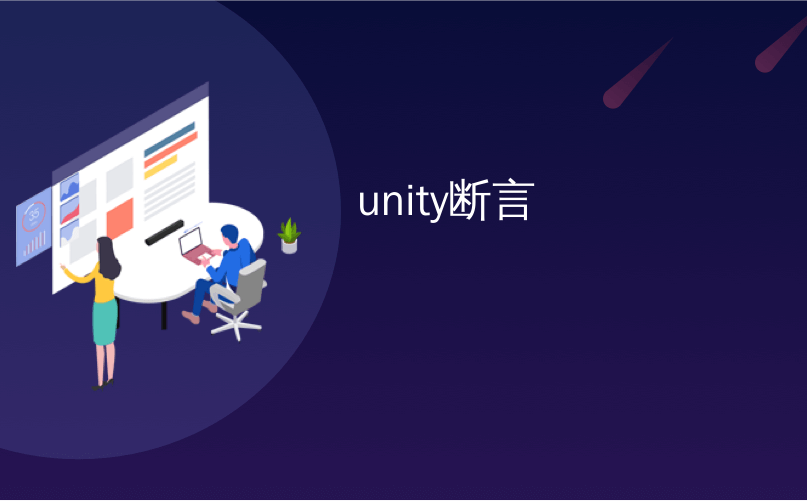
unity断言
Unity 5.1 shipped with a brand new Assertion Library. In this post, we will explain what an assertion is and how you can use it to improve runtime error diagnostics in your games.
Unity 5.1随附了全新的断言库。 在这篇文章中,我们将解释什么是断言,以及如何使用它来改善游戏中的运行时错误诊断。
什么是断言,我为什么要关心? (What is an assertion and why should I care?)
An assertion is a method that checks for a condition. If the condition is true, the method returns and the execution continues. If something unexpected happens and the assumption in the condition is not met, a message is printed that shows the callstack and optionally a user specified message. Let’s look at an example:
断言是一种检查条件的方法。 如果条件为true,则该方法返回并继续执行。 如果发生了意外情况,并且不满足该条件中的假设,则会显示一条消息,显示调用堆栈,并显示用户指定的消息(可选)。 让我们看一个例子:
|