#include "msp430x14x.h"
#include "iic.h"
#define CPU_F ((double)1000000)
#define delay_ms(x) __delay_cycles((long)(CPU_F*(double)x/1000.0))
#define delay_us(x) __delay_cycles((long)(CPU_F*(double)x/1000000.0))
unsigned int TA_OverflowCnt;
unsigned long int ResponseTime;
void display()
{
OLED_ShowCHinese(0,0,0);
OLED_ShowCHinese( 16,0,1);
OLED_ShowCHinese(32,0,2);
OLED_ShowCHinese(48,0,3);
OLED_ShowChar(64, 0,':',16);
OLED_ShowNum(0,6,ResponseTime,5,16);
OLED_ShowString(0, 2, "TAR:",16);
OLED_ShowNum(32,2,TAR,6,16);
OLED_ShowNum(0,4,TA_OverflowCnt,2,16);
}
void main( void )
{
WDTCTL = WDTPW + WDTHOLD;
Initial_LY096BG30();
OLED_Clear();
_EINT();
P2DIR |= BIT3 + BIT7;
_EINT();
while(1)
{
TA_OverflowCnt = 0;
P2OUT &= ~BIT3;
TACTL = TASSEL_1 + MC_2 + TAIE +TACLR;
while(P1IN & BIT0);
TACTL = TASSEL_1 + MC_0;
P2OUT |= BIT3;
ResponseTime = TA_OverflowCnt*65536 + TAR;
OLED_ShowNum(82,0,ResponseTime,5,16);
ResponseTime = (TA_OverflowCnt*1000000)/32768;
display();
__delay_cycles(3000000);
}
}
#pragma vector = TIMERA1_VECTOR
__interrupt void TA_ISR(void)
{
switch(TAIV)
{
case 2: break;
case 4: break;
case 10:
{
P2OUT ^= BIT7;
TA_OverflowCnt++;
}
break;
}
}
#ifdef iic_c
#define iic_ext
#else
#define iic_ext extern
#endif
#define uchar unsigned char
#define uint unsigned int
#define SET_SCL P2OUT|=BIT0;
#define CLR_SCL P2OUT&=~BIT0;
#define SET_SDA P2OUT|=BIT1;
#define CLR_SDA P2OUT&=~BIT1;
#define SDA_IN P2DIR&=~BIT1;
#define SDA_OUT P2DIR|=BIT1;
#define SCL_OUT P2DIR|=BIT0;
#define SDA_Read P2IN&BIT1
#define OLED_CMD 0
#define OLED_DATA 1
iic_ext void delay();
iic_ext void Initial_LY096BG30();
iic_ext void Delay_1ms(unsigned int Del_1ms);
iic_ext void Delay_50ms(unsigned int Del_50ms);
iic_ext void picture_1();
iic_ext void fill_picture(unsigned char fill_Data);
iic_ext uchar IIC_Addresing(uchar addr);
iic_ext void OLED_Clear();
iic_ext void OLED_ShowCHinese(uchar x,uchar y,uchar n);
iic_ext void OLED_ShowString(uchar x,uchar y,uchar *ptr,uchar size);
iic_ext void OLED_ShowChar(uchar x,uchar y,uchar ptr,uchar size);
iic_ext void OLED_DrawBMP(unsigned char x0, unsigned char y0,unsigned char x1, unsigned char y1,unsigned char BMP[]);
iic_ext void OLED_ShowNum(uchar x,uchar y,uint num,uchar len,uchar size);
iic_ext void OLED_DrawPoint(uint x,uchar y,uchar t);
iic_ext void OLED_Refreash_Gram();
iic_ext void OLED_Fill(uchar x1,uchar y1,uchar x2,uchar y2,uchar dot);
iic_ext void Oled_RunShow(uchar y,uchar num,uchar len,uchar betw);
iic_ext void OLED_ShowOneHZ(uint x,uchar y,uchar chr,uchar size,uchar mode);
iic_ext void OLED_Clear_kuai(uchar x,uchar y,uchar dat);
iic_ext void OLED_ShowHz(uchar x,uchar y,uchar len,uchar size);
iic_ext void OLED_ShowBattery(uchar x,uchar y,uchar no);
iic_ext void OLED_ShowBatCharge(uchar x,uchar y,uchar len,uchar size);
iic_ext void OLED_DS1302_Num(uchar x,uchar y,uint num,uchar size);
```c
#define iic_c
#include<msp430x14x.h>
#include"iic.h"
#include"oled.h"
void delay()
{
unsigned char q0;
for(q0=0;q0<3;q0++)
{
_NOP();
}
}
void IIC_Start()
{
SDA_OUT;
SCL_OUT;
SET_SDA;
SET_SCL;
delay();
CLR_SDA;
delay();
CLR_SCL;
delay();
}
void IIC_Stop()
{
SDA_OUT;
SCL_OUT;
CLR_SCL;
CLR_SDA;
delay();
SET_SCL;
delay();
SET_SDA;
delay();
}
uchar Write_IIC_Byte(uchar dat)
{
uchar ack=0;
uchar mask;
SDA_OUT;
delay();
for(mask=0x80;mask!=0;mask>>=1)
{
if((mask&dat)==0)
{
CLR_SDA;
}
else
{
SET_SDA;
}
delay();
SET_SCL;
delay();
CLR_SCL;
delay();
}
SET_SDA;
delay();
SDA_IN;
SET_SCL;
delay();
ack=SDA_Read;
CLR_SCL;
delay();
return ack;
}
void Write_IIC_Command(unsigned char IIC_Command)
{
IIC_Start();
Write_IIC_Byte(0x78);
Write_IIC_Byte(0x00);
Write_IIC_Byte(IIC_Command);
IIC_Stop();
}
void Write_IIC_Data(unsigned char IIC_Data)
{
IIC_Start();
Write_IIC_Byte(0x78);
Write_IIC_Byte(0x40);
Write_IIC_Byte(IIC_Data);
IIC_Stop();
}
void OLED_WR_Byte(uchar dat,uchar cmd)
{
if(cmd)
{
Write_IIC_Data(dat);
}
else
{
Write_IIC_Command(dat);
}
}
void fill_picture(unsigned char fill_Data)
{
unsigned char m,n;
for(m=0;m<8;m++)
{
OLED_WR_Byte(0xb0+m,OLED_CMD);
OLED_WR_Byte(0x00,OLED_CMD);
OLED_WR_Byte(0x10,OLED_CMD);
for(n=0;n<128;n++)
{
OLED_WR_Byte(fill_Data,OLED_DATA);
}
}
}
#if(0)
void picture_1()
{
unsigned char x,y;
unsigned int i=0;
for(y=0;y<8;y++)
{
Write_IIC_Command(0xb0+y);
Write_IIC_Command(0x00);
Write_IIC_Command(0x10);
for(x=0;x<128;x++)
{
Write_IIC_Data(show1[i++]);
}
}
}
#endif
void Delay_50ms(unsigned int Del_50ms)
{
unsigned int m;
for(;Del_50ms>0;Del_50ms--)
for(m=6245;m>0;m--);
}
void Delay_1ms(unsigned int Del_1ms)
{
unsigned char j;
while(Del_1ms--)
{
for(j=0;j<123;j++);
}
}
void OLED_Set_Pos(uchar x,uchar y)
{
OLED_WR_Byte(0xb0+y,OLED_CMD);
OLED_WR_Byte((x&0x0f),OLED_CMD);
OLED_WR_Byte(((x&0xf0)>>4)|0x10,OLED_CMD);
}
void OLED_Clear_kuai(uchar x,uchar y,uchar dat)
{
OLED_Set_Pos(x,y);
OLED_WR_Byte(dat,OLED_DATA);
}
void OLED_Fill(uchar x1,uchar y1,uchar x2,uchar y2,uchar dot)
{
uchar x,y;
for(x=x1;x<x2;x++)
{
for(y=y1;y<y2;y++)
OLED_Clear_kuai(x,y,dot);
}
}
void OLED_Clear()
{
uchar i,n;
for(i=0;i<8;i++)
{
OLED_WR_Byte(0xb0+i,OLED_CMD);
OLED_WR_Byte(0x00,OLED_CMD);
OLED_WR_Byte(0x10,OLED_CMD);
for(n=0;n<128;n++) OLED_WR_Byte(0,OLED_DATA);
}
}
void OLED_ShowChar(uchar x,uchar y,uchar ptr,uchar size)
{
uchar c=0,i=0;
c=ptr-' ';
if(size==16)
{
OLED_Set_Pos(x,y);
for(i=0;i<8;i++)
{
OLED_WR_Byte(F8X16[c*16+i],OLED_DATA);
}
OLED_Set_Pos(x,y+1);
for(i=0;i<8;i++)
{
OLED_WR_Byte(F8X16[c*16+i+8],OLED_DATA);
}
}
}
void OLED_ShowString(uchar x,uchar y,uchar *ptr,uchar size)
{
uchar j=0;
while(ptr[j]!='\0')
{
OLED_ShowChar(x,y,ptr[j],size);
x+=8;
if(x>120){x=0;y+=2;}
j++;
}
}
void OLED_ShowCHinese(uchar x,uchar y,uchar no)
{
uchar i;
OLED_Set_Pos(x,y);
for(i=0;i<16;i++)
{
OLED_WR_Byte(Hzk[2*no][i],OLED_DATA);
}
OLED_Set_Pos(x,y+1);
for(i=0;i<16;i++)
{
OLED_WR_Byte(Hzk[2*no+1][i],OLED_DATA);
}
}
void OLED_ShowBattery(uchar x,uchar y,uchar no)
{
uchar i;
OLED_Set_Pos(x,y);
for(i=0;i<16;i++)
{
OLED_WR_Byte(baterry[no][i],OLED_DATA);
}
}
void OLED_ShowBatCharge(uchar x,uchar y,uchar len,uchar size)
{
uchar i;
for(i=0;i<len;i++)
{
OLED_ShowBattery(x,y,i);
}
}
void OLED_ShowHz(uchar x,uchar y,uchar len,uchar size)
{
uchar i;
for(i=0;i<len;i++)
{
OLED_ShowCHinese(x,y,i);
x+=size;
}
}
uint oled_pow(uchar m,uchar n)
{
uint result=1;
while(n--) result*=m;
return result;
}
void OLED_ShowNum(uchar x,uchar y,uint num,uchar len,uchar size)
{
uchar t,temp;
uchar enshow=0;
for(t=0;t<len;t++)
{
temp=(num/oled_pow(10,len-t-1))%10;
if(enshow==0&&t<(len-1))
{
if(temp==0)
{
OLED_ShowChar(x+(size/2)*t,y,'0',size);
continue;
}
else enshow=1;
}
OLED_ShowChar(x+(size/2)*t,y,temp+'0',size);
}
}
void OLED_DS1302_Num(uchar x,uchar y,uint num,uchar size)
{
uchar dat[3];
dat[0]=(num>>4)+0x30;
dat[1]=(num&0x0f)+0x30;
dat[2]='\0';
OLED_ShowString(x,y,dat,size);
}
void OLED_DrawBMP(unsigned char x0, unsigned char y0,unsigned char x1, unsigned char y1,unsigned char BMP[])
{
uint j=0;
uchar x,y;
if(y1%8==0) y=y1/8;
else y=y1/8+1;
for(y=y0;y<y1;y++)
{
OLED_Set_Pos(x0,y);
for(x=x0;x<x1;x++)
{
OLED_WR_Byte(BMP[j++],OLED_DATA);
}
}
}
void Initial_LY096BG30()
{
OLED_WR_Byte(0xAE,OLED_CMD);
OLED_WR_Byte(0x00,OLED_CMD);
OLED_WR_Byte(0x10,OLED_CMD);
OLED_WR_Byte(0x40,OLED_CMD);
OLED_WR_Byte(0xB0,OLED_CMD);
OLED_WR_Byte(0x81,OLED_CMD);
OLED_WR_Byte(0xFF,OLED_CMD);
OLED_WR_Byte(0xA1,OLED_CMD);
OLED_WR_Byte(0xA6,OLED_CMD);
OLED_WR_Byte(0xA8,OLED_CMD);
OLED_WR_Byte(0x3F,OLED_CMD);
OLED_WR_Byte(0xC8,OLED_CMD);
OLED_WR_Byte(0xD3,OLED_CMD);
OLED_WR_Byte(0x00,OLED_CMD);
OLED_WR_Byte(0xD5,OLED_CMD);
OLED_WR_Byte(0x80,OLED_CMD);
OLED_WR_Byte(0xD8,OLED_CMD);
OLED_WR_Byte(0x05,OLED_CMD);
OLED_WR_Byte(0xD9,OLED_CMD);
OLED_WR_Byte(0xF1,OLED_CMD);
OLED_WR_Byte(0xDA,OLED_CMD);
OLED_WR_Byte(0x12,OLED_CMD);
OLED_WR_Byte(0xDB,OLED_CMD);
OLED_WR_Byte(0x30,OLED_CMD);
OLED_WR_Byte(0x8D,OLED_CMD);
OLED_WR_Byte(0x14,OLED_CMD);
OLED_WR_Byte(0xAF,OLED_CMD);
}
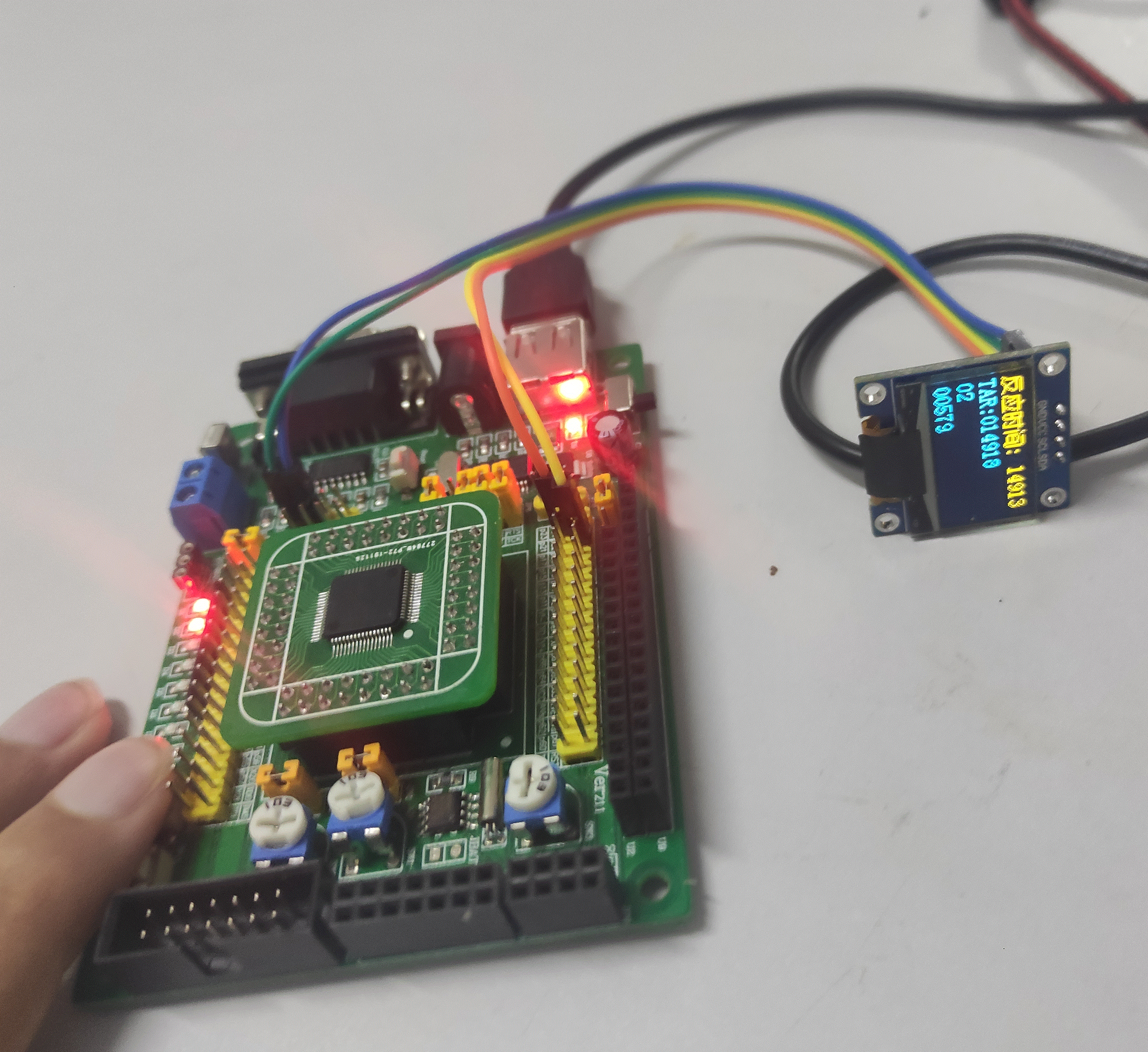