对警报线程池的警报线程
This seemingly simple UI element has a lot of hidden features. If you have ever developed an application, you probably have used it.
这个看似简单的UI元素具有许多隐藏功能。 如果您曾经开发过应用程序,则可能已经使用过。
An AlertDialog’s main function is to notify users of something that is going to happen that requires their immediate attention and an action to be made on their end. The uses of an AlertDialog are numerous and could range from:
AlertDialog的主要功能是通知用户即将发生的事情,这些事情需要他们的立即注意并最终采取措施。 AlertDialog的用途很多,范围可能是:
- Ask the user to grant permission for your application to do something (location) 要求用户授予您的应用程序执行某项操作的权限(位置)
- Alert the user to an action that is about to take place (remove photo) 提醒用户即将执行的操作(删除照片)
- Notify the user of what is going on (feature not available) 通知用户正在发生的事情(功能不可用)
What this article will not cover is the basic use cases and construction of an AlertDialog, but it will cover some more advanced uses and caveats of this UI element.
本文将不介绍AlertDialog的基本用例和构造,但将介绍此UI元素的一些更高级的用法和注意事项。
For this article, I will be showing examples written in Kotlin.
对于本文,我将展示用Kotlin编写的示例。
AlertDialog标题的长度 (Length of AlertDialog’s Title)
Setting the title on an AlertDialog is easy. Using the following method,
在AlertDialog上设置标题很容易。 使用以下方法,
builder.setTitle(title), //where title is a CharSequence.
What is not apparent from the code, is that the dialog’s title is limited to only two lines. Meaning, that if you write a long title, it will be truncated and you will see three dots (…) being displayed signifying the sentence is longer than it appears.
从代码中看不出来的是,对话框的标题仅限于两行。 意思是,如果您写了一个长标题,它将被截断,并且您将看到显示三个点(…),表示该句子的长度超过了它的显示长度。
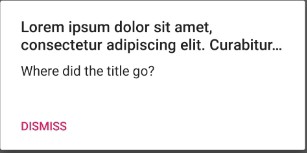
But what If we needed more room for our title? We can use the method setCustomTitle
which receives a View as a parameter.
但是,如果我们需要更多的标题空间呢? 我们可以使用setCustomTitle
方法,该方法接收一个View作为参数。
Using the above, we can easily create a TextView and style it to our liking to get the desired result.
使用上面的代码,我们可以轻松地创建一个TextView并根据自己的喜好对其进行样式设置,以获得所需的结果。
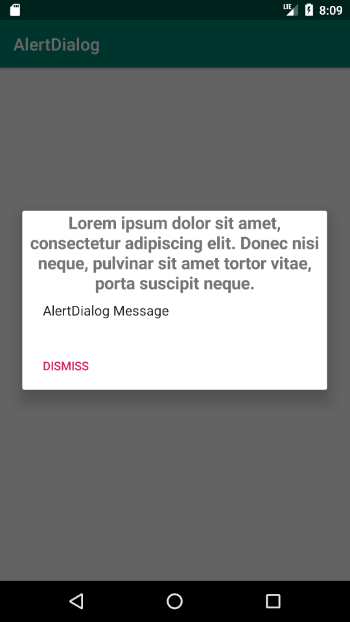
中立按钮 (The Neutral Button)
While you may be familiar with the approve or reject buttons associated with the AlertDialog, there is also one other type of button available. That button is the NeutralButton. What is special about this button is that it signals to the user that if he/she makes this choice, nothing will be affected.
尽管您可能熟悉与AlertDialog相关的批准或拒绝按钮,但还有另一种类型的按钮可用。 该按钮是NeutralButton 。 此按钮的特殊之处在于,它向用户发出信号,如果他/她做出了此选择,则不会受到任何影响。
If you take another look at the screenshot above, you can see that the button with the Dismiss text is a neutral button.
如果您再看一下上面的屏幕截图,您会看到带有“关闭”文本的按钮是一个中性按钮。
物品或讯息 (Items Or Message)
After setting the title, we can set the message of our alert dialog. Again, what if we wanted the user to select something in our alert dialog (out of a list)? For that, we can use the setItems method:
设置标题后,我们可以设置警报对话框的消息。 同样,如果我们希望用户在警报对话框中(从列表中)选择某些内容,该怎么办? 为此,我们可以使用setItems方法:
Or we can use the setSingleChoiceItems method:
或者我们可以使用setSingleChoiceItems方法:
Here, we come to a fork in the road, because we will have to choose what is more important to us: a list of items or the alert dialog’s message. We can’t have both. To quote the documentation:
在这里,我们走上了一条路,因为我们必须选择对我们更重要的内容:项目列表或警报对话框的消息。 我们不能两者兼得。 引用文档:
…Because the list appears in the dialog’s content area, the dialog cannot show both a message and a list…
…因为该列表出现在对话框的内容区域中,所以该对话框无法同时显示消息和列表…
When using an items list, it is a good idea to use an adapter to handle the interaction and management of our list of items. Below is a simple demonstration of just how to achieve that.
使用项目列表时,最好使用适配器来处理项目列表的交互和管理。 以下是如何实现该目标的简单演示。

If you don’t want to deal with an adapter, you can use the setSingleChoiceItems method like so:
如果您不想使用适配器,则可以使用setSingleChoiceItems方法,如下所示:
And you’ll get the following:
您将获得以下信息:
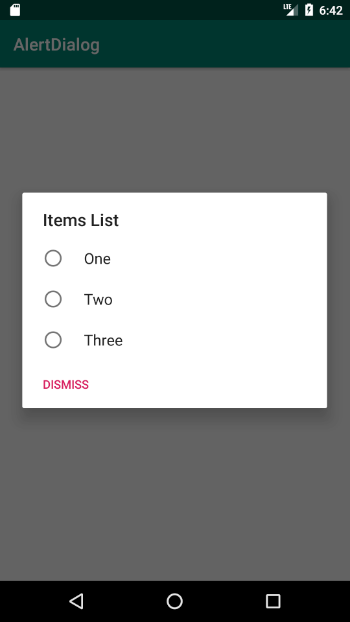
图像警报 (Image Alert)
What if we wanted to have a message and an image in our AlertDialog? We can achieve that by using the setView
method:
如果我们想在AlertDialog中显示消息和图像怎么办? 我们可以通过使用setView
方法来实现:
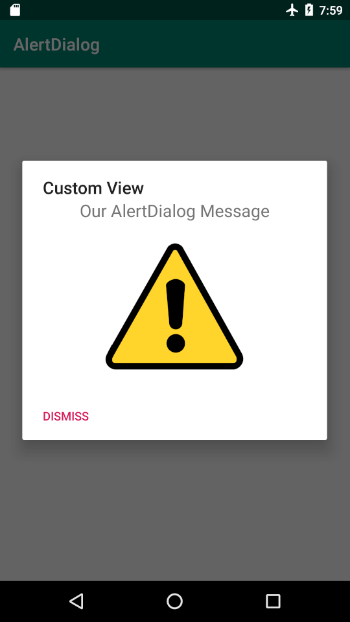
🙌 In fact, you may have noticed that even for the previous problem, we can use a custom view that has a listview and a textview to handle the scenario of a message and a list of items.
🙌实际上,您可能已经注意到,即使对于先前的问题,我们也可以使用具有listview和textview的自定义视图来处理消息和项目列表的情况。
Those are just some of the more allusive possibilities you have with the AlertDialog. If you have more examples like this, feel free to share them in the comments.
这些只是您使用AlertDialog所具有的更多暗示性可能性。 如果您有更多类似的示例,请随时在评论中分享。
You can see all the examples shown in this article in an application I created at this GitHub repository.
您可以在我在GitHub存储库中创建的应用程序中看到本文显示的所有示例。
翻译自: https://proandroiddev.com/what-you-might-not-know-about-the-alertdialog-2bdc55f3d907
对警报线程池的警报线程