颤振稳定性叶瓣图
Validating fields is common practice within apps, whether that be for simply checking that it is not empty or for determining whether the value entered or selected is valid based on specific criteria. From an end user’s perspective, validation errors can sometimes be ambiguous. Therefore, it is important for the app to let the user know exactly why something has gone wrong!
验证字段是应用程序中的常见做法,无论是简单地检查字段是否为空还是根据特定条件确定输入或选择的值是否有效。 从最终用户的角度来看,验证错误有时可能是模棱两可的。 因此,对于应用程序而言,重要的是要让用户确切地知道出了什么问题了!
The BLoC package for Flutter offers a fantastic state management approach that facilitates both testable and maintainable code.
Flutter的BLoC软件包提供了一种出色的状态管理方法,可简化可测试和可维护的代码。
We are going to use this BLoC pattern to add some validation logic to this very simple Flutter UI, which contains an email field and a submit button.
我们将使用此BLoC模式向此非常简单的Flutter UI添加一些验证逻辑,该UI包含一个电子邮件字段和一个提交按钮。
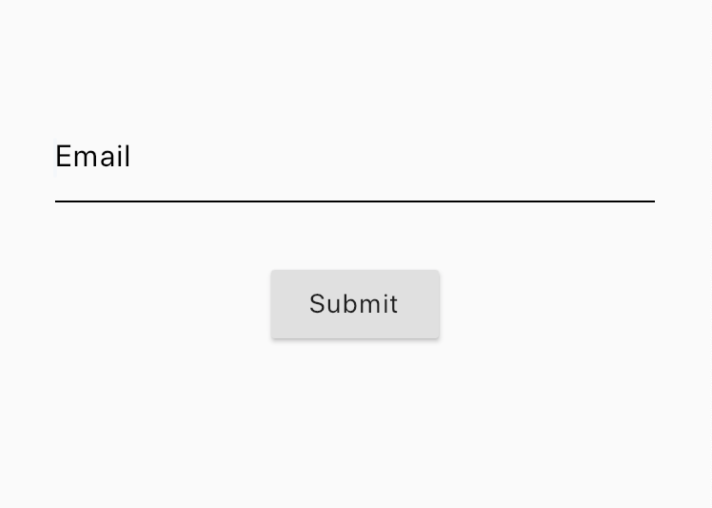
In this case, this email field is going to be considered valid if both of the following conditions are met:
在这种情况下,如果同时满足以下两个条件,则此电子邮件字段将被视为有效:
- It is not empty 它不是空的
- It matches our email validation regex 它与我们的电子邮件验证正则表达式匹配

These validation steps are going to take place within the BLoC itself. However due to their generic nature, we are going to separate this logic out into a Mixin so that we can reuse it in other BLoCs. (Email validation regex can be found here)
这些验证步骤将在BLoC本身内进行。 但是,由于它们的通用性,我们将把这个逻辑分离成一个Mixin,以便我们可以在其他BLoC中重用它。 (可在此处找到电子邮件验证正则表达式)
mixin ValidationMixin {
bool isFieldEmpty(String fieldValue) => fieldValue?.isEmpty ?? true;
bool validateEmailAddress(String email) {
if (email == null) {
return false;
}
return RegExp(
r'^(([^<>()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$')
.hasMatch(email);
}
}
The field’s value is then going to be validated through these functions and if it is not valid for whatever reason, we want to raise an error that is going to be displayed on our UI. However we do not want the BLoC to dictate exactly what the UI should render, instead it should just notify the UI that an error has occurred and it can then handle this accordingly.
然后,将通过这些函数来验证该字段的值,如果由于某种原因该字段的值无效,我们将引发一个错误,该错误将显示在我们的UI上。 但是,我们不希望BLoC确切指示UI应该呈现的内容,而只是通知UI发生了错误,然后BLoC可以相应地进行处理。
Therefore, we are going to create an enum to represent the state of the field, which can then be added to the BLoC’s state, this enum will simply have 2 values; Empty or Invalid.
因此,我们将创建一个表示字段状态的枚举,然后可以将其添加到BLoC的状态,该枚举将仅具有2个值; 为空或无效 。
enum FieldError { Empty, Invalid }
To begin the BLoC creation, we are then going to create the events that the UI is going to emit and the state that it will be receiving back.
为了开始BLoC的创建,我们将创建UI将要发出的事件以及它将被接收回的状态。
The only event that is required in this case, is a simple submit event that will send the text entered into the email field to the BLoC.
在这种情况下,唯一需要的事件是一个简单的提交事件,它将把在电子邮件字段中输入的文本发送到BLoC。
abstract class FormScreenEvent {}
class FormScreenEventSubmit extends FormScreenEvent {
final String email;
FormScreenEventSubmit(this.email);
}
The state will hold the error (if applicable) for the email field represented by the FieldError enum and a boolean to indicate if the submission event was successful. Finally, an isBusy boolean is used to notify the UI that the BLoC is processing data. This is a useful flag to have throughout an app and is therefore often abstracted to base classes that states can inherit from.
该状态将保留由FieldError枚举表示的电子邮件字段的错误(如果适用)和一个布尔值,以指示提交事件是否成功。 最后,一个isBusy布尔值用于通知UI BLoC正在处理数据。 这是整个应用程序中有用的标志,因此通常抽象为状态可以继承的基类。
class FormScreenState {
final bool isBusy;
final FieldError emailError;
final bool submissionSuccess;
FormScreenState({
this.isBusy: false,
this.emailError,
this.submissionSuccess: false,
});
}
We are now going to build out the BLoC itself, which is going to handle the events emitted from the UI and yield states back for the UI to respond to.
现在,我们将构建BLoC本身,该BLoC本身将处理从UI发出的事件并产生状态以供UI响应。
class FormScreenBloc extends Bloc<FormScreenEvent, FormScreenState>
with ValidationMixin {
FormScreenBloc();
@override
FormScreenState get initialState => FormScreenState();
@override
Stream<FormScreenState> mapEventToState(FormScreenEvent event) async* {
if (event is FormScreenEventSubmit) {
yield FormScreenState(isBusy: true);
if (this.isFieldEmpty(event.email)) {
yield FormScreenState(emailError: FieldError.Empty);
return;
}
if (!this.validateEmailAddress(event.email)) {
yield FormScreenState(emailError: FieldError.Invalid);
return;
}
yield FormScreenState(submissionSuccess: true);
}
}
}
This BLoC first yields a state that indicates that it is busy processing the data, such that the UI can inform the user that an action is taking place. After this, it proceeds to validate that the email is both not empty and matches the validation regex using the methods in the mixin. If the BLoC determines that the email is not valid it will yield FieldErrors accordingly, otherwise a success state is yielded.
此BLoC首先产生一个状态,指示其正在忙于处理数据,以便UI可以通知用户正在执行操作。 此后,它将继续使用mixin中的方法来验证电子邮件是否不为空并且是否与验证正则表达式匹配。 如果BLoC确定电子邮件无效,它将相应地产生FieldErrors ,否则产生成功状态。
Finally, it is time to tie this together with the UI!
最后,是时候将其与UI结合起来了!
class _FormScreenState extends State<FormScreen> {
FormScreenBloc _bloc;
final _emailController = TextEditingController();
@override
void initState() {
this._bloc = FormScreenBloc();
super.initState();
}
@override
void dispose() {
_emailController.dispose();
_bloc.close();
super.dispose();
}
@override
Widget build(BuildContext context) {
return BlocListener<FormScreenBloc, FormScreenState>(
bloc: this._bloc,
listener: (context, state) {
if (state.submissionSuccess) {
showDialog(
context: context,
child: AlertDialog(
title: Text('Submission success!'),
content: Text("Your submission was a success"),
actions: [
FlatButton(
child: Text('OK'),
onPressed: () => Navigator.of(context).pop(),
),
]),
);
}
},
child: Scaffold(
body: Padding(
padding: EdgeInsets.symmetric(horizontal: 30),
child: Center(
child: BlocBuilder<FormScreenBloc, FormScreenState>(
bloc: this._bloc,
builder: (context, state) {
if (state.isBusy) {
return CircularProgressIndicator();
}
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextField(
controller: this._emailController,
style: TextStyle(
color: this._hasEmailError(state)
? Colors.red
: Colors.black,
),
decoration: InputDecoration(
hintText: 'Email',
labelStyle: TextStyle(
color: this._hasEmailError(state)
? Colors.red
: Colors.black,
),
hintStyle: TextStyle(
color: this._hasEmailError(state)
? Colors.red
: Colors.black,
),
enabledBorder: this._renderBorder(state),
focusedBorder: this._renderBorder(state),
),
),
if (this._hasEmailError(state)) ...[
SizedBox(height: 5),
Text(
this._emailErrorText(state.emailError),
style: TextStyle(color: Colors.red),
),
],
SizedBox(height: 30),
RaisedButton(
child: Text('Submit'),
onPressed: () => this._bloc.add(FormScreenEventSubmit(
this._emailController.text)),
),
]);
}),
),
),
),
);
}
UnderlineInputBorder _renderBorder(FormScreenState state) =>
UnderlineInputBorder(
borderSide: BorderSide(
color: this._hasEmailError(state) ? Colors.red : Colors.black,
width: 1),
);
bool _hasEmailError(FormScreenState state) => state.emailError != null;
String _emailErrorText(FieldError error) {
switch (error) {
case FieldError.Empty:
return 'You need to enter an email address';
case FieldError.Invalid:
return 'Email address invalid';
default:
return '';
}
}
}
At first glance it seems as though there is a lot going on here! So let’s break it down.
乍一看似乎这里发生了很多事情! 因此,让我们对其进行分解。
Firstly, the submit button is now emitting an event to the BLoC and is sending through the current text held by the controller for the email field. Clicking this button is now going to trigger the BLoC to perform the validation logic.
首先,“提交”按钮现在向BLoC发出事件,并正在通过控制器保留的电子邮件字段的当前文本进行发送。 现在单击此按钮将触发BLoC执行验证逻辑。
The BLoC builder is going to rebuild every time a new state is yielded from the BLoC. Therefore it is first checking whether the state is busy, and if so it shows a progress indicator to the user to improve the feeling of responsiveness in the app as it dictates to the user that operations are taking place.
每当BLoC产生新状态时,BLoC构建器都会重建。 因此,首先要检查状态是否忙碌,如果是,则向用户显示进度指示器,以改善应用程序中响应性的感觉,因为它指示用户正在执行操作。
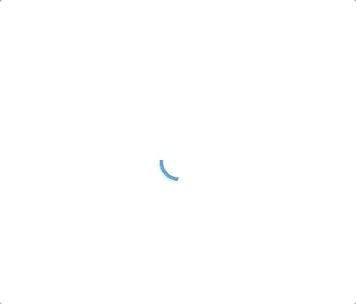
If the state is not busy however, the builder will render the form as shown earlier with one big difference… now if there is a validation error in the state, the email field’s appearance will change! This field will instead render as red to indicate an issue and a handy function is used to evaluate what error text should show below the field using the enum that we created! This new text field can easily be abstracted into its own reusable widget too.
但是,如果状态不忙,则构建器将呈现之前显示的表单,但有很大的不同……现在,如果状态中存在验证错误,则电子邮件字段的外观将发生变化! 该字段将改为显示为红色,以指示问题,并且使用方便的函数使用我们创建的枚举来评估应在字段下方显示哪些错误文本! 这个新的文本字段也可以轻松地抽象成它自己的可重用窗口小部件。
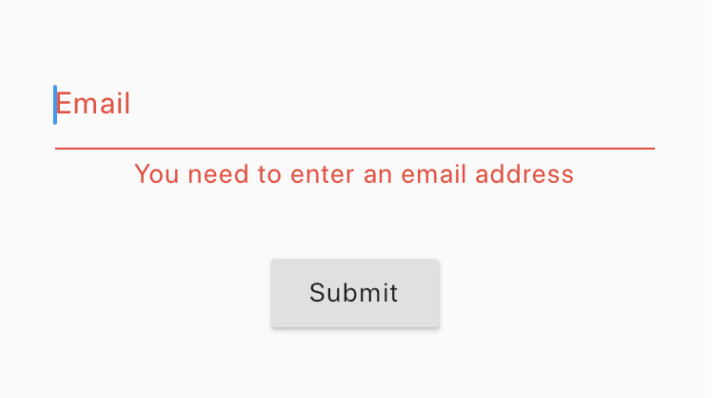

Lastly, the BLoC listener’s purpose here is to simply listen for whether the state indicates that there has been a submission success and to raise an alert dialog to the user to tell them that they have submitted a valid email!
最后,BLoC侦听器的目的是简单地侦听状态是否表明提交成功,并向用户发出警报对话框,告诉他们他们已经提交了有效的电子邮件!
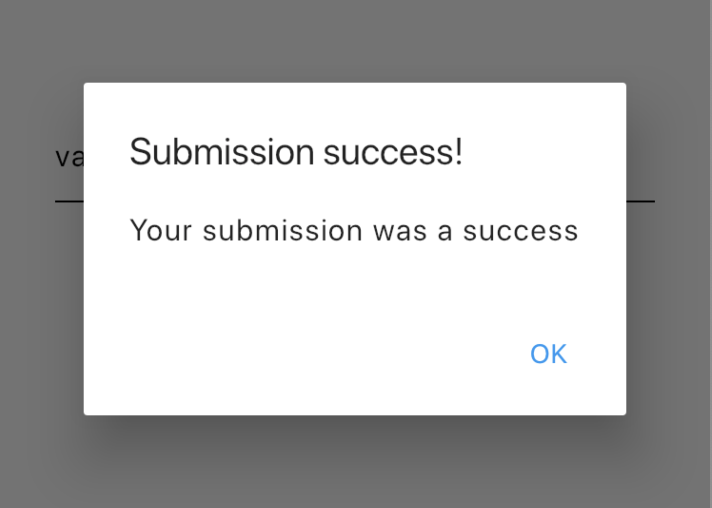
… and that’s it! We now have field validation in our app using the BLoC pattern!
…就是这样! 现在,我们使用BLoC模式在我们的应用程序中进行了字段验证!
If you’ve enjoyed this article, I have also published another on utilising the repository pattern effectively in Flutter.
如果您喜欢这篇文章,我还将发表另一篇有关在Flutter中有效利用存储库模式的文章。
The repo for this solution can be found at: https://github.com/luketg8 Validation_Example
可以在以下位置找到该解决方案的存储库: https : //github.com/luketg8 Validation_Example
翻译自: https://levelup.gitconnected.com/flutter-field-validation-using-bloc-pattern-19188076721d
颤振稳定性叶瓣图