紧接着上一篇,本博客在上一篇的基础上进一步绘制各个变量变化情况
主要应用tf.summary.scalar/tf.summary.histogram等等不同类型的图
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets('MNIST_data', one_hot=True)
iteration_num = 50
learning_rate = 0.2
batch_size = 64
n_batch = mnist.train.num_examples // batch_size
def analysis_variable(var):
with tf.name_scope("summaries"):
mean = tf.reduce_mean(var)
stddev = tf.sqrt(tf.reduce_mean(tf.square(var - mean)))
max = tf.reduce_max(var)
min = tf.reduce_min(var)
tf.summary.scalar('mean', mean)
tf.summary.scalar('stddev', stddev)
tf.summary.scalar('max', max)
tf.summary.scalar('min', min)
tf.summary.histogram('histogram', var)
with tf.name_scope('input'):
x = tf.placeholder(tf.float32, [None, 784], name='x_input')
y = tf.placeholder(tf.float32, [None, 10], name='y_input')
with tf.name_scope('weight'):
with tf.name_scope('w_weight'):
w = tf.Variable(tf.zeros([784, 10]))
with tf.name_scope('b_weight'):
b = tf.Variable(tf.zeros([10]))
with tf.name_scope('wx'):
wx = tf.matmul(x, w)
with tf.name_scope('softmax'):
prd = tf.nn.softmax(wx + b)
with tf.name_scope('loss'):
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y, logits=prd))
with tf.name_scope('train'):
train = tf.train.GradientDescentOptimizer(learning_rate).minimize(loss)
with tf.name_scope('accuracy_part'):
with tf.name_scope('correct_prd'):
correct_prd = tf.equal(tf.argmax(y, 1), tf.argmax(prd, 1))
with tf.name_scope('accuracy'):
accuracy = tf.reduce_mean(tf.cast(correct_prd, tf.float32))
init = tf.global_variables_initializer()
merged = tf.summary.merge_all()
with tf.Session() as sess:
sess.run(init)
writer = tf.summary.FileWriter('logs/', sess.graph)
for epoch in range(iteration_num):
for batch in range(n_batch):
batch_xs, batch_ys = mnist.train.next_batch(batch_size)
summary, _ = sess.run([merged, train], feed_dict={x: batch_xs, y: batch_ys})
writer.add_summary(summary, epoch)
acc = sess.run(accuracy, feed_dict={x: mnist.test.images, y: mnist.test.labels})
print('Iteration_num' + str(epoch) + ',Testing_acc is ' + str(acc))
首先在terminal上输入一下语句
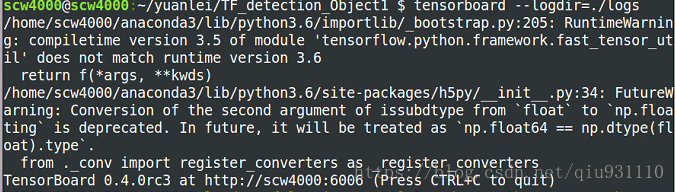
将最后那个网址打开可以看到如下图片所示
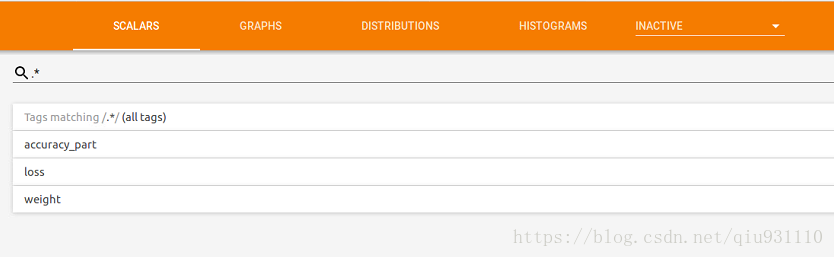
点开accuracy_part
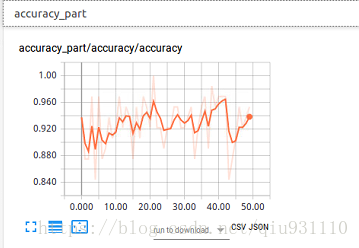
点开loss
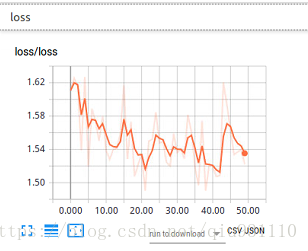
点开weight
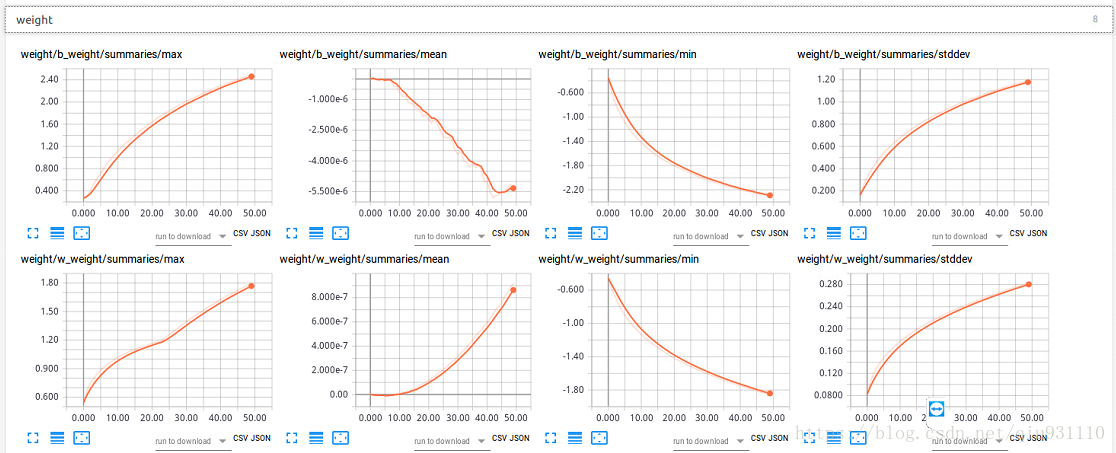
再点开目标栏的histogram
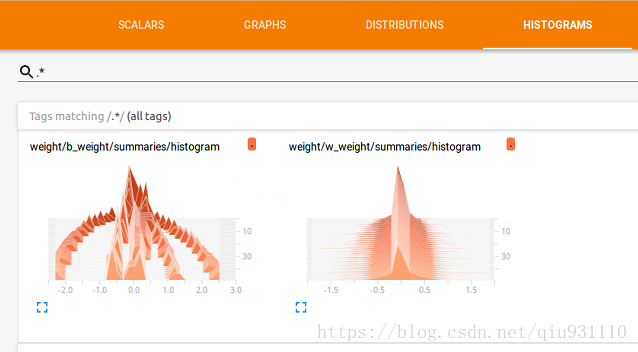
点开distribution
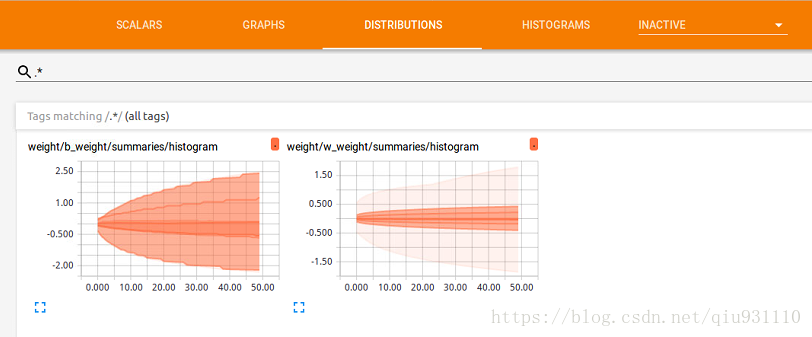