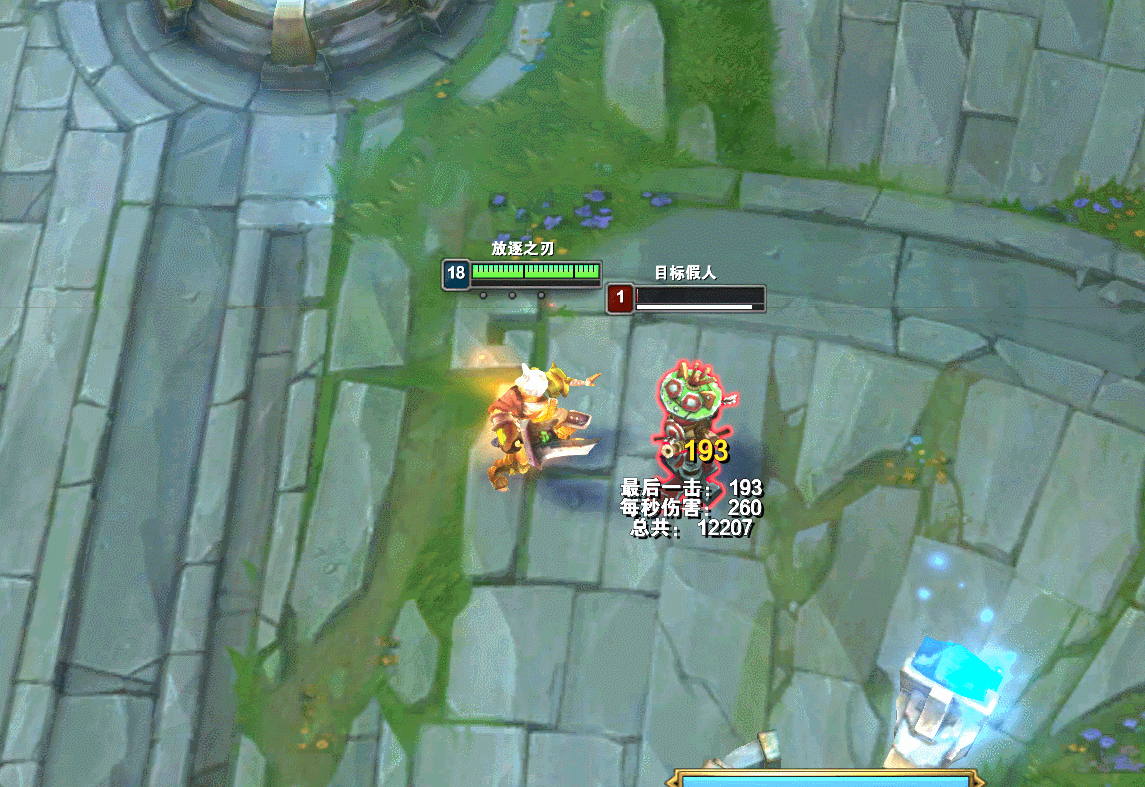
联盟优化首先要解决的是输入问题,这里首选语言是 Python,为什么要使用 Python 而不是使用 C++ ,原因如下:
- C++ 如果使用注入的方式来操作的话,效果是最好的,但是这样容易被检查进而封号;
- 如果不使用 C++ 的注入方式,使用 windll 中的 user32 来操作的话,Python 使用 ctypes 得到的效果和 C++ 基本一致;
- Python 是胶水语言,我们可以发挥想象力很方便的添加其他的功能;
一、windll 输入
在 Python 中使用 windll 输入主要是靠模拟 C++ 程序使用 ctypes 实现的,鼠标键盘的输入有两种方式,一种是 sendinput ,一种是 mouse_event 和 keybd_event;其中 mouse_event 和 keybd_event 已经被取代,因此这里只能使用 sendinput 函数来执行发送操作;
构建 sendinput 函数的结构参数如下:
from ctypes import (windll, byref, Union, POINTER, sizeof,
Structure, c_ulong, pointer, c_long, wintypes)
class POINT(Structure):
_fields_ = [("x", c_ulong),
("y", c_ulong)]
class MOUSEINPUT(Structure):
_fields_ = [("dx", c_long),
("dy", c_long),
("mouseData", wintypes.DWORD),
("dwFlags", wintypes.DWORD),
("time", wintypes.DWORD),
("dwExtraInfo", POINTER(c_ulong))]
class KEYBDINPUT(Structure):
_fields_ = [("wVk", wintypes.WORD),
("wScan", wintypes.WORD),
("dwFlags", wintypes.DWORD),
("time", wintypes.DWORD),
("dwExtraInfo", POINTER(c_ulong))]
class HARDWAREINPUT(Structure):
_fields_ = [("uMsg", wintypes.DWORD),
("wParamL"