using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class AsyncLoadScene : MonoBehaviour
{
public Image loadingSlider;//加载条,加载图片要为fille,选择horizontal
private float loadingSpeed = 1;
private float targetValue;
private AsyncOperation operation;
private string nextSceneName;
private bool isOperation; // 是否已开启异步加载协程
private void Awake()
{
// 清一下内存空间
Resources.UnloadUnusedAssets();
System.GC.Collect();
System.GC.WaitForPendingFinalizers();//挂起当前线程,直到处理终结器队列的线程清空该队列为止
}
void Start()
{
Init();
if(SceneManager.GetActiveScene().name == GameDefine.加载)
{
StartCoroutine(AsynLoading());
}
}
private void Init()
{
// 如果是菜单场景过来的就根据记录的场景名加载下一个场景,如果不是就加载菜单场景
nextSceneName = FjData.GetInstance().isMenuScene ? FjData.GetInstance().nextSceneName : GameDefine.菜单;
loadingSlider.fillAmount = 0.0f;
}
IEnumerator AsynLoading()
{
yield return new WaitForEndOfFrame();
operation = SceneManager.LoadSceneAsync(nextSceneName);
isOperation = true;
//阻止当加载完成自动切换
operation.allowSceneActivation = false;
yield return operation;
}
private void Update()
{
if (isOperation)
{
targetValue = operation.progress;
if (operation.progress >= 0.9f)
{
targetValue = 1.0f;
}
if (targetValue != loadingSlider.fillAmount)
{
//插值运算
loadingSlider.fillAmount = Mathf.Lerp(loadingSlider.fillAmount, targetValue, Time.deltaTime * loadingSpeed);
if (Mathf.Abs(loadingSlider.fillAmount - targetValue) < 0.01f)
{
loadingSlider.fillAmount = targetValue;
}
}
if (((int) loadingSlider.fillAmount * 100) == 100)
{
operation.allowSceneActivation = true;
}
}
}
private void OnDestroy()
{
isOperation = false;
StopCoroutine(AsynLoading());
}
}
unity异步加载
于 2023-10-10 11:41:51 首次发布
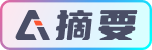