crud程序员
We create a simple APIs in Node js ( Node js Framework, express Framework and using sequelize). In this story, we will create a CRUD (Create, Read, Update, Delete) APIs. If you are new in the Node js and want to learn how to create the basic server and create a basic APIs in Node js, so please read my medium story.
我们在Node js(Node js框架,express框架和使用sequelize)中创建一个简单的API。 在这个故事中,我们将创建一个CRUD(创建,读取,更新,删除)API。 如果您是Node js的新手,并且想学习如何在Node js中创建基本服务器和创建基本API,那么请阅读我的中等故事。
让我们开始构建项目: (Let’s start building the project:-)
Creating a Folder we run the below command in the command prompt where you want to create the project folder.
创建文件夹我们在您要创建项目文件夹的命令提示符下运行以下命令 。
mkdir curd
cd curd
在Node中创建一个项目:- (Create a project in Node:-)
If you are new to Node js and doesn’t know how to create a project in Node.js, For creating a project we run the below command in the command prompt where you want to create the project.
如果您不熟悉Node js并且不知道如何在Node.js中创建项目,那么对于创建项目,我们在您要创建项目的命令提示符下运行以下命令 。
npm init
安装模块。 (Installing the modules.)
$ npm i express sequelize mysql validator body-parser dotenv
Express: Express is modular web framework for node js. Used as a web application framework.
Express: Express是用于节点js的模块化Web框架。 用作Web应用程序框架。
Sequelize : The sequelize is an Object relational mapping used to map an object syntax onto our database schemas.
Sequelize: sequelize是一个对象关系映射,用于将对象语法映射到我们的数据库模式。
Mysql: Mysql is a relational database with the scalability and flexibility that you want with the querying and indexing that you need
Mysql: Mysql 是一个关系数据库,具有所需的可伸缩性和灵活性,可用于所需的查询和索引编制
Validator: This library validates and sanitizes strings only.
验证程序:此库仅验证和清除字符串。
Body-parser: Extract the entire body portion of an incoming request stream and exposes it on req.body.
正文解析器:提取传入请求流的整个正文部分,并将其公开在req.body上。
Dotenv: Dotenv is a zero-dependancy module that loads environment variables from a .env file into process.env .
Dotenv: Dotenv是一个零依赖模块,可将环境变量从.env文件加载到process.env中。
这是我们的package.json的样子: (Here’s how our package.json will look like:)
{
"name": "curd1",
"version": "1.0.0",
"description": "Curd operation with node js using sequelize",
"main": "server.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "nodemon"
},
"author": "Arvind Kumar",
"license": "ISC",
"dependencies": {
"body-parser": "^1.19.0",
"config": "^3.3.1",
"dotenv": "^8.2.0",
"express": "^4.17.1",
"express-validator": "^6.6.1",
"jsonwebtoken": "^8.5.1",
"mysql": "^2.18.1",
"nodemon": "^2.0.4",
"sequelize": "^6.3.4",
"sql": "^0.78.0"
}
}
配置数据库: (Configure the DataBase:-)
We have to create a database in mysql.
我们必须在mysql中创建一个数据库。
Creating a database in your local server. we run the below command in the command prompt. you want to create the database.
在本地服务器中创建数据库。 我们在命令提示符下运行以下命令 。 您要创建数据库。
CREATE DATABASE dbname;
Creating a database table we run the below command in the command prompt. you want to create the database table.
创建数据库表,我们在命令提示符下运行以下命令 。 您要创建数据库表。
USE dbname;CREATE TABLE `users` (
`id` INT(10) NOT NULL AUTO_INCREMENT,
`name` VARCHAR(50) DEFAULT NULL,
`dateofbirth` DATE DEFAULT NULL,
`address` VARCHAR(100) DEFAULT NULL,
'mobile_number' int(10)DEFAULT NULL,
PRIMARY KEY (`id`)
)
We are creating a .env file where we can store all our database information.
我们正在创建一个.env文件,我们可以在其中存储所有数据库信息。
HOST=localhost
DB_USER=(database user name)
DB_PASSWORD=(database password)
DB=(database name)
We have to create a file .app/models/db.js under the root project.
我们必须在根项目下创建一个文件.app / models / db.js。
import the mysql & sequelize module in the file.
在文件中导入mysql&sequelize模块。
const mysql = require("mysql");
const Sequelize = require('sequelize');
require('dotenv').config()
//Sequelize connectionvar connection = new Sequelize(process.env.DB, process.env.DB_USER, process.env.DB_PASSWORD, {
host: process.env.HOST,
port: 3306,
dialect: 'mysql'
});
module.exports = connection;
Now we create index.js file to connect with the database.
现在,我们创建index.js文件以与数据库连接。
const sequelize = require("../models/db.js");
const Sequelize = require('sequelize');
const db = {};
db.Sequelize = Sequelize;
db.sequelize = sequelize;
db.userModel = require("../models/user.model.js")(sequelize, Sequelize);
module.exports = db;
创建模型: (Creating the model:-)
We create a new file inside ./models/user.model.js & import the required modules:
我们在./models/user.model.js中创建一个新文件并导入所需的模块:
module.exports = (sql, Sequelize) => {}
Now we will create the user’s schema (Schema defines the structure of the data)
现在我们将创建用户的架构(Schema定义了数据的结构)
const User= sql.define('tests', {
name: {
type: Sequelize.STRING
},
dateofbirth: {
type: Sequelize.DATE
},
address: {
type: Sequelize.STRING
},
mobile_number: {
type: Sequelize.STRING
}
},
{
timestamps: false
});
return User
创建API: (CREATING API:-)
We are going to route and test the registration API.
我们将路由和测试注册API。
路由请求: (Route the request:-)
The router is used to define the interaction with APIs and used to send and receive the requests from the server.
路由器用于定义与API的交互,并用于发送和接收来自服务器的请求。
We create the file ./routers/user.routes.js
我们创建文件./routers/user.routes.js
Now, we have to route the request post user data.
现在,我们必须路由请求后的用户数据。
app.post("/post-user-details",users.postuserdetails);
we create the function for user data save in database.
我们创建用于将用户数据保存在数据库中的功能。
static postuserdetails = async (req, res) => {
const userDetails =await UserModel.build({
name: req.body.name,
dateofbirth: req.body.dateofbirth,
address: req.body.address,
mobile_number: req.body.mobile_number,
});
await userDetails.save()
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data Save Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to insert data',
errors: error,
status: 400
});
}
Now we have to route the request fetch user data.
现在,我们必须路由请求以获取用户数据。
app.post("/get-user-details",users.getuserdetails);
We create the function for user data find in database.
我们创建用于在数据库中查找用户数据的功能。
static getuserdetails = async (req, res) => {
const userDetails = await UserModel.findAll();
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data find Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to find data',
errors: error,
status: 400
});
}
we have to route the request Update user data.
我们必须路由请求更新用户数据。
app.post("/update-details",users.updatedetails);
We create the function for user data Update in database.
我们在数据库中创建用于用户数据更新的功能。
static updatedetails = async (req, res) => {
const id=req.body.id;
const userDetails =await UserModel.update({
name: req.body.name,
dateofbirth: req.body.dateofbirth,
address: req.body.address,
mobile_number: req.body.mobile_number,
},
{where: {id: req.body.id} });
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data Update Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to update data',
errors: error,
status: 400
});
}
We have to route the request Delete user data.
我们必须路由请求删除用户数据。
app.post("/delete-details",users.deletedetails);
We create the function for user data Delete in database.
我们为数据库中的用户数据创建删除功能。
static deletedetails = async (req, res) => {
const id= req.body.id;
const userDetails = await UserModel.destroy({
where: { id: id }
});
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data Delete Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to Delete data',
errors: error,
status: 400
});
}
创建server.js文件。 (Create server.js file.)
This is the updated server.js file. In this file, we added the routes of REST APIs, active body-parser and sequelize.
这是更新的server.js文件。 在此文件中,我们添加了REST API,活动主体解析器和续集的路由。
const express = require("express");
const bodyParser = require("body-parser");
const Sequelize = require('sequelize');
const app = express();
app.use(bodyParser.json());
require("./app/routes/user.routes.js")(app);
const PORT = process.env.PORT || 7000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}.`);
})
Now, we create a final user.controller.js there contain all function.
现在,我们 创建一个最终的user.controller.js,其中包含所有功能。
require('dotenv').config()
const sql = require("../models/db.js");
const db = require("../models");
const sequelize = require('sequelize');
const UserModel = db.userModel;
const Op = require('sequelize').Op;
class UserController {
static postuserdetails = async (req, res) => {
const userDetails =await UserModel.build({
name: req.body.name,
dateofbirth: req.body.dateofbirth,
address: req.body.address,
mobile_number: req.body.mobile_number,
});
await userDetails.save()
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data Save Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to insert data',
errors: error,
status: 400
});
}
static getuserdetails = async (req, res) => {
const userDetails = await UserModel.findAll();
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data find Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to find data',
errors: error,
status: 400
});
}
static updatedetails = async (req, res) => {
const id=req.body.id;
const userDetails =await UserModel.update({
name: req.body.name,
dateofbirth: req.body.dateofbirth,
address: req.body.address,
mobile_number: req.body.mobile_number,
},
{where: {id: req.body.id} });
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data Update Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to update data',
errors: error,
status: 400
});
}
static deletedetails = async (req, res) => {
const id= req.body.id;
const userDetails = await UserModel.destroy({
where: { id: id }
});
if(!userDetails){
return res.status(200).send({
status: 404,
message: 'No data found'
});
}
res.status(200).send({
status: 200,
message: 'Data Delete Successfully'
});
}
catch(error){
console.log(error)
return res.status(400).send({
message:'Unable to Delete data',
errors: error,
status: 400
});
}
}
module.exports = UserController
全部路由请求: (All Route the request :-)
const users = require("../controllers/user.controller.js");
module.exports = app => {
app.post("/post-user-details",tests.postuserdetails);
app.post("/get-user-details",tests.getuserdetails);
app.post("/update-details",tests.updatedetails);
app.post("/delete-details",tests.deletedetails);
};
API调用: (API Calls :-)
Below are the all of these screenshots of all APIs call, which having REST APIs and result of these APIs.
以下是所有API调用的所有这些屏幕快照,其中包括REST API和这些API的结果。
Create user data in database :
在数据库中创建用户数据:
Now we create save url request.
现在,我们创建保存网址请求。
http://localhost:7000/post-user-details
This screen shot save the data in database.
此屏幕快照将数据保存在数据库中。
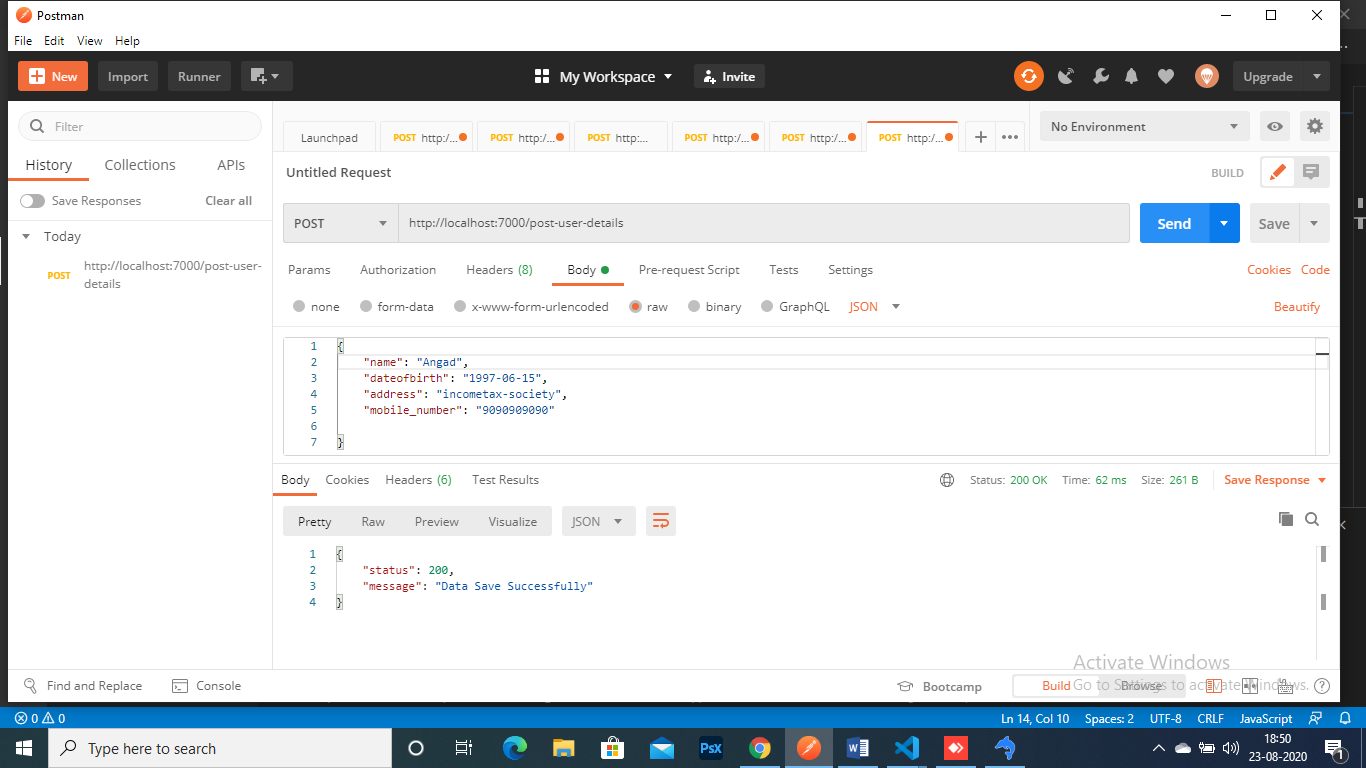
Find user data in database:
在数据库中查找用户数据:
Now we create find url request.
现在,我们创建查找网址请求。
http://localhost:7000/get-user-details
This screen shot find the data in database.
此屏幕快照查找数据库中的数据。
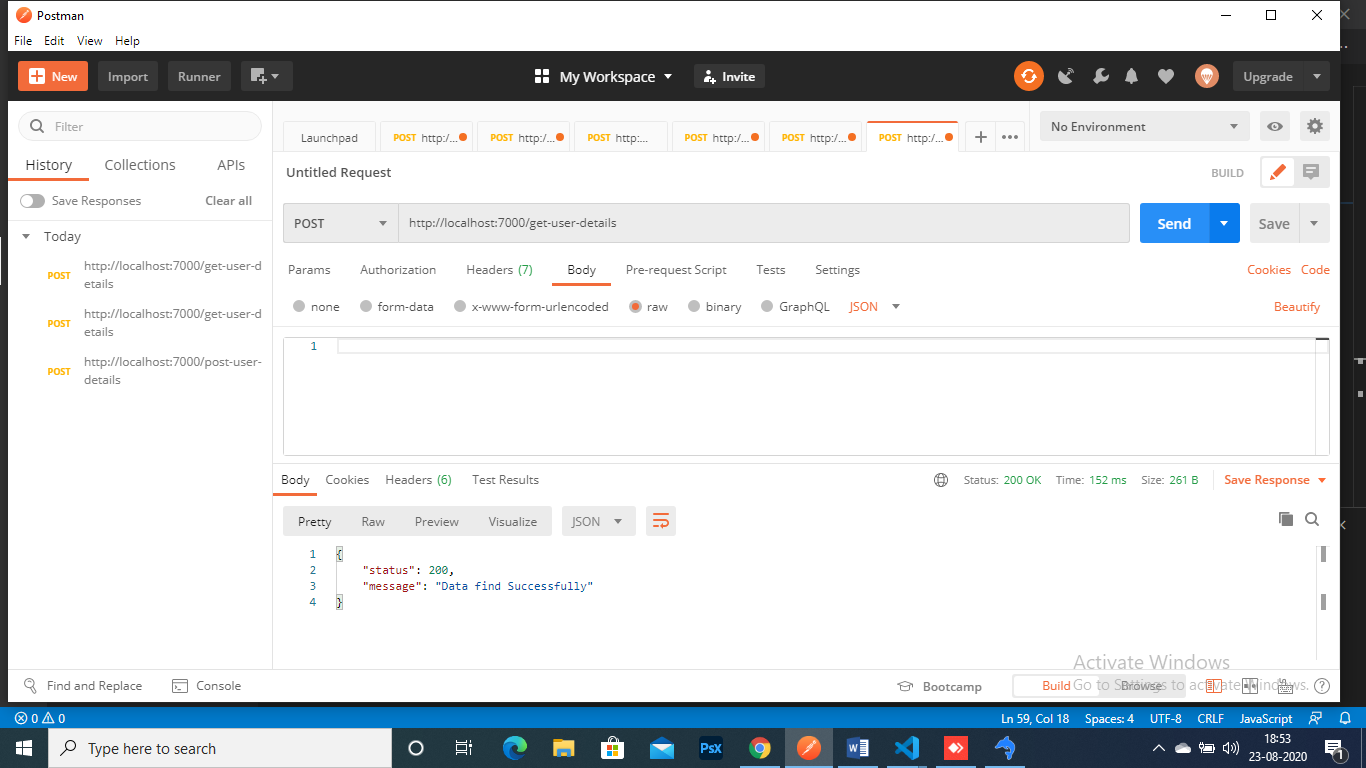
Update user data in database :
更新数据库中的用户数据:
Now we create update url request.
现在,我们创建更新URL请求。
http://localhost:7000/update-details
This screen shot update the data in database.
此屏幕快照更新了数据库中的数据。
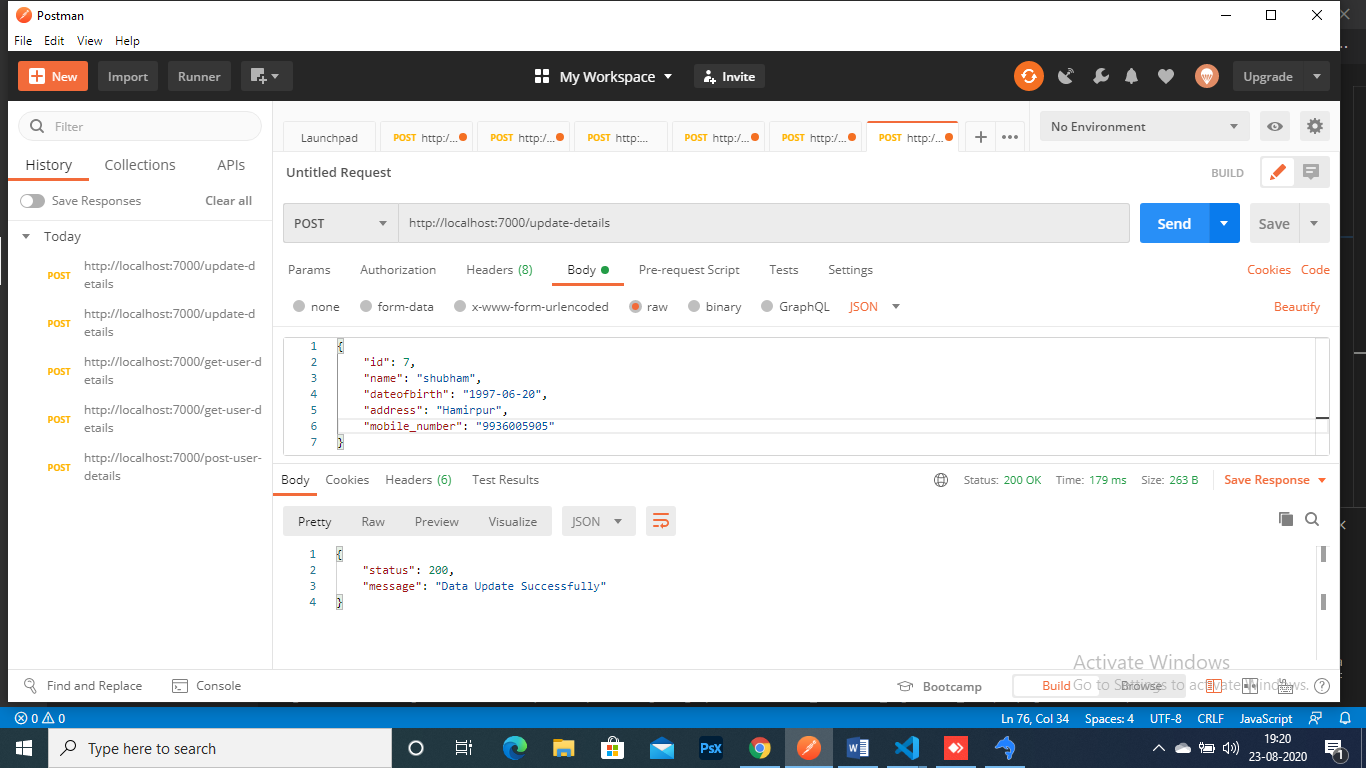
Delete user data in database :
删除数据库中的用户数据:
Now we create delete url request.
现在,我们创建删除网址请求。
http://localhost:7000/delete-details
This screen shot delete the data in database.
此屏幕快照删除了数据库中的数据。
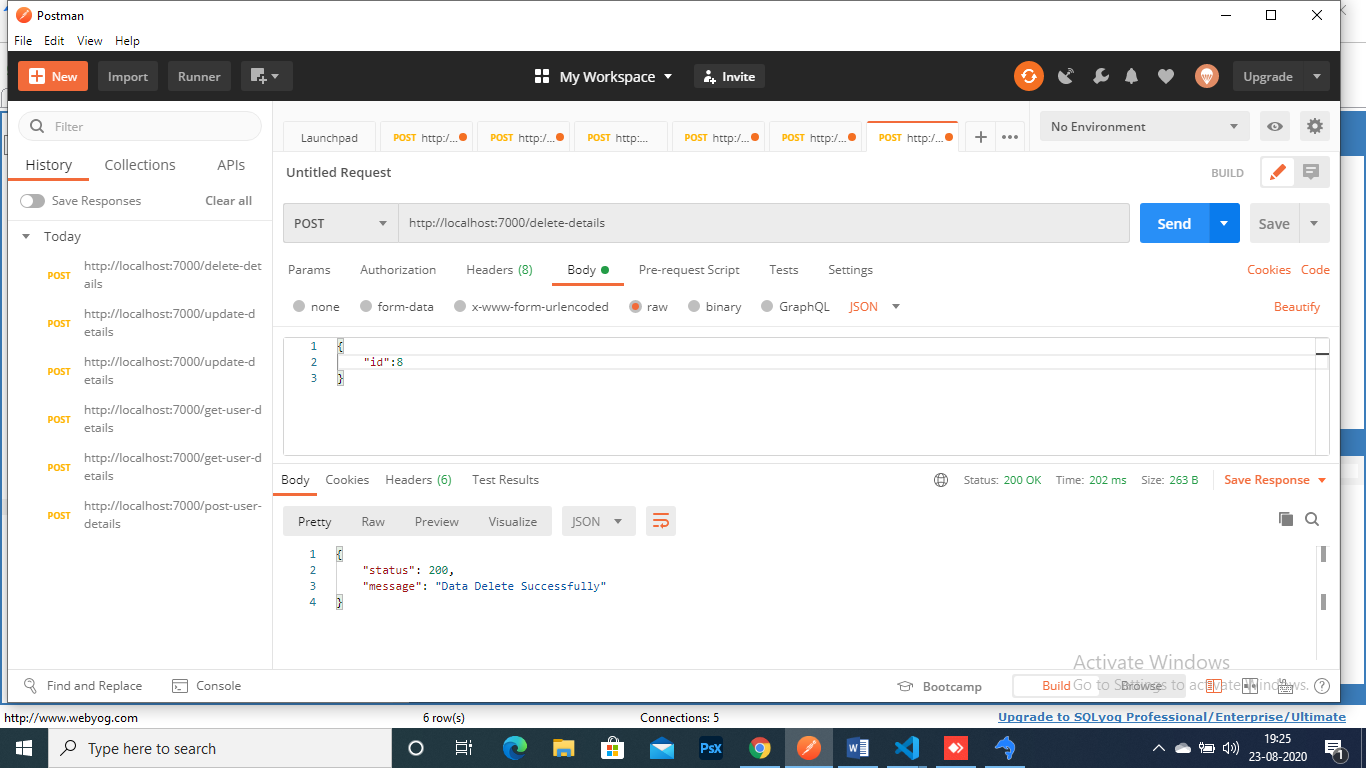
结论:- (Conclusion:-)
This is the best explanatory medium story of how to create REST APIs in Node.js with the help of Sequelize. If you have any doubts, please mail me on arvindgoel31550@gmail.com.
这是有关如何借助Sequelize在Node .js中创建REST API的最佳解释性媒体故事。 如果您有任何疑问,请发送电子邮件至arvindgoel31550 @ gmail.com。
For the ref. of the above code here.
对于参考。 以上代码在这里 。
翻译自: https://medium.com/@arvindgoel_67014/create-crud-application-in-node-js-with-sequelize-a46d21830d48
crud程序员