表面建模与曲面优化
在汽车设计中,表面建模和曲面优化是至关重要的步骤。这一部分将详细介绍如何在NX中进行高效的表面建模和曲面优化,以满足汽车设计的高精度和高质量要求。我们将从基础的表面建模技术开始,逐步深入到高级的曲面优化方法,并通过具体的例子来说明这些技术的应用。
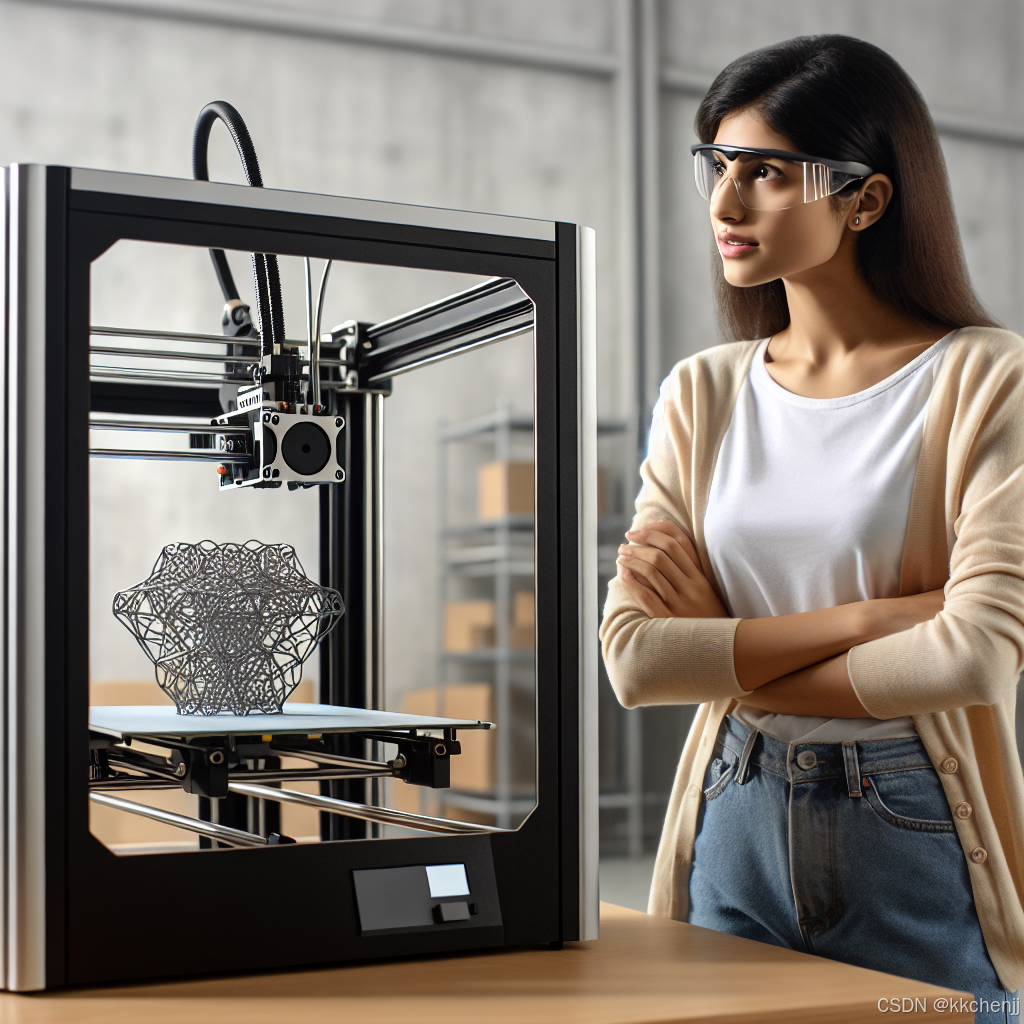
1. 表面建模基础
1.1 曲面创建方法
在NX中,有多种方法可以创建曲面,包括但不限于:
-
通过点创建曲面:使用多个点或点集来定义曲面的形状。
-
通过曲线创建曲面:使用曲线来构建曲面,如扫掠、放样、旋转等。
-
通过现有几何体创建曲面:从现有的几何体(如实体或其它曲面)中提取或生成新的曲面。
1.1.1 通过点创建曲