添加AI小人(蓝色的)
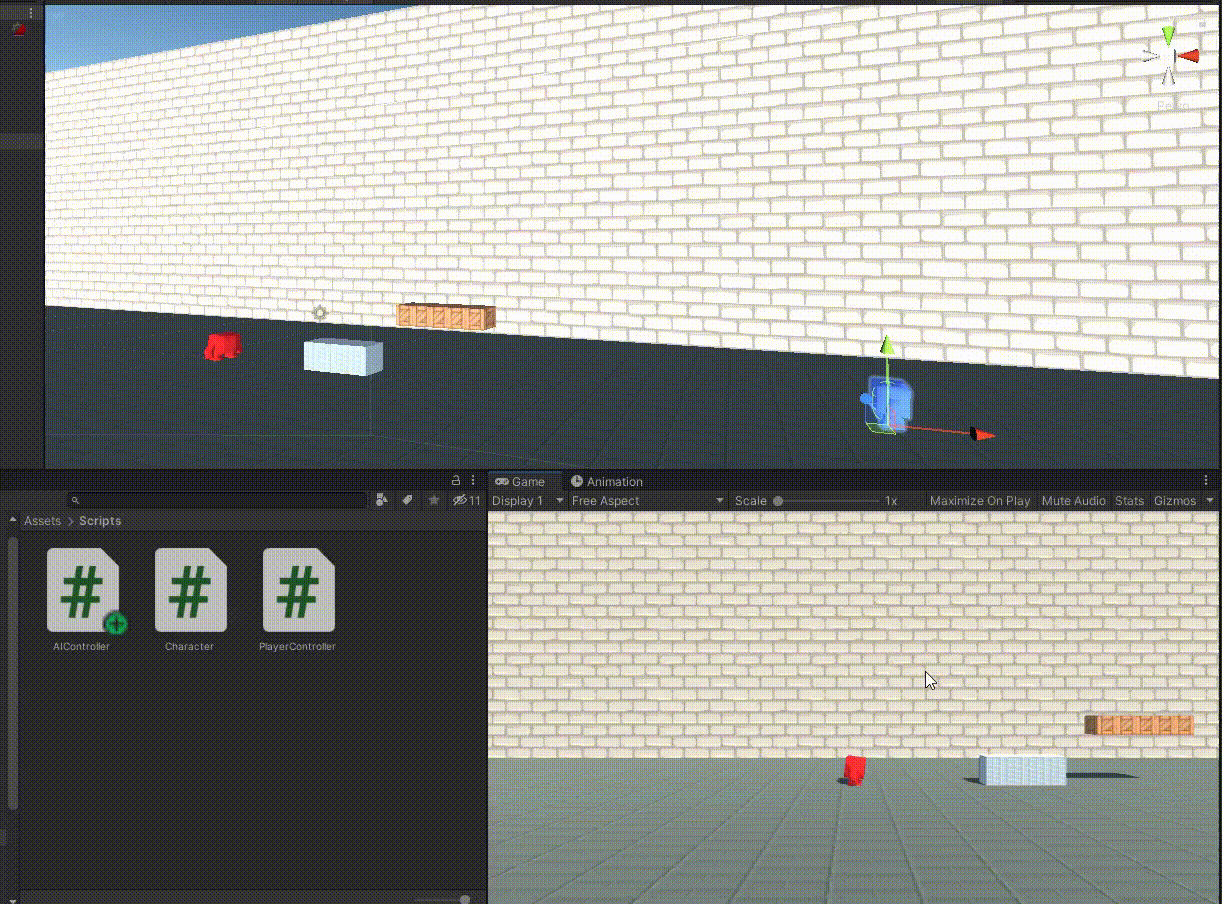
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class AIController : MonoBehaviour
{
Character character;
public float changeActTime = 2; //改变行动的时间间隔
public float jumpTime = 0.5f; //每隔0.5秒尝试跳跃
public float jumpChance = 30; //每次有30%概率跳跃
float inputX; //模拟横向输入 -1~1
bool jump = false;
void Start()
{
character = GetComponent<Character>();
StartCoroutine(ChangeAct());
StartCoroutine(AIJump());
}
void Update()
{
character.Move(inputX, jump);
jump = false;
}
IEnumerator ChangeAct()
{
while (true)
{
//改变inputX
inputX = Random.Range(-1f, 1f);
yield return new WaitForSeconds(changeActTime);
}
}
IEnumerator AIJump()
{
while (true)
{
int r = Random.Range(0, 100);
if(r < jumpChance)
{
jump = true;
}
yield return new WaitForSeconds(jumpTime);
}
}
}
踩踏攻击
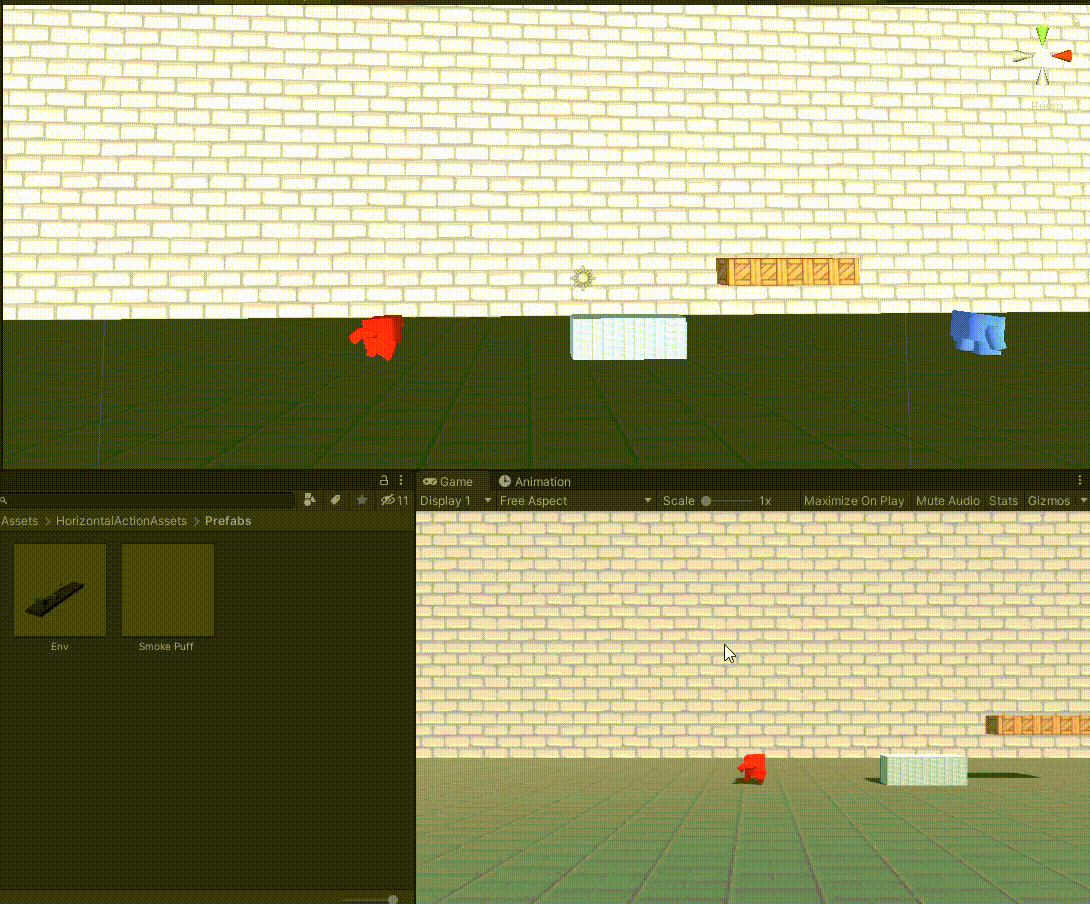
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Character : MonoBehaviour
{
CharacterController cc;
Animator animator;
Vector3 pendingVelocity; // 这一帧移动的向量
public float speed = 6;
public float jumpSpeed = 5;
bool isGround = false;
public GameObject deathParticle; //死亡粒子特效
void Start()
{
cc = GetComponent<CharacterController>();
animator = GetComponent<Animator>();
}
public void Move(float h,bool jump)
{
pendingVelocity.x = h * speed;
if (Mathf.Abs(h) > 0.1f)
{
//考虑旋转
Quaternion right = Quaternion.LookRotation(Vector3.forward);
Quaternion left = Quaternion.LookRotation(Vector3.back);
if (h > 0)
{
//往右转
transform.rotation = Quaternion.Slerp(transform.rotation, right, 0.05f);
}
else
{
//往左转
transform.rotation = Quaternion.Slerp(transform.rotation, left, 0.05f);
}
}
//处理跳跃逻辑
if (jump && isGround)
{
//正常跳跃
pendingVelocity.y = jumpSpeed;
isGround = false;
}
//考虑下落
if (!isGround)
{
pendingVelocity.y += Physics.gravity.y * 2 * Time.deltaTime;
}
else
{
pendingVelocity.y = 0;
}
cc.Move(pendingVelocity * Time.deltaTime);
//更新动画
UpdateAnim();
//踩踏攻击检测
AttackCheck();
}
private void FixedUpdate()
{
isGround = false;
Ray ray = new Ray(transform.position + new Vector3(0,0.2f,0),Vector3.down);
Vector3 start = transform.position + new Vector3(0, 0.2f, 0);
//Debug.DrawLine(start,start + new Vector3(0,-0.25f,0),Color.blue);
RaycastHit hit;
if(Physics.Raycast(ray,out hit, 0.25f, LayerMask.GetMask("Default")))
{
isGround = true;
}
Physics.Raycast(ray, out hit, 0.3f, LayerMask.GetMask("Default","Ground"));
}
void AttackCheck()
{
Ray ray = new Ray(transform.position + new Vector3(0, 0.2f, 0), Vector3.down);
RaycastHit hit;
if (Physics.Raycast(ray, out hit, 0.1f, LayerMask.GetMask("Cha")))
{
//被射线打中的物体,是hit.transform
Character cha = hit.transform.GetComponent<Character>();
cha.TakeDamage();
//反弹
Bounce();
}
}
GameObject temp;
public void TakeDamage()
{
Debug.Log(gameObject.name + "TakeDamage");
Destroy(gameObject);
GameObject go = Instantiate(deathParticle, transform.position, Quaternion.identity);
Destroy(go, 2); //2s后删除粒子
}
void Bounce()
{
pendingVelocity.y = jumpSpeed/2;
}
void UpdateAnim()
{
animator.SetFloat("Forward", cc.velocity.magnitude / speed);
animator.SetBool("IsGround", isGround);
}
private void OnCollisionEnter(Collision collision)
{
}
private void OnTriggerEnter(Collider other)
{
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class AIController : MonoBehaviour
{
Character character;
public float changeActTime = 2; //改变行动的时间间隔
public float jumpTime = 0.5f; //每隔0.5秒尝试跳跃
public float jumpChance = 30; //每次有30%概率跳跃
float inputX; //模拟横向输入 -1~1
bool jump = false;
void Start()
{
character = GetComponent<Character>();
StartCoroutine(ChangeAct());
StartCoroutine(AIJump());
}
void Update()
{
character.Move(inputX, jump);
jump = false;
}
IEnumerator ChangeAct()
{
while (true)
{
//改变inputX
inputX = Random.Range(-1f, 1f);
yield return new WaitForSeconds(changeActTime);
}
}
IEnumerator AIJump()
{
while (true)
{
int r = Random.Range(0, 100);
if(r < jumpChance)
{
jump = true;
}
yield return new WaitForSeconds(jumpTime);
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
Character character;
void Start()
{
character = GetComponent<Character>();
}
// Update is called once per frame
void Update()
{
float h = Input.GetAxis("Horizontal");
bool jump = Input.GetButtonDown("Jump");
character.Move(h,jump);
}
}