Redux is a light weighted State Management Tool that helps the components in our React App to communicate with each other. The simple concept behind this is that every state of the component is kept in a store that will be global. So that every component can access any state from that store.
Redux是一种轻量级的状态管理工具,可帮助我们的React App中的组件相互通信。 这背后的简单概念是,组件的每个状态都保存在全局存储中。 这样每个组件都可以访问该存储中的任何状态。
In this blog, let’s see a simple example of React-Redux. The logic is to increment and decrement a number on a button click with React-Redux.
在此博客中,我们来看一个简单的React-Redux示例。 逻辑是使用React-Redux单击按钮时增加和减少数字。
1.创建React App和初始设置(1. Creating React App and Initial Setup)
Initially, let’s create a new React App. Also, check if everything is fine with the created project.
首先,让我们创建一个新的React App。 另外,检查创建的项目是否一切正常。
npx create-react-app reactapp
cd reactapp
Now let’s install Redux.
现在,让我们安装Redux。
npm install redux
npm install react-redux
npm start
Now open http://localhost:3000/ and check if the server starts well. Now let’s create our views.
现在打开http:// localhost:3000 /并检查服务器是否正常启动。 现在让我们创建视图。
2.设计我们的观点 (2. Designing Our View)
Here, I am creating a simple React Class Component named Counter.js
with two buttons and a text where one button is for incrementing and the other is for decrementing. The incremented and decremented values will be displayed in the paragraph tag.
在这里,我正在创建一个名为Counter.js
的简单React类组件,它具有两个按钮和一个文本,其中一个按钮用于递增,另一个按钮用于递减。 递增和递减的值将显示在段落标签中。
src/Counter.js
src/Counter.js
import React, { Component } from 'react'export default class Counter extends Component {
render() {
return (
<div>
<h1>Counter</h1>
<button style={{ height: '40px', width: '150px' }}>Increment + </button>
<p>4</p>
<button style={{ height: '40px', width: '150px' }}>Decrement - </button>
</div>
)
}
}
After creating the Counter view, I am including the Counter component in my App.js
file.
创建Counter视图之后,我将Counter组件包含在App.js
文件中。
src/App.js
src/App.js
import React from 'react';
import logo from './logo.svg';
import './App.css';
import Counter from './Counter'function App() {
return (
<div className="App">
<Counter /> </div>
);
}export default App;
Now open your browser and navigate to http://localhost:3000/. You must see something like this.
现在打开浏览器并导航到http:// localhost:3000 / 。 您必须看到类似这样的内容。
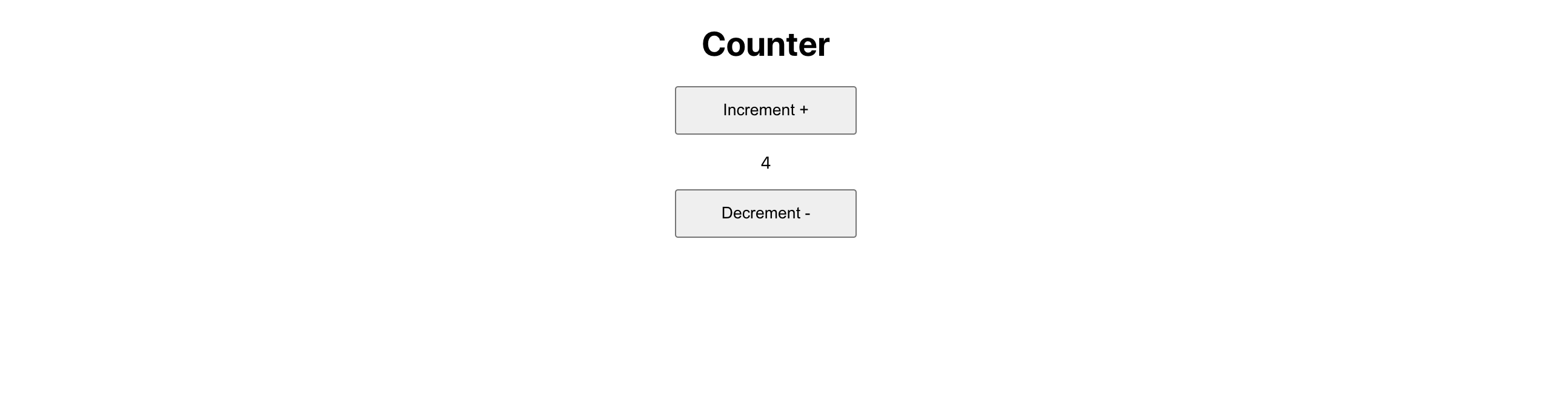
3.将React连接到Redux(3. Connecting React To Redux)
We know that a Redux app has a global store. In order to connect our component to that global store we use something called connect.
Connect helps us to get all the data from the store. Also, it helps us to dispatch actions.
我们知道Redux应用拥有全球商店。 为了将组件连接到该全球商店,我们使用一种叫做connect.
东西connect.
Connect帮助我们从商店中获取所有数据。 此外,它还有助于我们调度动作。
src/Counter.js
src/Counter.js
import React, { Component } from 'react'
import { connect } from 'react-redux'export class Counter extends Component {
render() {
return (
<div>
<h1>Counter</h1> <button style={{ height: '40px', width: '150px' }}>Increment +</button> <p>{this.props.val}</p> <button style={{ height: '40px', width: '150px' }}>Decrement -</button> </div>
)
}
}const mapStateToProps = state => ({
val: state.val
})export default connect(mapStateToProps)(Counter)
MapStateToProps is used for selecting a part of data from the store. We get all the data in the store only through MapStateToProps. It is also referred to as MapState.
MapStateToProps用于从存储中选择部分数据。 我们仅通过MapStateToProps获取存储中的所有数据。 也称为MapState。
We have connected our component to the redux store. But till now we haven’t created any store. So now let’s do it. To create a store, we use createStore
. This expects an argument which is our reducer. This reducer takes two arguments, which are the Initial State and the action. As the name tells, Initial State tells what is the initial value for any state. Here we are performing increment and decrement operations. So our initial state is 0. Once done, wrap the Counter
component by Provider
providing state as pros.
我们已将组件连接到redux存储。 但是到目前为止,我们还没有创建任何商店。 现在开始吧。 要创建商店,我们使用createStore
。 期望得到一个论点,这就是我们的约简。 该化简器有两个参数,分别是初始状态和动作。 顾名思义,Initial State告诉任何状态的初始值是多少。 在这里,我们正在执行递增和递减操作。 因此,我们的初始状态为0。完成后,按Provider
提供状态为Provider
包装Counter
组件。
src/App.js
src/App.js
import React from 'react';
import logo from './logo.svg';
import './App.css';
import Counter from './Counter'
import { createStore } from 'redux'
import { Provider } from 'react-redux'const initialState = {
val: 0
}function reducer(state = initialState, action){
return state;
}const store = createStore(reducer);function App() {
return (
<div className="App">
<Provider store={store}>
<Counter />
</Provider>
</div>
);
}export default App;
Now you must see our initial value being printed between those buttons. But still, the increment and decrement function won’t work since we haven’t dispatched any actions for those buttons. So now let’s do it.
现在,您必须看到在这些按钮之间打印了我们的初始值。 但是,由于我们尚未为这些按钮调度任何动作,因此增量和减量功能仍然无法使用。 现在开始吧。
4.调度动作 (4. Dispatching Actions)
Inside our reducer, create a switch case which performs increment operation if the action is INC
and decrement operation if the action is DEC
.
在我们的减速器内部,创建一个开关盒,如果动作为INC
,则执行递增操作;如果动作为DEC
,则执行递减操作。
src/App.js
src/App.js
function reducer(state = initialState, action){
switch (action.type) {
case "INC":
return {
val: state.val + 1
}
case "DEC":
return {
val: state.val - 1
}
default:
return state;
}
}
Now, create two functions for incrementing and decrementing. Call those functions in the onClick of increment and decrement button respectively.
现在,创建两个递增和递减函数。 分别在递增和递减按钮的onClick中调用这些函数。
Inside the increment function, dispatch action of type INC
and do the same for decrement with action type as DEC.
在增量函数中,调度INC
类型的动作,并对动作类型为DEC.
减量执行相同的操作DEC.
src/Counter.js
src/Counter.js
export class Counter extends Component {increment = () =>{
this.props.dispatch({type: 'INC'})
}decrement = () =>{
this.props.dispatch({type: 'DEC'})
}render() {
return (
<div>
<h1>Counter</h1> <button style={{ height: '40px', width: '150px' }} onClick={this.increment}>Increment +</button> <p>{this.props.val}</p> <button style={{ height: '40px', width: '150px' }} onClick={this.decrement}>Decrement -</button> </div>
)
}
}
Now Incrementing and Decrementing should work. Initially, this might be confusing. Practice this repeatedly with different examples on your own. Only then you will understand how the data flows.
现在,递增和递减应该起作用。 最初,这可能会造成混淆。 自己使用不同的示例反复进行练习。 只有这样,您才能了解数据的流动方式。
In my next blog, I am explaining how to perform CRUD with React-Redux. Stay Connected.
在我的下一个博客中,我将解释如何使用React-Redux执行CRUD。 保持联系。
Feel free to contact me for any queries. Email: sjlouji10@gmail.com Linkedin: https://www.linkedin.com/in/sjlouji/
如有任何疑问,请随时与我联系。 电子邮件:sjlouji10@gmail.com Linkedin: https ://www.linkedin.com/in/sjlouji/
The complete code can be found on my Github: https://github.com/sjlouji/Connecting-React-with-Redux
完整的代码可以在我的Github上找到: https : //github.com/sjlouji/Connecting-React-with-Redux
Happy coding!
编码愉快!