vtkSource的主要类型
目前整理的有下面几种source,对应有点类似threejs的mesh,通过一定的参数,就可以得到预制的几何体,这个在vtk里面叫source数据源。
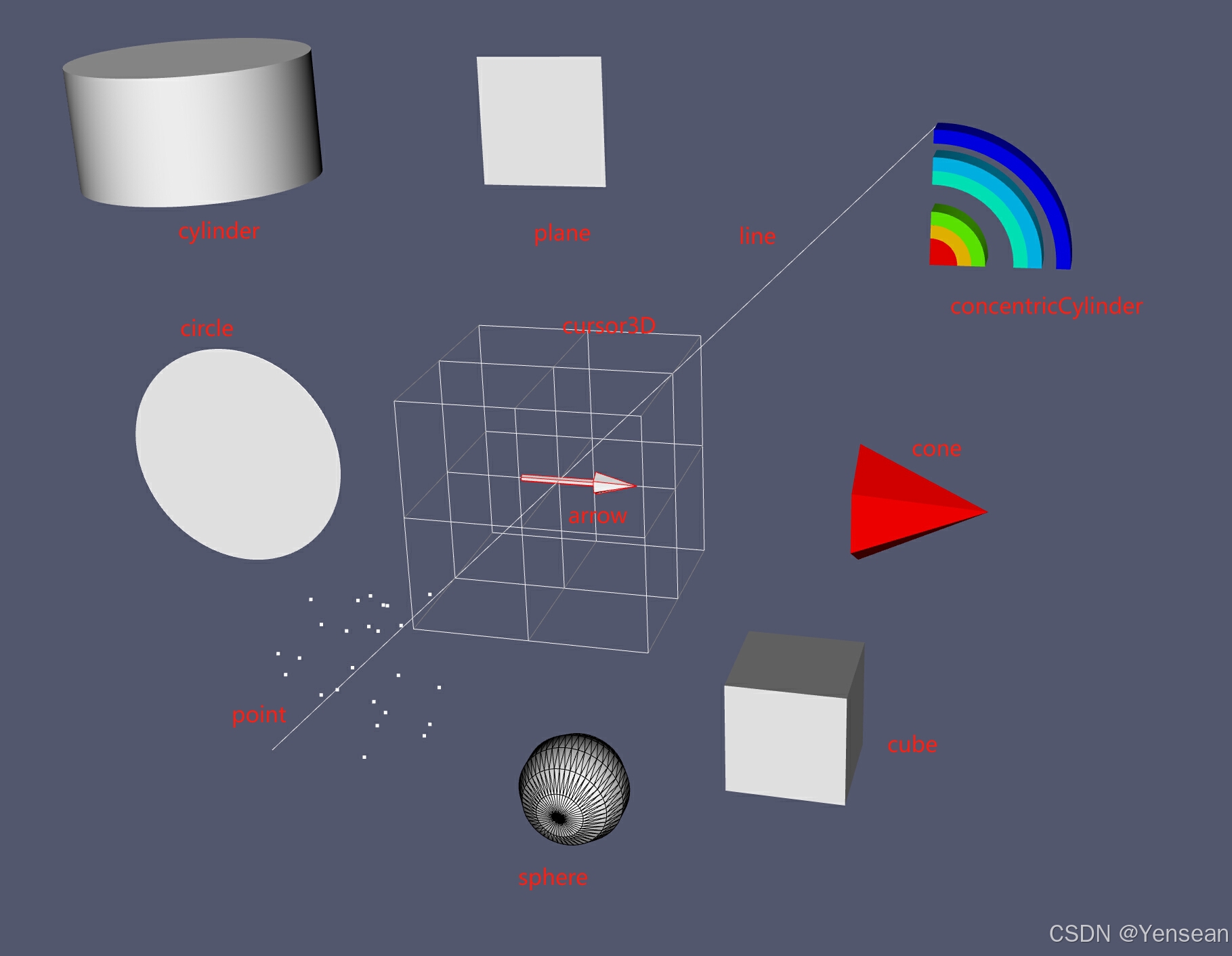
Cone 锥体
export function createCone() {
const source = vtkConeSource.newInstance({ height: 1.0 });
// this.coneSource = vtkCubeSource.newInstance({
// xLength: 5,
// yLength: 5,
// zLength: 5,
// });
source.set({
center: [3, 0, 0],
});
const mapper = vtkMapper.newInstance();
let coneOutPut = source.getOutputPort();
mapper.setInputConnection(coneOutPut);
const actor = vtkActor.newInstance();
actor.getProperty().setColor(1, 0, 0);
actor.setMapper(mapper);
return { source, mapper, actor };
}
Circle 圆形
export function createCircle() {
const source = vtkCircleSource.newInstance();
source.set({
center: [-3, 0, 0],
resolution: 120,
direction: [0, 0, 1],
});
const actor = vtkActor.newInstance();
const mapper = vtkMapper.newInstance();
source.setLines(true);
source.setFace(true);
actor.setMapper(mapper);
mapper.setInputConnection(source.getOutputPort());
return { source, mapper, actor };
}
Arrow 箭头
export function createArrow() {
const source = vtkArrowSource.newInstance();
const actor = vtkActor.newInstance();
const mapper = vtkMapper.newInstance();
actor.setMapper(mapper);
actor.getProperty().setEdgeVisibility(true);
actor.getProperty().setEdgeColor(1, 0, 0);
actor.getProperty().setRepresentationToSurface();
mapper.setInputConnection(source.getOutputPort());
return { source, mapper, actor };
}
ConcentricCylinder 同心圆
export function createConcentricCylinder() {
const source = vtkConcentricCylinderSource.newInstance({
height: 0.25,
center: [3, 2, 0],
radius: [0.2, 0.3, 0.4, 0.6, 0.7, 0.8, 0.9, 1],
cellFields: [0, 0.2, 0.4, 0.6, 0.7, 0.8, 0.9, 1],
startTheta: 0,
endTheta: 90,
resolution: 60,
skipInnerFaces: true,
});
source.setMaskLayer(3, true);
source.setMaskLayer(6, true);
const actor = vtkActor.newInstance();
const mapper = vtkMapper.newInstance();
actor.setMapper(mapper);
mapper.setInputConnection(source.getOutputPort());
return { source, mapper, actor };
}
Cube 方形
export function createCube() {
const source = vtkCubeSource.newInstance();
source.setCenter([2, -2, 0]);
const actor = vtkActor.newInstance();
const mapper = vtkMapper.newInstance();
actor.setMapper(mapper);
mapper.setInputConnection(source.getOutputPort());
return { source, mapper, actor };
}
Cursor3D 包围盒
export function createCursor3D() {
const source = vtkCursor3D.newInstance();
source.setFocalPoint([0, 0, 0]);
source.setModelBounds([-1, 1, -1, 1, -1, 1]);
const mapper = vtkMapper.newInstance();
mapper.setInputConnection(source.getOutputPort());
const actor = vtkActor.newInstance();
actor.setMapper(mapper);
return { source, mapper, actor };
}
Cylinder 圆柱体
export function createCylinder() {
const source = vtkCylinderSource.newInstance();
source.setCenter([-3, 3, 0]);
source.setResolution(100);
const actor = vtkActor.newInstance();
const mapper = vtkMapper.newInstance();
actor.setMapper(mapper);
mapper.setInputConnection(source.getOutputPort());
return { source, mapper, actor };
}
Line 线
export function createLine() {
const source = vtkLineSource.newInstance();
source.setPoint1([3, 3, 0]);
source.setPoint2([-3, -3, 0]);
const actor = vtkActor.newInstance();
const mapper = vtkMapper.newInstance();
actor.getProperty().setPointSize(10);
actor.setMapper(mapper);
mapper.setInputConnection(source.getOutputPort());
return { source, mapper, actor };
}
Plane 平面
export function createPlane() {
const source = vtkPlaneSource.newInstance();
source.setCenter([0, 3, 0]);
const mapper = vtkMapper.newInstance();
const actor = vtkActor.newInstance();
mapper.setInputConnection(source.getOutputPort());
actor.setMapper(mapper);
return { source, mapper, actor };
}
Point 点
export function createPoint() {
const source = vtkPointSource.newInstance({
numberOfPoints: 25,
radius: 1,
});
source.setCenter([-2, -2, 0]);
// source.setNumberOfPoints(25);
// source.setRadius(0.25);
const mapper = vtkMapper.newInstance();
const actor = vtkActor.newInstance();
actor.getProperty().setEdgeVisibility(true);
actor.getProperty().setPointSize(5);
mapper.setInputConnection(source.getOutputPort());
actor.setMapper(mapper);
return { source, mapper, actor };
}
Sphere 球
export function createSphere() {
const source = vtkSphereSource.newInstance();
source.setCenter([0, -3, 0]);
source.setThetaResolution(50);
const actor = vtkActor.newInstance();
const mapper = vtkMapper.newInstance();
actor.getProperty().setEdgeVisibility(true);
mapper.setInputConnection(source.getOutputPort());
actor.setMapper(mapper);
return { source, mapper, actor };
}
不能调整center的source
目前所列的source中,只有Arrow和Cursor3D没有setCenter的方法,只有圆心都在原点[0,0,0]的位置,查看一下对应的源码可以发现,其实是少了一句,对点的矩阵的偏移,其他source里面对应的核心代码是:
// Apply transformation to the points coordinates
vtkMatrixBuilder.buildFromRadian().translate(...model.center).rotateFromDirections([1, 0, 0], model.direction).apply(points);
这个坑得稍微注意一下
全部代码都放到github上了
新坑_Learning vtkjs_git地址
关注我,我持续更新vtkjs的example学习案例
也欢迎各位给我提意见,技术交流~
大鸿
WeChat : HugeYen
WeChat Public Account : BIM树洞
做一个静谧的树洞君
用建筑的语言描述IT事物;
用IT的思维解决建筑问题;
共建BIM桥梁,聚合团队。
本学习分享资料不得用于商业用途,仅做学习交流!!如有侵权立即删除!!