创建react应用程序
A basic guide to start using electron with React in minimum steps
以最少的步骤开始在React中使用电子的基本指南
Electron says that if you can build a website, you can build a cross-platform desktop app. Our beloved code editor VS Code uses electron, so does Whatsapp Desktop App. Electron uses Node.js runtime and Chromium rendering engine to build apps using HTML, CSS and JavaScript.
Electron说,如果您可以建立网站,则可以建立跨平台的桌面应用程序。 我们心爱的代码编辑器VS Code使用电子,Whatsapp Desktop App也是如此。 Electron使用Node.js运行时和Chromium渲染引擎来构建使用HTML,CSS和JavaScript的应用程序。
为什么要React (Why React)
React is an open-source JavaScript library for building user interfaces or UI components. Both functional(hooks) and class component approach is fun to learn. React is declarative and component based.
React是一个开放源代码JavaScript库,用于构建用户界面或UI组件。 功能(挂钩)和类组件方法都很有趣。 React是声明性的并且基于组件。
创建React应用 (Create-React-App)
To start building a new single page react application, React Community provides a boilerplate application to jump start app development.
为了开始构建新的单页React应用程序,React社区提供了一个样板应用程序来快速启动应用程序开发。
Note: Make sure you have Node.js installed in your system
注意:确保在系统中安装了Node.js
创建我们的React应用程序 (Creating our React Application)
With your CLI pointing to the folder you want to store your app, run
在CLI指向要存储应用程序的文件夹的情况下,运行
npx create-react-app electron-react-demo
which creates a new react application named electron-react-demo with required boilerplates.
这会创建一个新的React应用程序,该应用程序带有所需的样板,称为电子React演示。
cd electron-react-demo
npm start
to start your React app on localhost.
在本地主机上启动您的React应用程序。
You can now view electron-react-demo in the browser. Local: http://localhost:3000
现在,您可以在浏览器中查看电子React演示。 本地: http:// localhost:3000
The folder structure of electron-react-demo will be:
电子React演示的文件夹结构为:
electron-react-demo ├── README.md ├── node_modules ├── package.json ├── .gitignore ├── public │ ├── favicon.ico │ ├── index.html │ └── manifest.json └── src │├── App.css │├── App.js │├── App.test.js │├── index.css │├── index.js │├── logo.svg │├── serviceWorker.js └── setupTests.js
电子React演示├──README.md├──node_modules├──package.json├──.gitignore├──public│├──favicon.ico│├──index.html│└──清单。 json└──src│├──App.css│├──App.js│├──App.test.js│├──index.css│├──index.js│├──logo.svg│ ├──serviceWorker.js└──setupTests.js
安装电子 (Installing Electron)
We install electron as a dev-dependency
我们将电子安装为开发依赖
npm install -D electron
In src folder we create a new file electron.js to launch the electron application
在src文件夹中,我们创建一个新文件electron.js以启动电子应用程序
const electron = require('electron');
const app = electron.app;
const BrowserWindow = electron.BrowserWindow;let mainWindow;function createWindow() {mainWindow = new BrowserWindow({
width: 1024,
height: 768,
webPreferences:{
nodeIntegration:true
}
});mainWindow.loadURL('http://localhost:3000');mainWindow.webContents.openDevTools();mainWindow.on('closed', function () {
mainWindow = null
})}app.on('ready', createWindow);app.on('window-all-closed', function () {
if (process.platform !== 'darwin') {
app.quit()
}
});app.on('activate', function () {
if (mainWindow === null) {
createWindow()
}
});
Electron has two process: 1. Main process — electron.js launched on application start is the main process.2. Renderer process — BrowserWindows created in main process can access the renderer APIs for UI.
电子有两个过程:1.主要过程-在应用程序启动时启动的electronic.js是主要过程2。 渲染器进程—在主进程中创建的BrowserWindows可以访问UI的渲染器API。
The app API is used to control the event lifecycle of the application and creates window on event ‘ready’. BrowserWindow API is used to create and control browser windows. createWindow creates a new window with specified height and width and other relevant specifications. Open Chrome Developer tools using openDevTools(). Browser Window created listens for a ‘closed’ event to close the window. Browser Windows loads the localhost port on which the React app is running.
应用程序 API用于控制应用程序的事件生命周期,并在事件“就绪”上创建窗口。 BrowserWindow API用于创建和控制浏览器窗口。 createWindow创建一个具有指定高度和宽度以及其他相关规范的新窗口。 使用openDevTools()打开Chrome Developer工具。 创建的浏览器窗口侦听“ closed”事件以关闭窗口。 浏览器Windows会加载运行React应用程序的localhost端口。
在电子领域启动React应用 (Launching React Application in electron)
Add an entry point to electron
为电子添加入口点
We add a main entry point in package.json, which holds all the metadata related to the project.
我们在package.json中添加一个主入口点,其中包含与项目相关的所有元数据。
"main": "src/electron.js"
Also, to run electron we add a run target to electron in package.json,
另外,要运行电子,我们在package.json中向电子添加运行目标,
"electron":"electron ."
2. Running the below commands separately opens an Electron application with create-react-app content. We can make changes in App.js to edit the UI.
2.单独运行以下命令将打开一个带有create-react-app内容的Electron应用程序。 我们可以在App.js中进行更改以编辑UI。
npm start
npm run electron
Here, we have created an Desktop application with minimum effort for development. However, both React dev server and Electron must be launched separately and must be run in such a way that Electron is launched after react scripts are loaded.
在这里,我们创建了一个桌面应用程序,开发工作量最小。 但是,React开发服务器和Electron必须分别启动,并且必须以在加载React脚本后启动Electron的方式运行。
3. Using Foreman to simplify application launch
3.使用Foreman简化应用程序启动
Foreman is a process management tool, we add it using
工头是一种流程管理工具,我们使用
npm install -D foreman
We create a Procfile to manage the launch of two process i.e. React webpack-dev-server and electron as:
我们创建一个Procfile来管理两个进程的启动,即React webpack-dev-server和electronic作为:
react:npm start
electron:npm run electron
This solves our problem of separately launching the two processes. We add a new file named wait-for-react.js to deal with our issue waiting for React dev server to start and then launching electron.
这解决了我们分别启动两个过程的问题。 我们添加了一个名为wait-for-react.js的新文件来处理等待React开发服务器启动然后启动电子的问题。
// wait-for-react.js
const net = require('net');
const port = process.env.PORT ? (process.env.PORT - 100) : 3000;process.env.ELECTRON_START_URL = `http://localhost:${port}`;const client = new net.Socket();let startedElectron = false;
const tryConnection = () => client.connect({port: port}, () => {
client.end();
if(!startedElectron) {
console.log('starting electron');
startedElectron = true;
const exec = require('child_process').exec;
exec('npm run electron');
}
}
);tryConnection();client.on('error', (error) => {
setTimeout(tryConnection, 1000);
});
This simple node script checks if the React dev has started and then launches the electron process. We modify the earlier added Procfile
这个简单的节点脚本检查React开发人员是否已经启动,然后启动电子进程。 我们修改之前添加的Procfile
react:npm start
electron:npm run src/wait-for-react.js
4. Further, we make some changes to our package.json file:
4.此外,我们对package.json文件进行了一些更改:
Since, create-react-app builds an index.html that uses absolute paths by default. So, it fails to load it in Electron. However, there is a config option to change this, to set a homepage property in package.json. We set this property in current directory and npm run build will use this as a relative path.
从此以后, create-react-app会构建一个index.html ,它默认使用绝对路径。 因此,它无法将其加载到Electron中。 但是,有一个配置选项可以更改此设置,以在package.json中设置主页属性。 我们在当前目录中设置此属性, npm run build将使用此属性作为相对路径。
"homepage":"./"
2. npm start should launch our application as desired. However, currently this only launches the React dev server. Therefore, we add the following changes to package.json and Procfile respectively,
2. npm start应该根据需要启动我们的应用程序。 但是,当前这仅启动React开发服务器。 因此,我们分别将以下更改添加到package.json和Procfile中 ,
“start”: “nf start -p 3000”,
“electron-start”:”node src/wait-for-react”,
“react-start”:”react-scripts start”
Final Procfile,
最终Procfile,
react: npm run react-start
electron: npm run electron-start
These two complimentary changes, make us use npm start
to launch the application. Also, we add a .env file with BROWSER=none
in source directory to stop the React dev server to start in browser. Finally our package.json file is as
这两个互补的变化,使我们使用npm start
启动了应用程序。 另外,我们在源目录中添加一个BROWSER=none
的.env文件,以停止React开发服务器在浏览器中启动。 最后我们的package.json文件是
{
"name": "electron-react-demo",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^4.2.4",
"@testing-library/react": "^9.5.0",
"@testing-library/user-event": "^7.2.1",
"react": "^16.13.1",
"react-dom": "^16.13.1",
"react-scripts": "3.4.3"
},
"scripts": {
"start":"nf start -p 3000",
"react-start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject",
"electron": "electron ."
},
"eslintConfig": {
"extends": "react-app"
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
},
"homepage":"./",
"devDependencies": {
"electron": "^10.0.0",
"foreman": "^3.0.1"
},
"description": "This is a project demonstrating create-react-app with electron.",
"main": "src/electron.js",
"keywords": [
"electron",
"react"
],
"author": "*******@bobble.ai",
"license": "ISC"
}
Now, run npm start
and there, our electron app with React Front end is running.
现在,运行npm start
,然后运行带有React Front end的电子应用程序。
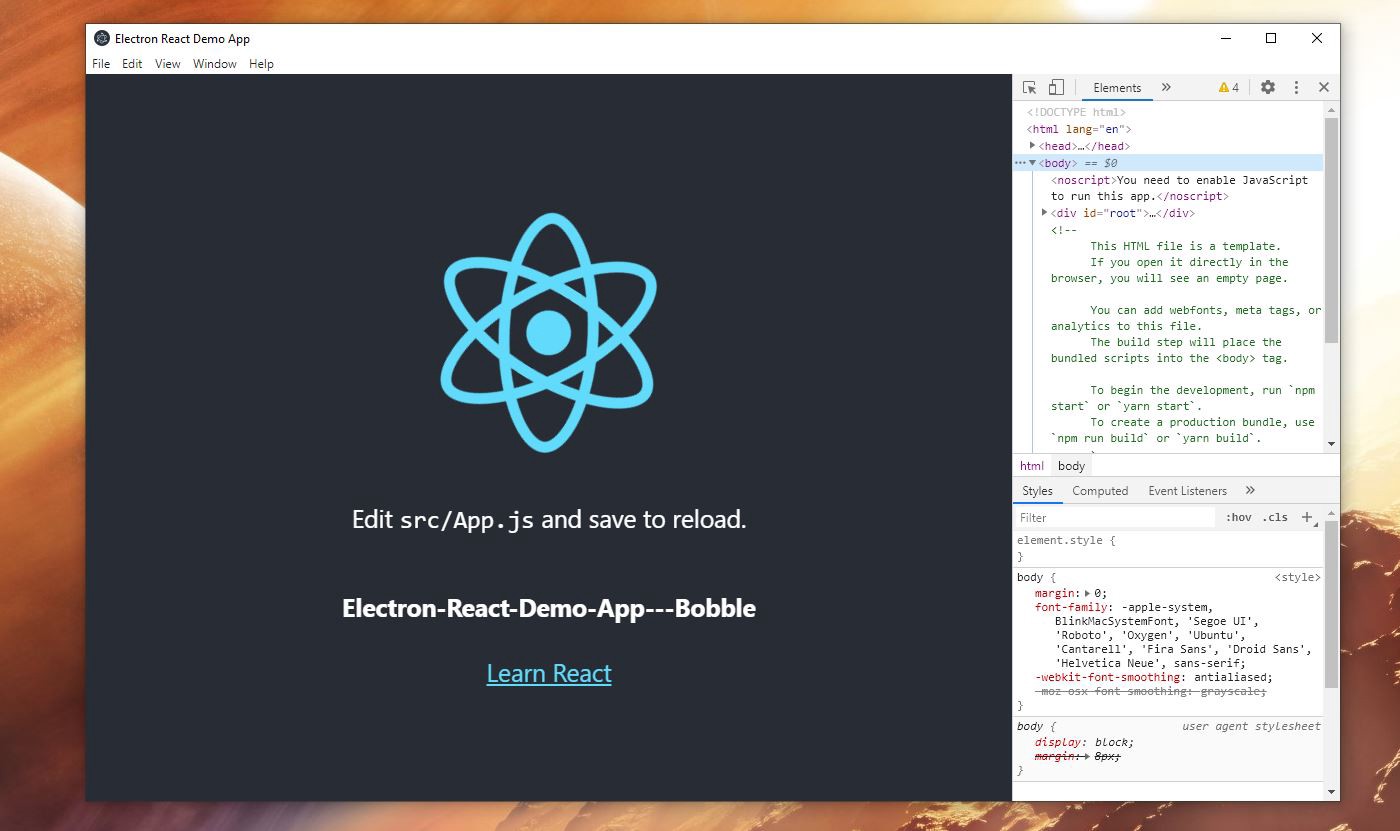
To access the file system or to use the Electron’s ipcRenderer, we need to import Electron in the React app with the following workaround,
要访问文件系统或使用Electron的ipcRenderer,我们需要使用以下解决方法在React应用程序中导入Electron,
const electron = window.require('electron');
In development, an environment variable ELECTRON_START_URL can specify the URL for mainWindow.loadURL
(in electron.js
).
在开发中,环境变量ELECTRON_START_URL可以指定mainWindow.loadURL
的URL(在electron.js
)。
In production, if the env var exists we use it, else we load the static index.html file.
在生产中,如果env var存在,则使用它,否则我们将加载静态index.html文件。
const startUrl = process.env.ELECTRON_START_URL || url.format({
pathname: path.join(__dirname, '/../build/index.html'),
protocol: 'file:',
slashes: true
});
mainWindow.loadURL(startUrl);
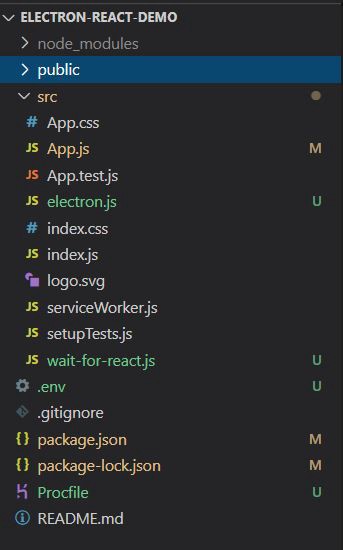
快来了 (Coming soon)
Electron packs a lot of APIs and has a lot to offer for developers e.g. creating frame-less Apps. Stay tuned for more exciting stories.
Electron打包了许多API,并为开发人员提供了许多功能,例如创建无框架的应用程序。 请继续关注更多激动人心的故事。
Thanks and Happy Coding!
谢谢,祝您编码愉快!
翻译自: https://medium.com/@ashish.sri/create-a-desktop-app-using-electron-and-react-6ab76e3935f2
创建react应用程序