字符串replaceall
With the most recent version of v8, we now have several new JavaScript features available in all major browsers — one of which is String.prototype.replaceAll
. It is used for replacing all occurrences of a given string or a regular expression with another string. It looks like this:
在最新版本的v8中,我们现在在所有主流浏览器中都提供了一些新JavaScript功能-其中之一是String.prototype.replaceAll
。 它用于用另一个字符串替换所有出现的给定字符串或正则表达式。 看起来像这样:
'lorem ipsum dolor sit amet'.replaceAll(' ', '-');
// -> 'lorem-ipsum-dolor-sit-amet'
It is a very simple addition to String.prototype.replace
. Being the micro-optimization geek that I am, I decided to take a look at how this new feature performs compared to its alternatives. The purpose of this article, therefore, is to showcase this new feature and encourage the readers to approach new features from a different perspective.
这是String.prototype.replace
的非常简单的补充。 作为我的微优化专家,我决定看一下该新功能与其替代产品相比的性能。 因此,本文的目的是展示此新功能,并鼓励读者从不同的角度使用新功能。
到目前为止,替代方案是什么? (What Were the Alternatives So Far?)
Dating back to the early days of JavaScript, the prototype of String
provided us with a function called replace
that essentially does the same thing as replaceAll
, but it replaces only the first occurrence of the searchValue
. Example:
String
的原型可以追溯到JavaScript的早期,它为我们提供了一个称为replace
的函数,该函数本质上与replaceAll
相同,但仅替换了第一次出现的searchValue
。 例:
'lorem ipsum dolor sit amet'.replace(' ', '-');
// -> 'lorem-ipsum dolor sit amet'
This is useful but undesirable if you want to replace all occurrences of a text. One solution to that problem is to use a RegExp to define the searchValue
. The same example as above can easily be rewritten with a RegExp to achieve the same behavior as replaceAll
:
如果您要替换所有出现的文本,这很有用,但不是所希望的。 解决该问题的一种方法是使用RegExp定义searchValue
。 可以使用RegExp轻松重写与上述相同的示例,以实现与replaceAll
相同的行为:
'lorem ipsum dolor sit amet'.replace(/ /g, '-');
// -> 'lorem-ipsum-dolor-sit-amet
This works well for our case. It is also obvious to anyone who has some basic knowledge of regular expressions.
这对于我们的情况很好。 对于任何具有正则表达式基础知识的人来说,这也是显而易见的。
Let’s make things a bit more complicated. Say we have a case where we need our searchValue
to be dynamic. For instance, assume that we get our searchValue
as a parameter instead of hardcoding it. In this case, creating a regular expression from this variable gets a little more complicated. An example:
让我们做些复杂的事情。 假设有一种情况,我们需要我们的searchValue
是动态的。 例如,假设我们将searchValue
作为参数而不是对其进行硬编码。 在这种情况下,从该变量创建正则表达式会变得更加复杂。 一个例子:
function removeFromText(text, strToBeRemoved) {
return text.replace(new RegExp(strToBeRemoved, 'g'), '');
}
This works. What the code does is also still quite clear. But generating a regular expression dynamically is not always straightforward. Special characters in the given string can easily mess with the regular expression:
这可行。 代码的作用还很清楚。 但是动态生成正则表达式并不总是那么简单。 给定字符串中的特殊字符很容易与正则表达式混淆:
removeFromText('lorem ipsum dolor sit amet', ' ');
// -> 'loremipsumdolorsitamet' ✅ works
removeFromText('lorem+ipsum+dolor+sit+amet', '+');
// -> throws error ❌
removeFromText('lorem+ipsum+dolor+sit+amet', '+');
// -> still throws error ❌
removeFromText('lorem+ipsum+dolor+sit+amet', '\\+');
// -> 'loremipsumdolorsitamet' ✅ works
It still works, but the way it works is a lot more cryptic now. The users of the function must know that they have to sanitize the string before passing it to the function. The other alternative to this is to have removeFromText()
sanitize the received input. In that case, however, we make the implementation of removeFromText()
unnecessarily complicated.
它仍然可以工作,但是现在它的工作方式变得更加神秘。 函数的用户必须知道在将字符串传递给函数之前必须清理字符串。 另一个替代方法是让removeFromText()
清理接收到的输入。 但是,在这种情况下,我们使removeFromText()
的实现不必要地变得复杂。
The last alternative to solve the problems described above is to chain .split()
and .join()
to achieve the same behavior as replaceAll
:
解决上述问题的最后一个选择是链接.split()
和.join()
以实现与replaceAll
相同的行为:
function removeFromText(text, strToBeRemoved) {
return text.split(strToBeRemoved).join('');
}
removeFromText('lorem+ipsum+dolor+sit+amet', '+');
// -> 'loremipsumdolorsitamet' ✅
Now this one covers all our use cases. The drawback of this one is that what the code does is a lot less obvious. Splitting a string into an array only to merge it again seems wasteful and doesn’t tell us anything about replacing a string. It is more of a hack than doing what we want to do directly.
现在,这涵盖了我们所有的用例。 这样做的缺点是代码所做的事情不那么明显。 仅将字符串拆分为数组以再次合并似乎是浪费的,并且没有告诉我们任何有关替换字符串的信息。 它比直接执行我们想做的更多的是破解。
For all these reasons, we now have our syntactic sugar replaceAll()
. I'd like to think that it should be more performant than its alternatives, considering that its closest alternative is creating and destroying an array. So I ran some benchmarks.
由于所有这些原因,我们现在有了语法糖replaceAll()
。 考虑到最接近的替代方案是创建和销毁数组,我想认为它应该比替代方案更具性能。 所以我跑了一些基准。
让我们比较一下性能 (Let’s Compare the Performance)
I started by generating a string that is 1,300 characters long using a Lorem Ipsum generator. Then I created test cases of several ways to replace all occurrences of a text in a string. I then used jsben.ch to benchmark these test cases. I did this on Chrome, Safari, and Firefox. The results are below:
我首先使用Lorem Ipsum生成器生成了一个1300个字符长的字符串。 然后,我创建了几种替换字符串中所有出现的文本的测试用例。 然后,我使用jsben.ch对这些测试用例进行基准测试。 我是在Chrome,Safari和Firefox上执行此操作的。 结果如下:
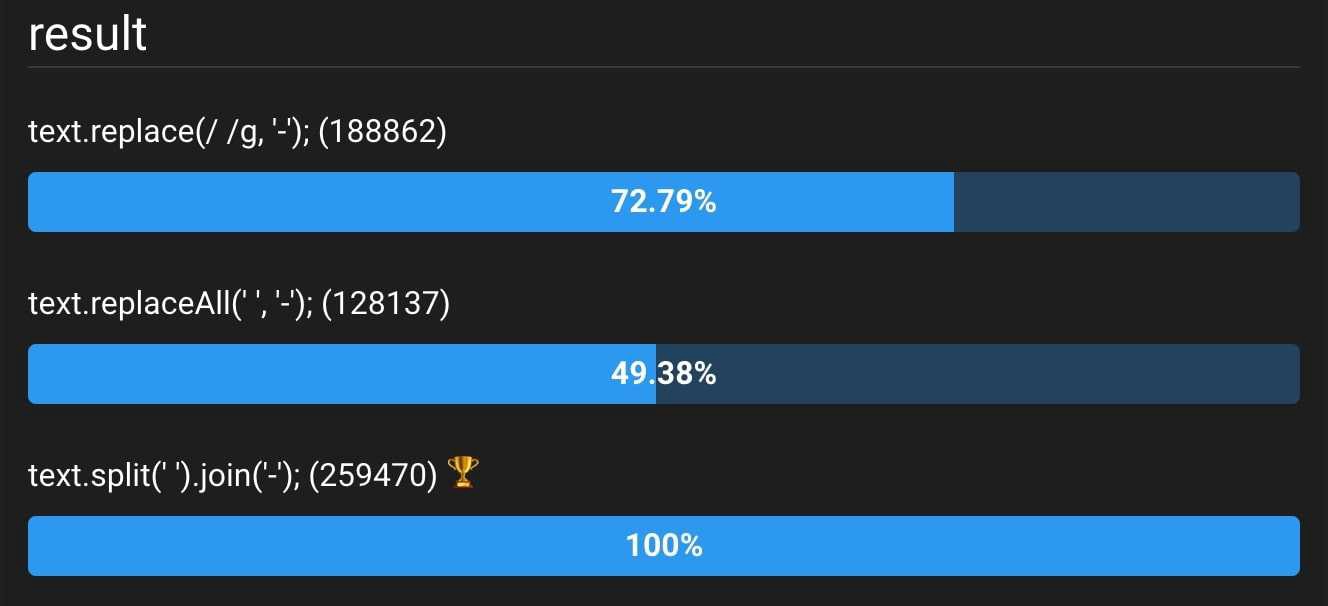
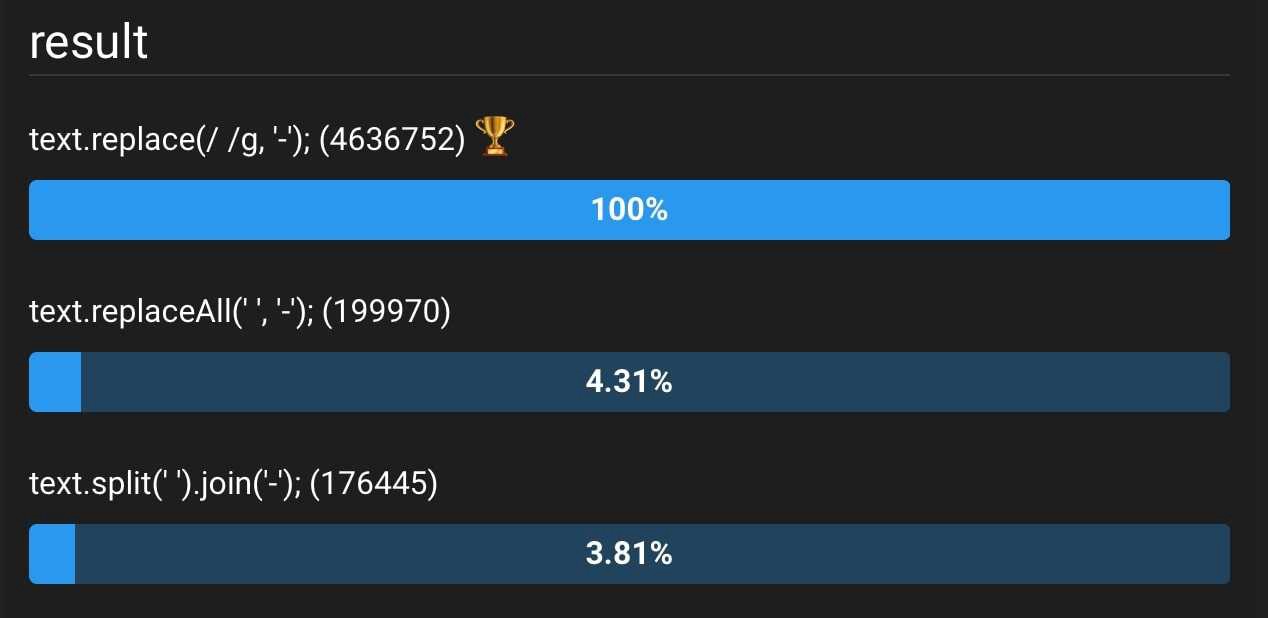

I was expecting replaceAll()
to be slightly faster than the alternatives. You can imagine that I was quite surprised to see that the results dramatically change for every browser. However, replaceAll()
is not the fastest in any of the browsers. It is quite disappointing to see that a feature that was added to be a helper function is actually slower than its alternatives.
我期望replaceAll()
比替代方法要快一些。 您可以想象,当每个浏览器的结果都发生巨大变化时,我感到非常惊讶。 但是, replaceAll()
在所有浏览器中都不是最快的。 看到添加为辅助功能的功能实际上比其替代功能慢的做法令人非常失望。
You can run the same benchmarks I ran to see for yourself.
判决 (Verdict)
So, should we refactor all our code?
那么,我们应该重构所有代码吗?
If your code is supposed to be so performant that you are performing micro-optimizations on your code, then you should probably use the alternatives instead. A super-performant code is often not needed when coding with JavaScript, but it can still happen if you are developing a utility library or a framework.
如果您的代码性能如此之高,以至于您正在对代码执行微优化,那么您可能应该使用替代方法。 使用JavaScript进行编码时,通常不需要高性能代码,但是如果您正在开发实用程序库或框架,它仍然可能会发生。
If you are developing for a user-facing application, however, your code would be more readable if you used more obvious functions to achieve your goal. Therefore, it would be better to use replaceAll()
and similar helpers to make the purpose of your code more obvious to the readers.
但是,如果您正在开发面向用户的应用程序,则使用更明显的功能实现目标时,代码将更具可读性。 因此,最好使用replaceAll()
和类似的助手来使您的代码的目的对读者更加明显。
字符串replaceall