广度优先搜索算法生成攻击图
The default code that throttles logins in Laravel is very basic: it will throttle the combination of the device IP and the user ID (the email by default) if the login fails. That’s it.
Laravel中限制登录的默认代码是非常基本的:如果登录失败,它将限制设备IP和用户ID(默认为电子邮件)的组合。 而已。
Okay, that will work if someone tries “guess” the user password. But the problem with that implementation is that you can try with other users, since the throttling will start fresh new when you change the email. Rinse and repeat:
好的,如果有人尝试“猜测”用户密码,那将起作用。 但是该实现的问题在于您可以与其他用户一起尝试,因为当您更改电子邮件时,限制将重新开始。 冲洗并重复 :
Over 800 login attempts were made without triggering the application’s rate-limiting mechanism.
在不触发应用程序的速率限制机制的情况下,进行了800多次登录尝试 。
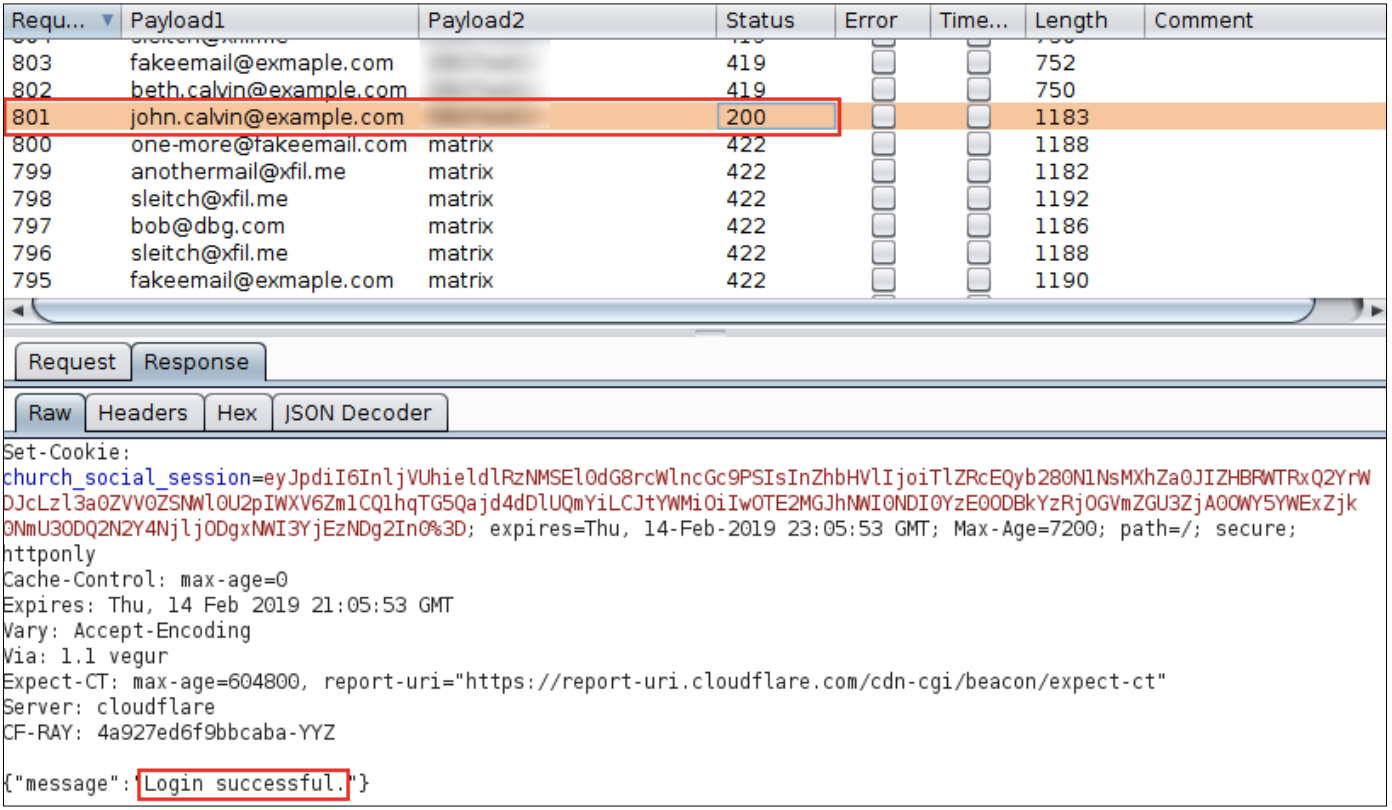
This is a big problem since there are a lot of bots that take email list with vulnerable passwords, navigate around the internet for applications, and check if the user has an account with the same password so it can be hijacked, or even attempt an elaborate phishing plan.
这是一个很大的问题,因为有许多漫游器会使用带有易受攻击的密码的电子邮件列表,在Internet上导航以查找应用程序以及检查用户是否拥有使用相同密码的帐户,从而使其能够被劫持,甚至尝试精心设计。网络钓鱼计划。
There are a couple of solutions, though.
不过,有两种解决方案。
解决方案A:限制IP (Solution A: Throttle the IP)
Since the problem with the throttling is the combination of the device IP plus the User ID, we can just eliminate the User ID from the equation. This will throttle the IP altogether.
由于节流的问题是设备IP加上用户ID的组合,因此我们只需从公式中消除用户ID。 这将完全限制IP。
Because some IP may be behind a NAT, this may throttle the whole network behind it because of some rogue device trying to force its way in, but personally that is a problem that cannot be tackled by you unless you use HTTP_X_FORWARDED_FOR
, and even then, is an unreliable header since it can be changed, while the real IP can’t (unless you’re behind a load balancer)
由于某些IP可能位于NAT之后,因此可能由于某些流氓设备试图强行HTTP_X_FORWARDED_FOR
而限制了其后的整个网络,但就个人而言,除非您使用HTTP_X_FORWARDED_FOR
,否则您HTTP_X_FORWARDED_FOR
,即使这样,是一个不可靠的标头,因为它可以更改,而真实IP则不能(除非您位于负载均衡器的后面)
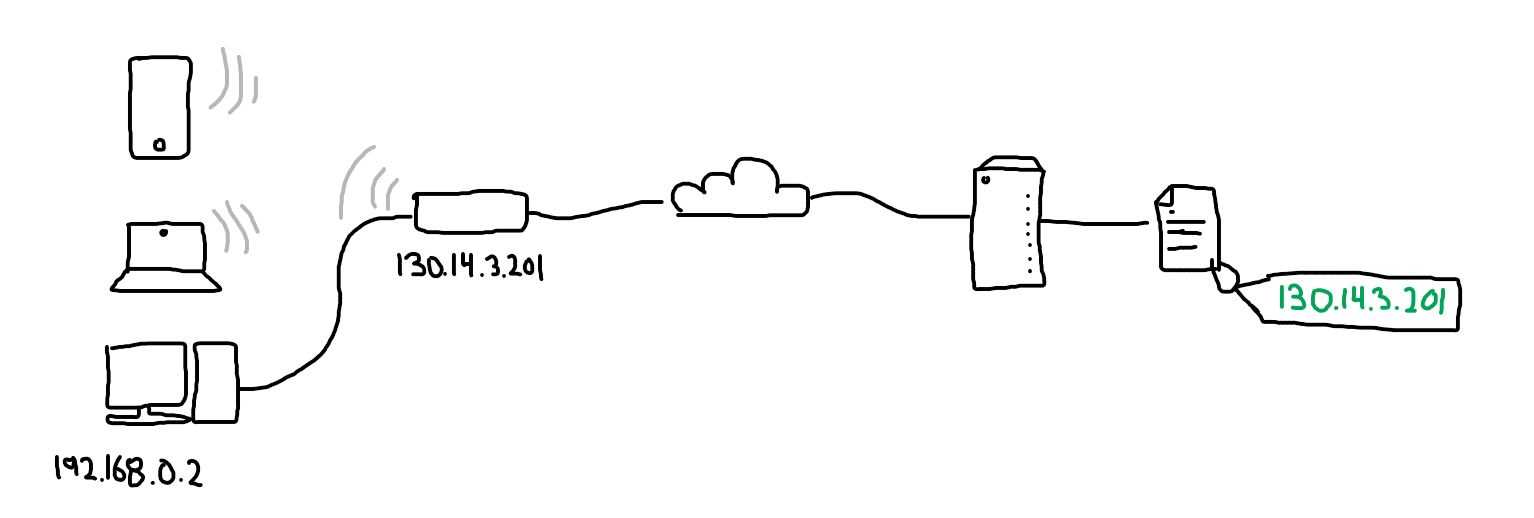
Anyway, assuming your app will be fine, we can just override the throttle key, which is conveniently returned by the throttleKey()
method, in your LoginController
:
无论如何,假设您的应用程序可以正常运行,我们就可以在自己的LoginController
重写节流键,该键可以由节流键throttleKey()
方法方便地返回:
/**
* Get the throttle key for the given request.
*
* @param \Illuminate\Http\Request $request
* @return string
*/
protected function throttleKey(Request $request)
{
return Str::lower(get_class($this). '|' . $request->ip());
}
We will use the get_class()
method to avoid throttling the whole application, but just the controller itself. You can also use the Request path using $request->path()
. At your discretion.
我们将使用get_class()
方法来避免限制整个应用程序,而只是限制控制器本身。 您还可以使用$request->path()
使用$request->path()
。 由您自行决定。
Now, consider this as a not sane option. Okay, it may work for IPv4 addresses, but for IPv6, you will have to have patience since there are more than 4.3 billion and someone could just renew its IP to anything else.
现在,将此视为不明智的选择。 好的,它可能适用于IPv4地址,但是对于IPv6,您将必须有耐心,因为有超过43亿,有人可以将其IP更新为其他任何内容。
解决方案B:提交重新验证码 (Solution B: Put a f*cking reCAPTCHA)
I’m gonna get yelled, but there is no other reliable solution apart from throttling the IP and stop a bot in their tracks. reCAPTCHA is a good way to protect your forms against bots, which in a default installation are handled by the Login, Registration, Verification Resend and Password Forgot controllers.
我会大喊大叫,但是除了节制IP并阻止机器人进入其轨道之外,没有其他可靠的解决方案。 reCAPTCHA是保护表单不受bot攻击的好方法,在默认安装中,bot是由Login , Registration , Verification Resend和Password Forgot控制器处理的。
While some may just put a reCAPTCHA checkbox in their forms, for me the most simple solution is to integrate Invisible reCAPTCHA (v2) and automatically bind it to the submit button of your page:
尽管有些人可能只是在表单中放置了reCAPTCHA复选框,但对我而言,最简单的解决方案是集成Invisible reCAPTCHA(v2)并将其自动绑定到页面的提交按钮:
The invisible reCAPTCHA badge does not require the user to click on a checkbox, instead it is invoked directly when the user clicks on an existing button on your site or can be invoked via a JavaScript API call. (…) By default only the most suspicious traffic will be prompted to solve a captcha.
不可见的reCAPTCHA徽章不需要用户单击复选框,而是在用户单击站点上的现有按钮时直接调用,也可以通过JavaScript API调用来调用。 (…)默认情况下,只会提示最可疑的流量来解决验证码。
Behind the scenes, you can just use the official reCAPTCHA PHP client to verify the challenge using a middleware:
在幕后,您可以使用官方reCAPTCHA PHP客户端使用中间件来验证挑战:
<?php
namespace App\Http\Controllers\Middleware;
use Closure;
use ReCaptcha\ReCaptcha;
use Illuminate\Validation\ValidationException;
class CheckReCaptcha
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
* @throws \Illuminate\Validation\ValidationException
*/
public function handle($request, Closure $next)
{
$recaptcha = new ReCaptcha(config('recaptcha.invisible.secret'));
$response = $recaptcha->verify($request->input('g-recaptcha-response'), $request->ip());
if (!$response->isSuccess()) {
throw ValidationException::withMessages([
'recaptcha' => 'Please solve the reCAPTCHA again'
])->redirectTo(back()->getTargetUrl());
}
return $next($request);
}
}
The Invisible reCAPTCHA will appear in the form only if the interaction is deemed suspicious, so you won’t need to do anything more than including the middleware into your routes:
仅当交互被视为可疑时,Invisible reCAPTCHA才会以表格的形式出现,因此您无需做任何事情,只需要在路由中包括中间件即可:
Route::post('login', 'LoginController@login')
->middleware('App\Http\Controllers\Middleware\CheckReCaptcha')
And walá. No more breadth-first attack.
和瓦拉。 不再需要广度优先攻击。
翻译自: https://medium.com/swlh/laravel-tackling-down-the-breadth-first-attack-141849da3bd
广度优先搜索算法生成攻击图