jetpack4.1.1
Nowadays, there are many things going on. Likely to be in the mobile industry, with Android, IOS, Flutter, etc. And there is this one more thing called The Jetpack Compose.
如今,发生了很多事情。 可能是移动行业中的Android,IOS,Flutter等。还有另外一件事叫做The Jetpack Compose。
In this episode, I’ll discuss the basics of the Jetpack Compose, starting from the making a basic app. The explanation includes:
在本集中,我将从制作一个基本应用程序开始讨论Jetpack Compose的基础知识。 解释包括:
- What is Jetpack Compose? 什么是Jetpack Compose?
- Version of the IDE IDE版本
- The project’s folder structure 项目的文件夹结构
- Necessary libraries and gradle changes 必要的库和gradle更改
- setContent() setContent()
- MaterialTheme() MaterialTheme()
- Custom Functions 自定义功能
- Text() 文本()
- Composable & Preview keyword 可组合和预览关键字
什么是Jetpack Compose? (What is Jetpack Compose?)
Jetpack Compose is a toolkit for building native Android applications without bothering to design the UI separately by using the XML language. Jetpack Compose uses declarative functions to create all the UI components by calling it in a systematic manner. By doing so, it simplifies and accelerates UI development on Android with less code, powerful tools, and intuitive Kotlin APIs.
Jetpack Compose是一个工具包,用于构建本机Android应用程序,而无需使用XML语言来单独设计UI。 Jetpack Compose使用声明性函数通过系统地调用它来创建所有UI组件。 这样,它可以以更少的代码,功能强大的工具和直观的Kotlin API简化并加速Android上的UI开发。
IDE版本 (Version of the IDE)
The IDE required to build Jetpack Compose applications is Android Studio Canary of version 4.1 or higher.
构建Jetpack Compose应用程序所需的IDE是4.1或更高版本的Android Studio Canary 。
Note: As the Jetpack Compose is still in development phase, thus it should not be used in a production applications.
注意:由于Jetpack Compose仍处于开发阶段,因此不应在生产应用程序中使用。
创建应用 (Creating the app)
Step 1: Click on the create new project option as shown below:
步骤1:单击创建新项目选项,如下所示:

Step 2: Select Empty Compose Activity from the Project Template and then hit next as shown below:
步骤2:从项目模板中选择Empty Compose Activity ,然后单击下一步,如下所示:

Step 3: Then you will move towards the Configure Your Project Screen, where you will have to change the name of the project to the Just Text App, change the Package name, change the save location as you please, leave the rest as it is and hit the finish button to load the project as shown below:
步骤3:然后,您将进入“ 配置项目”屏幕,在该屏幕中,您必须将项目名称更改为Just Text App ,更改包名称 ,随意更改保存位置 ,其余部分保持原样。然后单击完成按钮以加载项目,如下所示:
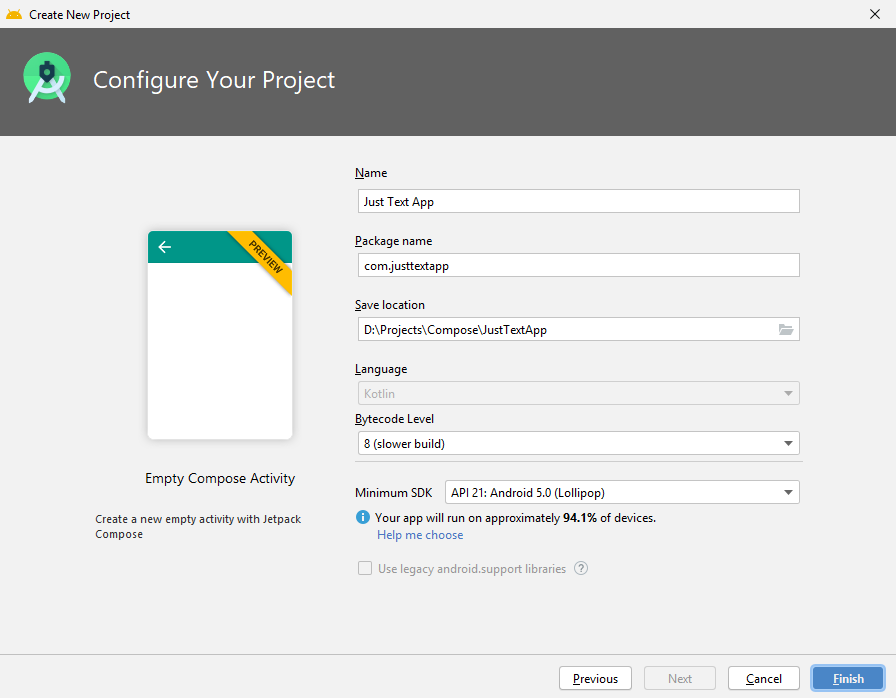
After the loading process completes, the project will look something like this into your MainActivity.kt file as shown below:
加载过程完成后,项目将在您的MainActivity.kt文件中看起来像这样,如下所示:
package com.justtextappimport android.os.Bundleimport androidx.appcompat.app.AppCompatActivityimport androidx.compose.Composableimport androidx.ui.core.Textimport androidx.ui.core.setContentimport androidx.ui.material.MaterialThemeimport androidx.ui.tooling.preview.Previewclass MainActivity : AppCompatActivity() {override fun onCreate(savedInstanceState: Bundle?) {super.onCreate(savedInstanceState)setContent {MaterialTheme {Greeting("Android")}
}}
}
@Composablefun Greeting(name: String) {Text(text = "Hello $name!")
}
@Preview
@Composablefun DefaultPreview() {MaterialTheme {Greeting("Android")}}
As you can see above, the code which I’ve mentioned looks very similar to that of Kotlin. But we will discuss about the code later.
正如您在上面看到的,我提到的代码与Kotlin的代码非常相似。 但是我们稍后将讨论代码。
项目的文件夹结构 (The project’s folder structure)
When to open up the project panel from the left-hand side of your IDE, collapse the res folder and you will come to know that there is a layout folder in it, which we used to see in our every Android application project. That clears out that there is no requirement for any xml file to design the UI of the app. The UI and the Logic both will be solely created into MainActivity.kt file only by using the Compose’s functions.
从IDE左侧打开项目面板时,请折叠资源 文件夹,您将知道其中有一个布局文件夹,我们曾经在每个Android应用程序项目中都看到过该文件夹。 这清楚了,不需要任何xml文件来设计应用程序的UI。 仅通过使用Compose的功能,UI和逻辑都将单独创建到MainActivity.kt文件中。
必要的库和gradle更改 (Necessary libraries and gradle changes)
Open up your project level build.gradle file and check for the libraries and its versions. If it is as same as shown below or higher than that then there is no need to make any changes over here in this file.
打开您的项目级别的build.gradle文件,并检查库及其版本。 如果与下面显示的相同或更高,则无需在此文件中进行任何更改。
// Top-level build file where you can add configuration options common to all sub-projects/modules.buildscript {ext.kotlin_version = "1.3.71"repositories {google()
jcenter()}dependencies {classpath "com.android.tools.build:gradle:4.1.0-alpha05"classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files}
}allprojects {repositories {google()
jcenter()}
}task clean(type: Delete) {delete rootProject.buildDir}
Then go to your app-level build.gradle file which looks like as shown below:
然后转到您的应用程序级别的build.gradle文件,如下所示:
plugins {id 'com.android.application'id 'kotlin-android'id 'kotlin-android-extensions'
}android {compileSdkVersion 29
buildToolsVersion "29.0.3"defaultConfig {applicationId "com.justtextapp"minSdkVersion 21
targetSdkVersion 29
versionCode 1
versionName "1.0"testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}buildTypes {release {minifyEnabled falseproguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}compileOptions {sourceCompatibility JavaVersion.VERSION_1_8targetCompatibility JavaVersion.VERSION_1_8
}kotlinOptions {jvmTarget = '1.8'
}buildFeatures {compose true
}
}dependencies {implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version"implementation 'androidx.core:core-ktx:1.2.0'implementation 'androidx.appcompat:appcompat:1.1.0'implementation 'com.google.android.material:material:1.1.0'implementation 'androidx.ui:ui-framework:0.1.0-dev03'implementation 'androidx.ui:ui-layout:0.1.0-dev03'implementation 'androidx.ui:ui-material:0.1.0-dev03'implementation 'androidx.ui:ui-tooling:0.1.0-dev03'testImplementation 'junit:junit:4.13'androidTestImplementation 'androidx.test.ext:junit:1.1.1'androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0'
}
Now here in the dependencies block, we need to make a change in the version of our framework, layout, material and tooling dependencies. Increase their version to 0.1.0-dev08 as shown below.
现在,在依赖关系块中,我们需要更改框架,布局,材质和工具依赖关系的版本。 如下所示将其版本增加到0.1.0-dev08 。
dependencies {implementation 'androidx.ui:ui-framework:0.1.0-dev08'implementation 'androidx.ui:ui-layout:0.1.0-dev08'implementation 'androidx.ui:ui-material:0.1.0-dev08'implementation 'androidx.ui:ui-tooling:0.1.0-dev08'
}
Also, check this line into gradle-wrapper.properties file as shown below:
另外,将此行检入gradle-wrapper.properties文件,如下所示:
distributionUrl=https\://services.gradle.org/distributions/gradle-6.3-all.zip
If the version is as same as the above or higher then it's fine, but if it is lower than there is a need for an upgrade.
如果版本与上面的版本相同或更高,则可以,但是如果版本低于需要升级的版本。
Let's move towards the MainActivity.kt file.
让我们转到MainActivity.kt文件。
setContent()函数/块 (setContent() function/block)
This function/block is used to define the layout of the activity. It is meant to replace the .xml file which we used to set the layout file into our activity file i.e. like this setContent(R.layout.xml_file_name)
此功能/块用于定义活动的布局。 它旨在将用于设置布局文件的.xml文件替换为我们的活动文件,即类似setContent(R.layout.xml_file_name)
setContent {
// Code here...
}
MaterialTheme()函数/块 (MaterialTheme() function/block)
This component defines the styling principles from the Material design specification. It includes Material components, as it defines key values such as base colors and typography. Material components such as Button and Checkbox use this definition to set default values.
该组件根据材料设计规范定义样式原则。 它包括材料成分,因为它定义了诸如基色和版式之类的关键值。 诸如“按钮”和“复选框”之类的材质组件使用此定义来设置默认值。
MaterialTheme {
//Code here...}
自定义功能 (Custom Functions)
The custom functions are those which are created by the developer. The Jetpack Compose gives us the authority to create our own functions. So, here as we see the Greeting function which is the default code of the Jetpack Compose’s template. We will change the name of that function to make it our own.
自定义函数是由开发人员创建的。 Jetpack Compose授权我们创建自己的功能。 因此,在这里我们看到了Greeting函数,它是Jetpack Compose模板的默认代码。 我们将更改该函数的名称以使其成为我们自己的函数。
fun Greeting(name: String) {//Code here...
}
Change the name of the function from Greeting() to TextLayout() holding a parameter of name as of String data type.
将函数名称从Greeting()更改为TextLayout(),其中保留name参数为String数据类型。
fun TextLayout(name: String) {
//Code here...
}
Similarly, make the changes into our MaterialTheme{} block too as it holds this function into it to load the UI and render it on the screen. Also, change the value of the TextLayout() function as shown below:
同样,也对我们的MaterialTheme {}块进行更改,因为该块将其包含在其中,以加载UI并将其呈现在屏幕上。 另外,如下所示更改TextLayout()函数的值:
MaterialTheme {TextLayout("Jetpack. This is Just Text App")}
Text()函数 (Text() function)
This function is used to display text and thus provides accessibility information. It uses a default style as the currentTextStyle defined by the theme. In addition, if no text color is manually specified in style then the text color will be contentColor.
此功能用于显示文本,从而提供辅助功能信息。 它使用默认样式作为主题定义的currentTextStyle 。 此外,如果未在样式中手动指定文本颜色,则文本颜色将为contentColor 。
Text(text = "Hello $name!")
The name word used in the double quotation along with the dollar symbol is used for concatenating the string.
双引号中使用的名称单词以及美元符号用于连接字符串。
Also, change the import library of the text into the MainActivity.kt file.
另外,将文本的导入库更改为MainActivity.kt文件。
Change from:
更改自:
import androidx.ui.core.Text
To this:
对此:
import androidx.ui.foundation.Text
This will resolve the error into the MainActivity.kt file.
这会将错误解决到MainActivity.kt文件中。
Now, with another function, change the name of the function here too with the same value into the parameter.
现在,使用另一个函数,在此处也将具有相同值的函数名称更改为参数。
The DefaultPreview() function is used to render the UI of this app into the Android Studio only. It holds the MaterialTheme’s block to render the UI as same as it will render on that of the emulator or a physical device. It uses the Preview and Composable annotations to make the rendering happen into the editor.
DefaultPreview()函数用于仅将此应用程序的UI渲染到Android Studio中。 它包含MaterialTheme的块,以呈现与在模拟器或物理设备上呈现的UI相同的UI。 它使用Preview和Composable批注使渲染发生在编辑器中。
fun DefaultPreview() {MaterialTheme {TextLayout("Jetpack. This is Just Text App")}}
可组合和预览关键字 (Composable & Preview keyword)
Composable: This keyword is used by applying to a function or lambda to indicate that the function/lambda can be used as part of a composition to describe a transformation from application data into a tree or hierarchy.Annotating a function or expression with Composable changes the type of that function or expression.
Composabl E:此关键字用于通过施加到功能或λ,以指示该功能/λ可以作为组合物的一部分来描述从应用程序数据转换成树状或hierarchy.Annotating与可组合的变化的功能或表达该函数或表达式的类型。
Composable functions can only ever be called from within another Composable function. A useful mental model for Composable functions is that an implicit “composable context” is passed into a Composable function, and is done so implicitly when it is called from within another Composable function. This “context” can be used to store information from previous executions of the function that happened at the same logical point of the tree.
可组合的功能永远只能从另一个组合的函数中调用。 对于可组合函数有用的思维模型是将隐式“可组合上下文”传递到可组合函数中,并且在从另一个可组合函数中调用它时会隐式完成。 该“上下文”可用于存储在树的同一逻辑点处发生的功能的先前执行获得的信息。
Preview: This keyword is applied to @Composable methods with no parameters to render the UI into the Android Studio.
预览:此关键字应用于无参数的@Composable方法,以将UI渲染到Android Studio中。
That’s it. Finally, run the code into your respective emulators or on an Android device.
而已。 最后,将代码运行到您各自的仿真器中或在Android设备上。
Note: For the source code of this project, you can visit my GitHub account and you will find a bunch of little projects. But, whoever wants to refer only this project then search for the same named package in my repository. The link is given below:
注意 :有关该项目的源代码,您可以访问我的GitHub帐户,您会发现很多小项目。 但是,只要想只引用此项目的人,然后在我的存储库中搜索相同的命名包。 链接如下:
Do contribute if you think there is a need for any betterment or issue into the code and do provide a response below in the comment section, as I would like to improve myself further. Thank you in advance. Happy coding!
如果您认为需要对代码进行任何改进或改进,请做出贡献,并在下面的注释部分中提供答复,因为我想进一步提高自己。 先感谢您。 编码愉快!
翻译自: https://medium.com/kotlin-mumbai/jetpack-compose-series-1-basics-ep-1-just-text-app-4acb42f5d865
jetpack4.1.1