1.源代码
import pygame
import random
# 初始化游戏
pygame.init()
# 游戏窗口尺寸
WINDOW_WIDTH = 800
WINDOW_HEIGHT = 600
# 方块大小和颜色
BLOCK_SIZE = 30
COLORS = [
(0, 0, 0), # 黑色
(255, 0, 0), # 红色
(0, 255, 0), # 绿色
(0, 0, 255), # 蓝色
(255, 255, 0), # 黄色
(255, 165, 0), # 橙色
(0, 255, 255), # 青色
(128, 0, 128) # 紫色
]
# 初始化游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("俄罗斯方块")
# 定义游戏区域,使用二维列表表示,0代表空格
game_area = [[0] * (WINDOW_WIDTH
# 定义各种形状的俄罗斯方块
tetriminos = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 1], [1, 0, 0]],
[[1, 1, 1], [0, 0, 1]]
]
# 初始化当前俄罗斯方块的位置和形状
current_tetrimino = random.choice(tetriminos)
current_x = (WINDOW_WIDTH
current_y = 0
# 定义函数:绘制游戏区域
def draw_game_area():
for y in range(WINDOW_HEIGHT
for x in range(WINDOW_WIDTH
if game_area[y][x] != 0:
pygame.draw.rect(window, COLORS[game_area[y][x]], (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 定义函数:检查当前俄罗斯方块是否触底或碰撞到其他方块
def check_collision():
for y in range(len(current_tetrimino)):
for x in range(len(current_tetrimino[0])):
if current_tetrimino[y][x] == 1:
if current_y + y >= WINDOW_HEIGHT
return True
return False
# 定义函数:将当前俄罗斯方块放置到游戏区域
def place_tetrimino():
for y in range(len(current_tetrimino)):
for x in range(len(current_tetrimino[0])):
if current_tetrimino[y][x] == 1:
game_area[current_y + y][current_x + x] = len(COLORS) - 1
# 定义函数:消除满行
def clear_lines():
lines_cleared = 0
y = WINDOW_HEIGHT
while y >= 0:
if all(game_area[y]):
del game_area[y]
game_area.insert(0, [0] * (WINDOW_WIDTH
lines_cleared += 1
else:
y -= 1
return lines_cleared
# 游戏循环
running = True
clock = pygame.time.Clock()
fall_timer = 0
fall_interval = 1000 # 方块下落间隔,单位毫秒
score = 0
while running:
window.fill(0)
draw_game_area()
# 方块下落
current_time = pygame.time.get_ticks()
if current_time - fall_timer >= fall_interval:
fall_timer = current_time
current_y += 1
if check_collision():
current_y -= 1
place_tetrimino()
lines_cleared = clear_lines()
score += lines_cleared
if lines_cleared > 0:
fall_interval = max(fall_interval - 50, 200) # 加快方块下落速度
current_tetrimino = random.choice(tetriminos)
current_x = (WINDOW_WIDTH
current_y = 0
if check_collision():
running = False
# 处理用户输入事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_x -= 1
if check_collision():
current_x += 1
elif event.key == pygame.K_RIGHT:
current_x += 1
if check_collision():
current_x -= 1
elif event.key == pygame.K_DOWN:
current_y += 1
if check_collision():
current_y -= 1
elif event.key == pygame.K_UP:
# 旋转方块
rotated_tetrimino = []
for i in range(len(current_tetrimino[0])):
rotated_row = [current_tetrimino[j][i] for j in range(len(current_tetrimino) - 1, -1, -1)]
rotated_tetrimino.append(rotated_row)
if not check_collision():
current_tetrimino = rotated_tetrimino
# 绘制当前俄罗斯方块
for y in range(len(current_tetrimino)):
for x in range(len(current_tetrimino[0])):
if current_tetrimino[y][x] == 1:
pygame.draw.rect(window, COLORS[len(COLORS) - 1], ((current_x + x) * BLOCK_SIZE, (current_y + y) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
pygame.display.update()
clock.tick(60)
pygame.quit()
2.游戏运行
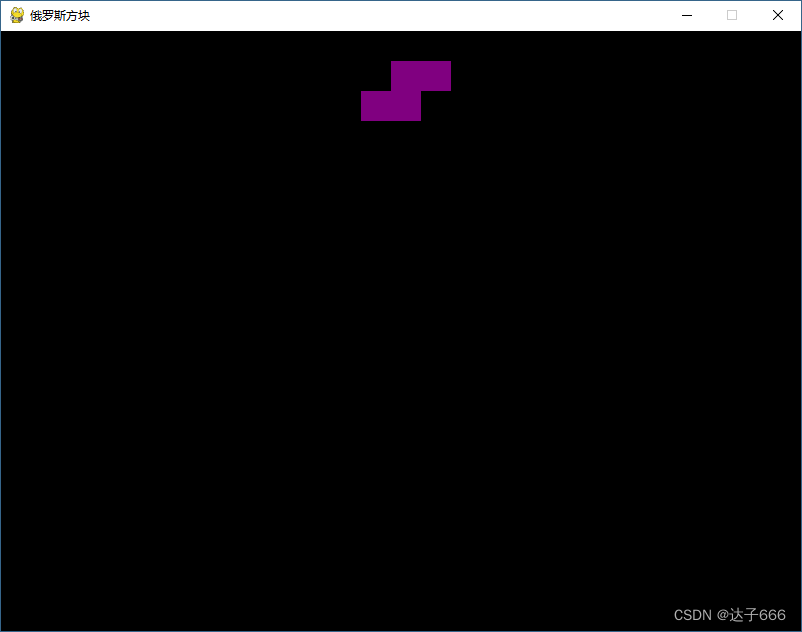