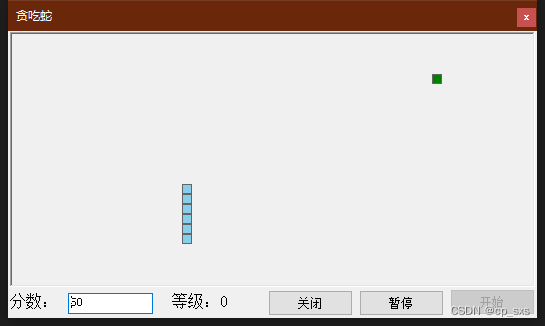
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Curriculum_design
{
internal interface IDraw
{
void DrawSnake();
}
}
using System;
using System.Collections;
using System.Collections.Generic;
using System.Diagnostics;
using System.Drawing;
using System.Linq;
using System.Reflection.Emit;
using System.Runtime.Remoting.Messaging;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Curriculum_design
{
internal class Snake : IDraw
{
public System.Windows.Forms.Label _body;
private Color _color = Color.SkyBlue;
private Way way = Way.WEST;
private int speed = 500;
private int foodCount = 0;
private ArrayList snake;
private bool hasFood = false;
public Color Color { get => _color; set => _color = value; }
public Way SnakeWay
{
set
{
this.way = value;
}
get
{
return this.way;
}
}
public int Speed { get => speed;set => speed = value; }
public int FoodCount { get => foodCount; set => foodCount = value; }
public void DrawSnake()
{
snake = new ArrayList();
for(int i = 0;i < 5;i++)
{
_body = new System.Windows.Forms.Label();
_body.BackColor = _color;
_body.Size = new Size(10, 10);
_body.BorderStyle = BorderStyle.FixedSingle;
_body.Location = new Point(200 + i * 10, 150);
snake.Add(_body);
}
}
public ArrayList GetSnake()
{
return snake;
}
public void Move(Control control)
{
if(!this.hasFood)
{
control.Controls.Remove(control.GetChildAtPoint(((System.Windows.Forms.Label)snake[snake.Count - 1]).Location));
snake.RemoveAt(snake.Count - 1);
}
System.Windows.Forms.Label temp = new System.Windows.Forms.Label();
CopyBody(temp, (System.Windows.Forms.Label)snake[0]);
switch(this.way)
{
case Way.WEST:
{
temp.Left -= 10;
snake.Insert(0, temp);
break;
}
case Way.EAST:
{
temp.Left += 10;
snake.Insert(0, temp);
break;
}
case Way.NORTH:
{
temp.Top -= 10;
snake.Insert(0, temp);
break;
}
case Way.SOUTH:
{
temp.Top += 10;
snake.Insert(0, temp);
break;
}
}
control.Controls.Add((System.Windows.Forms.Label)snake[0]);
if(this.hasFood)
{
this.hasFood = false;
}
}
public bool Eat(Point food)
{
if (((System.Windows.Forms.Label)snake[0]).Left == food.X && ((System.Windows.Forms.Label)snake[0]).Top == food.Y)
{
this.hasFood = true;
return true;
}
return false;
}
public void CopyBody(System.Windows.Forms.Label x, System.Windows.Forms.Label y)
{
x.Location = y.Location;
x.BackColor = y.BackColor;
x.Size = y.Size;
x.BorderStyle = y.BorderStyle;
}
}
}
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Curriculum_design
{
internal class Food
{
private Point foodPoint;
private Color foodColor = Color.Green;
private Size foodSize = new Size(10, 10);
public Point FoodPoint { get => foodPoint;set => foodPoint = value; }
public Color FoodColor { get => foodColor;set => foodColor = value; }
public Size FoodSize { get => foodSize; set => foodSize = value; }
}
}
using System;
using System.Collections;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Runtime.ExceptionServices;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
using static System.Windows.Forms.VisualStyles.VisualStyleElement.Tab;
namespace Curriculum_design
{
public enum Way
{
NORTH,
SOUTH,
WEST,
EAST,
}
public partial class Form1 : Form
{
Snake snake = new Snake();
Food foods = new Food();
private Thread game;
private bool flag = false;
private delegate void DrawDele();
private DrawDele drawDelegate;
public Form1()
{
InitializeComponent();
tbScore.Text = "0";
btnSuspend.Enabled = false;
CheckForIllegalCrossThreadCalls = false;
}
private void btnStart_Click(object sender, EventArgs e)
{
if (snake._body != null)
panel1.Controls.Clear();
snake.DrawSnake();
game = new Thread(new ThreadStart(StartGame));
game.Start();
this.btnStart.Enabled = false;
btnSuspend.Enabled = true;
}
private void StartGame()
{
drawDelegate = new DrawDele(PutFood);
this.Invoke(drawDelegate, null);
while (true)
{
Thread.Sleep(snake.Speed);
if (this.IsGameOver())
{
btnSuspend.Enabled = false;
btnStart.Enabled = true;
MessageBox.Show("游戏结束,您的分数:" + tbScore.Text,"提示");
tbScore.Text = "0";
if (game.IsAlive)
{
game.Abort();
}
}
if (snake.Eat(foods.FoodPoint))
{
snake.FoodCount++;
SetText(Convert.ToString(snake.FoodCount * 50));
drawDelegate = new DrawDele(KillFood);
this.Invoke(drawDelegate, null);
drawDelegate = new DrawDele(PutFood);
this.Invoke(drawDelegate, null);
}
ShowScoreAndLevel();
drawDelegate = new DrawDele(MoveSnake);
this.Invoke(drawDelegate, null);
}
}
private void ShowScoreAndLevel()
{
if (tbScore.Text == "100")
{
snake.Speed = 400;
snake.Color = Color.Red;
foods.FoodColor = Color.Red;
lblevel.Text = "等级:1";
}
if (tbScore.Text == "200")
{
snake.Speed = 300;
snake.Color = Color.Black;
lblevel.Text = "等级:2";
}
if (tbScore.Text == "300")
{
snake.Speed = 200;
lblevel.Text = "等级:3";
}
if (tbScore.Text == "400")
{
snake.Speed = 100;
lblevel.Text = "等级:4";
}
if (tbScore.Text == "500")
{
snake.Speed = 50;
lblevel.Text = "等级:5";
}
if (tbScore.Text == "600")
{
MessageBox.Show("恭喜您,成功通关!分数为:" + tbScore.Text, "提示");
if (game.IsAlive)
{
game.Abort();
}
}
}
private void PutFood()
{
Random rand = new Random();
int x = rand.Next(510);
int y = rand.Next(230);
Label food = new Label();
food.Size = foods.FoodSize;
food.BorderStyle = BorderStyle.FixedSingle;
food.BackColor = foods.FoodColor;
if (x < 10)
x += 10;
if (y < 10)
y += 10;
food.Location = new Point(x % 10 == 0 ? x : x + (10 - x % 10),y % 10 == 0 ? y : y + (10 - y % 10));
foods.FoodPoint = new Point(food.Left, food.Top);
panel1.Controls.Add(food);
}
private void KillFood()
{
panel1.Controls.Remove(panel1.GetChildAtPoint(foods.FoodPoint));
}
private bool IsGameOver()
{
ArrayList temp = snake.GetSnake();
Label head = (Label)temp[0];
foreach(Label lb in temp.GetRange(1,temp.Count - 1))
{
if(lb.Left == head.Left && lb.Top == head.Top)
return true;
}
if (((Label)snake.GetSnake()[0]).Left == 0 || ((Label)snake.GetSnake()[0]).Left == 520 ||
((Label)snake.GetSnake()[0]).Top == 0 || ((Label)snake.GetSnake()[0]).Top == 240)
return true;
return false;
}
private void MoveSnake()
{
snake.Move(panel1);
}
private delegate void SetTextCallback(string text);
private void SetText(string text)
{
if (this.tbScore.InvokeRequired)
{
SetTextCallback sc = new SetTextCallback(SetText);
this.Invoke(sc, new object[] { text });
}
else
this.tbScore.Text = text;
}
private void Form1_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyData == Keys.Left)
this.snake.SnakeWay = (this.snake.SnakeWay == Way.EAST) ? Way.EAST : Way.WEST;
else if (e.KeyData == Keys.Right)
this.snake.SnakeWay = (this.snake.SnakeWay == Way.WEST) ? Way.WEST : Way.EAST;
else if (e.KeyData == Keys.Up)
this.snake.SnakeWay = (this.snake.SnakeWay == Way.SOUTH) ? Way.SOUTH : Way.NORTH;
else if (e.KeyData == Keys.Down)
this.snake.SnakeWay = (this.snake.SnakeWay == Way.NORTH) ? Way.NORTH : Way.SOUTH;
}
private void btnSuspend_Click(object sender, EventArgs e)
{
if (flag == false)
{
flag = true;
game.Suspend();
btnSuspend.Text = "继续";
}
else
{
flag = false;
game.Resume();
btnSuspend.Text = "暂停";
}
}
private void btnClose_Click(object sender, EventArgs e)
{
if(game != null)
game.Abort();
this.Close();
}
}
}