时间序列压缩python
Sequence unpacking in python allows you to take objects in a collection and store them in variables for later use. This is particularly useful when a function or method returns a sequence of objects. In this post, we will discuss sequence unpacking in python.
python中的序列解压缩使您可以将对象收集到集合中,并将它们存储在变量中以备后用。 当函数或方法返回对象序列时,这特别有用。 在本文中,我们将讨论python中的序列解压缩。
Let’s get started!
让我们开始吧!
A key feature of python is that any sequence can be unpacked into variables by assignment. Consider a list of values corresponding to the attributes of a specific run on the Nike run application. This list will contain the date, pace (minutes), time (minutes), distance (miles) and elevation (feet) for a run:
python的一个关键特性是,可以通过赋值将任何序列解压缩为变量。 考虑与Nike run应用程序上特定跑步的属性对应的值列表。 此列表将包含跑步的日期,速度(分钟),时间(分钟),距离(英里)和海拔(英尺):
new_run = ['09/01/2020', '10:00', 60, 6, 100]
We can unpack this list with appropriately named variables by assignment:
我们可以通过赋值来使用适当命名的变量来解压缩此列表:
date, pace, time, distance, elevation = new_run
We can then print the values for these variables to validate that the assignments were correct:
然后,我们可以打印这些变量的值以验证分配是否正确:
print("Date: ", date)
print("Pace: ", pace, 'min')
print("Time: ", time, 'min')
print("Distance: ", distance, 'miles')
print("Elevation: ", elevation, 'feet')

The elements in the sequence we are unpacking can be sequences as well. For example, in addition to the average pace of the overall run, we can have a tuple of mile splits:
我们要解包的序列中的元素也可以是序列。 例如,除了总体运行的平均速度外,我们还可以进行英里划分的元组:
new_run_with_splits = ['09/01/2020', '10:00', 60, 6, 100, ('12:00', '12:00', '10:00', '11;00', '8:00', '7:00')]
Let’s now unpack our new sequence:
现在让我们解压我们的新序列:
date, pace, time, distance, elevation, splits = new_run_with_splits
And we can print the mile splits:
我们可以打印英里分割:
print("Mile splits: ", splits)

We can even further unpack the splits list in the previous code. Let’s unpack the split lists with variables denoting the mile number:
我们甚至可以进一步解压缩先前代码中的拆分列表。 让我们使用表示英里数的变量来分解拆分列表:
date, pace, time, distance, elevation, (mile_2, mile_2, mile3_, mile_4, mile_5, mile_6) = new_run_with_splits
Let’s print the mile variables:
让我们打印mile变量:
print("Mile Two: ", mile_2)
print("Mile Three: ", mile_3)
print("Mile Four: ", mile_4)
print("Mile Five: ", mile_5)
print("Mile Six: ", mile_6)
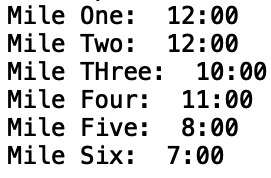
We can also use the ‘_’ character to leave out unwanted values. For example if we want to leave out date and elevation we do:
我们还可以使用'_'字符来省略不必要的值。 例如,如果我们要省略日期和海拔高度,则可以执行以下操作:
_, pace, time, distance, _, (mile_2, mile_2, mile_3, mile_4, mile_5, mile_6) = new_run_with_splits
We can also unpack an arbitrary number of elements using the python “star expression” (*). For example, if we want to store the first and last variables and store the middle values in a list we can do the following:
我们还可以使用python“星号表达式”(*)解压缩任意数量的元素。 例如,如果我们要存储第一个和最后一个变量并将中间值存储在列表中,则可以执行以下操作:
date, pace, time, distance, elevation, (first, *middle, last) = new_run_with_splits
Let’s print the first, middle and last variables:
让我们打印第一个,中间和最后一个变量:
print("First: ", first)
print("Middlle: ", middle)
print("Last: ", last

I’d like to emphasize that the objects in our sequence can be of any type. For example, we could have used a dictionary instead of a tuple for the mile splits:
我想强调一下,我们序列中的对象可以是任何类型。 例如,对于英里分割,我们可以使用字典而不是元组:
new_run_with_splits_dict = ['09/01/2020', '10:00', 60, 6, 100, {'mile_1': '12:00', 'mile_2':'12:00', 'mile3':'10:00', 'mile_4':'11;00', 'mile_5':'8:00', 'mile_6':'7:00'}]
Let’s unpack our new list:
让我们解压缩我们的新列表:
date, pace, time, distance, elevation, splits_dict = new_run_with_splits_dict
Now we can access the mile split values by key:
现在我们可以通过键访问英里分割值:
print("Mile One: ", splits_dict['mile_1'])
print("Mile Two: ", splits_dict['mile_2'])
print("Mile Three: ", splits_dict['mile_3'])
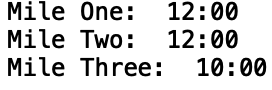
I’ll stop here but I encourage you to play around with the code yourself.
我将在这里停止,但我鼓励您自己尝试使用该代码。
结论 (CONCLUSIONS)
In this post, we discussed how to unpack sequences in python. First, we showed how to unpack a list of values associated with a run published to the Nike Run application. We also showed that the values in the sequences can also be sequences, where the sequence or its elements can be stored in separate variables for later use. We then showed how we can leave values out using the underscore character. Next, we discussed how to unpack an arbitrary number of objects using the python “star expression”. Finally, we demonstrated how we can unpack a dictionary object from a list of objects and access the dictionary values by key. I hope you found this post interesting/useful. The code in this post is available on GitHub. Thank you for reading!
在这篇文章中,我们讨论了如何在python中解压缩序列。 首先,我们展示了如何解压缩与发布到Nike Run应用程序中的跑步相关的值列表。 我们还显示了序列中的值也可以是序列,其中序列或其元素可以存储在单独的变量中以备后用。 然后,我们展示了如何使用下划线字符来省略值。 接下来,我们讨论了如何使用python“ star expression”解压缩任意数量的对象。 最后,我们演示了如何从对象列表中解压缩字典对象并通过键访问字典值。 希望您觉得这篇文章有趣/有用。 这篇文章中的代码可以在GitHub上找到 。 感谢您的阅读!
翻译自: https://towardsdatascience.com/sequence-unpacking-in-python-14d995f9a619
时间序列压缩python