cdh添加节点给定模板
Template Engine is the presentation layer that actually renders our data in HTML format. By default, express provides us a function to send HTML files. Using the function we can send only static pages. If we need to inject any data in an HTML file, we need a template engine. Also, Templating engines split our code into multiple components like header, footer, body, and so on. So we can reuse any component in any layout. This makes our application more scalable and helps us to create an HTML template with minimal code.
模板引擎是表示层,它实际上以HTML格式呈现我们的数据。 默认情况下,express为我们提供了发送HTML文件的功能。 使用该功能,我们只能发送静态页面。 如果需要将任何数据注入HTML文件,则需要模板引擎。 此外,模板引擎将我们的代码分成多个组件,例如页眉,页脚,正文等。 因此,我们可以重用任何布局中的任何组件。 这使我们的应用程序更具可伸缩性,并帮助我们以最少的代码创建HTML模板。
For NodeJS, there are many template engines like Pug
, Haml.js
, EJS
, React
, hbs
, Squirrelly
, and so on. In this blog, I am explaining EJS which is one of the most used template engines.
对于NodeJS,有许多模板引擎,例如Pug
, Haml.js
, EJS
, React
, hbs
, Squirrelly
等。 在此博客中,我将解释EJS,它是最常用的模板引擎之一。
什么是EJS? (What is EJS?)
EJS is one of the template engines used with Node JS to generate HTML markups with plain Javascript. EJS stands for Embedded JavaScript Templates. It can be used both on the client and the server-side.
EJS是与Node JS一起用于使用纯Javascript生成HTML标记的模板引擎之一。 EJS代表嵌入式JavaScript模板。 它可以在客户端和服务器端使用。
1.创建一个Node.js项目(1. Creating a Node.js Project)
Create a new directory and initialize node with the command npm init
.
创建一个新目录并使用命令npm init
初始化节点。
mkdir expresstemplate
cd expresstemplate/
npm init -y
npm i nodemon
Express JS is an open-source web framework for node JS. The below command installs express to our project.
Express JS是用于节点JS的开源Web框架。 下面的命令将express安装到我们的项目中。
npm install express --save
package.json
package.json
"scripts": {
"start": "nodemon index.js",
"test": "echo \"Error: no test specified\" && exit 1"
},
index.js
index.js
const express = require('express')
var app = express()app.get('/', function(req,res){
res.send('Hello world')
})app.listen(8000,function(){
console.log('Listening to PORT 8000')
})
2.呈现HTML文件 (2. Rendering an HTML file)
Initially let’s render a static HTML template. I am creating a simple bootstrap HTML page named as index.html
in the project’s root directory.
首先,让我们呈现一个静态HTML模板。 我正在项目的根目录中创建一个名为index.html
的简单引导HTML页面。
index.html
index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
<title>Template Enginer Node JS</title>
</head>
<body>
<div class='container' style="margin-top: 100px">
<div class="hero-unit">
<h1>Bootstrap</h1>
<p>
<a class="btn btn-primary btn-large">
SignIn
</a>
</p>
</div>
</div> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script> </body>
</html>
Now, create a route /hello
which sends our index.html
file as a response to the client.
现在,创建一个路由/hello
,它将我们的index.html
文件作为响应发送给客户端。
index.js
index.js
const express = require('express')
var app = express()app.get('/', function(req,res){
res.send('Hello world')
})
app.get('/hello', function(req,res){
res.sendFile(__dirname + '/index.html')
})app.listen(8000,function(){
console.log('Listening to PORT 8000')
})
Once done, visit http://localhost:8000/ in the browser. You should see a page like this.
完成后,在浏览器中访问http:// localhost:8000 / 。 您应该会看到一个这样的页面。
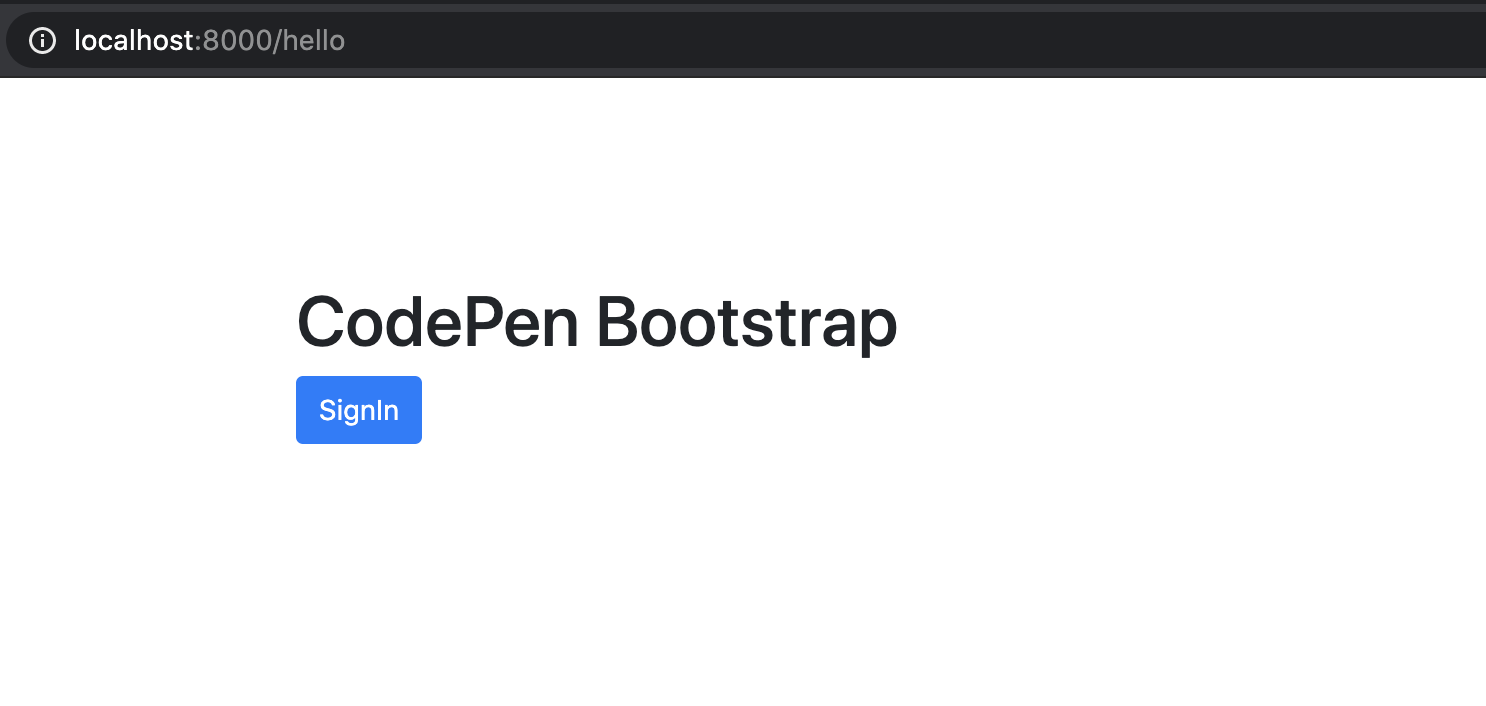
We can use this when we are handling a static file. In the case of dynamic layouts, this will not be suitable since we can't inject any values to the HTML page. This is the reason why we are moving towards EJS or other Template Engines.
我们在处理静态文件时可以使用它。 对于动态布局,这将是不合适的,因为我们无法向HTML页面注入任何值。 这就是我们转向EJS或其他模板引擎的原因。
3.渲染EJS模板 (3. Rendering EJS template)
As said earlier, EJS is one of the most commonly used template Engines that work on both the server and client-side.
如前所述,EJS是在服务器和客户端上均可使用的最常用的模板引擎之一。
基本语法 (Basic Syntax)
a. Unbuffered code for conditionals <% code %>
— This syntax is used to handle conditions. The syntax is commonly used for rendering a value or a template conditionally.
一种。 条件语句的未缓冲代码<% code %>
-此语法用于处理条件。 该语法通常用于有条件地呈现值或模板。
<% if (user) { %> //ToDo: Perform something if user exist
<% } else { %> //ToDo: Perform something if user does not exist
<% } %>
b. Escapes HTML by default <%= code %>
— This syntax is used to render value.
b。 默认情况下转义HTML <%= code %>
-此语法用于呈现值。
<h2><%= user.name %></h2>
c. Unescaped buffering <%- code %>
— This syntax is used when we want to include an external template.
C。 未转义的缓冲<%- code %>
-当我们要包括外部模板时使用此语法。
<%- include('../views/profile')-%>
The installation and setup of EJS is too simple. Use the below command to install EJS for your project.
EJS的安装和设置太简单了。 使用以下命令为您的项目安装EJS。
npm install ejs
After installation, create a folder named views
in the root directory. After creating it, add the below line in the server file. In my case, it is the index.js
file. The below line tells express that my templates are kept under the folder views
.
安装后,在根目录中创建一个名为views
的文件夹。 创建它之后,在服务器文件中添加以下行。 就我而言,它是index.js
文件。 下一行告诉我,我的模板保存在文件夹views
。
index.js
index.js
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'ejs')
Just for the demonstration, I am creating a simple HTML page with an extension ejs
.
为了演示,我正在创建一个扩展名为ejs
的简单HTML页面。
views/hello.ejs
views/hello.ejs
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
<title>Template Enginer Node JS</title>
</head>
<body>
<div class='container' style="margin-top: 100px">
<div class="hero-unit">
<h1>Welcome <%= param %></h1>
<p>
<a class="btn btn-primary btn-large">
SignUp
</a>
</p>
</div>
</div><script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script><script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script><script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script></body>
</html>
In the server file, I am adding a new route, that renders my hello.ejs
file. With that file, I am sending some parameters. This parameter will be received in the name of param
. So by wrapping param
with EJS syntax, it returns me the parameter sent from the route.
在服务器文件中,我添加了一条新路径,该路径呈现了hello.ejs
文件。 有了该文件,我正在发送一些参数。 该参数将以param
的名称接收。 因此,通过使用EJS语法包装param
,它会向我返回从路由发送的参数。
index.js
index.js
const express = require('express')
var app = express()app.get('/', function(req,res){
res.send('Hello world')
})app.get('/hello', function(req,res){
res.sendFile(__dirname + '/index.html')
})app.get('/hello/:name',function(req,res){
res.render('hello',{param: req.params.name})
})app.listen(8000,function(){
console.log('Listening to PORT 8000')
})
When I navigate to http://localhost:8000/hello/louji, It returns me Welcome Louji. If you navigate to http://localhost:8000/hello/medium, it will return Welcome Medium.
当我导航到http:// localhost:8000 / hello / louji时,它向我返回Welcome Louji。 如果导航到http:// localhost:8000 / hello / m edium,它将返回Welcome Medium。
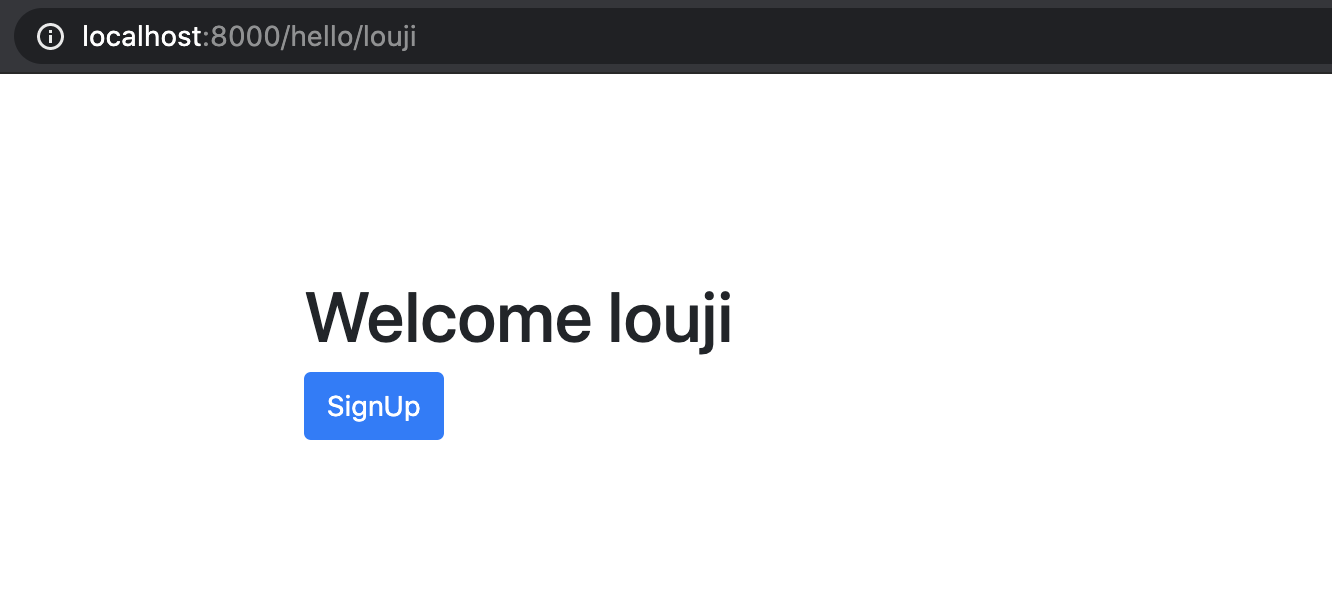
4.包括模板(4. Including Templates)
Apart from injecting values into a template, EJS also helps us to reuse HTML components.
除了将值注入模板之外,EJS还帮助我们重用HTML组件。
Below, I have two files named header.ejs
and footer.ejs
. I am creating both the templates inside the views
folder.
下面,我有两个名为header.ejs
和footer.ejs
文件。 我正在views
文件夹中创建两个模板。
views/header.ejs
views/header.ejs
<html lang="en">
<body>
<header>
<nav class="navbar navbar-expand-md navbar-dark fixed-top bg-dark">
<a class="navbar-brand" href="#">Carousel</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarCollapse" aria-controls="navbarCollapse" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarCollapse">
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link disabled" href="#">Disabled</a>
</li>
</ul>
<form class="form-inline mt-2 mt-md-0">
<input class="form-control mr-sm-2" type="text" placeholder="Search" aria-label="Search">
<button class="btn btn-outline-success my-2 my-sm-0" type="submit">Search</button>
</form>
</div>
</nav>
</header>
</body>
</html>
views/footer.ejs
views/footer.ejs
<html lang="en">
<body>
<footer class="container">
<p class="float-right"><a href="#">Back to top</a></p>
<p>© 2017-2018 Company, Inc. · <a href="#">Privacy</a> · <a href="#">Terms</a></p>
</footer>
</body>
</html>
In my hello.ejs
file, I am using something called include,
Which includes the header.ejs
and footer.ejs
in the hello.ejs
file. Likewise, we can reuse the same header and footer in any layouts.
在我的hello.ejs
文件中,我使用了一个名为include,
东西include,
其中包括hello.ejs
文件中的header.ejs
和footer.ejs
。 同样,我们可以在任何布局中重复使用相同的页眉和页脚。
views/hello.ejs
views/hello.ejs
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
<title>Template works</title>
</head>
<body>
<%- include('../views/header.ejs')-%>
<div class='container' style="margin-top: 100px">
<div class="hero-unit">
<h1>Welcome <%= param %></h1>
<p>
<a class="btn btn-primary btn-large">
SignUp
</a>
</p>
</div>
</div>
<%- include('../views/footer.ejs')-%> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script>
</body>
</html>

Do check my previous blog, where I have explained about Models and Database
Feel free to contact me for any queries. Email: sjlouji10@gmail.com. Linkedin: https://www.linkedin.com/in/sjlouji/
如有任何疑问,请随时与我联系。 电子邮件:sjlouji10@gmail.com。 Linkedin: https : //www.linkedin.com/in/sjlouji/
Complete code on my GitHub:
我的GitHub上的完整代码:
Happy coding!
编码愉快!
翻译自: https://medium.com/javascript-in-plain-english/node-js-template-engine-bb87c9357e3d
cdh添加节点给定模板