from paddleocr import PaddleOCR, draw_ocr
import os
import cv2
from PIL import Image
import matplotlib.pyplot as plt
# Paddleocr目前支持的多语言语种可以通过修改lang参数进行切换
# 例如`ch`, `en`, `fr`, `german`, `korean`, `japan`
ocr = PaddleOCR(use_angle_cls=True, lang="en") # need to run only once to download and load model into memory
# 一张图片
path = 'VINImages/SMALL'
# path = '../yolov5-mask-42-master/runs/detect/exp13'
# path = r"D:\BUFFER\Pycharm\RecognitionOCR\images\ROI_Images"
# path = r"D:\BUFFER\Pycharm\RecognitionOCR\images\rudeOCR"
# 单张图片
# result = ocr.ocr(img_path, cls=True)
# # # 文件夹所有图片
# path = r"D:\BUFFER\Pycharm\DectVIN\VINImages\BIG"
def findVin_Index(vinSets):
list_vin = []
for i in vinSets:
address_index = [x for x in range(len(vinSets)) if vinSets[x] == i]
list_vin.append([i, address_index])
dict_address = dict(list_vin)
vin_index = []
vin_value = []
for key, value in dict_address.items():
vin_index.append(len(value))
vin_value.append(key)
real_vin = vin_value[vin_index.index(max(vin_index))]
# for k in range(len(real_vin)):
# if real_vin[k] == 'I':
# real_vin[k] = '1'
# if real_vin[k] == 'o':
# real_vin[k] = '0'
return real_vin,dict_address.get(real_vin)
# possibleVIN = []
# scoresVin = []
for filename in os.listdir(path): # listdir的参数是文件夹的路径
img_path = path + '\\' + filename
# print(filenames)
img_orig = cv2.imread(img_path, 1)
print(img_path)
# 文件夹下所有图片
result = ocr.ocr(img_path, cls=True)
possibleVIN = []
scoresVin = []
# # 显示结果
for line in result:
# print(line)
image = Image.open(img_path).convert('RGB')
boxes = [line[0] for line in result]
txts = [line[1][0] for line in result]
scores = [line[1][1] for line in result]
if len(txts[0]) == 17 and scores[0] > 0.98:
possibleVIN.append(txts[0])
scoresVin.append(scores[0])
# im_show = draw_ocr(image, boxes, txts, scores, font_path='./fonts/simfang.ttf')
# im_show = Image.fromarray(im_show)
# plt.imshow(im_show)
# plt.show()
allScores = 0.0
if possibleVIN:
print("possibleVIN",possibleVIN)
realvin, realvin_index = findVin_Index(possibleVIN)
print("scoresVin", scoresVin)
print("realvin_index",realvin_index)
for vin_item in realvin_index:
allScores = allScores + scoresVin[vin_item]
# if allScores:
# realScore = allScores/len(realvin_index)
# # print("realvin_index", realvin_index)
# # print("realVin", realvin)
# print("real-score",realScore)
cv2.putText(img_orig, (" %s, %0.5f" % (realvin, allScores/len(realvin_index))), (50, 50),
cv2.FONT_HERSHEY_SIMPLEX, 1, (255, 0, 0), 2)
cv2.imshow("vin", img_orig)
cv2.waitKey()
# im_show.show()
# im_show.save('res/result.jpg')
paddleocr识别VIN码
于 2022-04-28 09:14:38 首次发布
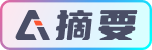