- 委托是一种特殊的类,它允许捕捉对方法的引用,并像传递其他对象那样传递该引用,像调用其他方法那样调用该方法。
- 默认委托包括无返回值的泛型委托Action<>、有返回值的泛型委托Func<>。
- 调用方法的方式有两种,一种是利用方法名直接调用,另一种是利用委托间接调用。
- 同步调用是在同一线程内依次调用,异步调用是在不同线程内同时调用。
- 直接同步调用:利用方法名。
- 间接同步调用:调用委托的Invoke()。
- 显式异步调用:利用Thread类或者Task类。
- 隐式异步调用:调用委托的BeginInvoke()。
- 调用Invoke()后需要等待方法执行完毕才能继续执行主线程,调用BeginInvoke()后可以继续执行主线程。
- 多播委托是利用操作符【+=】和【-=】将多个方法的引用赋给委托对象,使其一次性可以依次调用多个方法。
- 一般情况下默认委托完全可以满足需求,不需要再声明自定义委托。
using System;
namespace _11
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("*****测试无参的Action*****");
Action action1 = new Action(Test1.Print1);
action1();
Console.WriteLine("==========");
action1 += Test2.Print1;
action1();
Console.WriteLine("==========");
action1 -= Test1.Print1;
action1.Invoke();
Console.WriteLine("==========");
action1 -= Test2.Print1;
action1?.Invoke();
Console.WriteLine("==========");
Console.WriteLine();
Console.WriteLine("*****测试有参的Action*****");
Action<string> action2 = new Action<string>(Test1.Print2);
action2("Test1");
Console.WriteLine("==========");
action2 += Test2.Print2;
action2("Test1Test2");
Console.WriteLine("==========");
action2 -= Test1.Print2;
action2.Invoke("Test2");
Console.WriteLine("==========");
action2 -= Test2.Print2;
action2?.Invoke("null");
Console.WriteLine("==========");
Console.WriteLine();
Console.WriteLine("*****测试一个参数的Func*****");
Func<string, string> func1 = new Func<string, string>(Test1.Print3);
Console.WriteLine(func1.Invoke("Test1"));
Console.WriteLine("==========");
Console.WriteLine();
Console.WriteLine("*****测试多个参数的Func*****");
Func<string, int, string> func2 = new Func<string, int, string>(Test1.Print4);
Console.WriteLine(func2.Invoke("Test1", 3));
Console.WriteLine("==========");
Console.WriteLine();
Console.WriteLine("*****测试自定义委托*****");
Delegete1 delegete1 = new Delegete1(Test1.Print1);
delegete1.Invoke();
Console.WriteLine("==========");
Delegete2 delegete2 = new Delegete2(Test1.Print2);
delegete2.Invoke("Test1");
Console.WriteLine("==========");
Delegete3 delegete3 = new Delegete3(Test1.Print3);
Console.WriteLine(delegete3.Invoke("Test1"));
Console.WriteLine("==========");
Delegete4<string, int, string> delegete4 = new Delegete4<string, int, string>(Test1.Print4);
Console.WriteLine(delegete4.Invoke("Test1", 3));
Console.WriteLine("==========");
Console.WriteLine();
}
}
class Test1
{
public static void Print1()
{
Console.WriteLine("Test1");
}
public static void Print2(string str)
{
Console.WriteLine(str);
}
public static string Print3(string str)
{
return str;
}
public static string Print4(string str, int count)
{
string newStr = str;
for (int i = 1; i < count; i++)
{
newStr += str;
}
return newStr;
}
}
class Test2
{
public static void Print1()
{
Console.WriteLine("Test2");
}
public static void Print2(string str)
{
Console.WriteLine(str);
}
}
delegate void Delegete1();
delegate void Delegete2(string str);
delegate string Delegete3(string str);
delegate TResult Delegete4<T1, T2, TResult>(T1 t1, T2 t2);
}
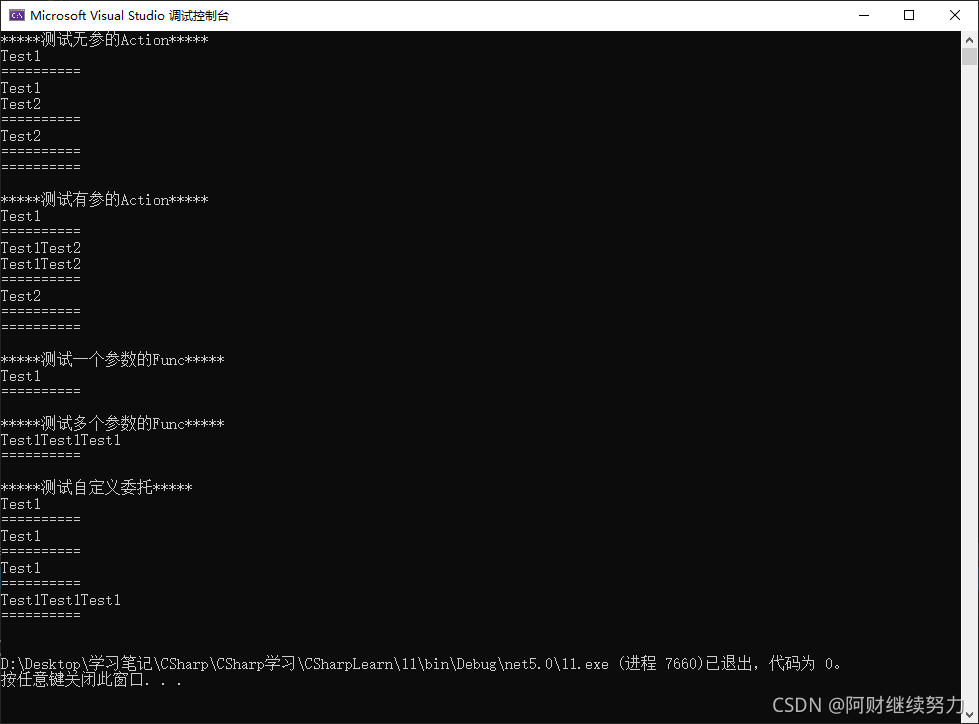