#ifndef __SYS_HELPER_H__
#define __SYS_HELPER_H__
#include <ETH.h>
#include "node_data.h"
#include "stdio.h"
#include "version.h"
#include <map>
#include "basic_node.hpp"
#include <Preferences.h>
#include <WiFi.h>
#include <iostream>
#include <string>
#include"SPIFFS.h"
#include <stdio.h>
#include <string.h>
#include <sys/unistd.h>
#include <sys/stat.h>
#include "esp_err.h"
#include "esp_log.h"
#include "esp_spiffs.h"
#define PARA_FLASH_NAME "nvs"
using namespace std;
Preferences preferences;
bool node_setting = false;
esp_vfs_spiffs_conf_t conf = {
.base_path = "/fat", //根目录
.partition_label = NULL, //分区标签,指针类型
.max_files = 5, //该目录下能存储的最大文件数
.format_if_mount_failed = true //如果挂载失败则会重启
};
template <class T>
void loadNodeSysSetting(T &data)
{
preferences.begin("sys", false);
preferences.getBytes("sys", &data, sizeof(T));
preferences.end();
};
template <class T>
void saveNodeSysSetting(T &data)
{
preferences.begin("sys", false);
preferences.putBytes("sys", &data, sizeof(T));
preferences.end();
};
template <class T>
void loadNodeSetting(T &data)
{
preferences.begin("sensor", false);
preferences.getBytes("sensor", &data, sizeof(T));
preferences.end();
};
template <class T>
void saveNodeSetting(T &data)
{
preferences.begin("sensor", false);
preferences.putBytes("sensor", &data, sizeof(T));
preferences.end();
};
void sensor_restart()
{
digitalWrite(SENSOR_POWER_PIN, HIGH);
delay(1000);
node_setting = false;
Serial.println("nodeRestart");
}
void system_restart()
{
Serial.println("ESP32Restart");
ESP.restart();
}
uint16_t crc16(uint8_t *data, int len)
{
uint16_t crc = 0xFFFF;
for (int pos = 0; pos < len; pos++)
{
crc ^= (uint16_t)data[pos];
for (int i = 8; i != 0; i--)
{
if ((crc & 0x0001) != 0)
{
crc >>= 1;
crc ^= 0xA001;
}
else
crc >>= 1;
}
}
return crc;
}
void send_debug_message(AsyncUDP *send_testmsg_udp, std::string testmsg)
{
const uint8_t* data = reinterpret_cast<const uint8_t*>(testmsg.c_str());
send_testmsg_udp->writeTo(data,testmsg.length(),DEFAULT_ETH_GATEWAY, NODE_TEST_UDP_LOCAL_PORT);
}
//初始化spiffs
void init_spiffs(void){
Serial.println("Initializing SPIFFS");
//挂载spiffs文件,挂载之后可以使用C库进行读写文件
esp_err_t ret = esp_vfs_spiffs_register(&conf);
if (ret != ESP_OK) {
if (ret == ESP_FAIL) {
Serial.println("Failed to mount or format filesystem");
} else if (ret == ESP_ERR_NOT_FOUND) {
Serial.println("Failed to find SPIFFS partition");
} else {
Serial.println("Failed to initialize SPIFFS");
}
return;
}
//这部分可以暂时不看
#ifdef CONFIG_EXAMPLE_SPIFFS_CHECK_ON_START
Serial.println("Performing SPIFFS_check().");
ret = esp_spiffs_check(conf.partition_label);
if (ret != ESP_OK) {
Serial.println("SPIFFS_check() failed");
return;
} else {
Serial.println("SPIFFS_check() successful");
}
#endif
//获取文件系统已经使用的大小和剩余大小
size_t total = 0, used = 0;
ret = esp_spiffs_info(conf.partition_label, &total, &used);
if (ret != ESP_OK) {
Serial.println("Failed to get SPIFFS partition information. Formatting...");
esp_spiffs_format(conf.partition_label);
return;
} else {
Serial.printf("Partition size: total: %d, used: %d", total, used);
Serial.println();
}
#
// 检查分区大小信息
if (used > total) {
Serial.println("Number of used bytes cannot be larger than total. Performing SPIFFS_check().");
ret = esp_spiffs_check(conf.partition_label);
// 也可以用来修复损坏的文件,清理未引用的页面等。
// More info at https://github.com/pellepl/spiffs/wiki/FAQ#powerlosses-contd-when-should-i-run-spiffs_check
if (ret != ESP_OK) {
Serial.println("SPIFFS_check() failed");
return;
} else {
Serial.println("SPIFFS_check() successful");
}
}
}
void write_log(std::string testmsg){
testmsg += "<br>";
size_t total = 0, used = 0;
FILE* f;
esp_err_t ret = esp_spiffs_info(conf.partition_label, &total, &used);
Serial.printf("Partition size: total: %d, used: %d", total, used);
Serial.println();
if (used > 10240){
f = fopen("/fat/log.txt", "w");
fclose(f);//关闭文件
}
f = fopen("/fat/log.txt", "a");
if (f == NULL) {
Serial.println("Failed to open file for writing");
return;
}
char* cstr = const_cast<char*>(testmsg.c_str());
fprintf(f, cstr);//写入字符
fclose(f);//关闭文件
}
//卸载文件系统
void ds_spiffs_deinit(void){
// All done, unmount partition and disable SPIFFS
esp_vfs_spiffs_unregister(conf.partition_label);
}
#endif // __SYS_HELPER_H__
esp 32
最新推荐文章于 2024-08-05 17:11:11 发布
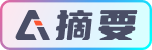