四、创建多个线程、数据共享问题分析、案例代码
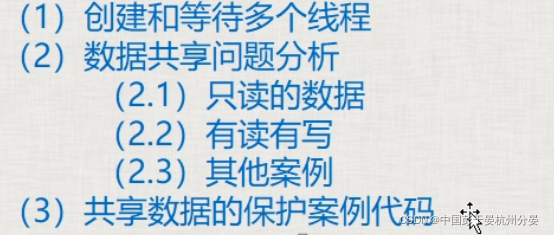
1.创建和等待多个线程
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <string>
#include <vector>
#include <thread>
using namespace std;
void TestThread(int num)
{
cout << "TextThread线程开始执行了,线程编号 = " << num << endl;
cout << "TextThread线程结束了,线程编号 = " << num << endl;
}
int main(int argc, char* argv[])
{
std::vector<std::thread> vec_threads;
for (int i = 0; i < 10; i++)
{
vec_threads.push_back(std::thread(TestThread, i));
}
for (auto iter = vec_threads.begin(); iter != vec_threads.end(); ++iter)
{
iter->join();
}
cout << "I LOve China!" << endl;
system("pause");
return 0;
}
- (1)多个线程执行顺序是乱的,跟操作系统内部对线程的运行调度机制有关
- (2)主线程等待所有子线程运行结束,最后主线程结束,推荐使用join
- (3) 把thread放到容器里管理,看起来像个thread对象数组
2.数据共享问题分析
2.1只读的数据
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <string>
#include <vector>
#include <thread>
using namespace std;
std::vector<int> vec_nums = { 1, 2, 3 };
void TestThread(int num)
{
cout << "TextThread线程编号 = " << std::this_thread::get_id() <<
" vec_nums = {" << vec_nums[0] << "," << vec_nums[1] << "," <<
vec_nums[2] << "}" << endl;
}
int main(int argc, char* argv[])
{
std::vector<std::thread> vec_threads;
for (int i = 0; i < 10; i++)
{
vec_threads.push_back(std::thread(TestThread, i));
}
for (auto iter = vec_threads.begin(); iter != vec_threads.end(); ++iter)
{
iter->join();
}
cout << "I LOve China!"<<endl;
system("pause");
return 0;
}
- 只读的数据是安全稳定的,不需要什么特别的处理手段,直接读就可以。
2.2有读有写
- 2个线程写,8个线程读,如果代码没有特别的处理,程序肯定崩溃
- 最简单的处理:读的时候不能写,写的时候不能读;2个线程不能同时写,8个线程不能同时读。
2.3其他案例

3.共享数据的保护案例代码
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <string>
#include <list>
#include <thread>
using namespace std;
class A
{
public:
void inMesgRecvQueue()
{
for (int i = 0; i < 100000; ++i)
{
cout << "inMesgRecvQueue执行,插入一个元素" << i << endl;
list_msg.push_back(i);
}
}
void outMesgRecvQueue()
{
for (int i = 0; i < 100000; ++i)
{
if (!list_msg.empty())
{
int command = list_msg.front();
list_msg.pop_front();
}
else
{
cout << "消息队列为空" << endl;
}
}
cout << "outMesgRecvQueue end" << endl;
}
protected:
private:
std::list<int> list_msg;
};
int main(int argc, char* argv[])
{
A myobja;
std::thread myOutMsgobj(&A::outMesgRecvQueue, &myobja);
std::thread myInMsgobj(&A::inMesgRecvQueue, &myobja);
myOutMsgobj.join();
myInMsgobj.join();
system("pause");
return 0;
}