1.1 What is an embedded system?
- Shrinking led to the first single-chip computer processor, Intel’s 4-bit 4004 in 1971, known as a microprocessor (“micro” meaning small).
- An embedded system is a computer system embedded in another device.
- Desktop PC Not embedded in another device.
- Electronic drums (musical instrument) True
- Elevator (lift) True
- Cloud server False
- Cardiac pacemaker True
- Integrated circuits (a.k.a. ICs or chips), on which microprocessors are implemented, have been doubling in transistor capacity roughly every 18 months, a trend known as Moore’s Law.
【习题总结】
- Moore’s Law suggests IC capacity doubles about every 18 months.
- Consider a chip in a smartphone today. If Moore’s Law holds, that chip in 6 years would hold how many times more transistors?222*2 = 16
- Consider a chip in a smartphone today. If Moore’s Law holds, the transistors within that chip in 12 years would occupy what fraction of the chip’s current size? 1 / 256
1.2 Basic components
- A bit (short for “binary digit”) is one digit of such a two-valued item.
- A bit’s changing value over time is called a digital signal.
- A switch is an electromechanical component with a pair of electrical contacts.
- A light emitting diode or LED is a semiconductor with a pair of contacts.
- A microcontroller is a programmable component that reads inputs and writes outputs according to some internally-stored program that computes.
- The while (1) { } loop is a common feature of a C program for embedded systems and is called an infinite loop, causing the contained statements to repeat continually.
- The switch and buttons are examples of sensors, which convert physical phenomena into digital inputs to the embedded system.
- The LED is an example of an actuator, which converts digital outputs from the embedded system into physical phenomena.
【习题总结】
- A button outputs 1 Volt when pressed, 0 Volts otherwise. False The actual voltage varies on the electrical setup, but a common abstraction is to consider a press as a 1 (not 1 Volts, just 1).
- An LED’s electronics are designed to turn on when the input voltage exceeds 1 Volt.** False** The actual voltage varies depending on the LED. But an abstraction is to say that a 1 turns on an LED (not 1 Volts, just 1).
- A microcontroller performs computations that convert input values to output values. True
- A microcontroller cannot be programmed. False
1.3 RIMS
- RIMS (Riverside-Irvine Microcontroller Simulator) is a graphical web-based tool that supports C programming and simulated execution of RIM.
- Pressing “Compile” translates the program code to executable machine code.True
- When a program is executing (by pressing Run), the values of inputs A0, A1, A2, …, cannot be changed. Flase In fact, during execution is when the user will want to change the input values, to see how the program responds.
- #include “RIMS.h” is required atop all C files for RIMS. True
- The “Slowest” simulation speed causes a highlighting of the next C statement to execute.True
1.4 Timing diagrams
- A timing diagram shows time proceeding to the right, and plots the value of bit signals as either 1 (high) or 0 (low).
- A change on a signal is called an event.
- If a signal changes from 0 to 1, the event is called rising. 1 to 0 is called falling.
- A pulse is a signal portion started by a rising event and ended by a falling event (looks like a camel hump).
1.5 Testing
- Because testing usually can’t cover all input combinations, testing should cover border cases, which are fringe or extreme cases such as all inputs being 0s and all inputs being 1s, and then various normal cases.
- If code has branches, then good testing also ensures that every statement in the code is executed at least once, known as 100% code coverage.
- To manually trace a program means to mentally execute the program, perhaps with the aid of paper and pencil.
- Input value combinations, known as test vectors, can be described in RIMS rather than each input value combination being generated by clicking on switches.
- The check output event is also known as an assertion.
2.1 C in embedded systems
【习题】
- C and the related C++ language represented the main language for over 80% of embedded programmers (from 2013 survey).True
- C was originally designed for embedded systems.False C was created in 1972 for mainframe systems; embedded systems didn’t really exist in 1972 (processors were too big).
- Assembly language is the main language for nearly half of embedded programmers.
Flase Assembly dominated in early embedded systems days (1980s) due to the need for extremely efficient code, but today’s compilers generate efficient code, plus most embedded systems are too big to manage in assembly. Sometimes critical program pieces still need to be written in assembly though. - Fluency in C is rarely useful outside embedded systems.False C is still used in desktop/server systems. Also, C is the basis for languages like C++, Objective-C, C#, and even Java. And, many people believe that C fluency makes for strong programmers in any language due to better program-execution knowledge.
2.2 C data types
2.3 RIMS implicitly defined I/O variables
RIMS implicit variables
unsigned char A; // Built-in variable A, representing RIMS' 8 input
// pins as a single 8-bit variable
unsigned char B; // Built-in variable B, representing RIMS' 8 output
// pins as a single 8-bit variable
- Built-in grouped bits like A and B enable the programmer to treat RIMS’ 8 input bits or 8 output bits as an 8-bit decimal number.
【习题总结】
- Write a statement, ending with a semicolon, for each desired behavior (unless otherwise specified). Write “not possible” if appropriate.
- Set RIMS’ first output pin to 1.B0 = 1;
- Set RIMS’ 8 output bits to decimal 0.B = 0;
- Set RIMS’ first output to RIMS’ last input. B0 = A7
- Set RIMS’ outputs to RIMS’s inputs minus 1, treating each as a decimal number.**B = A - 1; **
- Set A7 to 1. Not possible
- Set variable x to B7’s current value.x=B7;
- Write an expression (without parentheses) that evaluates to true if A5 is 1.
if(A5){
x = B0;
}
2.5 Bitwise operators
- & : bitwise AND — 1 if both bit-operands are 1s.
- | : bitwise OR — 1 if either or both bit-operands are 1s.
- ^ : bitwise XOR — 1 if exactly one of the two bit-operands is 1 (eXclusive OR)
- ~ : bitwise NOT — 1 if the bit-operand is 0; 0 if bit-operand is 1.
#include "RIMS.h"
void main()
{
unsigned char sound1 = 0;
unsigned char sound2 = 0;
while (1) {
sound1 = A & 0x0F; // 0000A3A2A1A0
sound2 = A >> 4; // A7A6A5A4A3A2A1A0 --> 0000A7A6A5A4
B = sound1 + sound2;
}
}
2.6 Shift operators
- bitpat << amt : Left shift bitpat by amt positions
- bitpat >> amt : Right shift bitpat by amt positions
2.7 Bit access functions
Expression to set a particular bit to 1.
x | (0x01 << k) // Evaluates to x but with k'th bit set to 1
Expression to set a particular bit to 0.
x & ~(0x01 << k) // Evaluates to x but with k'th bit set to 0
SetBit() function to set a particular bit to 0 or 1.
unsigned char SetBit(unsigned char x, unsigned char k, unsigned char b) {
return (b ? (x | (0x01 << k)) : (x & ~(0x01 << k)) );
// Set bit to 1 Set bit to 0
}
- The function uses C’s ternary conditional operator (?😃.
Example using SetBit() function.
unsigned char i, val;
val = A0;
for (i = 0; i < 4; i++) {
B = SetBit(B, i, val);
}
GetBit() function to get a particular bit.
unsigned char GetBit(unsigned char x, unsigned char k) {
return ((x & (0x01 << k)) != 0);
}
2.8 Rounding and overflow
- Expressions involving integer division should be treated with extra care due to error that is introduced from rounding during integer division, wherein any fraction is truncated.
- Postponing division, as in (a * b * c) / d, can reduce occurrences of overflow. False Doing division sooner can prevent intermediate values from becoming very large.
- Given unsigned char variables a, b, and c, if each may range from 0-100, then (a+b+c)/3 may overflow.
- T
- Given unsigned char variables a and b, if each may range from 0-100, then (a + b) / (2 * a) may overflow.F a+b may reach 200, which is less than 255. Likewise, 2 *a may reach 200, which is less than 255.
- If a function’s parameters p1 and p2 and return type are all unsigned short types, casting parameters first to unsigned long types may reduce overflow. T
- If a function has a parameter that has been cast to a larger type to prevent overflow, multiplying the parameter by 10, then dividing a final result by 10, can further reduce overflow.F Such multiplication and division can reduce rounding error, not overflow.
3.1 Time-ordered behavior
- C uses a sequential instructions computation model, wherein statements (instructions) in a list are executed sequentially (one after another) until the list’s end is reached.
- A sequential instructions model is good for capturing algorithms that transform given input data into output data, known as data processing behavior.
【习题总结】
- Raise a toll-gate, wait for a car to pass, lower the toll gate. Time-ordered
- Find the maximum value in a set of 100 integers.Data-processing
- Given two four-bit inputs, compute their sum and average as two four-bit outputs.Data-processing
- When a person is detected approaching the front of a door, automatically open the door until sensors in front of and behind the door no longer detect anybody.Time-ordered
- A wrong-way system has 10 sensors on a freeway offramp. If a car is driving the wrong way, the sensors will detect a car in the opposite order as normal. The system should flash a “Wrong way” sign and notify the police. Time-ordered
3.2 State machines
- A state machine is a computation model intended for capturing time-ordered behavior.
- A drawing of a state machine is called a state diagram.
- For a state machine to be precisely defined, transitions leaving a particular state should have mutually exclusive transition conditions
Pulse counting with reset SM.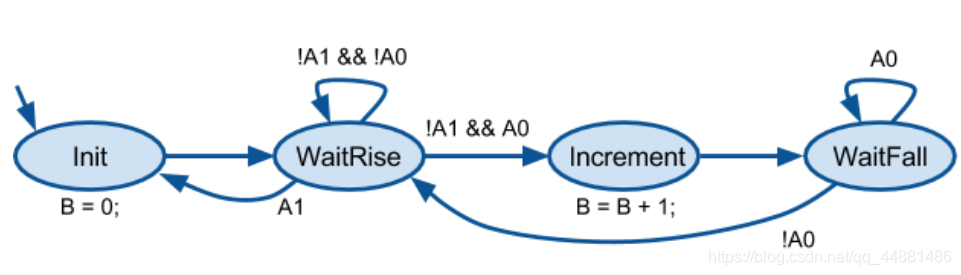
Given the above SM input sequence, type the SM’s current state at the specified times. At time 0 ms, the answer is: Init.
- 0s : Init
- 0.5s:WaitRise
- 1s:WaitFall
- 1.5s: WaitRise
- What is the integer value of B at time 4 s? 3
- What is the integer value of B at time 5 s?0
【问题总结】
- An SM tick consists of:Transitioning to the next state and executing that state’s actions once
- How many SM ticks occur per second? Many
- In the SM model, which is true about ticks and input events?Tick occur faster than events
3.3 RIBS
- The RIBS (Riverside-Irvine Builder of State machines) tool supports graphical state diagram capture of SMs.
3.4 Implementing an SM in C
#include "RIMS.h"
enum LA_States { LA_SMStart, LA_s0, LA_s1 } LA_State;
void TickFct_Latch()
{
switch(LA_State) { // Transitions
case LA_SMStart: // Initial transition
LA_State = LA_s0;
break;
case LA_s0:
if (!A0) {
LA_State = LA_s0;
}
else if (A0) {
LA_State = LA_s1;
}
break;
case LA_s1:
if (!A0) {
LA_State = LA_s0;
}
else if (A0) {
LA_State = LA_s1;
}
break;
default:
LA_State = LA_SMStart;
break;
} // Transitions
switch(LA_State) { // State actions
case LA_s0:
break;
case LA_s1:
B0 = A1;
break;
default:
break;
} // State actions
}
void main() {
B = 0x00; // Initialize outputs
LA_State = LA_SMStart; // Indicates initial call
while(1) {
TickFct_Latch();
}
}
- enum is a C construct for defining a new data type, in contrast to built-in types like char or short, whose value can be one of an “enumerated” list of values.
- The SM’s tick function carries out one tick of the SM.
#include "RIMS.h"
enum CR_States { CR_SMStart, CR_Init, CR_WaitRise, CR_Increment, CR_WaitFall } CR_State;
void TickFct_Carousel()
{
switch(CR_State) { // Transitions
case CR_SMStart: // Initial transition
CR_State = CR_Init;
break;
case CR_Init:
CR_State = CR_WaitRise;
break;
case CR_WaitRise:
if (A1) {
CR_State = CR_Init;
}
else if (!A1 && !A0) {
CR_State = CR_WaitRise;
}
else if (!A1 && A0) {
CR_State = CR_Increment;
}
break;
case CR_Increment:
CR_State = CR_WaitFall;
break;
case CR_WaitFall:
if (!A0) {
CR_State = CR_WaitRise;
}
else if (A0) {
CR_State = CR_WaitFall;
}
break;
default:
CR_State = CR_SMStart;
break;
} // Transitions
switch(CR_State) { // State actions
case CR_Init:
B = 0;
break;
case CR_WaitRise:
break;
case CR_Increment:
B = B + 1;
break;
case CR_WaitFall:
break;
default:
break;
} // State actions
}
void main() {
B = 0x00; // Initialize outputs
CR_State = CR_SMStart; // Indicates initial call
while(1) {
TickFct_Carousel();
}
}
【习题总结】
- The tick function’s first switch statement will execute the current state’s actions. F
- The first switch statement’s first case will include: case LT_SMStart: LT_State = LT_unlit;T
- The first switch statement’s second case will be for the transitions going to state Unlit.F
- The tick function’s second switch statement will include: case (LT_Unlit): if (!A0) { B0 = 1;}…F
- The SM to C method uses an if-else statement for multiple transitions, but could have just used multiple if statements.T
- If a state has no actions, the state should be omitted from the second case statement.F
- The break statements could be removed from the switch statements without changing behavior, but should be included for clarity.F
- A transition from a state back to that same state can be omitted from the first switch statement without changing the SM’s behavior.T
3.5 Variables, statements, and conditions in SMs
【问题总结】
- A programmer can declare variables within each SM state.F
- An SM variable maintains its value across SM ticks.T
- Failing to write an SM variable in a particular state causes that variable to become 0.F
- When implementing an SM in C, the SM’s variables should be declared in the main() function.F
- A state’s actions may include a for loop.T
- A state’s actions may include a function call.T
- The statement if (A0) {…} should not appear in a state’s actions.F
- The statement while (A0) {…} should not appear in a state’s actions.T
Remember that transition conditions are expressions, not statements, so should not end with a semicolon. For each, write the most direct answer. Ex: For “A1 and A0 are true”, write A1 && A0, in that order, without parentheses, and without == 1
- Write the condition for a transition that should be taken if either A1 or A0 is true.A1 || A0
- Write the condition that detects that A is greater than or equal to 99.A >= 99
- Write the condition that detects that A2A1A0 is 010. Use individual bit variables A2, A1, A0.!A2 && A1 && !A0
- A designer intended to have one transition taken if A0 is 1; otherwise, a second transition is taken if A1 is 0 and a third taken if A1 is 1. The designer wrote the conditions A0, !A1, and A1, which are not mutually exclusive. Fix the second condition.!A1 && !A0
- A designer intended to have one transition taken if exactly one of A1 or A0 is 1, and a second transition taken if both are 0s. The designer wrote the conditions as (A1 && !A0) || (!A1 && A0) and as !A1 && !A0. A third transition is missing; write its condition.A1 && A0
- A designer has two transitions leaving a state. One transition’s condition is (A3 || A2 || A1). The second transition’s condition is “other”. What expression does the other represent? Write the expression with the fewest changes to the first expression.**!(A3 || A2 || A1)
** - A designer has three transitions leaving a state. One transition’s condition is (!A1 && !A0). A second transition’s condition is (A1 && A0). A third transition’s condition is “other”. What expression does the other represent? Start with: !((!A1 … Don’t try to simplify.!((!A1 && !A0) || (A1 && A0))
3.6 Mealy actions
- The earlier state machine model associates actions with states only, known as a Moore-type state machine.
【习题总结】
- A Mealy action occurs at which point during an SM tick?While taking a transition to the next state
- Can a Mealy action include an if-else statement?yes
- Integer variable X is 0. A transition with action X = X + 1 points to a state with action X = X + 2. If that transition is taken during an SM tick, what is X after the tick?
3
3.7 How to capture behavior as an SM
- The above process suggests creating one state at a time, creating that state’s actions and all the states outgoing transitions, before moving on to create another state.F
- Step 3 for the emergency door example determined that a transition with condition A0 is needed from Armed to Unarmed.F
- Upon adding a transition !A0 from Armed to Unarmed, the transition A1 from Armed should be changed to A1 && A0.T
3.8 Testing an SM
- scenario is a sequence of inputs that should cause a particular sequence of state.
- Such testing is in contrast to merely generating a good set of input combinations for a system lacking internal states, each input combination known as a test vector.
【习题总结】
Match the test vectors with the desired test scenario for the above emergency door system. Assume each set of test vectors is applied immediately after starting the system, so the starting state is always Unarmed. Each vector is for A1A0, so 01 means A1 is 0 and A0 is 1.
- 01, 11, 01, 11:Arm the system open the door close the door
- 01, 00, 01, 00:Arm the syste, disarm,arm,disarm
- 01, 00, 10:Arm the system, disarm the system open the door
- 01, 10:Arm the system then simulataneously open the door and disarm the system
3.9 Capture/convert process
- The first step is to capture the desired behavior using a computation model appropriate for the desired system behavior, such as SMs. The second step is to convert that captured behavior into an implementation, such as C that will run on a microprocessor.
#include "RIMS.h"
unsigned char x;
enum EX_States { EX_SMStart, EX_S0, EX_S2, EX_S1 } EX_State;
void TickFct_Example() {
switch(EX_State) { // Transitions
case EX_SMStart:
EX_State = EX_S0;
break;
case EX_S0:
if (1) {
EX_State = EX_S1;
}
break;
case EX_S2:
if (A3) {
EX_State = EX_S2;
}
else if (!A3) {
EX_State = EX_S1;
}
break;
case EX_S1:
if (!A3) {
EX_State = EX_S1;
}
else if (A3) {
EX_State = EX_S2;
x = A & 0x07;
B = x;
}
break;
default:
EX_State = EX_SMStart;
break;
}
switch(EX_State) { // State actions
case EX_S0:
x = 0;
B = x;
break;
case EX_S2:
break;
case EX_S1:
break;
default:
break;
}
}
void main() {
EX_State = EX_SMStart; // Initial state
B = 0; // Init outputs
while(1) {
TickFct_Example();
}
}
- Many other state machine models exist, such as UML state machines.
【习题总结】
- “Capture” refers to describing desired system behavior in the most appropriate computation model.T
- “Convert” refers to describing desired system behavior directly in C.F
- A system has two 4-bit inputs, and continually sets a 5-bit output to their average. The system is best captured as an SM, then converted to C.F
- Because an SM can be converted to C, then C directly supports the SM computation model.F
4.1 Time-interval behavior
- Time-interval behavior is system functionality where events must be separated by specified intervals of real time. Consider a system that should repeatedly blink an LED on for 500 ms and off for 500 ms.
【习题总结】
- A toll booth gate should rise when the operator presses a button, and stay risen until no car is detected near the gate.F
- A system should set B0 to 1 whenever A0 has been one for at least one second.T
- An electronic turnstyle should count up whenever someone passes through (A0 will pulse), lighting an LED while they pass. When the 1000th person passes through, the turnstyle should sound a tone for five seconds.T
4.2 Synchronous SMs
- The tick rate can instead be set to a specific rate such as 500 ms, known as the SM’s period. An SM with a specific period is a synchronous SM, or synchSM for short.
Type B’s value as eight bits, as in: 10000000. Assume the synchSM starts in state Init at time 0 sec. If appropriate, type: Unknown. - What is B at time 0.2 sec? 00000001
- What is B at time 1.5 sec?**00000010 **
- At what time will a tick cause NextLed’s if branch to be executed? Just type an integer (assumed to be in seconds)).8
4.3 SynchSMs and time intervals for inputs
- One common input time-interval scenario is to read input values only at certain times, each read known as a sample.
【习题总结】
Consider the above RunnerTime synchSM, starting in state Init at time 0 sec. Each question follows the previous one in time. Type B’s value as an integer.
- Assume A0 and A1 have remained 0s. What is B at time 5 sec?0
- Assume a runner reaches A0 just before time 5 sec (e.g., at 4.95 sec). What is B at time 6 sec, if the runner has not yet reached A1?0
- The runner reaches A1 at time 6.05 sec and causes A1 to pulse from 6.1 sec to 6.3 sec. Assuming A1 and A0 stay 0 after then, what is B at time 7 sec?6
In the above example, would the result be different if the A0 pulse lasted 450 ms versus lasting 200 ms? Answer yes or no.** No **
4.4 Choosing a period for different time intervals
- The idea is to choose the period as the greatest common divisor or gcd of the required time intervals, and then use multiple states (or counting within a state) to obtain the actual desired interval.
4.5 Microcontrollers with timers
- A timer is a hardware component that can be programmed to tick at a user-specified rate, such as once every 100 ms.
- Interrupt means to temporarily stop execution of the main program and call a special C function known as an interrupt service routine or ISR.
- In RIMS, the ISR is called TimerISR. The TimerISR function can be defined by the user as follows:
void TimerISR() {
// user inserts code here
}
-T he user sets the timer’s tick rate by calling another RIMS built-in function, TimerSet(period), where period is an unsigned short indicating the tick period in milliseconds. To activate the timer, the user calls TimerOn().
- A flag is a global variable used by different parts of a C program to communicate basic status information with one another.
A simple example using an ISR to set a flag.
#include "RIMS.h"
volatile unsigned char TimerFlag = 0;
void TimerISR() {
TimerFlag = 1;
}
void main() {
B = 0;//Initialize output
TimerSet(1000); // Timer period = 1000 ms (1 sec)
TimerOn(); // Turn timer on
while (1) {
B0 = !B0; // Toggle B0
while(!TimerFlag) {} // Wait 1 sec
TimerFlag = 0;
// NOTE: better style would use a synchSM
// This example just illustrates use of an ISR and flag
}
}
- A small percentage of microcontrollers come with a built-in timer.F
- A timer tick causes the hardware to automatically stop the microcontrollers main program, and call an ISR.T
- Standard timers are set to tick every one second.F
- After an ISR finishes executing, the main() function starts from its beginning again.F
- If a main() program has 10 instructions, and a timer is set to tick every 1 second, then 10 instructions will execute between each ISR call.F
- If a timer is set to tick every 100 ms and enabled, then the ISR will be called every 100 ms.F
- The TimerFlag variable was declared volatile because the compiler might not realize the variable is being updated, and may therefore optimize away code that shouldn’t be.T
4.6 Converting a synchSM to C
#include "RIMS.h"
volatile unsigned char TimerFlag=0; // ISR raises, main() lowers
void TimerISR() {
TimerFlag = 1;
}
enum BL_States { BL_SMStart, BL_LedOff, BL_LedOn } BL_State;
void TickFct_Blink() {
switch ( BL_State ) { //Transitions
case BL_SMStart:
BL_State = BL_LedOff; //Initial state
break;
case BL_LedOff:
BL_State = BL_LedOn;
break;
case BL_LedOn:
BL_State = BL_LedOff;
break;
default:
BL_State = BL_SMStart;
break;
}
switch (BL_State ) { //State actions
case BL_LedOff:
B0 = 0;
break;
case BL_LedOn:
B0 = 1;
break;
default:
break;
}
}
void main() {
B = 0; //Init outputs
TimerSet(2000);
TimerOn();
BL_State = BL_SMStart; // Indicates initial call to tick-fct
while (1) {
TickFct_Blink(); // Execute one synchSM tick
while (!TimerFlag){} // Wait for BL's period
TimerFlag = 0; // Lower flag
}
}
#include "RIMS.h"
volatile unsigned char TimerFlag;
void TimerISR() {
TimerFlag = 1 ;
}
enum BOA_States { BOA_SMStart, BOA_Init, BOA_BlinkOn, BOA_BlinkOff } BOA_State;
TickFct_BlinkLongOff() {
int i;
switch(BOA_State) { // Transitions
case BOA_SMStart:
BOA_State = BOA_Init;
break;
case BOA_Init:
if (1) {
BOA_State = BOA_BlinkOn;
i = 0;
}
break;
case BOA_BlinkOn:
if (i<2) {
BOA_State = BOA_BlinkOn;
}
else if (!(i < 2)) {
BOA_State = BOA_BlinkOff;
i = 0;
}
break;
case BOA_BlinkOff:
if (!(i<6)) {
BOA_State = BOA_BlinkOn;
i = 0;
}
else if (i < 6) {
BOA_State = BOA_BlinkOff;
}
break;
default:
BOA_State = BOA_SMStart;
} // Transitions
switch(BOA_State) { // State actions
case BOA_Init:
B = 0x00;
break;
case BOA_BlinkOn:
B0 = 1;
++i;
break;
case BOA_BlinkOff:
B0 = 0;
++i;
break;
default:
break;
} // State actions
}
int main() {
const unsigned int periodBlinkLongOff = 500;
TimerSet( periodBlinkLongOff);
TimerOn();
BOA_State = BOA_SMStart; // Initial state
B = 0; // Init outputs
while(1) {
TickFct_BlinkLongOff();
while( !TimerFlag);
TimerFlag = 0;
} // while (1)
} // Main
4.7 State actions should never wait
- A state can have a action such as while(!A0) as long as the designer can reasonably expect A0 to be pressed quickly.F
- Run to completion means that the actions of a state always should execute and reach their end.T
- Functions should not be called in state actions.F
- In the extended crosswalk SM example above, counters are used instead of while loops in the state actions.T
5.1 Concurrent synchSMs
- A task is a unique continuously-executing behavior, such as the task of toggling an LED whenever a button is pressed, or the task of sounding an alarm when motion is sensed.
- Concurrent tasks are tasks that execute at the same time.
- A block diagram shows each task as a block (a rectangle), and uses a directed line to show that a block writes to an output (or reads from an input).
【习题总结】
Consider the above LedShow two-task system. Assume the system starts at time 0 in the initial states LedOff and T0.
- In what state is task BlinkLeds at time 1001 ms?** LedOn **
- In what state is task ThreeLeds at time 1001 ms?** T1**
- In what state is task BlinkLeds at time 4001 ms?** LedOff **
- In what state is task ThreeLeds at time 4001 ms?** T1**
- The system starts in states LedOff and T0. At what earliest time will the system again enter both those states? ** 6000**
5.2 Shared variables
【习题总结】
Consider the above Motion-triggered lamp three-task system. Assume the system starts at time 0 in the initial states, and that A0 becomes 1 at time 100 ms and stays 1 until time 550 ms. Enter times in ms without units, as in: 500.
- At what time does DetectMotion set global variable mtn to 1?400
- At what time does IlluminateLamp set B1 to 1? Assume that IlluminateLamp’s ticks occur slightly after DetectMotion’s when those ticks are for the same time.**400 **
- After A0 has been 0 for at least 400 milliseconds, could the LED still be on? Answer yes or no.No
- Which situation can cause the above IlluminateLamp synchSM to miss the pulse on mtn, given that IlluminateLamp and DetectMotion both have 200 ms periods?DetectMontion ticks slightly after illuminateLamp at 200 ms and slightly before at 400ms
5.3 Converting multiple synchSMs to C
#include "RIMS.h"
// LedShow C code, having two tasks
volatile unsigned char TimerFlag=0;
void TimerISR() {
TimerFlag = 1;
}
enum BL_States { BL_SMStart, BL_LedOff, BL_LedOn } BL_State;
void TickFct_BlinkLed() {
... // Standard switch statements for SM
}
enum TL_States { TL_SMStart, TL_T0, TL_T1, TL_T2 } TL_State;
void TickFct_ThreeLeds() {
... // Standard switch statements for SM
}
void main() {
B = 0; // Init outputs
TimerSet(1000);
TimerOn();
BL_State = BL_SMStart;
TL_State = TL_SMStart;
while (1) {
TickFct_BlinkLed(); // Tick the BlinkLed synchSM
TickFct_ThreeLeds(); // Tick the ThreeLeds synchSM
while (!TimerFlag){} // Wait for timer period
TimerFlag = 0; // Lower flag raised by timer
}
}
unsigned char cnt;
unsigned char i;
while (1) { // Repeatedly look for four 1s on A
cnt=0;
for (i=0; i<8; i++) {
if (GetBit(A, i)) {
cnt++;
}
}
B1 = (cnt >= 4);
}
The synchSM of a sequential code task gets ticked just like other synchSMs.
while (1) {
TickFct_BlinkLed(); // Tick the BlinkLed synchSM
TickFct_ThreeLeds(); // Tick the ThreeLeds synchSM
TickFct_CountFour(); // Tick the CountFour synchSM (was seq code task)
while (!TimerFlag){} // Wait for timer period
TimerFlag = 0; // Lower flag raised by timer
}
Sequential code synchSM simplified in C.
unsigned char cnt;
unsigned char i;
void TickFct_CountFour() { // single-state synchSM
cnt=0;
for (i=0; i<8; i++) {
if (GetBit(A, i)) {
cnt++;
}
}
B1 = (cnt >= 4);
}
【问题总结】
When converting two 500 ms tasks to C:
- Only slight modification of each synchSM tick function’s internal code is necessary as compared to if each synchSM was the only task.F
- The main() function can be written to call the two tick functions in either order.T
- The order in which the tick functions are called in C will not affect the system’s output.F
- If the statement B7 = !A7 is a third task, the task can be re-captured as a single-state synchSM with a period of 500 ms.T
- If an SM (no period) should execute along with the two synchSMs, the SM should be converted to a synchSM with a 1 ms period before converting to C.F
- A synchSM with just one state, having just one transition with condition true and pointing back to that state, can have a tick function just having the state’s actions (and no switch statements).T
5.4 Converting synchSM local variables to C
- A variable’s scope refers to the regions of a system that can see that variable. For a multiple synchSM system, a local variable’s scope is limited to a particular synchSM, while a global variable’s scope spans multiple synchSMs.
- Prepending the C keyword static to the local variable definitions causes those variables to be permanent and thus maintain their values across function calls, as required to correctly execute a synchSM.
Global and local variables converted to C, using state local variables.
unsigned char mtn; // Global variable for synchSMs
// is converted to C global
void TickFct_DetectMotion() { // DetectMotion synchSM tick function
...
}
void TickFct_IlluminateLamp() { // IlluminateLamp synchSM tick function
static unsigned char cnt; // Local synchSM variable converted to
// C static local
...
}
【习题总结】
- Typically, a variable named i used for ticking a particular state a specific number of times is defined as a synchSM global variable.F
- A variable set by one synchSM and read by another synchSM must be defined globally in a multi-synchSM system.T
- When converting to C, a multi-synchSM system’s global variable should be converted to a C global variable.T
- When converting to C, a synchSM’s local variable should be converted to a C global variable.F
- Prepending the keyword static to a function definition causes all local variables to have their values remembered between calls to the function.F
- In a multi-synchSM system, only one synchSM can have a local variable named i for counting. A second synchSM wanting a similar counter variable should use a different name like j.T
5.5 Keeping distinct behaviors distinct
【习题总结】
Indicate whether the behavior would be best captured as two synchSMs or just one synchSM.
- B0 always blinks 500 ms on and 500 ms off, while B7 always blinks 1000 ms on and 500 ms off.2
- B0 always blinks 500 ms on and 500 ms off. B7 sounds a tone whenever B0 is blinking on.One
- A button press on A7 causes A3…A0 to be sampled and B to output the average of the previous four samples. Any pulse on A7 less than 200 ms is ignored.2
5.6 Task communication
- Communication is the sharing of information by one task with another.
- The most basic communication method involves one task writing to a global variable and another task (or multiple tasks) reading that variable – the variable is shared.
- A handshake is a method for task X to cause task Y to carry out some behavior.
【问题总结】
- Only one task should write to a global shared variable, and only one task should read that global variable.F
- A handshake requires two signals, a request signal set by the first task, and an acknowledge task set by the second task.T
- A handshake only works if the two tasks operate at the same rate.F
5.7 Queues
- Message passing is the communication behavior of sending a data item from a sender task to a receiver task such that the item is treated as a distinct message that is transferred.
- A queue can hold up to N data items.
- The term FIFO is commonly used instead of queue, short for first-in first-out, which describes how data is inserted and read/removed. A queue with no data items is said to be empty and cannot be popped (until after a push), while a size N queue with N data items inserted is said to be full and cannot be pushed (until after a pop). A queue is sometimes called a buffer. Items in a queue are said to be queued or buffered.
- Push: Add an item to the back of a queue
- queue:A FIFO data structure used to hold multiple items
- FIFO: First-in-first-out describes how a data structure interacts with items
- message passing: Treats inter-task communication as distinct message items
- pop:Remove an item from the front of a queue
For the following questions, refer to the above synchSMs. All function definitions of Q4uc can be found in the previous table. If both synchSMs are scheduled to tick, assume Receive ticks first. - What is the size of the queue (number of items)?4
- After 101 ms, will the queue ever be empty? no
- What is the item at the front of the queue after 350 ms?1
- What is the value of B after 550 ms?1
- What is the item at the front of the queue after 550 ms?2
- What are the contents of the queue after 550 ms? Answer using commas to separate queue items, as in “5, 7, 14, 2”.2, 3, 4, 5
- What are the contents of the queue after 650 ms? Answer using commas to separate queue items, as in “5, 7, 14, 2”.2, 3, 4, 5