一. c++ 内存分区机制
请阅读这篇文章: C++内存分区
1. 栈区数据由编译器自动分配释放
栈区存放数据:
- 局部变量
- 形参(其实就是局部变量)
注意事项:不要返回局部变量/形参的地址,栈区开辟的数据由编译器自动释放
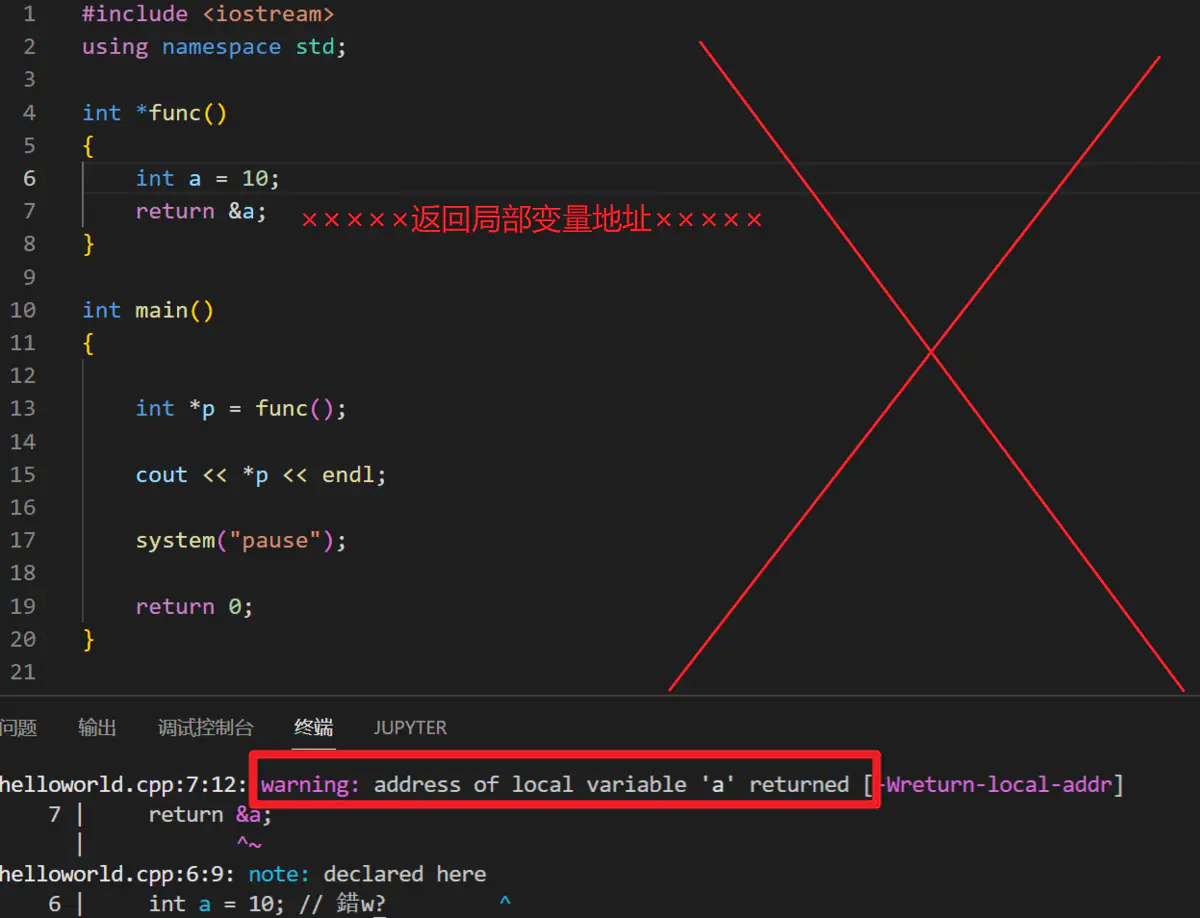
2. 堆区数据由程序员分配释放
由程序员分配释放,若程序员不释放,程序结束时由操作系统回收
在C++中主要利用new
在堆区开辟内存, 利用delete
释放内存
3. new 和 delete
-
new
: 开辟内存会返回 指向内存的指针 -
delete
: 释放内存
#include <iostream>
using namespace std;
int *func1()
{
//利用new在堆区创建数据
int *a = new int(10);
cout << "a address: " << a << endl;
return a;
}
int main()
{
int *p = func1();
cout << "p address: " << p << endl;
cout << *p << endl;
//利用delete释放堆区数据
delete p;
}
new一个数组
#include <iostream>
using namespace std;
int *func1()
{
//利用new在堆区创建数据
int *arr = new int[10];
cout << "arr address: " << arr << endl;
for (int i = 0; i < 10; i++)
{
arr[i] = i + 100;
}
return arr;
}
int main()
{
int *p_arr = func1();
cout << "p_arr address: " << p_arr << endl;
cout << *p_arr << endl;
for (int i = 0; i < 10; i++)
{
cout << p_arr[i] << endl;
}
//利用delete释放堆区数据
delete p_arr;
}
在C++11中,还可以提供一个元素初始化器的花括号列表,
int* p3 = new int[5]{0, 1, 2, 3, 4};
二. 引用
C++推荐用引用技术,因为语法方便,引用本质是指针常量,但是所有的指针操作编译器都帮我们做了
1. 引用
作用: 给变量起别名
语法: 数据类型 &别名 = 原名
可以理解为: 新建一个变量b, 让它的地址 == a的地址, 那其实b和a是同一个变量了
#include <iostream>
using namespace std;
int main()
{
int a = 10;
int &b = a;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "&a = " << &a << endl;
cout << "&b = " << &b << endl;
b = 100;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
return 0;
}
2. 引用的注意事项
- 引用不能只创建不初始化, 不可以:
, 要:int &b;
int &b = a;
- 引用一旦建立, 是不能修改的
3. 引用做函数参数
作用:函数传参时,可以利用引用的技术让形参修饰实参
优点:可以简化指针修改实参
#include <iostream>
using namespace std;
void mySwap(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int a = 10;
int b = 20;
mySwap(a, b);
cout << "a:" << a << " b:" << b << endl;
return 0;
}
// 可以理解为 形参a的地址绑定到了 实参a上, 简称 : 形参a对实参a的引用
有了引用, 我们就可以不穿指针啦! 直接用形参修饰实参,就相当于在操作实参。
通过引用参数产生的效果同按地址传递是一样的。引用的语法更清楚简单
4. 引用做函数返回值
作用:引用是可以作为函数的返回值存在的,返回引用就是返回某个变量
注意:不要返回局部变量引用
//返回:静态变量a的引用
int& test02() {
static int a = 20;
return a;
}
int main() {
int& ref2 = test02(); //相对于ref2引用了a
cout << "ref2 = " << ref2 << endl;
cout << "ref2 = " << ref2 << endl;
return 0;
}
返回值可以作为左值
#include <iostream>
using namespace std;
//返回静态变量引用
int& test02() {
static int a = 20;
return a;
}
int main() {
//如果函数做左值,那么必须返回引用
test02() = 20; //因为test02返回的是a的引用, 所以本句就相当于在修改a
int& ref = test02();
cout << "test02() = " << test02() << endl;
cout << "ref2 = " << ref << endl;
return 0;
}
5. 常量引用
常量引用可以让实参变为只读
#include <iostream>
using namespace std;
//返回静态变量引用
int test02(const int &a)
{
// a = 20; //error: assignment of read-only reference 'a'
cout << a << endl;
return a;
}
int main()
{
int a =10;
cout << "test02() = " << test02(a) << endl;
return 0;
}
三. 函数的重载
1. 函数重载
作用:函数名可以相同,提高复用性
函数重载满足条件:
- 同一个作用域下
- 函数名称相同
- 函数参数类型不同 或者 个数不同 或者 顺序不同
注意: 函数的返回值不可以作为函数重载的条件
//函数重载需要函数都在同一个作用域下
void func()
{
cout << "func 的调用!" << endl;
}
void func(int a)
{
cout << "func (int a) 的调用!" << endl;
}
void func(double a)
{
cout << "func (double a)的调用!" << endl;
}
void func(int a ,double b)
{
cout << "func (int a ,double b) 的调用!" << endl;
}
void func(double a ,int b)
{
cout << "func (double a ,int b)的调用!" << endl;
}
//函数返回值不可以作为函数重载条件
//int func(double a, int b)
//{
// cout << "func (double a ,int b)的调用!" << endl;
//}
int main() {
func();
func(10);
func(3.14);
func(10,3.14);
func(3.14 , 10);
system("pause");
return 0;
}
2. 不用方式的引用也可以作为重载
- 不用方式的引用也可以作为重载
//1、引用作为重载条件
void func(int &a)
{
cout << "func (int &a) 调用 " << endl;
}
void func(const int &a)
{
cout << "func (const int &a) 调用 " << endl;
}
3. 当函数重载遇到默认值参数时, 会产生歧义并报错, 应该避免
四. 文件操作
C++中对文件操作需要包含头文件 <fstream>
1. 两种文件类型
- 文本文件 - 文件以文本的ASCII码形式存储在计算机中
- 二进制文件 - 文件以文本的二进制形式存储在计算机中,用户一般不能直接读懂它们
2. 操作文件的三大类
- ofstream:写操作
- ifstream: 读操作
- fstream : 读写操作
3. 文件打开方式:
打开方式 | 解释 |
---|---|
ios::in | 为读文件而打开文件 |
ios::out | 为写文件而打开文件 |
ios::ate | 初始位置:文件尾 |
ios::app | 追加方式写文件 |
ios::trunc | 如果文件存在先删除,再创建 |
ios::binary | 二进制方式 |
注意: 文件打开方式可以配合使用,利用|操作符
例如:用二进制方式写文件 ios::binary | ios:: out
4. 文本文件写操作
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ofstream ofs;
ofs.open("test.txt", ios::out);
ofs << "姓名:张三" << endl;
ofs << "性别:男" << endl;
ofs << "年龄:18" << endl;
ofs.close();
return 0;
}
5. 文本文件读操作
(1). 方法一 : 用右移运算符读取
第一种方式 : 用右移运算符, 把ifs里面的数据移动到 buf中, 当ifs读完时, 会返回false, 打破while
#include <iostream>
#include <fstream>
using namespace std;
void test01()
{
ifstream ifs;
ifs.open("test.txt", ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败" << endl;
return;
}
// 第一种方式 : 用右移运算符, 把ifs里面的数据移动到 buf中
// 当ifs读完时, 会返回false, 打破while
char buf[1024] = { 0 };
while (ifs >> buf)
{
cout << buf << endl;
}
ifs.close();
}
int main()
{
test01();
return 0;
}
(2). 方法二 : 用ifs的getline方法一行行读取至buf中
用getline把ifs里面的数据读取到 buf中, 当ifs读完时, getline会返回false, 打破while
#include <iostream>
#include <fstream>
using namespace std;
void test01()
{
ifstream ifs;
ifs.open("test.txt", ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败" << endl;
return;
}
// 第二种方式 : 用ifs.getline把ifs里面的数据读取到 buf中
// 当ifs读完时, getline会返回false, 打破while
char buf[1024] = {0};
while (ifs.getline(buf, sizeof(buf)))
{
cout << buf << endl;
}
ifs.close();
}
int main()
{
test01();
return 0;
}
(3). 方法三 : 用全局getline函数读取数据, 用C++ 字符串接收
此getline不是ifs.getline
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
void test01()
{
ifstream ifs;
ifs.open("test.txt", ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败" << endl;
return;
}
// 第三种方式 : 用getline把ifs里面的数据读取到字符串buf中
// 当ifs读完时, getline会返回false, 打破while
string buf;
while (getline(ifs, buf))
{
cout << buf << endl;
}
ifs.close();
}
int main()
{
test01();
return 0;
}
6. 二进制文件写操作
以二进制的方式对文件进行读写操作, 打开方式要指定为 ==ios::binary==
对文件进行操作时, 尽量使用C语言的char型数组, 而非string
二进制方式写文件主要利用流对象调用成员函数write
函数原型 :ostream& write(const char * buffer,int len);
参数解释:字符指针buffer指向内存中一段存储空间。len是读写的字节数
#include <fstream>
#include <string>
class Person
{
public:
char m_Name[64];
int m_Age;
};
//二进制文件 写文件
void test01()
{
//1、包含头文件
//2、创建输出流对象
ofstream ofs("person.txt", ios::out | ios::binary);
//3、打开文件
//ofs.open("person.txt", ios::out | ios::binary);
Person p = {"张三" , 18};
//4、写文件
ofs.write((const char *)&p, sizeof(p));
//5、关闭文件
ofs.close();
}
int main() {
test01();
return 0;
}
7. 二进制文件读操作
二进制方式读文件主要利用流对象调用成员函数read
函数原型:istream& read(char *buffer,int len);
参数解释:字符指针buffer指向内存中一段存储空间。len是读写的字节数
#include <fstream>
#include <string>
class Person
{
public:
char m_Name[64];
int m_Age;
};
void test01()
{
ifstream ifs("person.txt", ios::in | ios::binary);
if (!ifs.is_open())
{
cout << "文件打开失败" << endl;
}
Person p;
ifs.read((char *)&p, sizeof(p));
cout << "姓名: " << p.m_Name << " 年龄: " << p.m_Age << endl;
}
int main() {
test01();
return 0;
}