canopen 对象字典
Many languages have their own dictionaries, these are “general-purpose data structures for storing a group of objects” (Wikipedia, 2019). In the python dictionary, some of the methods return view objects. These view objects are dynamic view objects, meaning that when the dictionary changes, the view reflects these changes. Dict view objects also support set-like operations, membership tests, and more built-in functions. This article will explore dictionary view objects in greater detail with the aim of helping you to understand the basic concepts at play here, as well as share some examples of how you may encounter them as you continue on your coding journey.
许多语言都有自己的字典,它们是“用于存储一组对象的通用数据结构”( Wikipedia,2019年 )。 在python字典中,某些方法返回视图对象。 这些视图对象是动态视图对象 ,这意味着当字典更改时,视图将反映这些更改。 Dict视图对象还支持类似集合的操作,成员资格测试以及更多内置函数。 本文将更详细地探索字典视图对象,以帮助您理解此处的基本概念,并分享一些示例,说明您在继续进行编码过程中可能会遇到的问题。
这个故事中涉及的主题。 (Topics covered in this story.)
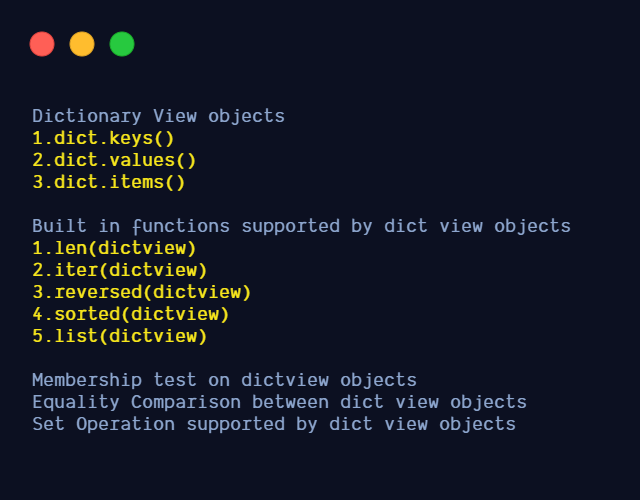
字典视图对象 (Dictionary View Objects)
The objects returned by dict.keys(), dict.values(), and dict.items() are view objects. They provide a dynamic view of the dictionary’s entries, which means that when the dictionary changes, the view reflects these changes.
dict.keys(),dict.values()和dict.items()返回的对象是视图对象。 它们提供了字典条目的动态视图,这意味着当字典更改时,该视图将反映这些更改。
dict.keys() (dict.keys())
dict.keys() return a new view of dictionary keys. If we update the dictionary then the dictionary view objects are also dynamically updated.
dict.keys()返回字典键的新视图 。 如果我们更新字典,那么字典视图对象也会动态更新。
d = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
k = d.keys()
print(k)
# Output:dict_keys(['a', 'b', 'c', 'd'])
print(type(k))
# Output:<class 'dict_keys'>
# updating the dictionary
d['e'] = 5
print(d)
# dynamic view object is updated automatically
print(k)
# Output:dict_keys(['a', 'b', 'c', 'd', 'e'])
dict.values() (dict.values())
dict.values() return a new view of the dictionary’s values. If we update the dictionary then the dictionary view objects are also dynamically updated.
dict.values()返回字典值的新视图 。 如果我们更新字典,那么字典视图对象也会动态更新。
d = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
v = d.values()
print(v)
# Output:dict_values([1, 2, 3, 4])
print(type(v))
# Output:<class 'dict_values'>
# updating the dictionary
d['e'] = 5
print(d)#Output:{'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5}
# dynamic view object is updated automatically
print(v)
# Output:dict_values([1, 2, 3, 4, 5])
dict.items() (dict.items())
These return a new view of the dictionary’s items ((key, value)
pairs). If we update the dictionary then the dictionary view objects are also dynamically updated.
它们返回字典项 ( (key, value)
对)的新视图 。 如果我们更新字典,那么字典视图对象也会动态更新。
d = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
i = d.items()
print(i)
# Output:dict_items([('a', 1), ('b', 2), ('c', 3), ('d', 4)])
print(type(i))
# Output:<class 'dict_items'>
# updating the dictionary
d['e'] = 5
print(d)#Output:{'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5}
# dynamic view object is updated automatically
print(i)
# Output:dict_items([('a', 1), ('b', 2), ('c', 3), ('d', 4), ('e', 5)])
字典视图对象支持的内置函数: (Built-in functions supported by dictionary view objects:)
- len(dictview) len(dictview)
- iter(dictview) iter(dictview)
- reversed(dictview) 逆转(dictview)
- sorted(dictview) 排序(dictview)
- list(dictview) 列表(dictview)
1.len(dictview)Returns the number of entries in the dictionary.
1.len(dictview)返回字典中的条目数 。
d = {'g': 1, 'b': 2, 'c': 3, 'd': 4}
k=d.keys()
print (len(k))#Output:4
v=d.values()
print (len(v))#Output:4
i=d.items()
print (len(i))#Output:4
2. iter(dictview)Returns an iterator over the keys, values, or items (represented as tuples of (key, value)
) in the dictionary. Keys, values, and items are iterated over insertion order.
2. iter(dictview)返回在字典中的键,值或项目(表示为(key, value)
元组)上的迭代器。 键,值和项在插入顺序上进行迭代。
iter(dict)
will return an iterator over keys alone.
iter(dict)
将仅通过键返回一个迭代器。
Example 1. Iterating over keys- Converting iterator to list using list() constructor:
示例1.遍历键-使用list()构造函数将迭代器转换为列表:
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
k=d.keys()
print (iter(d))#Output:<dict_keyiterator object at 0x011DE050>
#Converting iterator to listprint (list(iter(d)))#Output:['g', 'b', 'c', 'd']
Example 2. Iterating over the values- Accessing elements in iterator using for loop:
示例2.遍历值-使用for循环访问迭代器中的元素:
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
v=d.values()
print (iter(v))#Output:<dict_valueiterator object at 0x011DE050>
#Accessing elements in iterator using for loopfor i in iter(v):
print (i)'''
Output:
1
5
3
4
'''
Example 3. Iterating over the items. Accessing the elements in the iterator using the next() function:
示例3.对项目进行迭代。 使用next()函数访问迭代器中的元素:
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
i=d.items()
i1=iter(i)#Output:<dict_valueiterator object at 0x011DE050>
#Accessing elements in itertaor using next() fucntionprint (next(i1))#Output:('g', 1)print (next(i1))#Output:('b', 5)print (next(i1))#Output:('c', 3)
Example 4. Iterating over the dictionary (It will iterate over keys alone):
示例4.遍历字典(它将仅遍历键):
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
print (iter(d))#Output:<dict_keyiterator object at 0x011DE050>print (list(iter(d)))#Output:['g', 'b', 'c', 'd']
Example 5. Create a list of (value, key) pairs from the dictionary:
例子5.从字典创建一个(值,键)对的列表:
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
z=zip(d.values(),d.keys())
print (z)#Output:<zip object at 0x03199528>print (list(z))#Output:[(1, 'g'), (5, 'b'), (3, 'c'), (4, 'd')]
Example 6. Convert a dictionary to value: key pair:
例子6.将字典转换为值:密钥对:
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
d1={v:k for v in d.values() for k in d.keys()}
print (d1)#Output:{1: 'd', 5: 'd', 3: 'd', 4: 'd'}
NB: Iterating views while adding or deleting entries in the dictionary may raise a RuntimeError or fail to iterate over all entries.
注意:在字典中添加或删除条目时迭代视图可能会引发RuntimeError或无法迭代所有条目。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}for i in iter(d.keys()):
if i=='c':
del d[i]
print (i)#Output:RuntimeError: dictionary changed size during iteration
3. reversed(dictview)
3.反转(dictview)
Returns a reverse iterator over the keys, values, or items of the dictionary. The view will be iterated in reverse order of the insertion. Changed in version 3.8: Dictionary views are now reversible.
返回字典的键,值或项目的反向迭代器 。 该视图将以与插入相反的顺序进行迭代。 在3.8版中进行了更改:词典视图现在是可逆的。
reversed(dict)
-Returns a reverse iterator over keys alone in the dictionary
reversed(dict)
-仅在字典中的键上返回反向迭代器
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
k=reversed(d.keys())
print (list(k))#Output:['d', 'c', 'b', 'g']
v=reversed(d.values())
print (list(v))#Output:[4, 3, 5, 1]
i=reversed(d.items())
print (list(i))#Output:[('d', 4), ('c', 3), ('b', 5), ('g', 1)]
#return a reversed iterator of keys alone
d1=reversed(d)
print (list(d1))#Output:['d', 'c', 'b', 'g']
4.sorted(dictview)
4.sorted(dictview)
Return a sorted list over the keys, values, or items of the dictionary.
在字典的键,值或项目上返回排序的列表 。
sorted(dict)
-Return a sorted list of keys alone in the dictionary.
sorted(dict)
仅在字典中返回键的排序列表。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
print (sorted(d.keys()))#Output:['b', 'c', 'd', 'g']
print (sorted(d.values()))#Output:[1, 3, 4, 5]
print (sorted(d.items()))#Output:[('b', 5), ('c', 3), ('d', 4), ('g', 1)]
d1=print (sorted(d))#Output:['b', 'c', 'd', 'g']
5. list(dictview)
5.列表(dictview)
Return a list over the keys, values, or items of the dictionary.
返回一个LIS牛逼的键,值或字典的项目。
list(dict)
-Return a list of keys alone in the dictionary.
list(dict)
仅在字典中返回键列表。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
print (list(d.keys()))#Output:['g', 'b', 'c', 'd']print (list(d.values()))#Output:[1, 5, 3, 4]print (list(d.items()))#Output:[('g', 1), ('b', 5), ('c', 3), ('d', 4)]d1=print (list(d))#Output:['g', 'b', 'c', 'd']
会员资格测试: (Membership Test:)
x in dictview
x in dictview
Return True
if x is in the underlying dictionary’s keys, values or items (in the latter case, x should be a (key, value)
tuple).
如果x在基础字典的键,值或项目中(在后一种情况下, x应该是(key, value)
元组) (key, value)
则返回True
。
x in dict
x in dict
Returns True
if x is in the dictionary's keys.
如果x在字典的键中,则返回True
。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
print ('a' in d.keys())#Output:Falseprint ('b' in d.keys())#Output:Trueprint (1 in d.values())#Output:Trueprint (2 in d.values())#Output:Falseprint (('g',1) in d.items())#Output:Trueprint (1 in d)#Output:Falseprint ('b' in d)#Output:True
dictview对象之间的相等比较。 (Equality Comparison between the dictview objects.)
An equality comparison between one dict.values()
view and another will always return False
. This also applies when comparing dict.values()
to itself.
一个dict.values()
视图和另一个dict.values()
视图之间的相等比较将始终返回False
。 在将dict.values()
与自身进行比较时,这也适用。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
d1= {'g': 1, 'b': 5, 'c': 3, 'd': 4}
v=d.values()
v1=d.values()
print (v)#Output:dict_values([1, 5, 3, 4])print (v1)#Output:dict_values([1, 5, 3, 4])print (v==v1)#Output:False
An equality comparison between one dict.keys()
view and another will return True
if both contain the same elements (regardless of ordering). Otherwise, it returns False
.
如果一个dict.keys()
视图与另一个dict.keys()
视图之间的相等性比较如果都包含相同的元素(无论顺序如何dict.keys()
,则将返回True
。 否则,它返回False
。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
d1= {'b':5,'g':1, 'c': 3, 'd': 4}
k=d.keys()
k1=d1.keys()#Comparing dictionaries having same keys(different order)print (k==k1)#Output:Trued2={'a':3}#Comparing dictionaries having different keysprint (d.keys()==d2.keys())#Output:False
An equality comparison between one dict.items()
view and another will return True
if both contain the same elements(regardless of ordering). Otherwise, it returns False
.
如果一个dict.items()
视图与另一个dict.items()
视图之间的相等性比较如果都包含相同的元素,则返回True
(无论顺序如何)。 否则,它返回False
。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
d1= {'b':5,'g':1, 'c': 3, 'd': 4}
k=d.items()
k1=d1.items()#Comparing dictionaries having same items(different order)print (k==k1)#Output:Trued2={'a':3}#Comparing dictionaries having different itemsprint (d.items()==d2.items())#Output:False
Dictionaries compare equal if and only if they have the same (key, value)
pairs (regardless of ordering).
当且仅当字典具有相同的(key, value)
对时(key, value)
字典才会比较相等(无论顺序如何)。
d = {'g': 1, 'b': 5, 'c': 3, 'd': 4}
d1= {'b':5,'g':1 ,'c': 3, 'd': 4}
print (d==d1)#Output:True
设置字典视图对象支持的操作 (Set Operation supported by dict view objects)
The set doesn’t contain duplicate elements. Similarly, the dictionary also contains unique keys only. So keys views are set-like since their entries are unique and hashable.
该集合不包含重复的元素。 同样,字典也仅包含唯一键。 因此, 键视图 就像集合一样,因为它们的条目是唯一且可哈希的。
If all values are hashable, so that (key, value)
pairs are unique and hashable, then the item’s view is also set-like. Values views are not treated as set-like since the entries are generally not unique.
如果所有值都是可哈希的,因此(key, value)
对是唯一且可哈希的,则该项的视图也将像集合一样。 由于条目通常不是唯一的,因此值视图不会被视为类似集合的视图。
For set-like views, all of the operations defined for the abstract base class collections.abc.Set
are available (for example, ==
, <
, or ^
).
对于类似集合的视图,为抽象基类collections.abc.Set
定义的所有操作均可用(例如==
, <
或^
)。
Refer to my story for all set operations
Example 1. Set Operations supported in keys views:
示例1.在键视图中设置支持的操作:
d = {'f': 1, 'b': 5, 'c': 3, 'd': 4}
d1= {'b':5,'e':1, 'a': 3}
k=d.keys()
k1=d1.keys()
print (k > k1)#Output:Falseprint (k & k1) #Output:{'b'}print (k ^ k1) #Output:{'f', 'e', 'a', 'd', 'c'}print (k | k1) #Output:{'d', 'b', 'e', 'c', 'f', 'a'}
Example 2. Performing set operations on values views will raise TypeError:
示例2.在值视图上执行设置操作将引发TypeError:
d = {'f': 1, 'b': 5, 'c': 3, 'd': 4}
d1= {'b':5,'e':1, 'a': 3}
v=d.values()
v1=d1.values()
print (v > v1)#Output:TypeError: '>' not supported between instances of 'dict_values' and 'dict_values'
Example 3. Performing set operations on item’s views which contain hashable values:
示例3.对包含可哈希值的项目视图执行设置操作:
All of Python’s immutable built-in objects are hashable. Mutable containers (such as lists or dictionaries) are not hashable. If all values are hashable, so that (key, value)
pairs are unique and hashable, then the items view is also set-like. In this example, the values mentioned are hashable. So, we can perform set operations on items view:
Python的所有不可变内置对象都是可哈希的。 可变容器(例如列表或字典)不可散列。 如果所有值都是可哈希的,那么(key, value)
对是唯一且可哈希的,那么items视图也将是set-like的 。 在此示例中,提到的值是可哈希的。 因此,我们可以在项目视图上执行设置操作:
d = {'f': 1,'a':2,'c':3}
d1 = {'f': 1,'c':3}
i=d.items()
i1=d1.items()
print (i > i1)#Output:Trueprint (i & i1) #Output:{('f', 1), ('c', 3)}print (i ^ i1) #Output:{('a', 2)}print (i | i1) #Output:{('f', 1), ('c', 3), ('a', 2)}
Example 4. Performing set operations on item’s views that contain not hashable values:
示例4.在包含不可哈希值的项目视图上执行设置操作:
d = {'f': 1,'a':2,'c':3}
d1 = {'f': [1,2],'c':3}
i=d.items()
i1=d1.items()
print (i > i1)#Output:TypeError: unhashable type: 'list'
注意:使用的Python版本是3.8.1 。 (Note: Python version used is 3.8.1.)
结论: (Conclusion:)
In this article we have covered a lot of concepts including that dictionary view objects (dict.keys(), dict.values(), and dict.items()) are dynamic view objects, that when dictionary entries change, view objects are also updated dynamically, and that built-in functions and membership tests on dict will work on keys alone, whereas on dictview they will work on keys, values, and items. Keys views and items view with hashable values are set like and we can perform set operations on that. Set operations are not supported in values-view. I hope that you have found this article helpful, thanks for reading!
在本文中,我们涵盖了许多概念,包括字典视图对象(dict.keys(),dict.values()和dict.items())是动态视图对象,当字典条目发生更改时,视图对象也将是动态视图对象。动态更新,并且dict上的内置函数和成员资格测试将仅对键起作用,而在dictview上,它们将对键,值和项起作用。 带有可哈希值的键视图和项视图的设置类似于,我们可以对此执行设置操作。 值视图不支持设置操作。 希望本文对您有所帮助,感谢您的阅读!
资源(Python文档): (Resources(Python Documentation):)
翻译自: https://codeburst.io/dictionary-view-objects-101-480b72f71dec
canopen 对象字典