1、strstr-whiledowhile模型
#include <stdio.h>
#include<string.h>
#include<stdlib.h>
int main02(int argc, char *argv[])
{
int ncount = 0;
char *p= "abcd1234abcd1234kdabcdkdj873abcd321";//求字符串中abcd出现的次数
while((p = strstr(p,"abcd"))!='\0')
{
ncount++;
p += strlen("abcd");
printf("p:%s\n",p);
if(*p == NULL)
{
break;
}
}
printf("ncount:%d",ncount);
printf("Hello World!\n");
return 0;
}
int main01(int argc, char *argv[])
{
char *p= "abcd1234abcd1234kdabcdkdj873abcd321";//求字符串中abcd出现的次数
int ncount = 0;
do
{
p = strstr(p,"abcd");
if(p != NULL)
{
ncount++;
p += strlen("abcd");
printf("p:%s\n",p);
}
else
{
break;
}
}while(*p != '\0');
printf("ncount:%d",ncount);
printf("Hello World!\n");
return 0;
}
int getCount(char *mystr,char *sub,int *ncount)
{
char *tmpMystr = mystr;
char *tmpSub = sub;
int tmpCount = 0;
if(mystr == NULL||sub == NULL)
{
return -1;
}
while((mystr = strstr(mystr,sub))!='\0')
{
tmpCount++;
mystr += strlen(sub);
printf("mystr:%s\n",mystr);
if(*mystr == NULL)
{
break;
}
}
*ncount = tmpCount;
return 0;
}
int main(int argc, char *argv[])
{
char *p= "abcd1234abcd1234kdabcdkdj873abcd321";//求字符串中abcd出现的次数
char *mysub = "abcd";
int ncount = 0;
int ret = getCount(p,mysub,&ncount);
printf("ncount:%d\n",ncount);
printf("Hello World!\n");
return 0;
}
2、两头堵模型
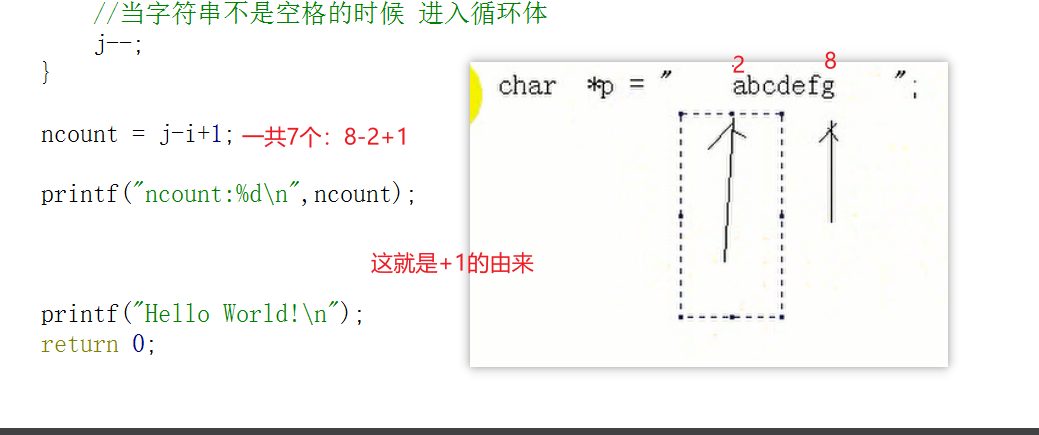
#include <stdio.h>
#include<string.h>
#include<stdlib.h>
int getCount(char *mystr,int *ncount)
{
char *tmpMystr = mystr;
int i = 0;
int j = 0;
int tmpCount = 0;
if(mystr == NULL || ncount == NULL)
{
return -1;
}
//假设是"123456" strlen=6 下标从0开始 就是0-5 所以-1,变成5更合适
j =strlen(tmpMystr) - 1;//因为下标是从0开始的 -1,
//isspace若参数c为空格字符,则返回非0,否则返回0。
while(isspace(tmpMystr[i]) && tmpMystr[i] !='\0')
{
//当字符串不是空格的时候 进入循环体
//走到非空格的时候就不走了
i++;
}
while(isspace(tmpMystr[j]) && tmpMystr[j] !='\0')
{
//当字符串不是空格的时候 进入循环体
j--;
}
tmpCount = j-i+1;
*ncount = tmpCount;
return 0;
}
//取出字符串的前后空格,中间的不进行处理
int trimSpace(char *mystr,char *newStr)
{
char *tmpMystr = mystr;
int i = 0;
int j = 0;
int tmpCount = 0;
if(mystr == NULL || newStr == NULL)
{
return -1;
}
//假设是"123456" strlen=6 下标从0开始 就是0-5 所以-1,变成5更合适
j =strlen(tmpMystr) - 1;//因为下标是从0开始的 -1,
//isspace若参数c为空格字符,则返回非0,否则返回0。
while(isspace(tmpMystr[i]) && tmpMystr[i] !='\0')
{
//当字符串不是空格的时候 进入循环体
//走到非空格的时候就不走了
i++;
}
while(isspace(tmpMystr[j]) && tmpMystr[j] !='\0')
{
//当字符串不是空格的时候 进入循环体
j--;
}
tmpCount = j-i+1;
strncpy(newStr,tmpMystr+i/*i,shi */,tmpCount);
//这个地方特别容易漏掉
newStr[tmpCount]='\0';
return 0;
}
//取出字符串的前后空格,中间的不进行处理,不开辟新的内存空间
//前提是 这个内存空间可以被修改
int trimSpace_new(char *mystr)
{
char *tmpMystr = mystr;
int i = 0;
int j = 0;
int tmpCount = 0;
if(mystr == NULL)
{
return -1;
}
//假设是"123456" strlen=6 下标从0开始 就是0-5 所以-1,变成5更合适
j =strlen(tmpMystr) - 1;//因为下标是从0开始的 -1,
//isspace若参数c为空格字符,则返回非0,否则返回0。
while(isspace(tmpMystr[i]) && tmpMystr[i] !='\0')
{
//当字符串不是空格的时候 进入循环体
//走到非空格的时候就不走了
i++;
}
while(isspace(tmpMystr[j]) && tmpMystr[j] !='\0')
{
//当字符串不是空格的时候 进入循环体
j--;
}
tmpCount = j-i+1;
strncpy(mystr,tmpMystr+i/*i,是因为前边的空格 */,tmpCount);
//这个地方特别容易漏掉
tmpMystr[tmpCount]='\0';
*mystr = *tmpMystr;
return 0;
}
int main(int argc, char *argv[])
{
char *p= " abcd123 45 ";//求字符串中非空格的次数,这个字符串再内存的全局区
char buf[1024] = " abcd123 45 ";
int i = 0;
int j = 0;
int ncount = 0;
char *myNewStr = (char*)malloc(100);
//假设是"123456" strlen=6 下标从0开始 就是0-5 所以-1,变成5更合适
j =strlen(p) - 1;//因为下标是从0开始的 -1,
//isspace若参数c为空格字符,则返回非0,否则返回0。
while(isspace(p[i]) && p[i] !='\0')
{
//当字符串不是空格的时候 进入循环体
//走到非空格的时候就不走了
i++;
}
while(isspace(p[j]) && p[j] !='\0')
{
//当字符串不是空格的时候 进入循环体
j--;
}
ncount = j-i+1;
printf("ncount:%d\n",ncount);
printf("----------------------------------\n");
getCount(p,&ncount);
printf("ncount:%d\n",ncount);
printf("----------------------------------\n");
trimSpace(p,myNewStr);
printf("myNewStr:%s\n",myNewStr);
printf("----------------------------------\n");
// trimSpace_new(p);//p只能读不能写 出错。。。。。
// printf("NewStr:%s\n",p);
trimSpace_new(buf);//buf可读可写
printf("NewStr:%s\n",buf);
printf("Hello World!\n");
return 0;
}
3、字符串反转模型
#include <stdio.h>
#include<string.h>
#include<stdlib.h>
//全局变量
int g_buf[100];
int inverse01(char *mystr)
{
int length = strlen(mystr);
char *p1 = mystr;//指向开头
char *p2 = mystr+ length -1;//指向结尾
while(p1<p2)
{
char c = *p1;
*p1 = *p2;
*p2 = c;
++p1;
--p2;
}
}
int main01(int argc, char *argv[])
{
char buf[]= "abcd12345";//字符串反转
inverse01(buf);
printf("buf:%s\n",buf);
printf("Hello World!\n");
return 0;
}
//通过递归的方式 逆向打印
//递归和全局变量(把逆序的结果放入全局变量)
//递归和非局变量(递归指针做函数参数)
//递归比较重要的2个点
//参数的入栈模型
//函数的嵌套调用返回流程
int inverse02(char *mystr)
{
if(mystr == NULL)//递归结束的异常条件
{
return -1;
}
if(*mystr == '\0')//递归结束的条件
{
return;
}
inverse02(mystr + 1);//此时没有执行打印,而是执行了 让 a b c d入栈
printf("%c ",*mystr);
return 0;
}
int inverse03(char *mystr)//把逆序的结果返回出来
{
if(mystr == NULL)//递归结束的异常条件
{
return -1;
}
if(*mystr == '\0')//递归结束的条件
{
return;
}
inverse02(mystr + 1);//此时没有执行打印,而是执行了 让 a b c d入栈
// printf("%c ",*mystr);
strncat(g_buf,mystr,1);//C库函数strcat()函数的功能是实现字符串的拼接
return 0;
}
int main03(int argc, char *argv[])
{
char buf[]= "abcd12345";//字符串反转
memset(g_buf,0,sizeof(g_buf));
// inverse02(buf);
// printf("buf02:%s\n",buf);
inverse03(buf);
printf("%s\n",g_buf);
printf("Hello World!\n");
return 0;
}
int inverse04(char *mystr,char *buf123)//把逆序的结果返回出来
{
if(mystr == NULL||buf123 == NULL)//递归结束的异常条件
{
return -1;
}
if(*mystr == '\0')//递归结束的条件
{
return;
}
inverse04(mystr + 1,buf123);//此时没有执行打印,而是执行了 让 a b c d入栈
// printf("%c ",*mystr);
strncat(buf123,mystr,1);//C库函数strcat()函数的功能是实现字符串的拼接
return 0;
}
int main(int argc, char *argv[])
{
char buf[]= "abcd12345";//字符串反转
memset(g_buf,0,sizeof(g_buf));
{
char mybuf[1024]={0};
inverse04(buf,mybuf);
printf("%s\n",mybuf);
}
printf("Hello World!\n");
return 0;
}