参考链接:
https://github.com/MurphyWan/Python-first-Practice/blob/master/D2_of_3Day_DoneWithPython.md
最近在看一个前辈的代码,发现她用了这个库进行数据处理的操作。刚开始的时候是有点看不懂的,就尝试着学习一下这个包,争取能够看懂前辈的代码。
1、导入matplotlib模块
from matplotlib import pyplot as plt
matplotlib/pyplot/plt的关系是什么?
pyplot是matplotlib的子库,plt是pyplot的简写(也可以是其他简写形式),后续导入模块中的函数中的函数的时候,直接用plt,而不必写全pyplot。
2、准备数据
import numpy as np
x = np.linspace(0, 10, 200) #使用numpy库生成数列
a = np.zeros_like(x) #print the same count of vector x
y = x ** 0.5
3、简单绘图1
plt.figure(figsize = (6, 4)) #width,height
plt.plot(x, y,'g--') #绿色
plt.plot(x, x-1,'k') #黑色
plt.plot(x,a,'b-') #蓝色
plt.savefig("plot.png")
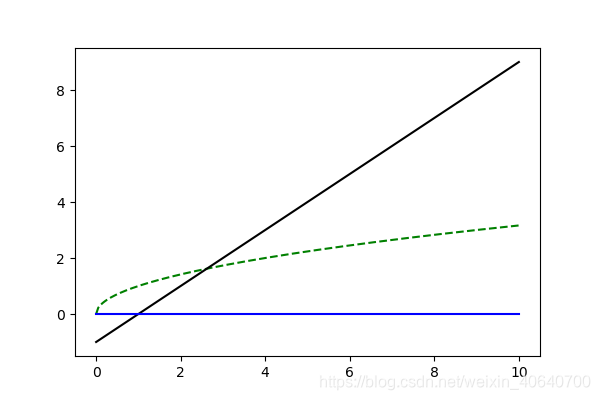
4、添加图例
x = np.linspace(0, 10, 200)
for n in range(2, 5):
y = x * n
plt.plot(x, y,label="x*"+str(n)) #label添加图例的标签
plt.legend(loc = "best") #图例的位置
plt.xlabel("X axis") #x坐标
plt.ylabel("Y axis") #y坐标
plt.xlim(-2, 10)
plt.title("Multi-plot e.g. ", fontsize = 18) #图的标题
plt.show()
plt.savefig("multi-plot.png")
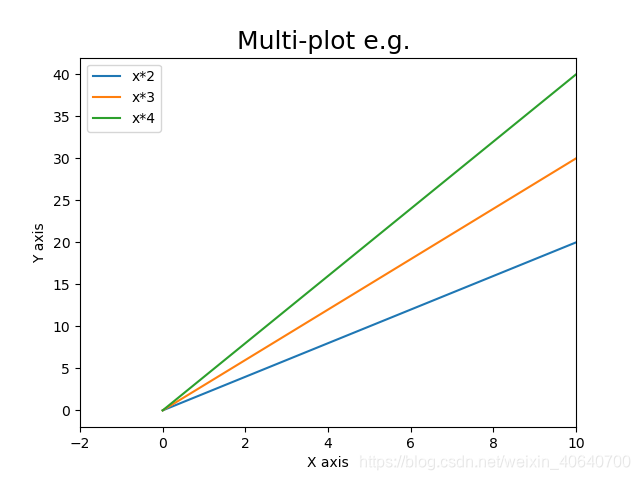
5、将画板分割给子图(使用fig.add_subplot()函数)
S = np.linspace(50, 151, 100)
fig = plt.figure(figsize=(12, 6))
#sub1 picture
sub1 = fig.add_subplot(121) # col, row, num
sub1.set_title('pic.A', fontsize = 18)
plt.plot(S,S*2, 'b-', lw = 2) #why not change? ylim!
#plt.plot(S, np.zeros_like(S), 'blue',lw = 4)
sub1.grid(True)
sub1.set_xlabel("control")
sub1.set_ylabel("expression")
sub1.set_xlim([60, 120])
sub1.set_ylim([60, 240])
#sub2 picture2
sub2 = fig.add_subplot(122)
sub2.set_title('pic.B', fontsize = 18)
plt.plot(S,S+2, 'r--', lw = 2)
#plt.plot(S, np.zeros_like(S), 'blue',lw = 4)
sub2.grid(True)
sub2.set_xlabel("tumor")
sub2.set_ylabel("expression")
sub2.set_xlim([60, 120])
sub2.set_ylim([60, 240])
plt.show()
*fig.add_subplot()函数括号中的数字是什么意思?
参考链接:https://www.jianshu.com/p/7b68e01952b4
如果数字是121,代表的是1*2的网格中的第1张图,对应地122,代表的就是1*2网格中的第2张图。
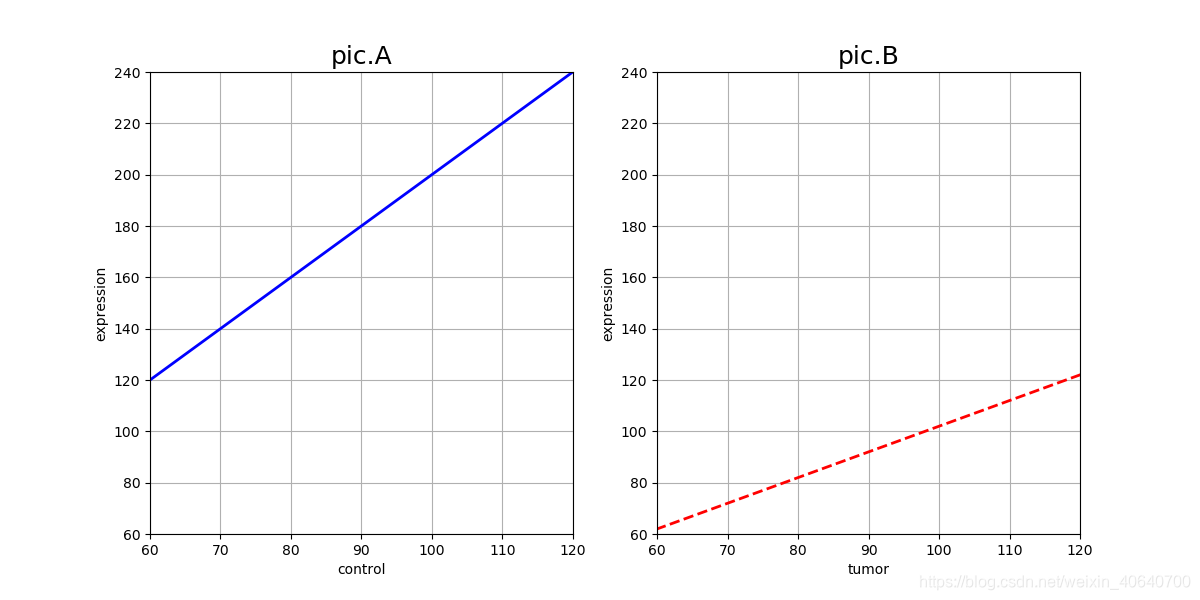
6、在图中添加注释(利用annotate函数)。
实例代码段(剖析这个函数中的各个属性什么意思):
ax.annotate("$\Delta$ hedge",
xy=(100, 0),
xytext=(110, -10),
arrowprops=dict(headwidth =3,
width = 0.5,
facecolor="black",
shrink=0.05
)
)
- "$\Delta$ hedge":刚开始的这个字符串代表的是要注释的文字。
- xy=(100,0):代表箭头指向的位置为横坐标100,纵坐标0。
- xytext=(110,-10):代表注释的文字的位置为横坐标110,纵坐标-10。
- arrowprops=dict():代表的是箭头的一些显示属性。headwidth表示箭头的头部的宽度,width表示箭头线的宽度,facecolor代表这个表示箭头身体的颜色。shrink箭头两端收缩的百分比(占总长),一般都是0.05。
参考链接:https://blog.csdn.net/leaf_zizi/article/details/82886755
利用上面的代码,给一张图作简单注释。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 200) #x?
y = x ** 0.5 #y?
fig = plt.figure(figsize = (6, 4)) #design the plot
fig1 = fig.add_subplot(111) #one picture
plt.plot(x,y,"g--") #draw the picture,use the plt.plot.
fig1.annotate("win", xy= \
(6,2.5),xytext\
=(7,2),\
arrowprops=dict(headwidth =5,\
width = 1, facecolor="black", \
shrink=0.05))
fig1.set_xlabel("counts")
fig1.set_ylabel("score")
fig1.set_title("Liming's score in this contest")
fig1.grid()
plt.show()
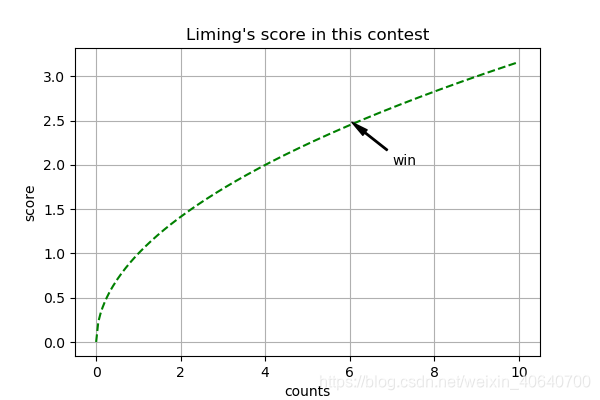
7、涉及到三个变量的三维作图(用的并不是很多)
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import cm
from mpl_toolkits.mplot3d import Axes3D
x, y = np.mgrid[-5:5:100j, -5:5:100j]
z = x**2 + y**2
fig = plt.figure(figsize=(8, 6))
ax = plt.axes(projection='3d')
surf = ax.plot_surface(x, y, z, rstride=1,\
cmap=cm.coolwarm, cstride=1, \
linewidth=0)
fig.colorbar(surf, shrink=0.5, aspect=5)
plt.title('3D plot of $z = x^2 + y^2$')
plt.show()
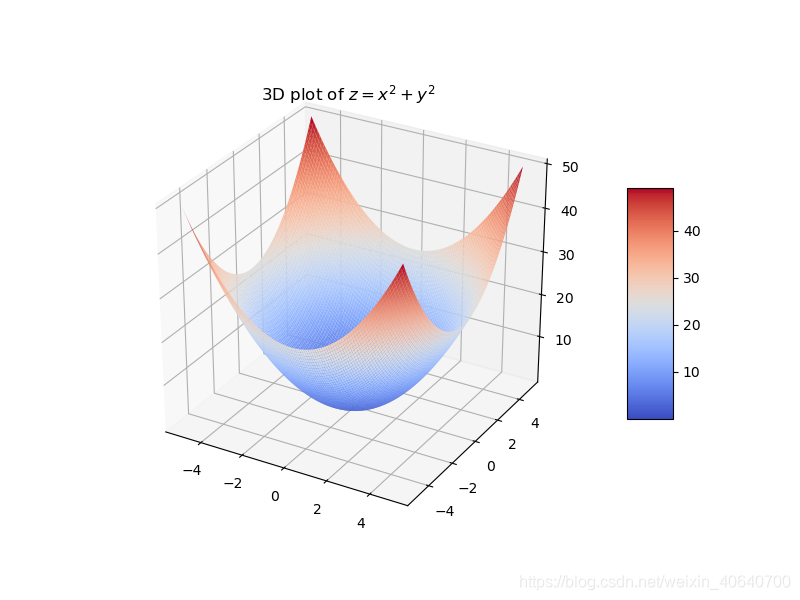
学到这里,基本上掌握了Matplotlib模块的基础操作。
对于这其中的一些细节,按照以后实际的需求再做更为深入的探究。