The map data type is simple to understand and use but the topic can cause confusion due to the wide variety of names used to refer to the same thing. On top of that JavaScript did not have its own map type until ES6 came along we we had to improvise with an ordinary Object.
map数据类型易于理解和使用,但是由于用于引用同一事物的名称种类繁多,因此该主题可能会造成混乱。 最重要的是,直到ES6出现,JavaScript才有了自己的地图类型,我们不得不临时使用一个普通的Object。
In this post I will run through what a map is before using both an Object and an ES6 Map.
在本文中,我将在使用对象和ES6映射之前,先对映射进行遍历。
The data structure generally referred to as a map in JavaScript, especially since the ES6 Map type was introduced, is also known as a dictionary or associative array, and sometimes as a hash table or hash. These last two names are somewhat misleading as they refer to one of the possible underlying implementations rather than the abstract set of behaviours.
数据结构在JavaScript中通常称为映射,尤其是自引入ES6 Map类型以来,也被称为字典或关联数组,有时也称为哈希表或哈希。 最后两个名称在某种程度上具有误导性,因为它们指的是可能的底层实现之一,而不是抽象的行为集。
A map is a collection of key/value pairs, or in other words a collection of names or labels used to refer to the corresponding data values. Within a map each key must be unique. The following operations are the minimum that need to be available although typically implementations provide more.
映射是键/值对的集合,换句话说就是用于引用相应数据值的名称或标签的集合。 在地图中,每个键必须唯一。 以下操作是需要可用的最少操作,尽管通常实现会提供更多操作。
- Insert new pairs 插入新对
- Delete existing pairs 删除现有对
- Editing existing pairs 编辑现有对
- Reading the value of a given key 读取给定键的值
The JavaScript Map type also provides the following functionality:
JavaScript Map类型还提供以下功能:
- Get the number of entries 获取条目数
- Remove all entries in a single operation 一次删除所有条目
- Get the key/value pairs 获取键/值对
- Get the keys 获取钥匙
- Get the values 获取值
- Check whether a key exists 检查密钥是否存在
- A forEach function to run a function on all entries forEach函数可在所有条目上运行函数
该项目 (The Project)
This is a quick and simple project which gives a very brief demo of the traditional Object-based map, and then a more comprehensive demo of the ES6 Map type in action. You can clone or download the source code rom the Github repository.
这是一个快速而简单的项目,它对传统的基于对象的地图进行了非常简短的演示,然后对实际的ES6 Map类型进行了更全面的演示。 您可以从Github存储库克隆或下载源代码。
(The project also includes a small utility file called console.js providing a function to output text to an element in an HTML page in a way which emulates a terminal.)
(该项目还包括一个名为console.js的小型实用程序文件,该文件提供了一种以模拟终端的方式将文本输出到HTML页面中的元素的功能。)
The JavaScript lives in a file called map.js, and this is the first part of that file.
JavaScript 驻留在名为map.js的文件中,这是该文件的第一部分。
As you can see the useObjectAsMap
function is called in window.onload
while the second function, useMap
which we’ll see later, is commented out.
如您所见,在window.onload
调用了useObjectAsMap
函数,而第二个函数useMap
(将在后面看到)被注释掉了。
The useObjectAsMap
function is very straightforward: it just creates an Object
and then adds a few properties to emulate a map data structure. I have then retrieved the object’s keys to print out the size and key/value pairs.
useObjectAsMap
函数非常简单:它只是创建一个Object
,然后添加一些属性来模拟地图数据结构。 然后,我检索了对象的键以打印出大小和键/值对。
There is nothing inherently wrong with this approach and no doubt it has been used millions of times. However, it isn’t perfect and the ES6 Map provides a more optimized implementation.
这种方法没有任何内在的错误,毫无疑问,它已经使用了数百万次。 但是,它并不完美,ES6 Map提供了更优化的实现。
If you open map.htm in your browser you’ll see something like this.
如果在浏览器中打开map.htm ,将会看到类似的内容。
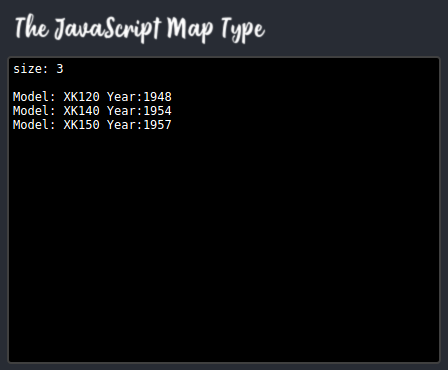
Now let’s look at the useMap
function.
现在让我们看一下useMap
函数。
This code should be self-explanatory but a few additional notes might be helpful.
这段代码应该是不言自明的,但是一些附加说明可能会有所帮助。
Key/value pairs are added with the
set
method.键/值对通过
set
方法添加。Code such as
代码如
jaguars[“XK120”] = 1948; // WRONG!
jaguars[“XK120”] = 1948; // WRONG!
does not cause an error but also does not add the item to the
不会导致错误,但也不会将该项目添加到
Map
.Map
。- I have used strings as keys but perhaps surprisingly you can use anything, including objects and functions. I haven’t had the opportunity to use a function as a key yet but hope to some day. (Software developers have some strange ambitions!) 我使用字符串作为键,但是也许令人惊讶的是您可以使用任何东西,包括对象和函数。 我还没有机会使用函数作为键,但是希望有一天。 (软件开发人员有一些野心!)
The number of items is retrieved using the
size
method.length
will return 0.使用
size
方法检索项目数。length
将返回0。We can check for the presence of a key using
has
.我们可以使用
has
来检查键的存在。You can get the key/value pairs, and therefore iterate the
Map
, using theentries
method.您可以获取键/值对,从而使用
entries
方法迭代Map
。You can also get the keys or values separately using
keys
andvalues
respectively.您也可以分别使用
keys
和values
分别获得键或值。If you have a key you can read the corresponding value with the
get
method.如果有键,则可以使用
get
方法读取相应的值。It is also possible to iterate the entries using
也可以使用来迭代条目
It is also possible to iterate the entries using
for (let [key, value] of jaguars)
也可以使用
for (let [key, value] of jaguars)
来迭代条目- The entries are returned by the iterators in the order they were inserted. 迭代器按插入顺序返回条目。
You can remove a single entry with the
delete
method.您可以使用
delete
方法删除单个条目。You can remove all entries with the
clear
method.您可以使用
clear
方法删除所有条目。Aside from being more intuitive to use than an
Object
, theMap
provides improved performance for frequent set and delete operations.除了比
Object
更直观易用之外,Map
还为频繁的设置和删除操作提供了改进的性能。
If you comment out useObjectAsMap
and uncomment useMap
in window.onload
and then refresh the page you will see the results of the various operations on our Map
object.
如果您在window.onload
注释掉useObjectAsMap
并取消注释useMap
,然后刷新页面,您将在Map
对象上看到各种操作的结果。
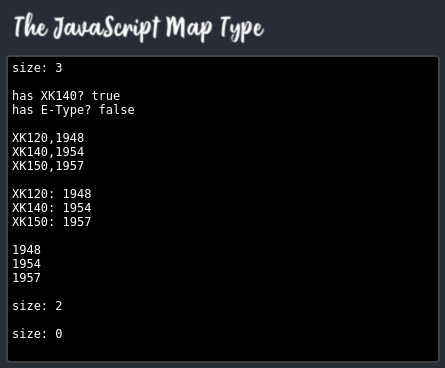
翻译自: https://medium.com/javascript-in-plain-english/the-javascript-map-type-ec7d3e1cff5d