前言
月初刚刚入职,公司用的是自己的Unity框架,看了大半个月感觉还是一头雾水,于是在网上找到一套关于框架知识的视频教学课程,希望能够有所收获。视频中知识点比较繁杂,记下笔记以供日后复习。
1.工厂模式
工厂模式:所有的对象的创造都由一个对象去创造。
在我理解就是工厂类、工厂方法根据不同的参数、条件创造对应的对象。设计模式重要的不是记住代码而是理解思想。
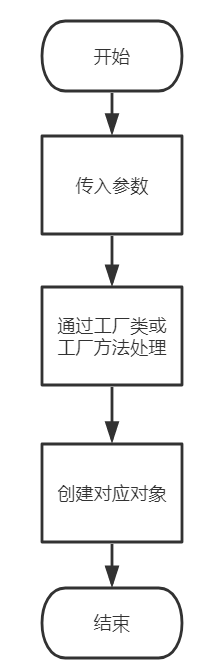
工厂类示例
//继承同一父类,根据参数实例子类。
public class Food //父类
{
public virtual void ShowMe()
{
}
}
public class TomatoFood : Food
{
public override void ShowMe()
{
base.ShowMe();
Debug.Log("TomatoFood");
}
}
public class EggFood : Food
{
public override void ShowMe()
{
base.ShowMe();
Debug.Log("EggFood");
}
}
public class Factory : MonoBehaviour
{
public Food ShowFactory(string name)
{
if(name == "TomatoFood")
{
return new TomatoFood();
}
else if(name == "EggFood")
{
return new EggFood();
}
else
{
return null;
}
}
}
public class Main
{
public void ShowMain()
{
Factory factory = new Factory();
Food food = factory.ShowFactory("EggFood");
}
}
工厂方法示例
//通过参数实例Image
public class SpriteFactory : MonoBehaviour
{
public Object[] allSprit;
int allIndex = 0;
void Start () {
allSprit = Resources.LoadAll ("Number");
}
//抽象出的工具类
public void LoadSprite(string name)
{
allSprit = Resources.LoadAll (name);
}
public GameObject GetSprite(int index)
{
GameObject tmpObj = new GameObject ("tmpGame");
Image tmpImage = tmpObj.AddComponent <Image>();
tmpImage.sprite = allSprit [index] as Sprite;
return tmpObj;
}
//工厂 方法
public GameObject GetImage(int index)
{
GameObject tmpObj = new GameObject ("tmpGame");
Image tmpImage = tmpObj.AddComponent <Image>();
tmpImage.sprite = allSprit [index] as Sprite;
return tmpObj;
}
void Update ()
{
if (Input.GetKeyDown (KeyCode.A)) {
GameObject tmpObj = GetImage (allIndex/10);
tmpObj.transform.parent = transform;
tmpObj.transform.position = new Vector3 (allIndex * 20, 20, 0);
}
}
}
2.存储讲解
2.1 堆栈理解
内存中一共分为三块区域:栈、堆、全局变量(static)
值类型保存在栈中,引用类型的声明保存在栈中,实际存储保存在堆中。
对象的声明(引用)保存在栈中,内容保存在堆中。
2.2 equl与==
1.equal 对比的是变量里的地址是否相等。
2. == 比较的内容是否一样。
3. string是特殊的引用类型, == 与 equal 相等。如果之后使用的string之前被创建过,会直接引用之前的内容。string的 == 操作符相当于(不确定)被重写过。
代码示例部分
void TestEqual()
{
//zhan 1001 //dui 3001
string tmpA = new string (new char[] {'H','L'});
// zhan 1002 // 3002
string tmpB = new string(new char[]{ 'H','L'});
Debug.Log (tmpA == tmpB); //true
Debug.Log (tmpA.Equals(tmpB));//true 因为string是特殊的引用类型
//zhan 1001
object tmpC = tmpA;
//zhan 1002
object tmpD = tmpB;
Debug.Log ("tmpc == tmpD");
Debug.Log (tmpC == tmpD); //false 因为未重写操作符
Debug.Log (tmpD.Equals(tmpC));//true 这里调用的是tmpB的重载,所以为true
Debug.Log ("Test Vector");
// zhan 1003 dui 3003
Vecter tmpVOne = new Vecter (1);
// zhan 1004 dui 3004
Vecter tmpVTwo = new Vecter (1);
object tmpVFour = tmpVTwo;
object tmpVFive = tmpVTwo;
Debug.Log (tmpVFour ==tmpVFive); //true 直接重载了
Debug.Log (tmpVFour.Equals(tmpVFive)); //true
Debug.Log (tmpVOne == tmpVTwo); //false 因为没有重写操作符,所以不是比较内容,重写后为true
Debug.Log (tmpVTwo.Equals(tmpVOne)); //false 地址不同
}
//操作符重写,重写后变true
public static bool operator ==(Vecter lhs, Vecter rhs)
{
if (lhs.xx == rhs.xx && lhs.yy == rhs.yy && lhs.name == rhs.name)
{
return true;
}
else
{
return false;
}
}
public static bool operator !=(Vecter lhs, Vecter rhs)
{
if (lhs.xx == rhs.xx && lhs.yy == rhs.yy && lhs.name == rhs.name)
{
return true;
}
else
{
return false;
}
}
2.3 函数参数传递
函数参数传递一共分为以下四种情况:
1.按值传递值类型
2.按值传递引用类型
3.按引用传递值类型
4.按引用传递引用类型
代码示例
public class TeachEqual : MonoBehaviour {
void Start () {
TestParams ();
}
public class Vector()
{
float xx;
public Vector(float xx)
{
this.xx == xx;
}
}
//x地址1005,结果为1,相当于赋值,并不改变实际内容(按值传递值类型)
public void Food(int x)
{
x = 10;
}
//输出为10,ref相当于直接拿来,操作地址中内容(按值传递引用类型)
public void Food2(ref int tmpX)
{
tmpX = 10;
}
//输出为10,相当于两个地址指向堆中同一内存(按引用传递值类型)
public void Food3(Vecter tmpVect1 )
{
tmpVect1.xx = 10;
}
//输出为1,tmpVect2指向tmpVect,但在函数中又指向了新的tmpVect,所以外界的tmpVect不受影响(按引用传递值类型)
public void Food4(Vecter tmpVect2)
{
// 10011 // 300010
Vecter tmpVect = new Vecter (10);
tmpVect2 = tmpVect;
}
//输出为10,ref相当于直接拿来,少了Food4中一次指向切换(按引用传递引用类型)
public void Food5(ref Vecter tmpVect2)
{
Vecter tmpVect = new Vecter (10);
tmpVect2 = tmpVect;
Vecter tmpVect3 = new Vecter(1);
tmpVect2 = tmpVect3;
}
void TestParams()
{
int a = 1;
Vecter tmpVect = new Vecter (1);
Food(a);
Food2(ref a);
Food3 (tmpVect);
Food4(tmpVect);
Food5 (ref tmpVect);
}