目录
配置
调试配置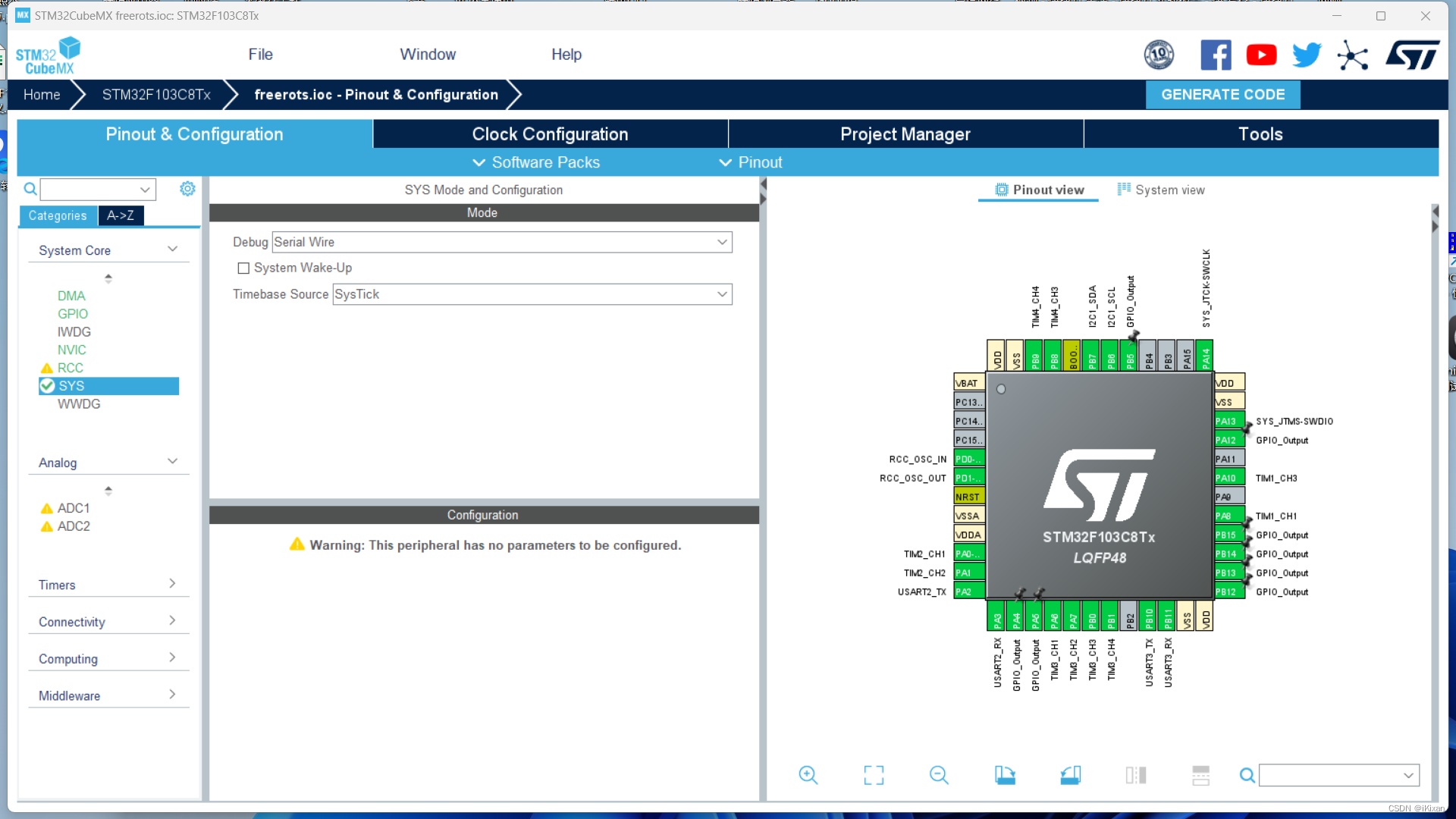
时钟配置
定时器配置
打开定时器3的PWM生成模式,预分频系数72-1,自动重装载系数1000-1,会生成一个周期为1ms,占空比精度为0.1%的PWM波。
配置GPIO
选择推挽输出,用于确定电机转向。
代码
motor.h
#ifndef __MOTOR_H__
#define __MOTOR_H__
void motor_Control(int a,int speed);
void motor_init();
#endif
motor.c
#include "motor.h"
#include "tim.h"
void motor_init(){
HAL_TIM_PWM_Start(&htim3,TIM_CHANNEL_1);
HAL_TIM_PWM_Start(&htim3,TIM_CHANNEL_2);
HAL_TIM_PWM_Start(&htim3,TIM_CHANNEL_3);
HAL_TIM_PWM_Start(&htim3,TIM_CHANNEL_4);
}
void motor_Control(int a,int speed){
if(a==1)
{
if(speed>=0)
{
if(speed>=1000)
{
speed=1000;
}
HAL_GPIO_WritePin(GPIOA, GPIO_PIN_4, GPIO_PIN_RESET);
HAL_GPIO_WritePin(GPIOA, GPIO_PIN_5, GPIO_PIN_SET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_1,speed);
}
else
{ if(speed<=-1000)
{
speed=-1000;
}
HAL_GPIO_WritePin(GPIOA, GPIO_PIN_4,GPIO_PIN_SET);
HAL_GPIO_WritePin(GPIOA, GPIO_PIN_5,GPIO_PIN_RESET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_1,-speed);
}
}
if(a==2)
{
if(speed>=0)
{
if(speed>=1000)
{
speed=1000;
}
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_12, GPIO_PIN_RESET);
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_13, GPIO_PIN_SET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_2,speed);
}
else
{
if(speed<=-1000)
{
speed=-1000;
}
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_12,GPIO_PIN_SET);
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_13, GPIO_PIN_RESET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_2,-speed);
}
}
if(a==3)
{
if(speed>=0)
{
if(speed>=1000)
{
speed=1000;
}
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_14, GPIO_PIN_RESET);
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_15, GPIO_PIN_SET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_3,speed);
}
else
{
if(speed<=-1000)
{
speed=-1000;
}
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_14,GPIO_PIN_SET);
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_15, GPIO_PIN_RESET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_3,-speed);
}
}
if(a==4)
{
if(speed>=0)
{
if(speed>=1000)
{
speed=1000;
}
HAL_GPIO_WritePin(GPIOA, GPIO_PIN_12, GPIO_PIN_RESET);
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_5, GPIO_PIN_SET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_4,speed);
}
else
{
if(speed<=-1000)
{
speed=-1000;
}
HAL_GPIO_WritePin(GPIOA, GPIO_PIN_12,GPIO_PIN_SET);
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_5 ,GPIO_PIN_RESET);
__HAL_TIM_SetCompare(&htim3, TIM_CHANNEL_4,-speed);
}
}
}