplicatedId);
}
}
}
} catch (Throwable thr) {
throw new RuntimeException(thr);
}
super.visitHeapDumpInstance(id, stackId, typeId, instanceData);
}
private void modifyIdInBuffer(byte[] buf, int off, ID newId) {
final ByteBuffer bBuf = ByteBuffer.wrap(buf);
bBuf.position(off);
bBuf.put(newId.getBytes());
}
@Override
public void visitHeapDumpPrimitiveArray(int tag, ID id, int stackId, int numElements, int typeId, byte[] elements) {
//重复的byte数组索引 重定向之后的 索引id
final ID deduplicatedID = mBmpBufferIdToDeduplicatedIdMap.get(id);
// Discard non-bitmap or duplicated bitmap buffer but keep reference key.
if (deduplicatedID == null || !id.equals(deduplicatedID)) {
//不记录重复的byte[]数据,直接return
if (!mStringValueIds.contains(id)) {
return;
}
}
super.visitHeapDumpPrimitiveArray(tag, id, stackId, numElements, typeId, elements);
}
};
}
}
Hprof文件裁剪的过程主要是裁剪了重复Bitmap的byte[]数据,裁剪的力度不是很大。(是不是可以只保留引用链,丢弃所有的PrimitiveArray?这里保留Bitmap的原因是回传之后,可以还原出png图片信息;感觉Bitmap用处不是很多,还狠很多裁剪的空间)。
最后是裁剪后的Hprof文件的上报,在CanaryResultService这个Service中
@Override
protected void onHandleWork(Intent intent) {
if (intent != null) {
final String action = intent.getAction();
if (ACTION_REPORT_HPROF_RESULT.equals(action)) {
final String resultPath = intent.getStringExtra(EXTRA_PARAM_RESULT_PATH);
final String activityName = intent.getStringExtra(EXTRA_PARAM_ACTIVITY);
if (resultPath != null && !resultPath.isEmpty()
&& activityName != null && !activityName.isEmpty()) {
doReportHprofResult(resultPath, activityName);
} else {
MatrixLog.e(TAG, “resultPath or activityName is null or empty, skip reporting.”);
}
}
}
}
private void doReportHprofResult(String resultPath, String activityName) {
try {
final JSONObject resultJson = new JSONObject();
// resultJson = DeviceUtil.getDeviceInfo(resultJson, getApplication());
resultJson.put(SharePluginInfo.ISSUE_RESULT_PATH, resultPath);
resultJson.put(SharePluginInfo.ISSUE_ACTIVITY_NAME, activityName);
Plugin plugin = Matrix.with().getPluginByClass(ResourcePlugin.class);
if (plugin != null) {
plugin.onDetectIssue(new Issue(resultJson));
}
} catch (Throwable thr) {
MatrixLog.printErrStackTrace(TAG, thr, “unexpected exception, skip reporting.”);
}
}
服务端分析裁剪后的Hprof文件
Java内存回收的原理是判断该对象是否有到GCRoot的引用链。此处分析Hprof的原则,也是获取泄漏的Activity到GCRoot的引用链。
首先,明确一下哪些对象属于GCRoot;
在Resource Canary的代码中,通过以下这些GCRoot类型来查找引用链
private void enqueueGcRoots(Snapshot snapshot) {
for (RootObj rootObj : snapshot.getGCRoots()) {
switch (rootObj.getRootType()) {
//Java栈帧中的局部变量
case JAVA_LOCAL:
Instance thread = HahaSpy.allocatingThread(rootObj);
String threadName = threadName(thread);
Exclusion params = excludedRefs.threadNames.get(threadName);
if (params == null || !params.alwaysExclude) {
enqueue(params, null, rootObj, null, null);
}
break;
case INTERNED_STRING:
case DEBUGGER:
case INVALID_TYPE:
// An object that is unreachable from any other root, but not a root itself.
case UNREACHABLE:
case UNKNOWN:
// An object that is in a queue, waiting for a finalizer to run.
case FINALIZING:
break;
//系统确认的一些GCRoot
case SYSTEM_CLASS:
//JNI的局部变量
case VM_INTERNAL:
// A local variable in native code.
//JNI的全局变量
case NATIVE_LOCAL:
// A global variable in native code.
//active线程持有的
case NATIVE_STATIC:
// An object that was referenced from an active thread block.
//用于同步锁的监控对象
case THREAD_BLOCK:
// Everything that called the wait() or notify() methods, or that is synchronized.
case BUSY_MONITOR:
case NATIVE_MONITOR:
case REFERENCE_CLEANUP:
// Input or output parameters in native code.
case NATIVE_STACK:
//Java类的静态变量
case JAVA_STATIC:
enqueue(null, null, rootObj, null, null);
break;
default:
throw new UnsupportedOperationException(“Unknown root type:” + rootObj.getRootType());
}
}
}
下面来看下分析的入口方法
private static void analyzeAndStoreResult(File hprofFile, int sdkVersion, String manufacturer,
String leakedActivityKey, JSONObject extraInfo) throws IOException {
final HeapSnapshot heapSnapshot = new HeapSnapshot(hprofFile);
//系统问题可能导致的一些泄漏,可以认为排除掉
final ExcludedRefs excludedRefs = AndroidExcludedRefs.createAppDefaults(sdkVersion, manufacturer).build();
//获取到Activity泄漏的结果
final ActivityLeakResult activityLeakResult
= new ActivityLeakAnalyzer(leakedActivityKey, excludedRefs).analyze(heapSnapshot);
DuplicatedBitmapResult duplicatedBmpResult = DuplicatedBitmapResult.noDuplicatedBitmap(0);
//Android sdk 26以下获取重复Bitmap的结果
if (sdkVersion < 26) {
final ExcludedBmps excludedBmps = AndroidExcludedBmpRefs.createDefaults().build();
duplicatedBmpResult = new DuplicatedBitmapAnalyzer(mMinBmpLeakSize, excludedBmps).analyze(heapSnapshot);
} else {
System.err.println("\n ! SDK version of target device is larger or equal to 26, "
- “which is not supported by DuplicatedBitmapAnalyzer.”);
}
…
}
ActivityLeakAnalyzer这个类就是分析从GCRoot到泄漏Activity实例的引用链。
private ActivityLeakResult checkForLeak(HeapSnapshot heapSnapshot, String refKey) {
long analysisStartNanoTime = System.nanoTime();
try {
final Snapshot snapshot = heapSnapshot.getSnapshot();
//找到泄漏的Activity实例
final Instance leakingRef = findLeakingReference(refKey, snapshot);
// False alarm, weak reference was cleared in between key check and heap dump.
//若找不到,说明已被回收
if (leakingRef == null) {
return ActivityLeakResult.noLeak(AnalyzeUtil.since(analysisStartNanoTime));
}
//寻找GCRoot到泄漏Activity的引用链
return findLeakTrace(analysisStartNanoTime, snapshot, leakingRef);
} catch (Throwable e) {
e.printStackTrace();
return ActivityLeakResult.failure(e, AnalyzeUtil.since(analysisStartNanoTime));
}
}
寻找泄漏Activity实例,是通过检测Activity泄漏时使用到的DestroyedActivityInfo类来判断的。
public class DestroyedActivityInfo {
//通过判断内存dump文件Hprof中实例的key与传入的key是否一致,判断是泄漏的Activity实例
public final String mKey;
public final String mActivityName;
//通过弱引用获取到这个实例
public final WeakReference mActivityRef;
public final long mLastCreatedActivityCount;
public int mDetectedCount = 0;
public DestroyedActivityInfo(String key, Activity activity, String activityName, long lastCreatedActivityCount) {
mKey = key;
mActivityName = activityName;
mActivityRef = new WeakReference<>(activity);
mLastCreatedActivityCount = lastCreatedActivityCount;
}
}
private Instance findLeakingReference(String key, Snapshot snapshot) {
// private static final String DESTROYED_ACTIVITY_INFO_CLASSNAME= “com.tencent.matrix.resource.analyzer.model.DestroyedActivityInfo”;
final ClassObj infoClass = snapshot.findClass(DESTROYED_ACTIVITY_INFO_CLASSNAME);
if (infoClass == null) {
throw new IllegalStateException("Unabled to find destroy activity info class with name: "
- DESTROYED_ACTIVITY_INFO_CLASSNAME);
}
List keysFound = new ArrayList<>();
//遍历DestroyedActivityInfo的所有实例
for (Instance infoInstance : infoClass.getInstancesList()) {
final List<ClassInstance.FieldValue> values = classInstanceValues(infoInstance);
// private static final String ACTIVITY_REFERENCE_KEY_FIELDNAME = “mKey”;
final String keyCandidate = asString(fieldValue(values, ACTIVITY_REFERENCE_KEY_FIELDNAME));
if (keyCandidate.equals(key)) {
// private static final String ACTIVITY_REFERENCE_FIELDNAME = “mActivityRef”;
final Instance weakRefObj = fieldValue(values, ACTIVITY_REFERENCE_FIELDNAME);
if (weakRefObj == null) {
continue;
}
final List<ClassInstance.FieldValue> activityRefs = classInstanceValues(weakRefObj);
//获取弱引用中的真正对象实例
return fieldValue(activityRefs, “referent”);
}
keysFound.add(keyCandidate);
}
throw new IllegalStateException(
"Could not find weak reference with key " + key + " in " + keysFound);
}
获取到泄漏的Activity实例之后,就需要找到GCToot到该实例的引用链。
private ActivityLeakResult findLeakTrace(long analysisStartNanoTime, Snapshot snapshot,
Instance leakingRef) {
//路径搜索帮助类,可以设置一些不用考虑的规则(不用搜索相关分叉)
ShortestPathFinder pathFinder = new ShortestPathFinder(mExcludedRefs);
//找到最短引用链,并返回结果
ShortestPathFinder.Result result = pathFinder.findPath(snapshot, leakingRef);
// False alarm, no strong reference path to GC Roots.
//无引用链
if (result.referenceChainHead == null) {
return ActivityLeakResult.noLeak(AnalyzeUtil.since(analysisStartNanoTime));
}
final ReferenceChain referenceChain = result.buildReferenceChain();
final String className = leakingRef.getClassObj().getClassName();
//若是命中exclude规则,返回无引用链
if (result.excludingKnown || referenceChain.isEmpty()) {
return ActivityLeakResult.noLeak(AnalyzeUtil.since(analysisStartNanoTime));
} else {
//返回Activity泄漏结果
return ActivityLeakResult.leakDetected(false, className, referenceChain,
AnalyzeUtil.since(analysisStartNanoTime));
}
}
findPath是发现引用链的核心方法
public Result findPath(Snapshot snapshot, Instance targetReference) {
final List targetRefList = new ArrayList<>();
targetRefList.add(targetReference);
final Map<Instance, Result> results = findPath(snapshot, targetRefList);
if (results == null || results.isEmpty()) {
return new Result(null, false);
} else {
return results.get(targetReference);
}
}
public Map<Instance, Result> findPath(Snapshot snapshot, Collection targetReferences) {
final Map<Instance, Result> results = new HashMap<>();
if (targetReferences.isEmpty()) {
return results;
}
clearState();
//找到GCRoot对象,并放入队列中
enqueueGcRoots(snapshot);
//是否忽略String对象
canIgnoreStrings = true;
for (Instance targetReference : targetReferences) {
if (isString(targetReference)) {
canIgnoreStrings = false;
break;
}
}
final Set targetRefSet = new HashSet<>(targetReferences);
while (!toVisitQueue.isEmpty() || !toVisitIfNoPathQueue.isEmpty()) {
ReferenceNode node;
if (!toVisitQueue.isEmpty()) {
node = toVisitQueue.poll();
} else {
node = toVisitIfNoPathQueue.poll();
if (node.exclusion == null) {
throw new IllegalStateException("Expected node to have an exclusion " + node);
}
}
// Termination
//找到完整引用链 GCRoot -> targetRef
if (targetRefSet.contains(node.instance)) {
results.put(node.instance, new Result(node, node.exclusion != null));
targetRefSet.remove(node.instance);
if (targetRefSet.isEmpty()) {
break;
}
}
//当前节点是否已经查看过
if (checkSeen(node)) {
continue;
}
if (node.instance instanceof RootObj) {
//如果是GCRoot,按照GCRoot的规则查找子节点
visitRootObj(node);
} else if (node.instance instanceof ClassObj) {
//如果是Class,按照Class的规则查找子节点
visitClassObj(node);
} else if (node.instance instanceof ClassInstance) {
//如果是实例,按照实例的规则查找子节点
visitClassInstance(node);
} else if (node.instance instanceof ArrayInstance) {
//如果是数组,按照数组的规则查找子节点
visitArrayInstance(node);
} else {
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
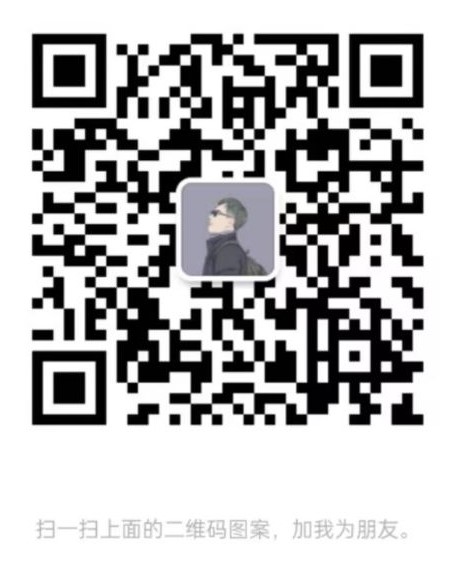
关于面试的充分准备
一些基础知识和理论肯定是要背的,要理解的背,用自己的语言总结一下背下来。
虽然 Android 没有前几年火热了,已经过去了会四大组件就能找到高薪职位的时代了。这只能说明 Android 中级以下的岗位饱和了,现在高级工程师还是比较缺少的,我能明显感觉到国庆后多了很多高级职位,所以努力让自己成为高级工程师才是最重要的。
好了,希望对大家有所帮助。
接下来是整理的一些Android学习资料,有兴趣的朋友们可以关注下我免费领取方式。
①Android开发核心知识点笔记
②对标“阿里 P7” 40W+年薪企业资深架构师成长学习路线图
③面试精品集锦汇总
④全套体系化高级架构视频
**Android精讲视频领取学习后更加是如虎添翼!**进军BATJ大厂等(备战)!现在都说互联网寒冬,其实无非就是你上错了车,且穿的少(技能),要是你上对车,自身技术能力够强,公司换掉的代价大,怎么可能会被裁掉,都是淘汰末端的业务Curd而已!现如今市场上初级程序员泛滥,这套教程针对Android开发工程师1-6年的人员、正处于瓶颈期,想要年后突破自己涨薪的,进阶Android中高级、架构师对你更是如鱼得水!
《Android学习笔记总结+移动架构视频+大厂面试真题+项目实战源码》,点击传送门即可获取!
只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!**
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
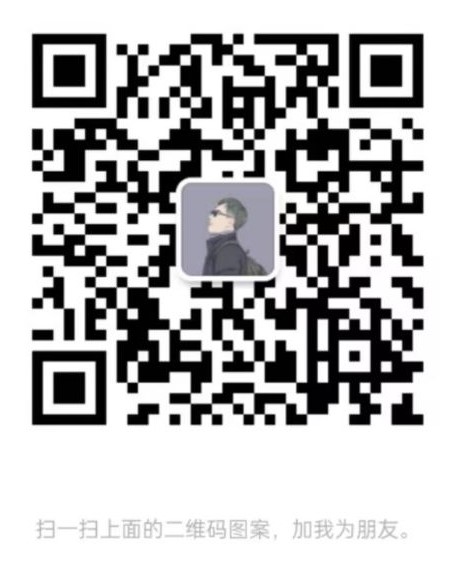
关于面试的充分准备
一些基础知识和理论肯定是要背的,要理解的背,用自己的语言总结一下背下来。
虽然 Android 没有前几年火热了,已经过去了会四大组件就能找到高薪职位的时代了。这只能说明 Android 中级以下的岗位饱和了,现在高级工程师还是比较缺少的,我能明显感觉到国庆后多了很多高级职位,所以努力让自己成为高级工程师才是最重要的。
好了,希望对大家有所帮助。
接下来是整理的一些Android学习资料,有兴趣的朋友们可以关注下我免费领取方式。
①Android开发核心知识点笔记
②对标“阿里 P7” 40W+年薪企业资深架构师成长学习路线图
[外链图片转存中…(img-xEB1urxZ-1712412235833)]
③面试精品集锦汇总
[外链图片转存中…(img-ELG71xnv-1712412235834)]
④全套体系化高级架构视频
**Android精讲视频领取学习后更加是如虎添翼!**进军BATJ大厂等(备战)!现在都说互联网寒冬,其实无非就是你上错了车,且穿的少(技能),要是你上对车,自身技术能力够强,公司换掉的代价大,怎么可能会被裁掉,都是淘汰末端的业务Curd而已!现如今市场上初级程序员泛滥,这套教程针对Android开发工程师1-6年的人员、正处于瓶颈期,想要年后突破自己涨薪的,进阶Android中高级、架构师对你更是如鱼得水!
[外链图片转存中…(img-faMiXmwR-1712412235834)]