ios轮播图创建
Give more life to a basic spinning image gallery.
为基本的旋转图像库赋予更多生命。
This tutorial builds off of a previous tutorial I did on creating a basic spinning wheel image carousel. Check that out here before moving on:
本教程以我之前创建基本的纺车图像传送带所做的教程为基础 。 在继续之前检查一下这里:
Or check out the video tutorial here:
或在此处查看视频教程:
我们有两种能力可以补充: (We have two abilities to add to our wheel:)
- Make sure the images don’t appear upside down when we rotate the wheel. 确保在旋转转轮时图像不会倒置。
Establish a snapping point; when we finish rotating the wheel, the wheel will automatically rotate to the card closest to that snapping point.
建立一个捕捉点 ; 当我们完成旋转轮子时,轮子将自动旋转到最接近该捕捉点的卡片。
让我们开始。 (Let’s begin.)
We’ll start by balancing the images so they don’t appear upside down. This is simple, we’ll just rotate the images in the opposite direction that we rotate the wheel…
我们将从平衡图像开始,以便它们不会上下颠倒。 这很简单,我们只需要沿与旋转轮子相反的方向旋转图像即可。

Note:1. We’ve converted the units that spin the wheel from radians to degrees by dividing ‘event.deltaY’ by 360.2. We’ve added ‘transitionDelay’ and ‘transitionDuration’ attributes to the wheel.
注意: 1.我们已将' event.deltaY '除以30.2,从而将使车轮旋转的单位从弧度转换为度。 我们已经在转盘中添加了“ transitionDelay ”和“ transitionDuration ”属性。
Now, before we get to writing that snapping point function, we need to setup two things:
现在,在开始编写该捕捉点函数之前,我们需要设置两件事 :
- We need some way of knowing when all of our images have loaded into the page; if not, the user could technically scroll the wheel before our gallery loads and this would break our code. 我们需要某种方式来知道何时所有图像都已加载到页面中。 如果不是这样,用户可以从技术上讲在我们的画廊加载之前滚轮,这会破坏我们的代码。
- We need some way of preventing the user from spinning the wheel while our snapping point function is running. 我们需要某种方法来防止用户在我们的捕捉点功能运行时旋转车轮。
To solve problem one, we’ll create a function that allows a counter in our “Wheel.js” component to increase, then pass that function to each “Card.js” component.
为了解决第一个问题 ,我们将创建一个函数,该函数允许“ Wheel.js ”组件中的计数器增加,然后将该函数传递给每个“ Card.js ”组件。
When a card/image mounts (‘componentDidMount()’ life cycle) it will execute that function.
当卡/图像挂载(' componentDidMount() '生命周期)时,它将执行该功能。

To solve problem two, we already have a mechanism that tells us when our wheel has stopped spinning, the ‘setTimeout’.’
为了解决问题二 ,我们已经有了一种机制,它告诉我们轮子何时停止旋转,即“ setTimeout ”。
We know that we want to call our ‘snap back’ function after the wheel stops, so we’ll track the ‘snap_in_progress’ variable using the state; and in our ‘onWheel’ listener we’ll just test that there isn’t a “snap in progress” and that all of our cards haveloaded.
我们知道,我们想在车轮停止后调用' snap back '函数,因此我们将使用state来跟踪' snap_in_progress '变量; 在我们的“ onWheel ”监听器中,我们将测试是否没有“ 进行中 ”,并且所有卡都已加载。

Note:We call the ‘snap_back()’ function after our wheel has stopped spinning. We’ll write this function later.
注意:车轮停止旋转后,我们将调用' snap_back() '函数。 我们稍后将编写此函数。
到那个捕捉功能... (On to that snapping function…)
We need to calculate three things:
我们需要计算三件事 :
- The angle that the snapping point falls on. 捕捉点落在的角度上。
- The closest card or image to that snapping point once our wheel stops spinning. 车轮停止旋转后,最接近该捕捉点的卡片或图像。
- The angle difference between the snapping point and that closest card. 捕捉点和最近的卡片之间的角度差。
所有这些都需要一些三角函数。 (All of this requires some Trigonometry.)
1.We’ll calculate the angle of the snapping point, by taking the X and Y coordinates of any card/image and feed it into an inverse tan function.
1.我们将通过获取任何卡/图像的X和Y坐标并将其馈入反正切函数来计算捕捉点的角度。


Note:1. We add the ‘snap_point’ to our state.2. In the ‘children_loaded()’ function, we establish the ‘X’ and ‘Y’ coordinates of our snapping point once all cards have loaded.3. We calculate the angle of our snapping point in the ‘snap_back()’ function.
注意: 1.我们将' snap_point '添加到我们的状态中2。 在“ children_loaded() ”函数中, 一旦所有卡都已加载 ,我们就为捕捉点建立了“ X”和“ Y”坐标。 3.我们在“ snap_back() ”函数中计算捕捉点的角度。
2. To determine the closest card to our snapping point, we’ll compare the distance from the snapping point to all the other cards/images and we’ll choose the distance with the lowest value.
2.为了确定离我们的捕捉点最近的卡片,我们将比较从捕捉点到所有其他卡片/图像的距离,并选择值最小的距离。

Note:We use the Pythagorean Theorem to calculate the distance between snapping points and cards.
注意:我们使用勾股定理来计算捕捉点和卡片之间的距离。

Note:1. We start by choosing any card to be the shortest distance, doesn’t matter which; we need to start with something.2. We then loop through all of the cards, including the one we picked (doesn’t matter), we calculate and compare distances to find the lowest number.
注意: 1.我们从选择任何一张最短距离的卡开始,这无关紧要; 我们需要从 .2。 开始 。 然后,我们遍历所有卡片,包括我们挑选的卡片(没关系),我们计算并比较距离以找到最小的卡片。
3.To get the difference in angles between our snapping point and closest card, we’ll calculate the angle of our closest card using the inverse tan function (like earlier), and we’ll just take the difference between the two.
3.为了获得我们的捕捉点和最接近的卡片之间的角度差异,我们将使用反正切tan函数(如先前所述)来计算最接近的卡片的角度,而我们将取两者之间的差异。

Finally, we’ll spin the wheel and images(in the opposite direction) using the ‘theta_between’ angle we’ve calculated…
最后 ,我们将使用我们计算出的“ theta_between ”角度旋转车轮和图像( 朝相反方向 )…

Note:1. The ‘if’ conditional tree makes sure the wheel is rotating in the correct direction based on which QUADRANT the snapping point and image fall in. For more information on this, check out my video tutorial; link down below.2. Remember to change the ‘snap_in_progress’ to false and keep track of how much the wheel spun with ‘theta’.
注:1,“ 如果 ”条件树可以确保车轮沿着正确的方向在此基础上象限捕捉点和图像落在旋转有关更多信息,请参阅我的视频教程; 链接低于 .2。 请记住将' snap_in_progress '更改为false,并跟踪使用' theta '旋转的车轮多少。
让我们快速跳转到我们的“ Card.js”并更新一些代码。 (Let’s quickly jump to our ‘Card.js’ and update some code.)
All we really need to do is convert this FUNCTION component into a CLASS component and remember to call that ‘amloaded()’ function in the ‘componentDidMount()’ life cycle function.
我们真正需要做的就是将这个FUNCTION组件转换为CLASS组件,并记住在' componentDidMount() '生命周期函数中调用该' amloaded() '函数。

Note: Easily change the JSX DIV element in the render function to a JSX IMG element.
注意:轻松将render函数中的JSX DIV元素更改为JSX IMG元素。
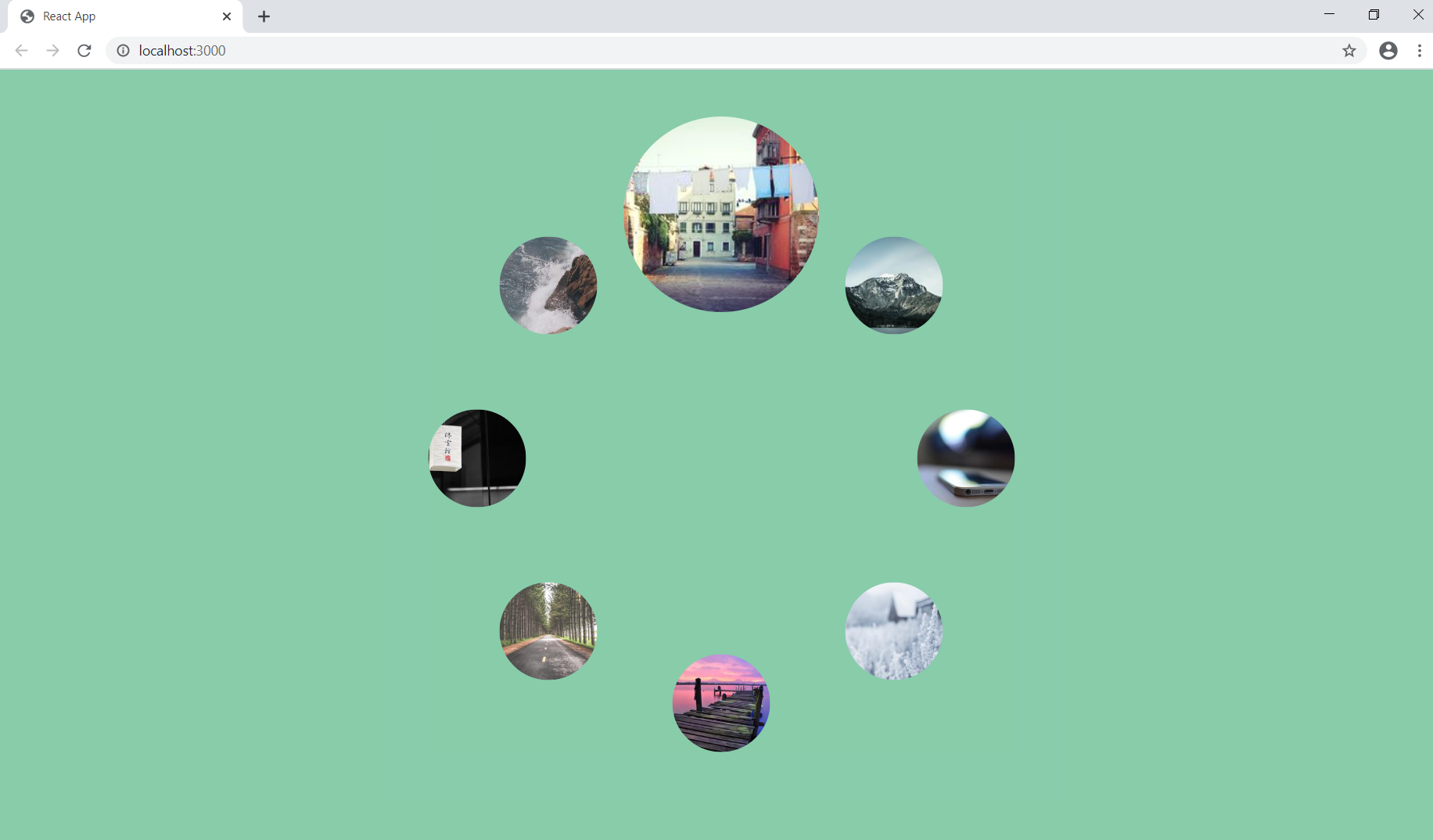
If you would like a more in-depth guide, check out my full video tutorial on YouTube, An Object Is A.
如果您想要更深入的指南,请查看我在YouTube上的完整视频教程“对象就是一个对象” 。
升级到高级ReactJS图像轮转盘(2020 Web设计) (UPGRADE to an ADVANCED ReactJS Image Wheel Carousel (2020 Web Design))
翻译自: https://medium.com/swlh/create-a-reactjs-advanced-wheel-carousel-b88ec253788d
ios轮播图创建