团子校招
近日,美团宣布开启面向 2025 届的校园招聘,招聘规模达 6000 人。
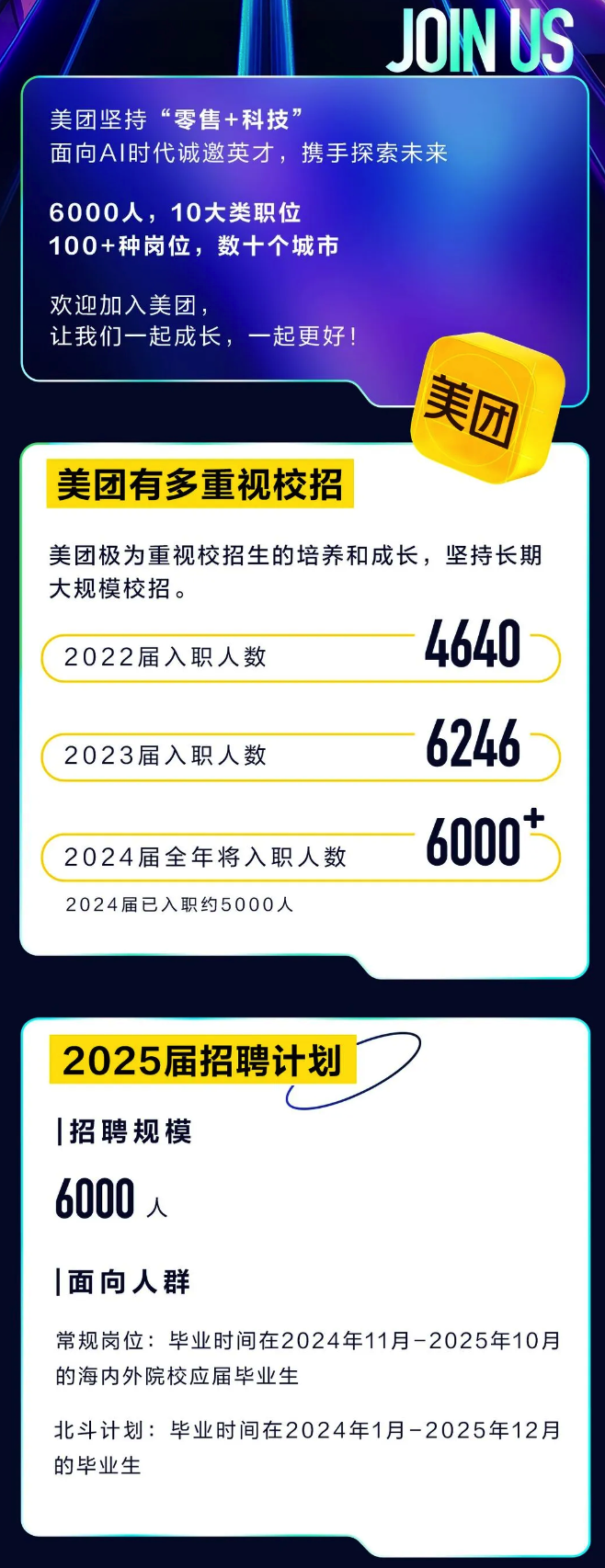
虽然相比京东(宣布招聘 16000+ 人)稍有逊色,但 6000 这个校招规模可一点不少。
要知道,京东是重自营的传统电商,16000+ 的招聘规模,必然包含了超大量"非技术"的岗位,例如物流、仓管、客服和行政等人员。
如果按照京东现有的技术正式员工比例(技术类正式员工 11 万,总正式员工 59 万)来折算,16000+ 的招聘规模里面,大概只有 3000 不到的 HC 是和技术岗位相关的。
美团则是专注本地生活的标准轻量化互联网企业,绝大多数的工作岗位和技术类相关,6000 的招聘规模折算出来的技术类岗位,必然是要多于京东的。
从美团过去几年的入职情况来看,美团既没有出现断崖式收缩 HC,也没有出现毁应届等新闻,总包也不算差,唯一美中不足的是没啥福利,因而也被戏称为"开水团"(只有开水是免费喝),但就目前的就业行情来看,将美团作为 TOP 5 的心仪公司来考虑,没啥毛病。
...
回归主题。
来一道和「美团-校招-T2」相关的变形题。
先来道当时的签到题,等大家觉得又行了,过两天再来道当时的压轴题。
题目描述
平台:LeetCode
题号:1410
「HTML 实体解析器」 是一种特殊的解析器,它将 HTML 代码作为输入,并用字符本身替换掉所有这些特殊的字符实体。
HTML 里这些特殊字符和它们对应的字符实体包括:
-
双引号:字符实体为 "
,对应的字符是"
。 -
单引号:字符实体为 '
,对应的字符是'
。 -
与符号:字符实体为 &
,对应对的字符是&
。 -
大于号:字符实体为 >
,对应的字符是>
。 -
小于号:字符实体为 <
,对应的字符是<
。 -
斜线号:字符实体为 ⁄
,对应的字符是/
。
给你输入字符串 text
,请你实现一个 HTML 实体解析器,返回解析器解析后的结果。
示例 1:
输入:text = "& is an HTML entity but &ambassador; is not."
输出:"& is an HTML entity but &ambassador; is not."
解释:解析器把字符实体 & 用 & 替换
示例 2:
输入:text = "and I quote: "...""
输出:"and I quote: \"...\""
示例 3:
输入:text = "Stay home! Practice on Leetcode :)"
输出:"Stay home! Practice on Leetcode :)"
示例 4:
输入:text = "x > y && x < y is always false"
输出:"x > y && x < y is always false"
示例 5:
输入:text = "leetcode.com⁄problemset⁄all"
输出:"leetcode.com/problemset/all"
提示:
-
-
字符串可能包含 个 ASCII
字符中的任意字符。
模拟
每个特殊字符均以 &
开头,最长一个特殊字符为 ⁄
。
从前往后处理 text
,若遇到 &
则往后读取最多 6 个字符(中途遇到结束字符 ;
则终止),若读取子串为特殊字符,将使用替换字符进行拼接,否则使用原字符进行拼接。
Java 代码:
class Solution {
public String entityParser(String text) {
Map<String, String> map = new HashMap<>(){{
put(""", "\"");
put("'", "'");
put("&", "&");
put(">", ">");
put("<", "<");
put("⁄", "/");
}};
int n = text.length();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < n; ) {
if (text.charAt(i) == '&') {
int j = i + 1;
while (j < n && j - i < 6 && text.charAt(j) != ';') j++;
String sub = text.substring(i, Math.min(j + 1, n));
if (map.containsKey(sub)) {
sb.append(map.get(sub));
i = j + 1;
continue;
}
}
sb.append(text.charAt(i++));
}
return sb.toString();
}
}
C++ 代码:
class Solution {
public:
string entityParser(string text) {
unordered_map<string, string> entityMap = {
{""", "\""},
{"'", "'"},
{"&", "&"},
{">", ">"},
{"<", "<"},
{"⁄", "/"}
};
int n = text.length();
string ans = "";
for (int i = 0; i < n; ) {
if (text[i] == '&') {
int j = i + 1;
while (j < n && j - i < 6 && text[j] != ';') j++;
string sub = text.substr(i, min(j + 1, n) - i);
if (entityMap.find(sub) != entityMap.end()) {
ans += entityMap[sub];
i = j + 1;
continue;
}
}
ans += text[i++];
}
return ans;
}
};
Python 代码:
class Solution:
def entityParser(self, text: str) -> str:
entity_map = {
""": "\"",
"'": "'",
"&": "&",
">": ">",
"<": "<",
"⁄": "/"
}
i, n = 0, len(text)
ans = ""
while i < n:
if text[i] == '&':
j = i + 1
while j < n and j - i < 6 and text[j] != ';':
j += 1
sub = text[i:min(j + 1, n)]
if sub in entity_map:
ans += entity_map[sub]
i = j + 1
continue
ans += text[i]
i += 1
return ans
TypeScript 代码:
function entityParser(text: string): string {
const entityMap: { [key: string]: string } = {
""": "\"",
"'": "'",
"&": "&",
">": ">",
"<": "<",
"⁄": "/"
};
const n = text.length;
let ans = "";
for (let i = 0; i < n; ) {
if (text[i] == '&') {
let j = i + 1;
while (j < n && j - i < 6 && text[j] != ';') j++;
const sub = text.substring(i, Math.min(j + 1, n));
if (entityMap[sub]) {
ans += entityMap[sub];
i = j + 1;
continue;
}
}
ans += text[i++];
}
return ans;
};
-
时间复杂度: ,其中 为最大特殊字符长度 -
空间复杂度: ,一个固定大小的哈希表
最后
巨划算的 LeetCode 会员优惠通道目前仍可用 ~
使用福利优惠通道 leetcode.cn/premium/?promoChannel=acoier,年度会员 有效期额外增加两个月,季度会员 有效期额外增加两周,更有超大额专属 🧧 和实物 🎁 福利每月发放。
我是宫水三叶,每天都会分享算法知识,并和大家聊聊近期的所见所闻。
欢迎关注,明天见。
更多更全更热门的「笔试/面试」相关资料可访问排版精美的 合集新基地 🎉🎉