本文运用了像素压缩算法 此方法可以运用很多(例如:图片太大却想要运用,就可使用像素压缩算法进行压缩处理)
效果图
三角形索引理解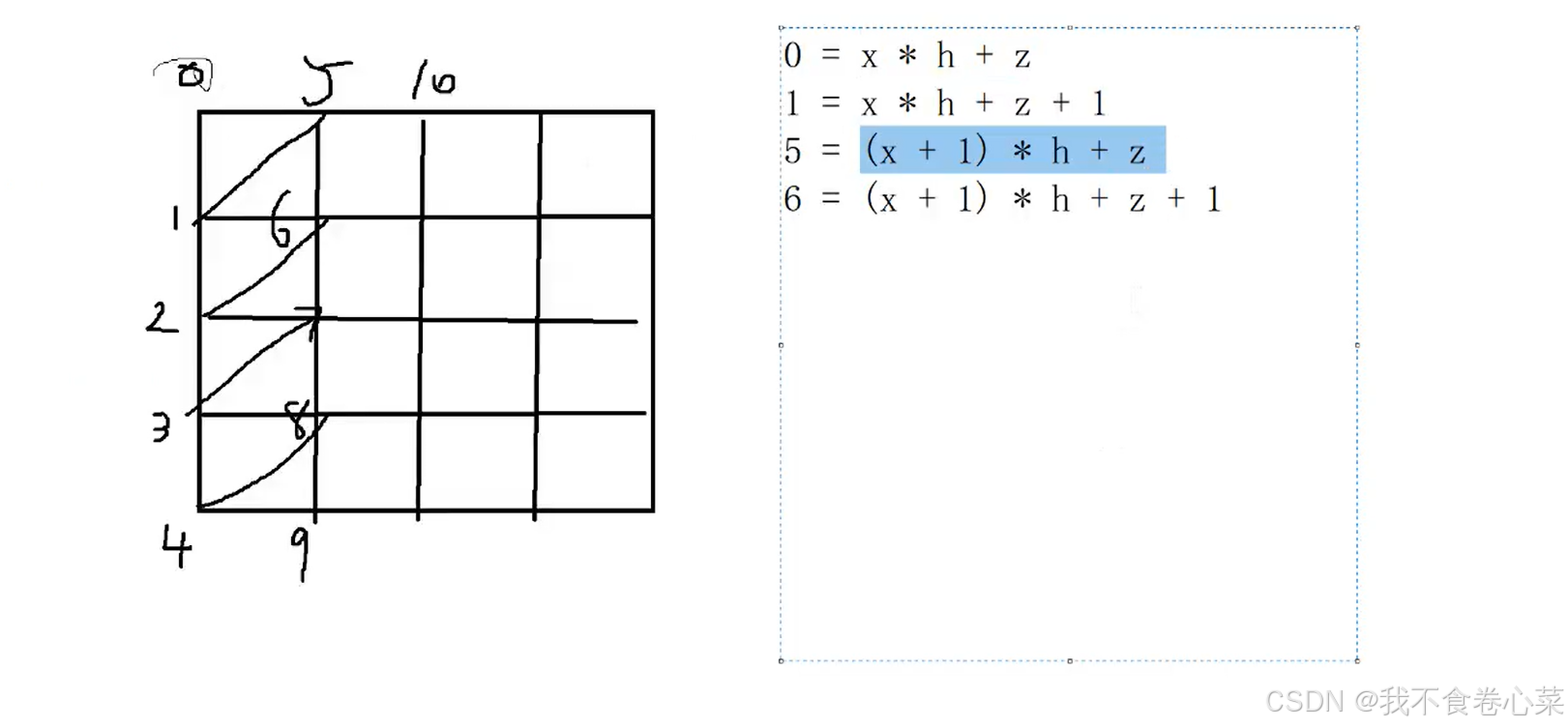
新项目创建一个Plane地板 将此脚本挂在Plane地板对象上
像素压缩算法
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyPlane : MonoBehaviour
{
public MeshFilter filter;
public MeshRenderer renderer;
public MeshCollider collider;
public Texture2D texture; //地图图片
public int w;
public int h;
// Start is called before the first frame update
void Start()
{
Mesh mesh = new Mesh();
VertexHelper vh = new VertexHelper();
int px = texture.width / w;
int py = texture.height / h;
for (int i = 0; i <= w; i++)
{
for (global::System.Int32 j = 0; j <= h; j++)
{
//像素压缩算法
//获取图片颜色 因为可能有些图片太大
List<Color> list=new List<Color>();
for (global::System.Int32 x = 0; x < px; x++)
{
for (global::System.Int32 z = 0; z < py; z++)
{
list.Add(texture.GetPixel(i * px + x, j * py + z));//GetPixel获取像素点
}
}
Color color=new Color(0,0,0,0);
foreach (var item in list)
{
color += item;
}
color=color/list.Count;
float y = color.grayscale; //灰度值
float uvx = (float)i / (float)w;
float uvy = (float)j / (float)h;
vh.AddVert(new Vector3(i, y, j), Color.white, new Vector2(uvx, uvy));
if (i != w && j != h)
{
//因为每次多一个顶点 所以h+1
vh.AddTriangle(i * (h + 1) + j, i * (h + 1) + j + 1, (i + 1) * (h + 1) + j);
vh.AddTriangle(i * (h + 1) + j + 1, (i + 1) * (h + 1) + j + 1, (i + 1) * (h + 1) + j);
//反面
//vh.AddTriangle(i * (h + 1) + j, (i + 1) * (h + 1) + j, i * (h + 1) + j + 1);
//vh.AddTriangle(i * (h + 1) + j + 1, (i + 1) * (h + 1) + j, (i + 1) * (h + 1) + j + 1);
}
}
}
//把顶点助手的信息写入mesh
vh.FillMesh(mesh);
filter.mesh = mesh;
renderer.material.mainTexture = texture;
collider.sharedMesh = mesh;
}
// Update is called once per frame
void Update()
{
}
}
柏林噪声法
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyPlane : MonoBehaviour
{
public MeshFilter filter;
public MeshRenderer renderer;
public MeshCollider collider;
public Color gao;
public Color di;
public Texture2D texture; //地图图片
public int w;
public int h;
// Start is called before the first frame update
void Start()
{
Mesh mesh = new Mesh();
VertexHelper vh = new VertexHelper();
int px = texture.width / w;
int py = texture.height / h;
for (int i = 0; i <= w; i++)
{
for (global::System.Int32 j = 0; j <= h; j++)
{
//像素压缩算法
//获取图片颜色 因为可能有些图片太大
//List<Color> list=new List<Color>();
//for (global::System.Int32 x = 0; x < px; x++)
//{
// for (global::System.Int32 z = 0; z < py; z++)
// {
// list.Add(texture.GetPixel(i * px + x, j * py + z));//GetPixel获取像素点
// }
//}
//Color color=new Color(0,0,0,0);
//foreach (var item in list)
//{
// color += item;
//}
//color=color/list.Count;
//float y = color.grayscale; //灰度值
//柏林噪声算法
float y = Mathf.PerlinNoise(i * 0.1f, j * 0.1f);
float uvx = (float)i / (float)w;
float uvy = (float)j / (float)h;
Color color = Color.Lerp(di, gao, y);
vh.AddVert(new Vector3(i, y*10, j), Color.white, new Vector2(uvx, uvy));
if (i != w && j != h)
{
//因为每次多一个顶点 所以h+1
vh.AddTriangle(i * (h + 1) + j, i * (h + 1) + j + 1, (i + 1) * (h + 1) + j);
vh.AddTriangle(i * (h + 1) + j + 1, (i + 1) * (h + 1) + j + 1, (i + 1) * (h + 1) + j);
//反面
//vh.AddTriangle(i * (h + 1) + j, (i + 1) * (h + 1) + j, i * (h + 1) + j + 1);
//vh.AddTriangle(i * (h + 1) + j + 1, (i + 1) * (h + 1) + j, (i + 1) * (h + 1) + j + 1);
}
}
}
texture.Apply();
//把顶点助手的信息写入mesh
vh.FillMesh(mesh);
filter.mesh = mesh;
renderer.material.mainTexture = texture;
collider.sharedMesh = mesh;
}
// Update is called once per frame
void Update()
{
}
}
柏林噪声法生成地图2地址:柏林噪声生成地图、水面、小地图生成、小地图映射水面、Mesh-CSDN博客
小菜不易,感谢观看,学到了就留下个支持的赞吧!
关注我,了解更多Unity相关知识哦!